Spring Cloud Discovery Eureka Client mit dem Beispiel verstehen
1. Das Zweck des Artikel
In einem verteilten System (Distributed System) sollen die Dienstleistungen (die Applikation) mit "Service Registration" registriert werden damit sie mit einander entdecken könnenIn den Artikel haben wir eine "Service Registration" benutzend die Techologie von Netflix (Spring Cloud Netflix Eureka Server) erstellt. Sie können die Unterricht nach den folgenden Pfad lernen:
OK, In dieser Unterricht werden wir diskussieren, wie Sie für eine Dienstleistung (die Applikation) in einem verteilten System konfigurieren um sie zu einem Eureka Client zu werden. D.h es wird mit "Serivce Registration" (Eureka Server) registriert.
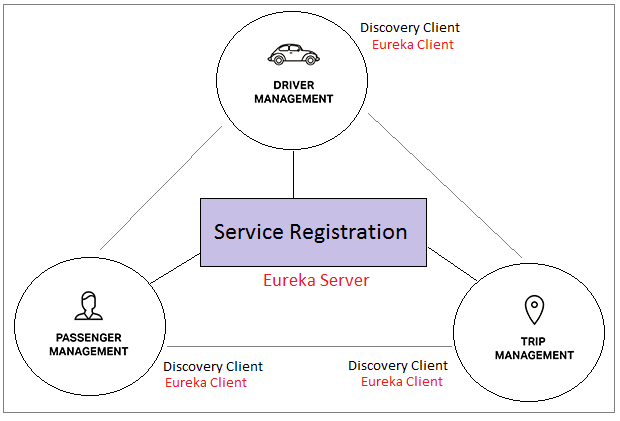
Die Eureka Client wird die Liste von anderen Eureka Client haben. Wir werden diese Liste mit der Kode Java manipulieren.
2. Das Projekt Spring Boot erstellen
Auf Eclipse erstellen Sie ein Projekt Spring Boot.
- Name: SpringCloudDiscoveryEurekaClient
- Group: org.o7planning
- Artifact: SpringCloudDiscoveryEurekaClient
- Description: Spring Cloud Discovery (Eureka Client)
- Package: org.o7planning.eurekaclient
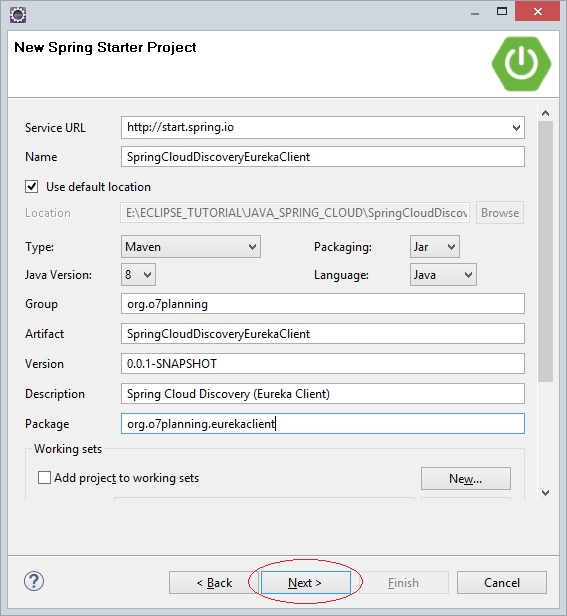
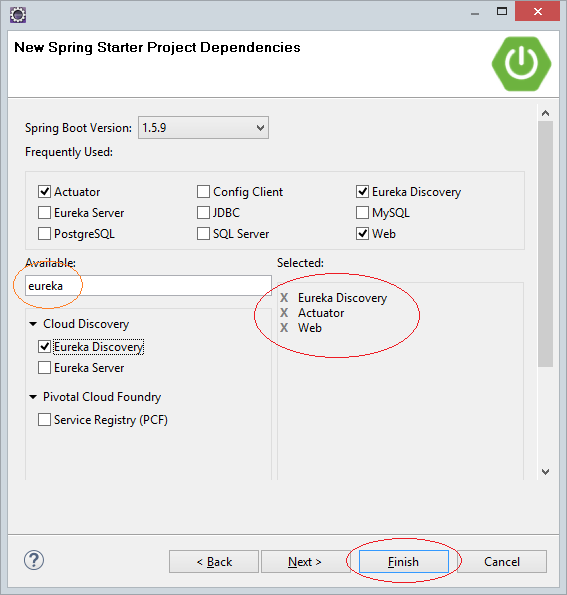
Das Projekt wurde erstellt
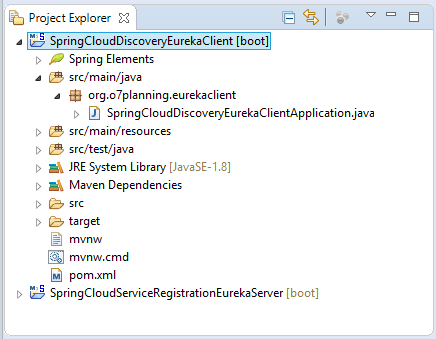
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>SpringCloudDiscoveryEurekaClient</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>SpringCloudDiscoveryEurekaClient</name>
<description>Spring Cloud Discovery (Eureka Client)</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>1.5.9.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
<spring-cloud.version>Edgware.RELEASE</spring-cloud.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-eureka</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-dependencies</artifactId>
<version>${spring-cloud.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
3. @EnableEurekaClient
@EnableEurekaClient verwenden um auf die Applikation zu annotieren (annotate), werden Sie diese Applikation zu einem Eureka Client zu wandeln.
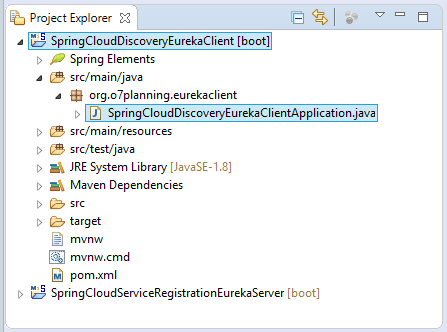
SpringCloudDiscoveryEurekaClientApplication.java
package org.o7planning.eurekaclient;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.netflix.eureka.EnableEurekaClient;
@EnableEurekaClient
@SpringBootApplication
public class SpringCloudDiscoveryEurekaClientApplication {
public static void main(String[] args) {
SpringApplication.run(SpringCloudDiscoveryEurekaClientApplication.class, args);
}
}
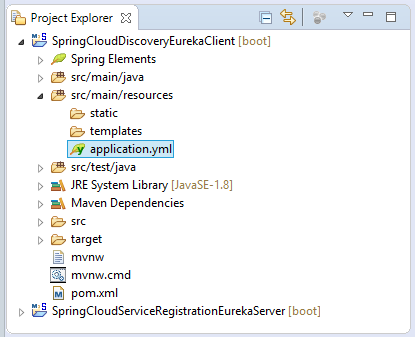
application.yml
spring:
application:
name: ABC-SERVICE # ==> This is Service-Id
---
# Items that apply to ALL profiles:
eureka:
instance:
appname: ABC-SERVICE # ==> This is a instance of ABC-SERVICE
client:
fetchRegistry: true
serviceUrl:
# defaultZone: http://my-eureka-server.com:9000/eureka
defaultZone: http://my-eureka-server-us.com:9001/eureka
# defaultZone: http://my-eureka-server-fr.com:9002/eureka
# defaultZone: http://my-eureka-server-vn.com:9003/eureka
server:
port: 8000
---
spring:
profiles: abc-service-replica01
eureka:
instance:
appname: ABC-SERVICE # ==> This is a instance of ABC-SERVICE
client:
fetchRegistry: true
serviceUrl:
defaultZone: http://my-eureka-server-us.com:9001/eureka
server:
port: 8001
---
spring:
profiles: abc-service-replica02
eureka:
instance:
appname: ABC-SERVICE # ==> This is a instance of ABC-SERVICE
client:
fetchRegistry: true
serviceUrl:
defaultZone: http://my-eureka-server-us.com:9001/eureka
server:
port: 8002
---
spring:
profiles: abc-service-replica03
eureka:
instance:
appname: ABC-SERVICE # ==> This is a instance of ABC-SERVICE
client:
fetchRegistry: true
serviceUrl:
defaultZone: http://my-eureka-server-us.com:9001/eureka
server:
port: 8003
---
spring:
profiles: abc-service-replica04
eureka:
instance:
appname: ABC-SERVICE # ==> This is a instance of ABC-SERVICE
client:
fetchRegistry: true
serviceUrl:
defaultZone: http://my-eureka-server-us.com:9001/eureka
server:
port: 8004
---
spring:
profiles: abc-service-replica05
eureka:
instance:
appname: ABC-SERVICE # ==> This is a instance of ABC-SERVICE
client:
fetchRegistry: true
serviceUrl:
defaultZone: http://my-eureka-server-us.com:9001/eureka
server:
port: 8005
4. Controller
Wenn Eureka Client mit Eureka Server (Service Registration) registriert, kann es die Liste von anderen mit dem Eureka Server registrierten Eureka Client
@Autowired
private DiscoveryClient discoveryClient;
...
// Get All Service Ids
List<String> serviceIds = this.discoveryClient.getServices();
// (Need!!) eureka.client.fetchRegistry=true
List<ServiceInstance> instances = this.discoveryClient.getInstances(serviceId);
for (ServiceInstance serviceInstance : instances) {
System.out.println("URI: " + serviceInstance.getUri();
System.out.println("Host: " + serviceInstance.getHost();
System.out.println("Port: " + serviceInstance.getPort();
}
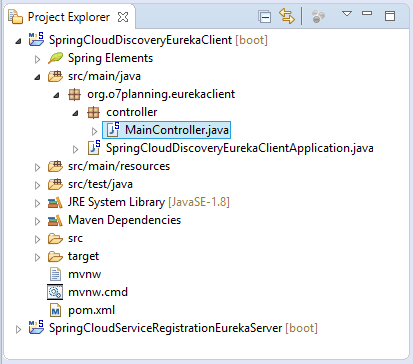
MainController.java
package org.o7planning.eurekaclient.controller;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.cloud.client.ServiceInstance;
import org.springframework.cloud.client.discovery.DiscoveryClient;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class MainController {
@Autowired
private DiscoveryClient discoveryClient;
@ResponseBody
@RequestMapping(value = "/", method = RequestMethod.GET)
public String home() {
return "<a href='showAllServiceIds'>Show All Service Ids</a>";
}
@ResponseBody
@RequestMapping(value = "/showAllServiceIds", method = RequestMethod.GET)
public String showAllServiceIds() {
List<String> serviceIds = this.discoveryClient.getServices();
if (serviceIds == null || serviceIds.isEmpty()) {
return "No services found!";
}
String html = "<h3>Service Ids:</h3>";
for (String serviceId : serviceIds) {
html += "<br><a href='showService?serviceId=" + serviceId + "'>" + serviceId + "</a>";
}
return html;
}
@ResponseBody
@RequestMapping(value = "/showService", method = RequestMethod.GET)
public String showFirstService(@RequestParam(defaultValue = "") String serviceId) {
// (Need!!) eureka.client.fetchRegistry=true
List<ServiceInstance> instances = this.discoveryClient.getInstances(serviceId);
if (instances == null || instances.isEmpty()) {
return "No instances for service: " + serviceId;
}
String html = "<h2>Instances for Service Id: " + serviceId + "</h2>";
for (ServiceInstance serviceInstance : instances) {
html += "<h3>Instance: " + serviceInstance.getUri() + "</h3>";
html += "Host: " + serviceInstance.getHost() + "<br>";
html += "Port: " + serviceInstance.getPort() + "<br>";
}
return html;
}
// A REST method, To call from another service.
// See in Lesson "Load Balancing with Ribbon".
@ResponseBody
@RequestMapping(value = "/hello", method = RequestMethod.GET)
public String hello() {
return "<html>Hello from ABC-SERVICE</html>";
}
}
5. Die Applikation auf die Eclipse laufen
Zuerst sollen Sie sicherstellen, dass Sie Eureka Server durchgeführt haben:
See the previous lesson to run an Eureka Server:
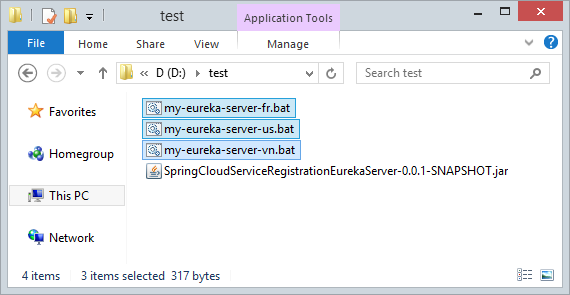
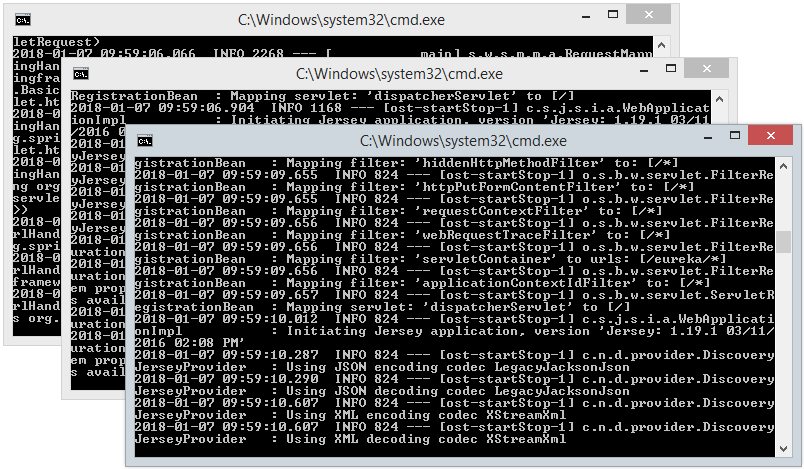
Danach können Sie auf die Eclipse Eureka Client direkt laufen. Es wird mit dem Eureka Server registriert.
Greifen Sie in die folgenden URL zu und Sie können die mit dem Eureka Server registrierten Eureka Client sehen
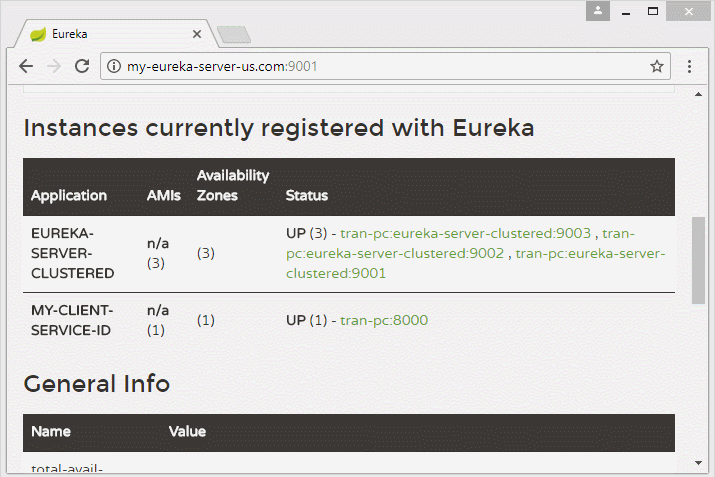
6. Die Kopien (replica) laufen
Benutzen Sie die Funktion "Maven Install" um die File jar aus Projekt zu erstellen. Klicken Sie die Rechtmaustaste auf Projekt und wählen
- Run As/Maven Install
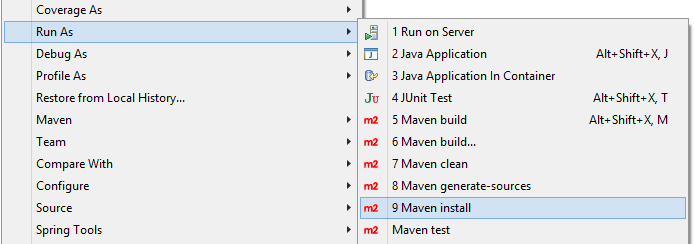
Und Sie haben eine File jar in dem Verzeichnis target vom Projekt
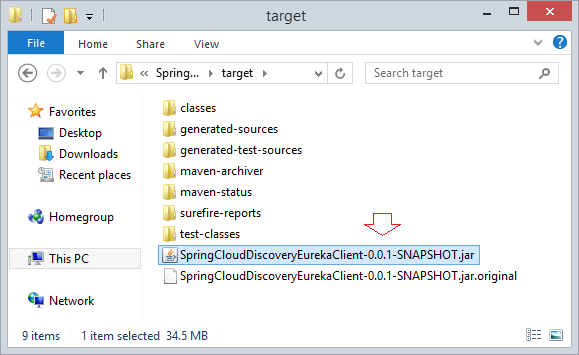
Die erstellte File jar in einem Verzeichnis kopieren und erstellen Sie 2 File BAT:
- abc-service-replica01.bat
- abc-service-replica02.bat
- abc-service-replica03.bat
- abc-service-replica04.bat
- abc-service-replica05.bat
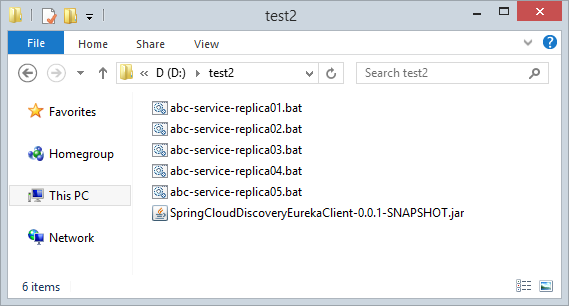
abc-service-replica01.bat
java -jar -Dspring.profiles.active=abc-service-replica01 SpringCloudDiscoveryEurekaClient-0.0.1-SNAPSHOT.jar
abc-service-replica02.bat
java -jar -Dspring.profiles.active=abc-service-replica02 SpringCloudDiscoveryEurekaClient-0.0.1-SNAPSHOT.jar
abc-service-replica03.bat
java -jar -Dspring.profiles.active=abc-service-replica03 SpringCloudDiscoveryEurekaClient-0.0.1-SNAPSHOT.jar
abc-service-replica04.bat
java -jar -Dspring.profiles.active=abc-service-replica04 SpringCloudDiscoveryEurekaClient-0.0.1-SNAPSHOT.jar
abc-service-replica05.bat
java -jar -Dspring.profiles.active=abc-service-replica05 SpringCloudDiscoveryEurekaClient-0.0.1-SNAPSHOT.jar
Die 2 File BAT laufen:
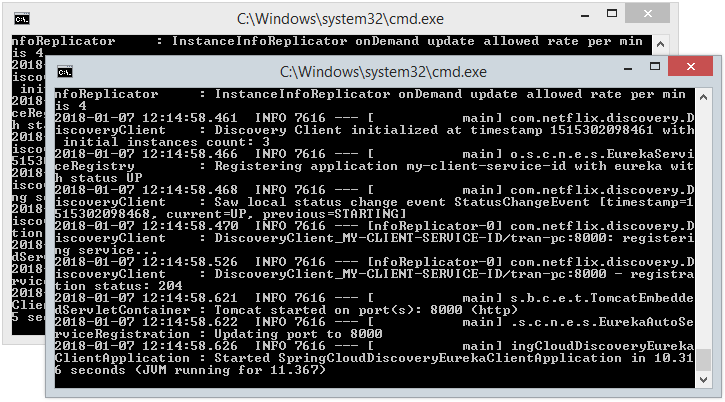
Auf Eureka Monitor können Sie die Eureka Client , die mit dem Eureka Server registriert werden
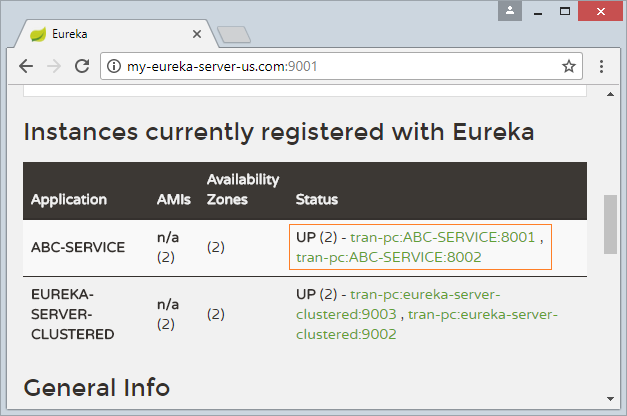
7. Test Discovery
OK, Nach dem Laufen vom Eureka Client könne Sie sehen, wie es die anderen Eureka Client entdeckt
Greifen Sie in die folgenden URL zu (Achtung: Warten Sie 30 Sekundären um sicherzustellen, dass Eureka Server und Eureka Client die Status von einander schon aktuellisiert werden
- http://localhost:8000 (If Eureka Client run from Eclipse)
- http://localhost:8001 (If Eureka Client run from BAT file)
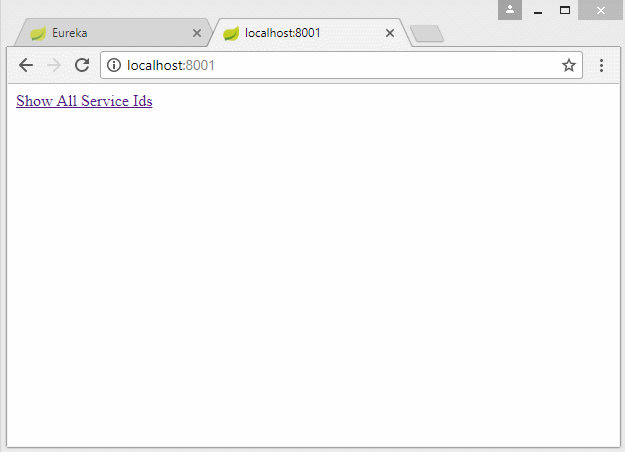
Anleitungen Spring Cloud
- Was ist Cloud Computing?
- Einführung in Netflix und seine Cloud-Computing-Technologie
- Einführung in Spring Cloud
- Spring Cloud Config Server mit dem Beispiel verstehen
- Spring Cloud Config Client mit dem Beispiel verstehen
- Spring Cloud Eureka Server mit dem Beispiel verstehen
- Spring Cloud Discovery Eureka Client mit dem Beispiel verstehen
- Load Balancing in Spring Cloud mit Ribbon und Beispiel verstehen
Show More