- NavigableMap
- NavigableMap Methods
- Examples
- descendingMap()
- navigableKeySet()
- descendingKeySet()
- subMap(K, boolean, K, boolean)
- headMap(K, boolean)
- tailMap(K, boolean)
- firstEntry()
- lastEntry()
- pollFirstEntry()
- pollLastEntry()
- lowerKey(K key)
- lowerEntry(K key)
- floorKey(K key)
- floorEntry(K key)
- ceilingKey(K key)
- ceilingEntry(K key)
- higherKey(K key)
- higherEntry(K key)
Die Anleitung zu Java NavigableMap
1. NavigableMap
NavigableMap ist eine Unterinterface der Interface SortedMap, deshalb funktioniert es also wie eine SortedMap. Darüber hinaus wird es durch Methoden ergänzt, die Navigation, Schlüsselsuche und Mappings ermöglichen.
NavigableMap ermöglicht beispielsweise das Navigieren in aufsteigender oder absteigender Reihenfolge von Schlüsseln und bietet Methoden wie lowerKey, floorKey, CeilingKey, HigherKey, LowerEntry, floorEntry, CeilingEntry, HighEntry.., um nach Schlüsseln und Mappings zu suchen.
public interface NavigableMap<K,V> extends SortedMap<K,V>
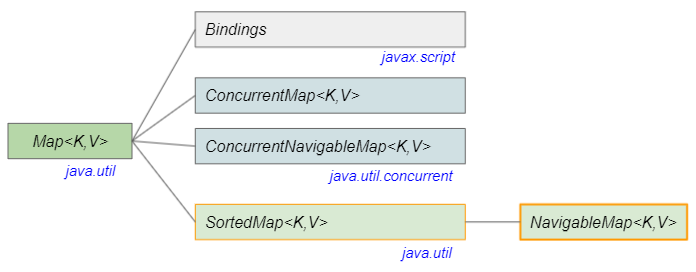
Map<K,V> | SortedMap<K,V> NavigableMap<K,V> |
Doppelte Schlüssel sind nicht erlaubt. | |
Kann Schlüssel null und die Werte null zulassen. | |
Die Reihenfogle der Schlüssel wird nicht garantiert. | Dei Schlüssel werden in aufsteigender Reihenfolge nach ihrer natütlichen Reihenfolge oder nach einem bereitgestellten Comparator sortiert. |
Alle Schlüssel von NavigableMap/SortedMap müssen von Typ Comparable (vergleichbar) sein oder Sie müssen einenComparator fürNavigableMap/SortedMap bereitstellen, damit es Schlüssel vergleicht. Andernfalls wird ClassCastException ausgelöst.
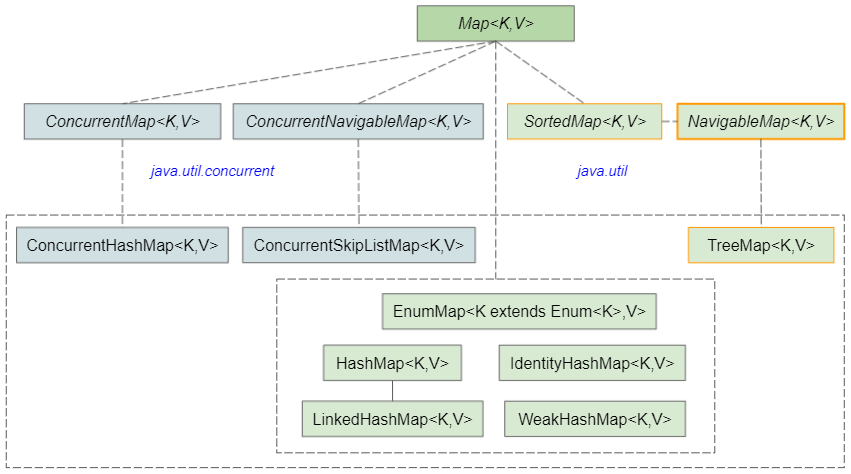
- TreeMap
- HashMap
- IdentityHashMap
- WeakHashMap
- LinkedHashMap
- EnumMap
- ConcurrentHashMap
- ConcurrentSkipListMap
2. NavigableMap Methods
Map.Entry<K,V> firstEntry();
Map.Entry<K,V> lastEntry();
Map.Entry<K,V> pollFirstEntry();
Map.Entry<K,V> pollLastEntry();
Map.Entry<K,V> lowerEntry(K key);
Map.Entry<K,V> floorEntry(K key);
Map.Entry<K,V> ceilingEntry(K key);
Map.Entry<K,V> higherEntry(K key);
K lowerKey(K key);
K floorKey(K key);
K ceilingKey(K key);
K higherKey(K key);
NavigableMap<K,V> descendingMap();
NavigableSet<K> navigableKeySet();
NavigableSet<K> descendingKeySet();
NavigableMap<K,V> subMap(K fromKey, boolean fromInclusive,
K toKey, boolean toInclusive);
NavigableMap<K,V> headMap(K toKey, boolean inclusive);
NavigableMap<K,V> tailMap(K fromKey, boolean inclusive);
// Inherited from SortedSet:
SortedMap<K,V> subMap(K fromKey, K toKey);
SortedMap<K,V> headMap(K toKey);
SortedMap<K,V> tailMap(K fromKey);
...
3. Examples
Z.B: Ein Objekt NavigableMap<Integer,String> enthätlt die Mappings zwischen dem Jahr der World Cup und dem Gastgeberland. Wir verwenden einige seiner Methoden um zu navigieren, Schlüssel und Mapping nach Bedarf zu finden.
NavigableMapEx1.java
package org.o7planning.navigablemap.ex;
import java.util.Map;
import java.util.NavigableMap;
import java.util.TreeMap;
public class NavigableMapEx1 {
public static void main(String[] args) {
// Map: World Cup Year --> Country Name
NavigableMap<Integer, String> worldCupMap = new TreeMap<>();
worldCupMap.put(1934, "Italy");
worldCupMap.put(1930, "Uruguay");
worldCupMap.put(1970, "Mexico");
worldCupMap.put(1966, "England");
worldCupMap.put(1962, "Chile");
worldCupMap.put(1958, "Sweden");
worldCupMap.put(1954, "Switzerland");
worldCupMap.put(1950, "Brazil");
worldCupMap.put(1938, "France");
System.out.println("--- World Cup Map ---:");
printMap(worldCupMap);
// Get a reverse view of the navigable map.
NavigableMap<Integer, String> reverseMap = worldCupMap.descendingMap();
System.out.println("\n--- World Cup Map (Reverse View) ---:");
printMap(reverseMap);
// World Cup Map (Year >= 1935)
NavigableMap<Integer, String> tailMap1 = worldCupMap.tailMap(1935, true);
System.out.println("\n--- World Cup Map (Year >= 1935) ---:");
printMap(tailMap1);
// World Cup Map (Year <= 1958)
NavigableMap<Integer, String> headMap1 = worldCupMap.headMap(1938, true);
System.out.println("\n--- World Cup Map (Year <= 1938) ---:");
printMap(headMap1);
// The first year of the World Cup after 1938.
int year1 = worldCupMap.higherKey(1938);
System.out.printf("%nThe first year of the World Cup after 1938: %d%n", year1);
// The last year of the World Cup before 1950.
int year2 = worldCupMap.lowerKey(1950);
System.out.printf("%nThe last year of the World Cup before 1950: %d%n", year2);
// The first World Cup after 1938.
Map.Entry<Integer, String> e1 = worldCupMap.higherEntry(1938);
System.out.printf("%nThe first World Cup after 1938: %d --> %s%n", e1.getKey(), e1.getValue());
// The last World Cup before 1950.
Map.Entry<Integer, String> e2 = worldCupMap.lowerEntry(1950);
System.out.printf("%nThe last World Cup before 1950: %d --> %s%n", e2.getKey(), e2.getValue());
}
private static void printMap(Map<Integer, String> map) {
for (Map.Entry<Integer, String> entry : map.entrySet()) {
System.out.println(entry.getKey() + " --> " + entry.getValue());
}
}
}
Output:
--- World Cup Map ---:
1930 --> Uruguay
1934 --> Italy
1938 --> France
1950 --> Brazil
1954 --> Switzerland
1958 --> Sweden
1962 --> Chile
1966 --> England
1970 --> Mexico
--- World Cup Map (Reverse View) ---:
1970 --> Mexico
1966 --> England
1962 --> Chile
1958 --> Sweden
1954 --> Switzerland
1950 --> Brazil
1938 --> France
1934 --> Italy
1930 --> Uruguay
--- World Cup Map (Year >= 1935) ---:
1938 --> France
1950 --> Brazil
1954 --> Switzerland
1958 --> Sweden
1962 --> Chile
1966 --> England
1970 --> Mexico
--- World Cup Map (Year <= 1938) ---:
1930 --> Uruguay
1934 --> Italy
1938 --> France
The first year of the World Cup after 1938: 1950
The last year of the World Cup before 1950: 1938
The first World Cup after 1938: 1950 --> Brazil
The last World Cup before 1950: 1938 --> France
Weitere Beispiel für die Verwendung von Comparator für NavigableMap/SortedMap:
4. descendingMap()
NavigableMap<K,V> descendingMap();
Gibt eine Ansicht in umgekehrter Reihenfolge der in dieser NavigableMap enthaltenen Mapping zurück. Das zurückgegebeneNavigableMap wird in absteigender Reihenfolge nach Schlüssel sortiert.
Die zurückgegebene NavigableMap bezieht sich auf die aktuelle NavigableMap. Die Änderungen an einer NavigableMap wirken sich auf die andere NavigableMap aus und umgekehrt.
NavigableMap_descendingMap_ex1.java
package org.o7planning.navigablemap.ex;
import java.util.Map;
import java.util.NavigableMap;
import java.util.TreeMap;
public class NavigableMap_descendingMap_ex1 {
public static void main(String[] args) {
// Map: World Cup Year --> Country Name
NavigableMap<Integer, String> worldCupMap = new TreeMap<>();
worldCupMap.put(1934, "Italy");
worldCupMap.put(1930, "Uruguay");
worldCupMap.put(1970, "Mexico");
worldCupMap.put(1966, "England");
worldCupMap.put(1962, "Chile");
worldCupMap.put(1958, "Sweden");
worldCupMap.put(1954, "Switzerland");
worldCupMap.put(1950, "Brazil");
worldCupMap.put(1938, "France");
System.out.println("--- World Cup Map ---:");
printMap(worldCupMap);
// Get a reverse view of the navigable map.
NavigableMap<Integer, String> reverseMap = worldCupMap.descendingMap();
System.out.println("\n--- World Cup Map (Reverse View) ---:");
printMap(reverseMap);
}
private static void printMap(Map<Integer, String> map) {
// Java 8 Syntax:
map.entrySet().stream().forEach(entry -> {
System.out.println(entry.getKey() + " --> " + entry.getValue());
});
}
}
Output:
--- World Cup Map ---:
1930 --> Uruguay
1934 --> Italy
1938 --> France
1950 --> Brazil
1954 --> Switzerland
1958 --> Sweden
1962 --> Chile
1966 --> England
1970 --> Mexico
--- World Cup Map (Reverse View) ---:
1970 --> Mexico
1966 --> England
1962 --> Chile
1958 --> Sweden
1954 --> Switzerland
1950 --> Brazil
1938 --> France
1934 --> Italy
1930 --> Uruguay
5. navigableKeySet()
NavigableSet<K> navigableKeySet();
Gibt eine Ansicht NavigableSet der in dieser NavigableMap enthaltenen Schlüssel zurück.
Die zurückgegebene NavigableMap bezieht sich auf die aktuelle NavigableMap. Änderungen an einer NavigableMap wirken sich auf die andere NavigableMap aus und umgekehrt.
- Durch das Hinzufügen oder Entfernen einer Mapping zu NavigableMap wird ein Element zu NavigableSet hinzugefügt oder daraus entfernt..
- Methoden zum Entfernen von Elementen aus NavigableSet wie Set.iterator().remove, Set.remove, Set.removeAll, Set.retainAll, Set.clear.. entfernen die entsprechenden Zuordnungen aus NavigableMap..
- Dieses Objekt NavigableSet unterstützt keine Operation Set.add, Set.addAll.
NavigableMap_navigableKeySet_ex1.java
package org.o7planning.navigablemap.ex;
import java.util.Map;
import java.util.NavigableMap;
import java.util.NavigableSet;
import java.util.TreeMap;
public class NavigableMap_navigableKeySet_ex1 {
public static void main(String[] args) {
// Map: World Cup Year --> Country Name
NavigableMap<Integer, String> worldCupMap = new TreeMap<>();
worldCupMap.put(1934, "Italy");
worldCupMap.put(1930, "Uruguay");
worldCupMap.put(1970, "Mexico");
worldCupMap.put(1966, "England");
worldCupMap.put(1962, "Chile");
worldCupMap.put(1958, "Sweden");
worldCupMap.put(1954, "Switzerland");
worldCupMap.put(1950, "Brazil");
worldCupMap.put(1938, "France");
System.out.println("--- World Cup Map ---:");
printMap(worldCupMap);
NavigableSet<Integer> navigableSetYears = worldCupMap.navigableKeySet();
System.out.println("\nYears: " + navigableSetYears);
// Remove some years from NavigableSet navigableSetYears
navigableSetYears.remove(1954);
navigableSetYears.remove(1950);
navigableSetYears.remove(1938);
navigableSetYears.remove(1934);
navigableSetYears.remove(1930);
System.out.println("\n--- World Cup Map (After removing some years from NavigableSet) ---:");
printMap(worldCupMap);
}
private static void printMap(Map<Integer, String> map) {
// Java 8 Syntax:
map.entrySet().stream().forEach(entry -> {
System.out.println(entry.getKey() + " --> " + entry.getValue());
});
}
}
Output:
--- World Cup Map ---:
1930 --> Uruguay
1934 --> Italy
1938 --> France
1950 --> Brazil
1954 --> Switzerland
1958 --> Sweden
1962 --> Chile
1966 --> England
1970 --> Mexico
Years: [1930, 1934, 1938, 1950, 1954, 1958, 1962, 1966, 1970]
--- World Cup Map (After removing some years from NavigableSet) ---:
1958 --> Sweden
1962 --> Chile
1966 --> England
1970 --> Mexico
6. descendingKeySet()
NavigableSet<K> descendingKeySet();
Gibt eine Ansicht NavigableSet in umgekehrter Reihenfolge der in dieser NavigableMap enthaltenen Schlüssel zurück. Entspricht dem Aufrufen von descendingMap().navigableKeySet().
Die zurückgegebene NavigableMap bezieht sich auf die aktuelle NavigableMap. Änderungen an einer NavigableMap wirken sich auf die andere NavigableMap aus und umgekehrt.
- Durch das Hinzufügen oder Entfernen einer Mapping zu NavigableMap wird ein Element zu NavigableSet hinzugefügt oder daraus entfernt.
- Die Methoden zum Entfernen von Elementen aus NavigableSet wie Set.iterator().remove, Set.remove, Set.removeAll, Set.retainAll, Set.clear.. entfernen die entsprechenden Mapping aus NavigableMap.
- Dieses Objekt NavigableSet unterstützt die Operation Set.add, Set.addAll nicht.
NavigableMap_descendingKeySet_ex1.java
package org.o7planning.navigablemap.ex;
import java.util.Iterator;
import java.util.Map;
import java.util.NavigableMap;
import java.util.NavigableSet;
import java.util.TreeMap;
public class NavigableMap_descendingKeySet_ex1 {
public static void main(String[] args) {
// Map: World Cup Year --> Country Name
NavigableMap<Integer, String> worldCupMap = new TreeMap<>();
worldCupMap.put(1934, "Italy");
worldCupMap.put(1930, "Uruguay");
worldCupMap.put(1970, "Mexico");
worldCupMap.put(1966, "England");
worldCupMap.put(1962, "Chile");
worldCupMap.put(1958, "Sweden");
worldCupMap.put(1954, "Switzerland");
worldCupMap.put(1950, "Brazil");
worldCupMap.put(1938, "France");
System.out.println("--- World Cup Map ---:");
printMap(worldCupMap);
NavigableSet<Integer> descendingKeySetYears = worldCupMap.descendingKeySet();
System.out.println("\nDescending Years: " + descendingKeySetYears);
// Remove some years from NavigableSet descendingKeySetYears
Iterator<Integer> iterator = descendingKeySetYears.iterator();
while(iterator.hasNext()) {
Integer year = iterator.next();
if(year <= 1954) {
iterator.remove();
}
}
System.out.println("\n--- World Cup Map (After removing some years from NavigableSet) ---:");
printMap(worldCupMap);
}
private static void printMap(Map<Integer, String> map) {
// Java 8 Syntax:
map.entrySet().stream().forEach(entry -> {
System.out.println(entry.getKey() + " --> " + entry.getValue());
});
}
}
Output:
--- World Cup Map ---:
1930 --> Uruguay
1934 --> Italy
1938 --> France
1950 --> Brazil
1954 --> Switzerland
1958 --> Sweden
1962 --> Chile
1966 --> England
1970 --> Mexico
Descending Years: [1970, 1966, 1962, 1958, 1954, 1950, 1938, 1934, 1930]
--- World Cup Map (After removing some years from NavigableSet) ---:
1958 --> Sweden
1962 --> Chile
1966 --> England
1970 --> Mexico
7. subMap(K, boolean, K, boolean)
NavigableMap<K,V> subMap(K fromKey, boolean fromInclusive,
K toKey, boolean toInclusive);
Gibt eine Ansicht eines Teils dieser NavigableMap zurück, die Mappings mit Schlüsselvon fromKey bis toKey enthält. Schließen Sie fromKey ein, wenn fromInclusivetrue ist, und schließen Sie toKey ein wenn toInclusivetrue ist.
Die zurückgegebene NavigableMap bezieht sich auf die aktuelle NavigableMap. Änderungen an einer NavigableMap wirken sich auf die andere NavigableMap aus und umgekehrt.
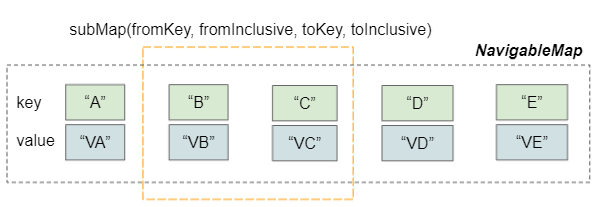
NavigableMap_subMap_ex1.java
package org.o7planning.navigablemap.ex;
import java.util.Map;
import java.util.NavigableMap;
import java.util.TreeMap;
public class NavigableMap_subMap_ex1 {
public static void main(String[] args) {
NavigableMap<String, String> myMap = new TreeMap<>();
myMap.put("A", "VA");
myMap.put("B", "VB");
myMap.put("C", "VC");
myMap.put("D", "VD");
myMap.put("E", "VE");
System.out.println(" -- myMap --");
printMap(myMap);
// A Sub Map ("B" <= key <= "C1")
NavigableMap<String, String> subMap = myMap.subMap("B", true, "C1", true);
System.out.println("\n -- subMap --");
printMap(subMap);
subMap.put("B1", "VB1");
subMap.put("B2", "VB2");
System.out.println("\n -- subMap (after putting some mappings to subMap) --");
printMap(subMap);
System.out.println("\n -- myMap (after putting some mappings to subMap) --");
printMap(myMap);
}
private static void printMap(Map<String, String> map) {
for (String s : map.keySet()) {
System.out.println(s + " --> " + map.get(s));
}
}
}
Output:
-- myMap --
A --> VA
B --> VB
C --> VC
D --> VD
E --> VE
-- subMap --
B --> VB
C --> VC
-- subMap (after putting some mappings to subMap) --
B --> VB
B1 --> VB1
B2 --> VB2
C --> VC
-- myMap (after putting some mappings to subMap) --
A --> VA
B --> VB
B1 --> VB1
B2 --> VB2
C --> VC
D --> VD
E --> VE
8. headMap(K, boolean)
NavigableMap<K,V> headMap(K toKey, boolean inclusive);
Gibt eine Ansicht eines Teils dieser NavigableMap zurück, einschließlich Mappings, deren Schlüssel kleiner als (oder gleich, wenn inclusive ist true) toKey ist.
Die zurückgegebene NavigableMap bezieht sich auf die aktuelle NavigableMap. Änderungen an einer NavigableMap wirken sich auf die andere NavigableMap aus und umgekehrt.
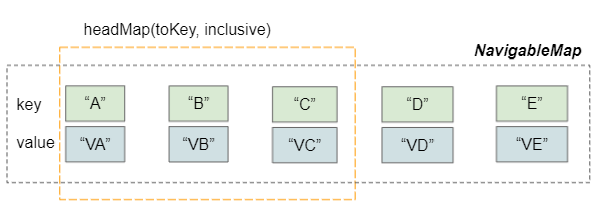
9. tailMap(K, boolean)
NavigableMap<K,V> tailMap(K fromKey, boolean inclusive);
Gibt eine Ansicht eines Teils dieser NavigableMap zurück, einschließlich Mapping, deren Schlüssel größer als (oder gleich, wenn inclusive is true) fromKey ist.
Die zurückgegebene NavigableMap bezieht sich auf die aktuelle NavigableMap. Änderungen an einer NavigableMap wirken sich auf die andere NavigableMap aus und umgekehrt.
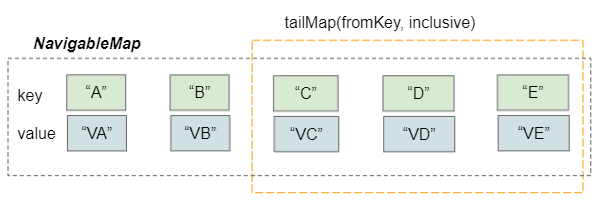
NavigableMap_tailMap_ex1.java
package org.o7planning.navigablemap.ex;
import java.util.Map;
import java.util.NavigableMap;
import java.util.TreeMap;
public class NavigableMap_tailMap_ex1 {
public static void main(String[] args) {
NavigableMap<String, String> myMap = new TreeMap<>();
myMap.put("A", "VA");
myMap.put("B", "VB");
myMap.put("C", "VC");
myMap.put("D", "VD");
myMap.put("E", "VE");
System.out.println(" -- myMap --");
printMap(myMap);
// A Tail Map (key >= "C")
NavigableMap<String, String> tailMap = myMap.tailMap("C", true);
System.out.println("\n -- tailMap --");
printMap(tailMap);
myMap.put("B1", "VB1");
myMap.put("D1", "VD1");
System.out.println("\n -- myMap (after putting some mappings to myMap) --");
printMap(myMap);
System.out.println("\n -- tailMap (after putting some mappings to myMap) --");
printMap(tailMap);
}
private static void printMap(Map<String, String> map) {
for (String s : map.keySet()) {
System.out.println(s + " --> " + map.get(s));
}
}
}
Output:
-- myMap --
A --> VA
B --> VB
C --> VC
D --> VD
E --> VE
-- tailMap --
C --> VC
D --> VD
E --> VE
-- myMap (after putting some mappings to myMap) --
A --> VA
B --> VB
B1 --> VB1
C --> VC
D --> VD
D1 --> VD1
E --> VE
-- tailMap (after putting some mappings to myMap) --
C --> VC
D --> VD
D1 --> VD1
E --> VE
10. firstEntry()
Map.Entry<K,V> firstEntry();
Gibt die erste Mapping (mit dem kleinsten Schlüssel) in dieser NavigableMap zurück oder null, wenn die NavigableMap leer ist.
11. lastEntry()
Map.Entry<K,V> lastEntry();
Gibt die letzte Zuordnung (mit dem größten Schlüssel) in dieser NavigableMap zurück oder null, wenn die NavigableMap leer ist.
12. pollFirstEntry()
Map.Entry<K,V> pollFirstEntry();
Entfernen Sie die erste Mapping in dieser NavigableMap und geben Sie sie zurück, oder null, wenn die NavigableMap leer ist.
13. pollLastEntry()
Map.Entry<K,V> pollLastEntry();
Entfernen Sie die letzte Mapping in dieser NavigableMap und geben Sie sie zurück, oder null, wenn die NavigableMap leer ist.
14. lowerKey(K key)
K lowerKey(K key);
Gibt den größten Schlüssel in dieser NavigableMap zurück, aber kleiner als der angegebene Schlüssel, oder null, wenn kein solcher Schlüssel vorhanden ist.
15. lowerEntry(K key)
Map.Entry<K,V> lowerEntry(K key);
Gibt die Mapping mit dem größten Schlüssel in dieser NavigableMap zurück, aber kleiner als der angegebene Schlüssel, oder null, wenn keine solche Mapping vorhanden ist.
16. floorKey(K key)
No ADS
K floorKey(K key);
Gibt den größten Schlüssel in dieser NavigableMap zurück, aber kleiner oder gleich dem angegebenen Schlüssel, oder null, wenn es keinen solchen Schlüssel gibt.
17. floorEntry(K key)
Map.Entry<K,V> floorEntry(K key);
Gibt die Mapping mit dem kleinsten Schlüssel in dieser NavigableMap zurück, der jedoch größer oder gleich dem angegebenen Schlüssel ist, oder null, wenn keine solche Mapping vorhanden ist.
18. ceilingKey(K key)
No ADS
K ceilingKey(K key);
Gibt den größten Schlüssel in dieser NavigableMap zurück, aber kleiner oder gleich dem angegebenen Schlüssel, oder null, wenn kein solcher Schlüssel vorhanden ist.
19. ceilingEntry(K key)
Map.Entry<K,V> ceilingEntry(K key);
Gibt die Mapping mit dem kleinsten Schlüssel in dieser NavigableMap zurück, der jedoch größer oder gleich dem angegebenen Schlüssel ist, oder null, wenn keine solche Mapping vorhanden ist.
No ADS
Die Anleitungen Java Collections Framework
- Die Anleitung zu Java PriorityBlockingQueue
- Die Anleitung zu Java Collections Framework
- Die Anleitung zu Java SortedSet
- Die Anleitung zu Java List
- Die Anleitung zu Java Iterator
- Die Anleitung zu Java NavigableSet
- Die Anleitung zu Java ListIterator
- Die Anleitung zu Java ArrayList
- Die Anleitung zu Java CopyOnWriteArrayList
- Die Anleitung zu Java LinkedList
- Die Anleitung zu Java Set
- Die Anleitung zu Java TreeSet
- Die Anleitung zu Java CopyOnWriteArraySet
- Die Anleitung zu Java Queue
- Die Anleitung zu Java Deque
- Die Anleitung zu Java IdentityHashMap
- Die Anleitung zu Java WeakHashMap
- Die Anleitung zu Java Map
- Die Anleitung zu Java SortedMap
- Die Anleitung zu Java NavigableMap
- Die Anleitung zu Java HashMap
- Die Anleitung zu Java TreeMap
- Die Anleitung zu Java PriorityQueue
- Die Anleitung zu Java BlockingQueue
- Die Anleitung zu Java ArrayBlockingQueue
- Die Anleitung zu Java TransferQueue
Show More