Die Anleitung zu Python Dictionary
1. Python Dictionary
Im Python ist das Wörterbuch (dictionary) ein Datentyp. Es ist eine Liste der Elemente, und jede Elemente ein Paar von Schlüssel und Wert (Key & value). Es is so ähnlich wie der Begriff von Map im Java.
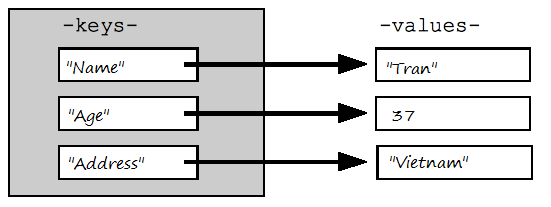
Die Wörterbücher sind die Objekt der Klasse dict.
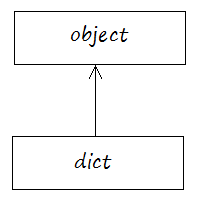
Um ein dictionary zu schreiben, benutzen Sie { } und darin schreiben Sie die Elemente. Die Elemente werden durch das Komma getrennt. Jede Elemente ist ein Paar von Schlüssel und Wert, die durch die Doppeltpunkt ( : ) getrennt werden
Zum Beispiel
# Dictionary
myinfo = {"Name": "Tran", "Age": 37, "Address" : "Vietnam" }
Sie können ein Objekt dictionary aus dem Constructor der Klasse dict.
createDictionaryFromClass.py
# Eine Dictionary durch den Constructor der Klasse dict erstellen
mydict = dict()
mydict["E01"] = "John"
mydict["E02"] = "King"
print ("mydict: ", mydict)
Output:
mydict: {'E01': 'John', 'E02': 'King'}
Die Merkmal der Wert (value) in dictionary:
- Jede Elemente (element) von dictionary ist ein Paar (Schlüssel und Wert). Die Wert ist irgendein Typ (string, Zahl, oder das von dem Benutzer definierte Typ,....), und kann wiederholen.
Das Merkmal des Schlüssel (key) in dictionary.
- Der Schlüssel in dictionary muss unveränderbar sein (immutable). Deshalb ist er string, Zahl, Tuple, ....
- Einige Typen werden nicht erlaubt wie List (die Liste), denn List ist ein veränderbares Typ (mutable).
- Die Schlüssel in dictionary dürfen nicht wiederholen
Zum Beispiel
dictionaryExample.py
# Dictionary
myinfo = {"Name": "Tran", "Age": 37, "Address" : "Vietnam" }
print ("myinfo['Name'] = ", myinfo["Name"])
print ("myinfo['Age'] = ", myinfo["Age"])
print ("myinfo['Address'] = ", myinfo["Address"])
Output:
myinfo['Name'] = Tran
myinfo['Age'] = 37
myinfo['Address'] = Vietnam
2. Dictionary aktualiseren
Dictionary erlaubt, eine Wert zu aktuellisieren, die einem Schlüssel entspricht. Es fügt eine Elemente ein wenn der Schlüssel in dictionary nicht existiert
updateDictionaryExample.py
# Dictionary
myinfo = {"Name": "Tran", "Age": 37, "Address" : "Vietnam" }
# Die Wert für den Schlussel (key) 'Address' aktuellisieren
myinfo["Address"] = "HCM Vietnam"
# Ein neues Element mit dem Schlussel (key) 'Phone' einfügen.
myinfo["Phone"] = "12345"
print ("Element count: ", len(myinfo) )
print ("myinfo['Name'] = ", myinfo["Name"])
print ("myinfo['Age'] = ", myinfo["Age"])
print ("myinfo['Address'] = ", myinfo["Address"])
print ("myinfo['Phone'] = ", myinfo["Phone"])
Output:
Element count: 4
myinfo['Name'] = Tran
myinfo['Age'] = 37
myinfo['Address'] = HCM Vietnam
myinfo['Phone'] = 12345
3. Dictionary löschen
Es gibt 2 Ausnahme bei der Entfernung einer Elemente aus dictionary
- Den Operator del benutzen
- Die Methode __delitem__(key) benutzen
deleteDictionaryExample.py
# (Key,Value) = (Name, Telefonenummer)
contacts = {"John": "01217000111", \
"Tom": "01234000111", \
"Addison":"01217000222", "Jack":"01227000123"}
print ("Contacts: ", contacts)
print ("\n")
print ("Delete key = 'John' ")
# Ein Element, das dem Schlussel 'John' entsprechen
del contacts["John"]
print ("Contacts (After delete): ", contacts)
print ("\n")
print ("Delete key = 'Tom' ")
# Ein Element, das dem Schlussel 'Tom' entsprechen
contacts.__delitem__( "Tom")
print ("Contacts (After delete): ", contacts)
print ("Clear all element")
# Alle Element ganz löschen.
contacts.clear()
print ("Contacts (After clear): ", contacts)
# Dictionary 'contacts' aus der Speicherung löschen
del contacts
# Der Fehler passiert bei Zugang der nicht-existierten Variable
print ("Contacts (After delete): ", contacts)
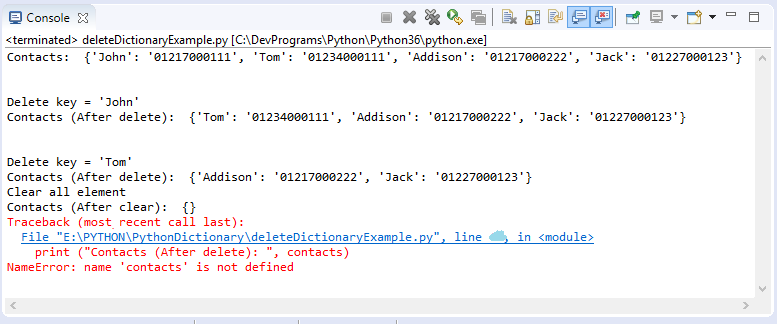
4. Die Funktion mit Dictionary
SN | Description |
len(dict) | Die Element von dict rückgeben. |
str(dict) | Die Funktion setzt zum String für dictionary um. |
type(variable) | Rückgabe der Variable-Typ, die eingegeben wird. Wenn die Eingabevariable dictionary ist, wird das Objekt der Vertretung der Klasse 'dict' rückgegeben. |
dir(clazzOrObject) | Rückgabe der Liste von Mitglieder der Klasse (oder Objekt), die eingegeben wird. Wenn die Klasse dict eingegeben wird, wird es die Mitgliedliste der Klasse dict.rückgegeben |
functionDictionaryExample.py
contacts = {"John": "01217000111" ,"Addison": "01217000222","Jack": "01227000123"}
print ("contacts: ", contacts)
print ("Element count: ", len(contacts) )
contactsAsString = str(contacts)
print ("str(contacts): ", contactsAsString )
# Ein Objekt vertritt die Klasse 'dict'.
aType = type(contacts)
print ("type(contacts): ", aType )
# Die Funktion dir(dict) gibt die Mitglieder der Klasse 'dict' zurück.
print ("dir(dict): ", dir(dict) )
# ------------------------------------------------------------------------------------
# ['__class__', '__contains__', '__delattr__', '__delitem__', '__dir__', '__doc__',
# '__eq__', '__format__', '__ge__', '__getattribute__', '__getitem__', '__gt__',
# '__hash__', '__init__', '__init_subclass__', '__iter__', '__le__', '__len__',
# '__lt__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__',
# '__setattr__', '__setitem__', '__sizeof__', '__str__', '__subclasshook__', 'clear',
# 'copy', 'fromkeys', 'get', 'items', 'keys', 'pop', 'popitem',
# 'setdefault', 'update', 'values']
# -------------------------------------------------------------------------------------
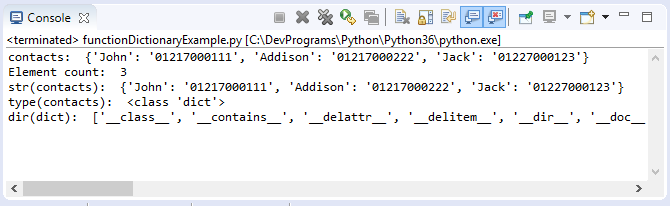
Anleitungen Python
- Lookup Python-Dokumentation
- Verzweigung in Python
- Die Anleitung zu Python Function
- Klasse und Objekt in Python
- Vererbung und Polymorphismus in Python
- Die Anleitung zu Python Dictionary
- Die Anleitung zu Python Lists
- Die Anleitung zu Python Tuples
- Die Anleitung zu Python Date Time
- Stellen Sie mit PyMySQL eine Verbindung zur MySQL-Datenbank in Python her
- Die Anleitung zu Python Exception
- Die Anleitung zu Python String
- Einführung in Python
- Installieren Sie Python unter Windows
- Installieren Sie Python unter Ubuntu
- Installieren Sie PyDev für Eclipse
- Konventionen und Grammatik-Versionen in Python
- Die Anleitung zum Python für den Anfänger
- Schleifen in Python
Show More