Die Anleitung zu AngularJS Filter
1. AngularJS Filters
Im AngularJS wird Filter benutzt um die Daten für den Benutzen zu formatieren.
Unter ist das die Syntax für die Verwendung von Filter:
Filters Usage
{{ expression [| filter_name[:parameter_value] ... ] }}
AngularJS hat einige grundlegenden Filter eingebaut und Sie können sie bereit verwenden.
Filter | Description |
lowercase | Format a string to lower case. |
uppercase | Format a string to upper case. |
number | Format a number to a string. |
currency | Format a number to a currency format. |
date | Format a date to a specified format. |
json | Format an object to a JSON string. |
filter | Select a subset of items from an array. |
limitTo | Limits an array/string, into a specified number of elements/characters. |
orderBy | Orders an array by an expression. |
2. uppercase/lowercase
upperlowercase-example.js
var app = angular.module("myApp", []);
app.controller("myCtrl", function($scope) {
$scope.greeting = "Hello World";
});
upperlowercase-example
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Filters</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<script src="upperlowercase-example.js"></script>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Filters: uppercase/lowercase</h3>
<p>Origin String: {{ greeting }}</p>
<p>Uppercase: {{ greeting | uppercase }}</p>
<p>Lowercase: {{ greeting | lowercase }}</p>
</div>
</body>
</html>
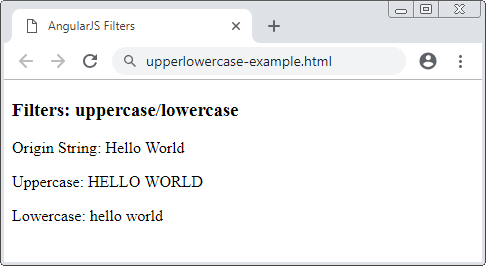
3. number
Der Filter number hilft bei der Formatierung einer Zahl in einem String.
Die Gebrauchweise im HTML:
<!-- fractionSize: Optional -->
{{ number_expression | number : fractionSize }}
Die Gebrauchweise im Javascript:
// fractionSize: Optional
$filter('number')( aNumber , fractionSize )
Die Parameter
aNumber |
number
string | Eine Zahl zu formatieren. |
fractionSize (Tùy chọn) | number
string | Eine Zahl für den Dezimale-Ort . Wenn Sie die Wert für diesen Paramter nicht bieten, hängt sein Wert von locale ab. Im Fall vom Default-locale ist die Wert 3. |
number-example.html
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Filters</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<script src="number-example.js"></script>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Filter: number</h3>
<p><b>Origin Number:</b> {{ revenueAmount }}</p>
<p><b>Default Number Format:</b> {{ revenueAmount | number }}</p>
<p><b>Format with 2 decimal places:</b> {{ revenueAmount | number : 2 }}</p>
</div>
</body>
</html>
number-example.js
var app = angular.module("myApp", []);
app.controller("myCtrl", function($scope) {
$scope.revenueAmount = 20011.2345;
});
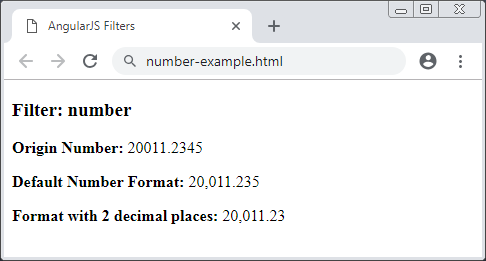
Zum Beipiel: eine number im Javascript verwenden:
number-example2.js
var app = angular.module("myApp", []);
app.controller("myCtrl", function($scope, $filter) {
var aNumber = 19001.2345;
// Use number filter in Javascript:
$scope.revenueAmountStr = $filter("number")(aNumber, 2);// ==> 19,001.23
});
4. currency
Der Filter currency wird benutzt um eine Zahl in die Wärung (z. B $1,234.56) zu formatieren. Im Grunde müssen Sie das Symbol von der Währung für die Formatierung anbieten, umgekehrt wird das momentane Default Symbol von der Währung von locale verwendet.
Die Gebrauchweise im HTML:
{{ amount | currency : symbol : fractionSize}}
Die Gebrauchweise im Javascript:
$filter('currency')(amount, symbol, fractionSize)
Die Paremeters:
amount | number | Eine Nummer |
symbol (optional) | string | Das Währungssymbol oder etwas ähnlich anzuzeigen. |
fractionSize (optional) | number | Die Nummer für den Dezimale-Ort. Wenn nicht angebietet, wird ihre Wert default auf momentane locale vom Browser basieren |
Zum Beispiel:
currency-example.js
var app = angular.module("myApp", []);
app.controller("myCtrl", function($scope) {
$scope.myAmount = 12345.678;
});
currency-example.html
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Filters</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<script src="currency-example.js"></script>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Filter: currency</h3>
Enter Amount:
<input type="number" ng-model="myAmount" />
<p>
<b ng-non-bindable>{{ myAmount | currency }}</b>:
<span>{{ myAmount | currency }}</span>
</p>
<p>
<b ng-non-bindable>{{ myAmount | currency : '$' : 2 }}</b>:
<span>{{ myAmount | currency : '$' : 2 }}</span>
</p>
<p>
<b ng-non-bindable>{{ myAmount | currency : '€' : 1 }}</b>:
<span>{{ myAmount | currency : '€' : 1 }}</span>
</p>
</div>
</body>
</html>
Oft steht das Symbol von der Währung vor das Geldmenge (z.B €123.45), das von locale abhängt. Sie können eine gewünschte locale bestimmen, z.B locale_de-de, dann bekommen Sie ein Ergebnis von 123.45€.
Die Bibliotheke locale vom AngularJS nachschlagen:
currency-locale-example.js
var app = angular.module("myApp", []);
app.controller("myCtrl", function($scope) {
$scope.myAmount = 12345.678;
});
currency-locale-example.html
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Filters</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<!-- Use locale_de-de -->
<!-- https://cdnjs.com/libraries/angular-i18n -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/angular-i18n/1.7.4/angular-locale_de-de.js"></script>
<script src="currency-locale-example.js"></script>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Filter: currency (LOCALE: de-de)</h3>
Enter Amount:
<input type="number" ng-model="myAmount" />
<p>
<b ng-non-bindable>{{ myAmount | currency }}</b>:
<span>{{ myAmount | currency }}</span>
</p>
<p>
<b ng-non-bindable>{{ myAmount | currency : '$' : 2 }}</b>:
<span>{{ myAmount | currency : '$' : 2 }}</span>
</p>
<p>
<b ng-non-bindable>{{ myAmount | currency : '€' : 1 }}</b>:
<span>{{ myAmount | currency : '€' : 1 }}</span>
</p>
</div>
</body>
</html>
5. date
Der Filter date wird benutzt um ein Objekt Date in einem String zu formatieren.
Die Gebrauchsweise vom Filter date im HTML:
{{ date_expression | aDate : format : timezone}}
Die Gebrauchweise vom Filter date im Javascript:
$filter('date')(aDate, format, timezone)
Die Parameters:
TODO
Zum Beispiel:
date-example.js
var app = angular.module("myApp", []);
app.controller("myCtrl", function($scope) {
$scope.myDate = new Date();
});
date-example.html
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Filters</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<script src="date-example.js"></script>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Filter: date</h3>
<p>
<!-- $scope.myDate = new Date() -->
<b ng-non-bindable>{{ myDate | date: 'yyyy-MM-dd HH:mm'}}</b>:
<span>{{ myDate | date: 'yyyy-MM-dd HH:mm'}}</span>
</p>
<p>
<b ng-non-bindable>{{1288323623006 | date:'medium'}}</b>:
<span>{{1288323623006 | date:'medium'}}</span>
</p>
<p>
<b ng-non-bindable>{{1288323623006 | date:'yyyy-MM-dd HH:mm:ss Z'}}</b>:
<span>{{1288323623006 | date:'yyyy-MM-dd HH:mm:ss Z'}}</span>
</p>
<p>
<b ng-non-bindable>{{1288323623006 | date:'MM/dd/yyyy @ h:mma'}}</b>:
<span>{{'1288323623006' | date:'MM/dd/yyyy @ h:mma'}}</span>
</p>
<p>
<b ng-non-bindable>{{1288323623006 | date:"MM/dd/yyyy 'at' h:mma"}}</b>:
<span>{{'1288323623006' | date:"MM/dd/yyyy 'at' h:mma"}}</span>
</p>
</div>
</body>
</html>
6. json
Der Filter json wird verwendet um ein Objekt in einem JSON formatierten String umzuwwandeln.
Die Gebrauchsweise im HTML:
{{ json_expression | json : spacing}}
Die Gebrauchweise im Javascript:
$filter('json')(object, spacing)
Die Parameters:
object | * | Irgendein Objekt JavaScript (Array und die primitiven Stil umfassen) |
spacing (optional) | number | Die Nummer der Leerzeichen wird für jede Einrückung (indentation) verwendet. Es ist nach Default 2. |
Zum Beispiel:
json-example.js
var app = angular.module("myApp", []);
app.controller("myCtrl", function($scope) {
$scope.department = {
id : 1,
name: "Sales",
employees : [
{id: 1, fullName: "Tom", gender: "Male"},
{id: 2, fullName: "Jerry", gender: "Male"}
]
};
});
json-example.html
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Filters</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<script src="json-example.js"></script>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Filter: json</h3>
<p>
<b ng-non-bindable>{{ department | json : 5 }}</b>:
<pre>{{ department | json : 5}}</pre>
</p>
<p>
<b ng-non-bindable>{{ department | json }}</b>:
<pre>{{ department | json }}</pre>
</p>
</div>
</body>
</html>
7. limitTo
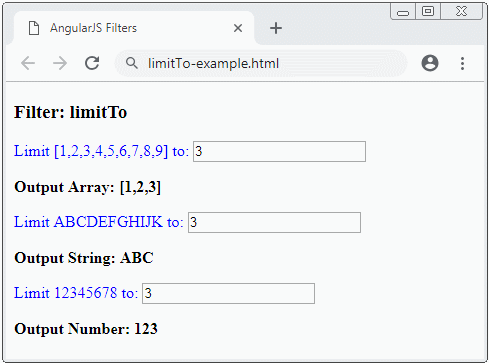
Die Gebrauchweise im HTML:
{{ input | limitTo : limit : begin}}
Die Gebrauchweise im Javascript:
$filter('limitTo')(input, limitLength, startIndex)
Die Parameters:
Zum Beispiel:
limitTo-example.js
var app = angular.module("myApp", [ ]);
app.controller("myCtrl", function($scope) {
$scope.anArray = [1,2,3,4,5,6,7,8,9];
$scope.aString = "ABCDEFGHIJK";
$scope.aNumber = 12345678;
$scope.arrayLimit = 3;
$scope.stringLimit = 3;
$scope.numberLimit = 3;
});
limitTo-example.html
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Filters</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.7.2/angular.min.js"></script>
<script src="limitTo-example.js"></script>
<style>
p {color: blue;}
</style>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtrl">
<h3>Filter: limitTo</h3>
<p>
Limit {{anArray}} to:
<input type="number" step="1" ng-model="arrayLimit">
</p>
<b>Output Array: {{ anArray | limitTo: arrayLimit }}</b>
<p>
Limit {{aString}} to:
<input type="number" step="1" ng-model="stringLimit">
</p>
<b>Output String: {{ aString | limitTo: stringLimit }}</b>
<p>
Limit {{aNumber}} to:
<input type="number" step="1" ng-model="numberLimit">
</p>
<b>Output Number: {{ aNumber | limitTo: numberLimit }}</b>
</div>
</body>
</html>
8. filter
Der filter wird verwendet um eine Untergruppe (subset) in einem Array auszuwwählen und ein neues Array zurückzugeben.
Die Gebrauchweise im HTML:
{{ inputArray | filter : expression : comparator : anyPropertyKey}}
Die Gebrauchweise im Javascript:
$filter('filter')(inputArray, expression, comparator, anyPropertyKey)