Verwenden Sie Scribe OAuth Java API mit Google OAuth 2
1. Das Problem mit Google Oauth1a
Es gibt ein Problem mit Google OAuth 1a. Seit 20-04-2015 unterstützt google offiziell OAuth 1 nicht mehr. Wenn Sie gerade Scribe Java API zum Umgang mit Google OAuth 1.0 benutzen, müssen Sie Ihre Code ändern.
Im Dokument führe ich Scribe Java API benutzen um mit Google OAuth 2.0 zu arbeiten
Im Dokument führe ich Scribe Java API benutzen um mit Google OAuth 2.0 zu arbeiten
2. Die Erstellung von der Applikation auf Google Developers Console
In the first time, you do not have any Project, so you have to create it. In most cases, you should name Project same your Website name (It is not compulsory).
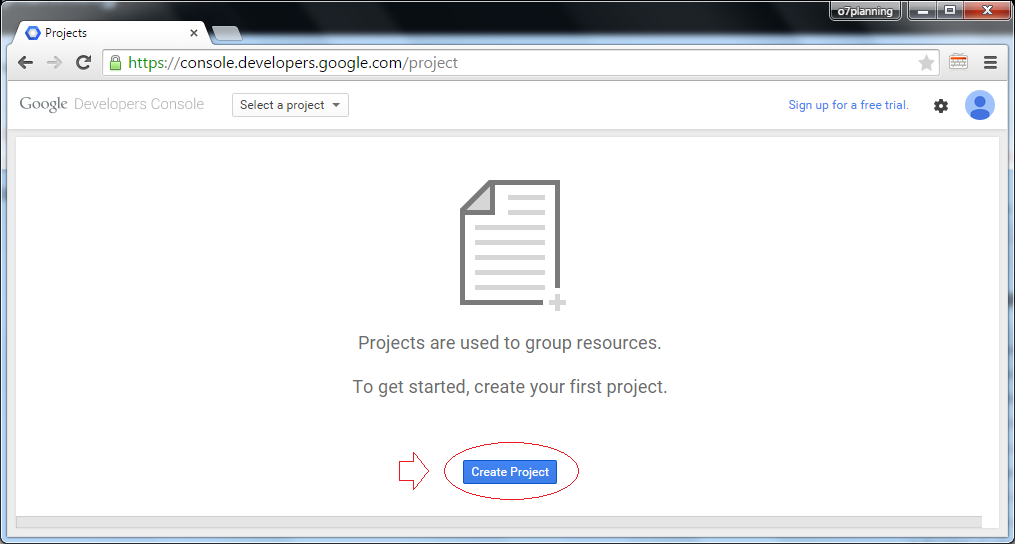
Project Name: ExampleWebsite
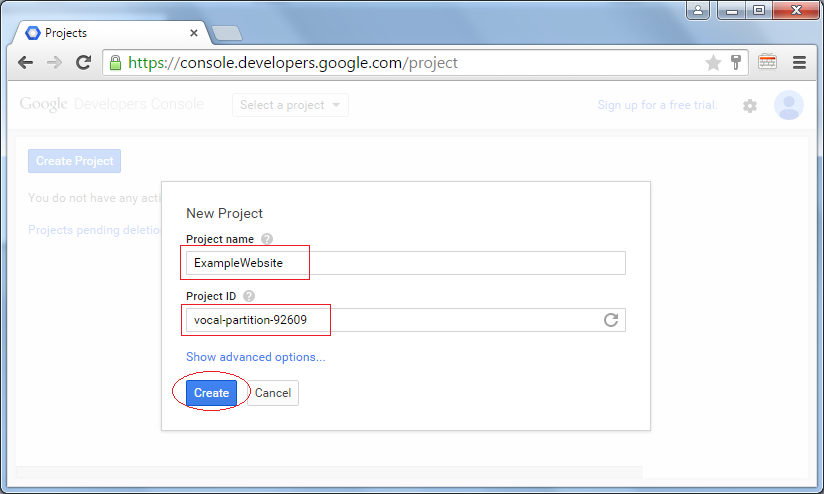
Project has been created:
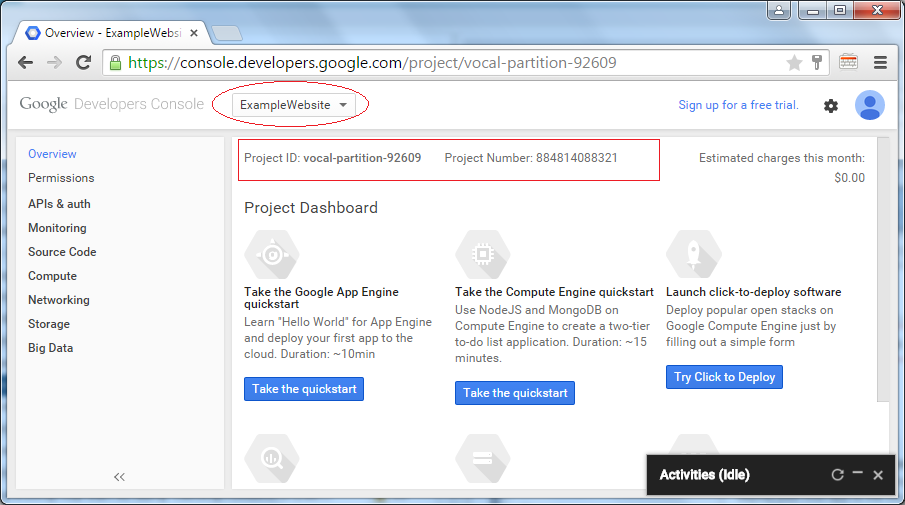
Goto "APIs and auth/Consent screen", to create a Product Name.
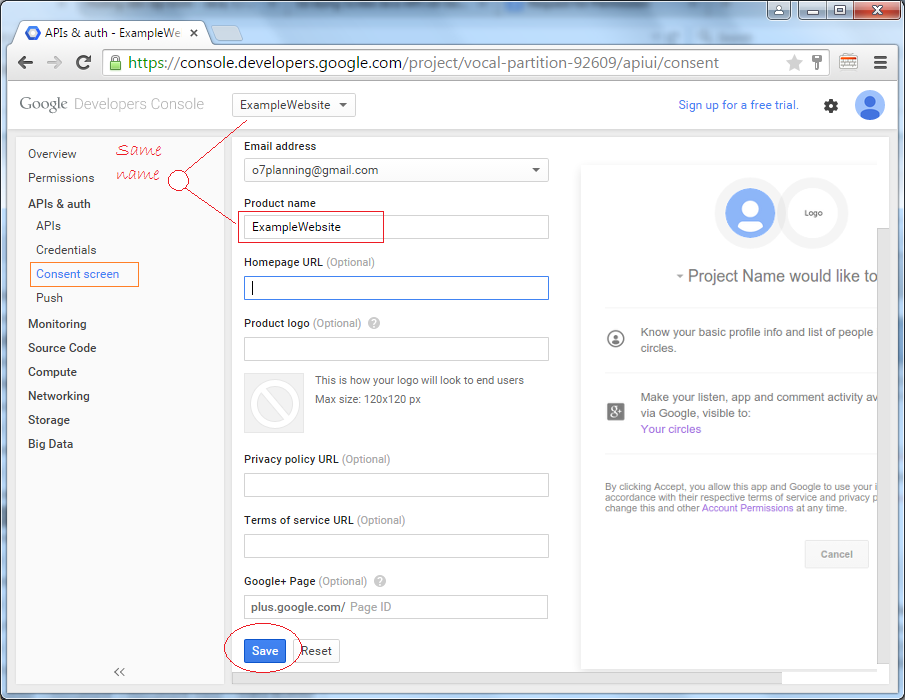
Create Client ID:
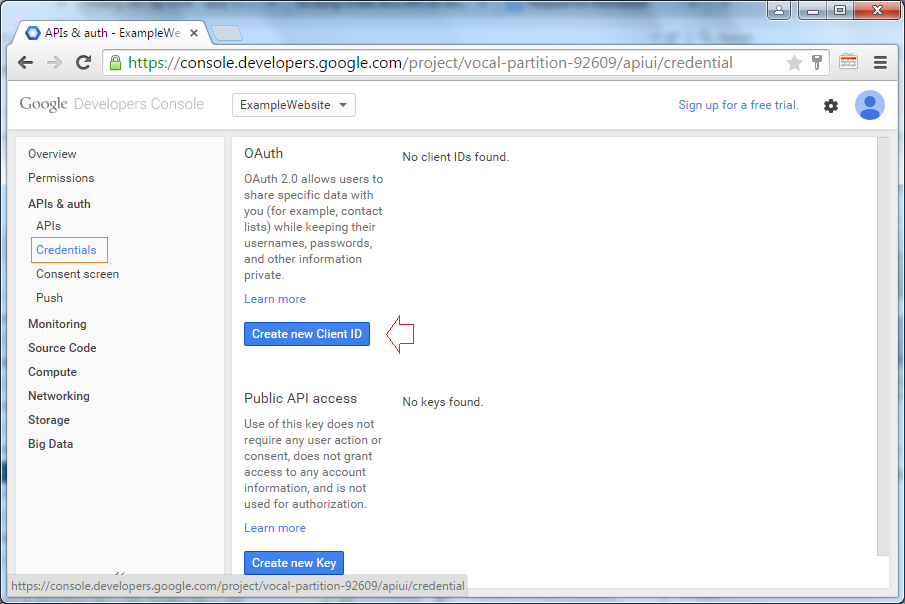
In case you use OAuth2.0 on a web application, you will have to create ClientID for that web application. Parallel, you declare the redirecting link (The link will be redirected to after user logs in his/her google account and allows your application to connect with Google OAuth).
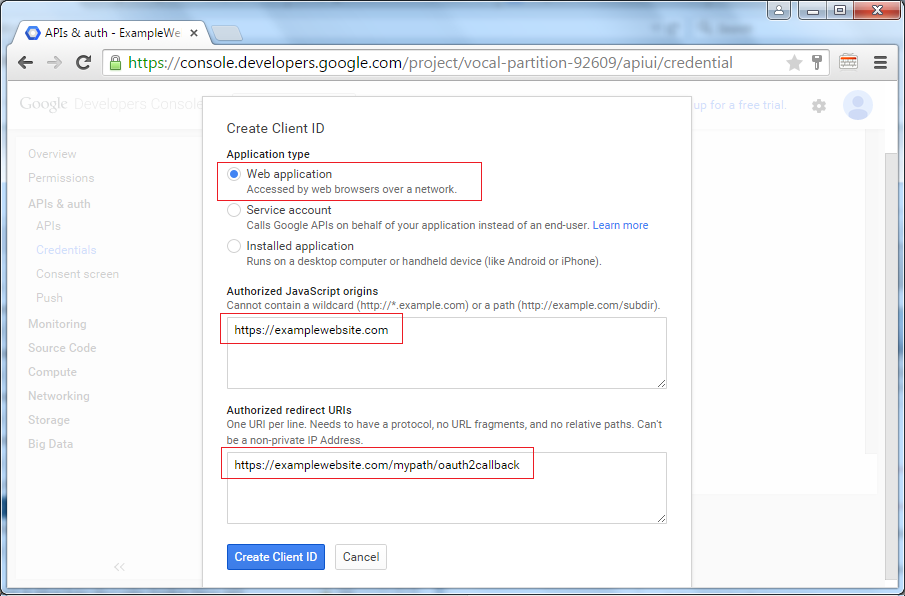
ClientID is created, in which the two most important kinds of information are Client ID and Client Secret. You need to have it in Java code (Illustrated in the following example).
Note: You can create multiple ClientIDinformation for your different applications.
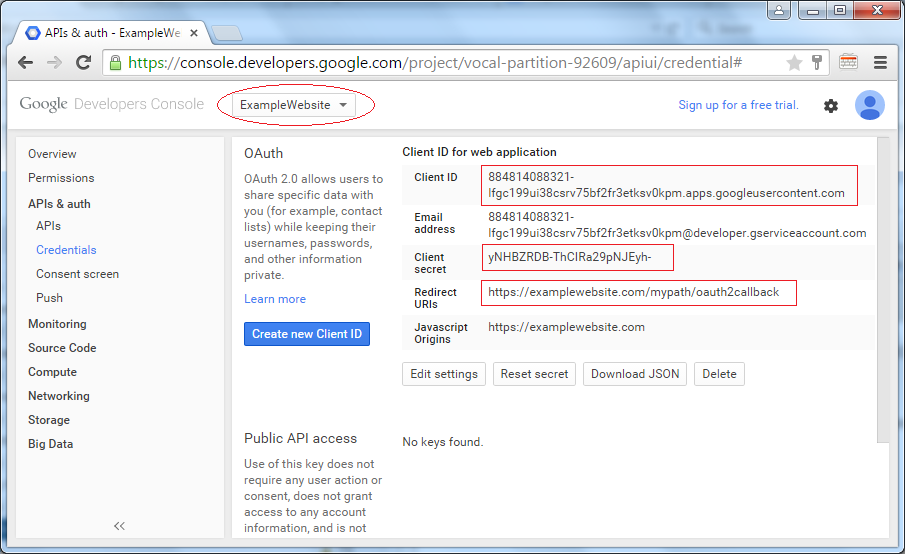
3. Die Erstellung vom Projekt und die Erklärung von der Bibliothek Scribe Java API
- File/New/Others
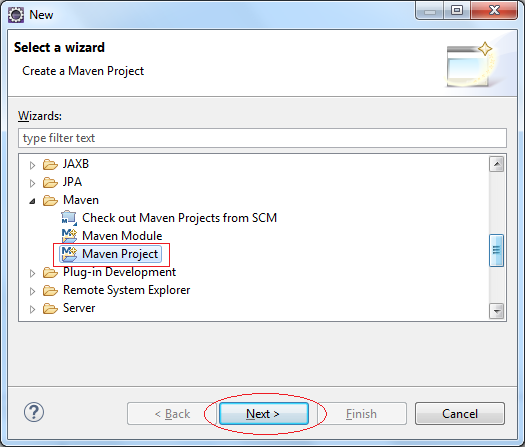
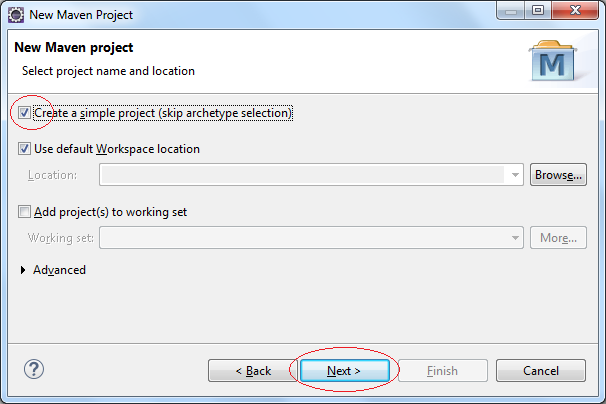
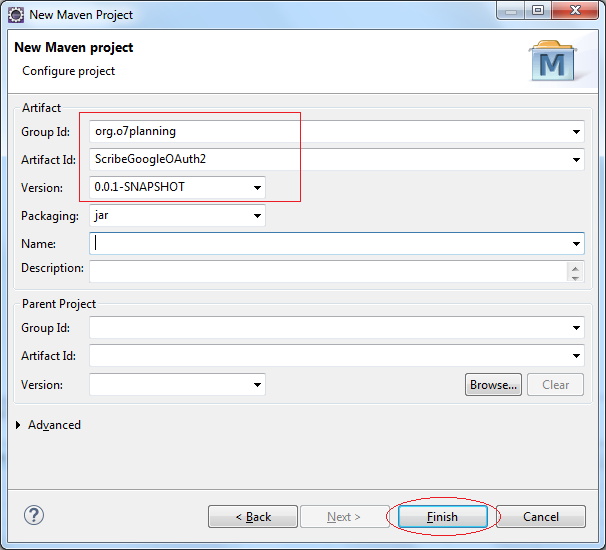
Ihr Project wurde erstellt.
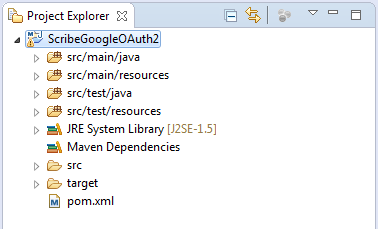
Die Konfiguration von Maven benutzt Scribe Java API:
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>ScribeGoogleOAuth2</artifactId>
<version>0.0.1-SNAPSHOT</version>
<dependencies>
<!-- http://mvnrepository.com/artifact/org.scribe/scribe -->
<dependency>
<groupId>org.scribe</groupId>
<artifactId>scribe</artifactId>
<version>1.3.7</version>
</dependency>
</dependencies>
</project>
4. Code Project
Scribe Java API ist ein API, das Ihnen bei der einfachen Umgang mit OAuth hilft. Es versteckt den Unterschied zwischen der Anbieter der Dienstleistung OAuth (Google, yahoo, facebook,..), und unterstützt OAuth 1a, OAuth 2.0
Zum Beispiel: Um mit LinkIn zu arbeiten, ist Ihre Kode
OAuthService service = new ServiceBuilder()
.provider(LinkedInApi.class)
.apiKey(YOUR_API_KEY)
.apiSecret(YOUR_API_SECRET)
.build();
Um mit Google OAuth 1a zu arbeiten, ist Ihre Kode:
OAuthService service = new ServiceBuilder()
.provider(GoogleApi.class)
.apiKey(YOUR_API_KEY)
.apiSecret(YOUR_API_SECRET)
.build();
Scribe Java API stellen die API für die unterschiedlichen Anbieter bereit
- FacebookApi
- GoogleApi
- FoursquareApi
- Foursquare2Api
- YahooApi
- TwitterApi
- ....
Leider wird GooleApi nur für Google OAuth 1 benutzt, nicht für Google OAuth 2.0 benutzt. Und Sie finden die Klasse Google2Api in der Bibliothek von Scribe 1.3.7 nicht (wegen des Urheberrecht vielleicht)
Deshalb sollen Sie in Ihrem Projekt eine Klasse Google2Api erstellen, Und beachten Sie, dass es in der Package org.scribe.builder.api liegen müssen
Deshalb sollen Sie in Ihrem Projekt eine Klasse Google2Api erstellen, Und beachten Sie, dass es in der Package org.scribe.builder.api liegen müssen
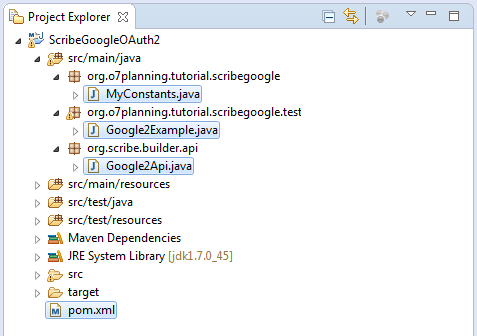
Google2Api.java
package org.scribe.builder.api;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import org.scribe.exceptions.OAuthException;
import org.scribe.extractors.AccessTokenExtractor;
import org.scribe.model.OAuthConfig;
import org.scribe.model.OAuthConstants;
import org.scribe.model.OAuthRequest;
import org.scribe.model.Response;
import org.scribe.model.Token;
import org.scribe.model.Verb;
import org.scribe.model.Verifier;
import org.scribe.oauth.OAuth20ServiceImpl;
import org.scribe.oauth.OAuthService;
import org.scribe.utils.OAuthEncoder;
import org.scribe.utils.Preconditions;
/**
* Google OAuth2.0 Released under the same license as scribe (MIT License)
*
* @author yincrash
*
*/
public class Google2Api extends DefaultApi20 {
private static final String AUTHORIZE_URL = "https://accounts.google.com/o/oauth2/auth?response_type=code&client_id=%s&redirect_uri=%s";
private static final String SCOPED_AUTHORIZE_URL = AUTHORIZE_URL
+ "&scope=%s";
@Override
public String getAccessTokenEndpoint() {
return "https://accounts.google.com/o/oauth2/token";
}
@Override
public AccessTokenExtractor getAccessTokenExtractor() {
return new AccessTokenExtractor() {
@Override
public Token extract(String response) {
Preconditions
.checkEmptyString(response,
"Response body is incorrect. Can't extract a token from an empty string");
Matcher matcher = Pattern.compile(
"\"access_token\" : \"([^&\"]+)\"").matcher(response);
if (matcher.find()) {
String token = OAuthEncoder.decode(matcher.group(1));
return new Token(token, "", response);
} else {
throw new OAuthException(
"Response body is incorrect. Can't extract a token from this: '"
+ response + "'", null);
}
}
};
}
@Override
public String getAuthorizationUrl(OAuthConfig config) {
// Append scope if present
if (config.hasScope()) {
return String.format(SCOPED_AUTHORIZE_URL, config.getApiKey(),
OAuthEncoder.encode(config.getCallback()),
OAuthEncoder.encode(config.getScope()));
} else {
return String.format(AUTHORIZE_URL, config.getApiKey(),
OAuthEncoder.encode(config.getCallback()));
}
}
@Override
public Verb getAccessTokenVerb() {
return Verb.POST;
}
@Override
public OAuthService createService(OAuthConfig config) {
return new GoogleOAuth2Service(this, config);
}
private class GoogleOAuth2Service extends OAuth20ServiceImpl {
private static final String GRANT_TYPE_AUTHORIZATION_CODE = "authorization_code";
private static final String GRANT_TYPE = "grant_type";
private DefaultApi20 api;
private OAuthConfig config;
public GoogleOAuth2Service(DefaultApi20 api, OAuthConfig config) {
super(api, config);
this.api = api;
this.config = config;
}
@Override
public Token getAccessToken(Token requestToken, Verifier verifier) {
OAuthRequest request = new OAuthRequest(api.getAccessTokenVerb(),
api.getAccessTokenEndpoint());
switch (api.getAccessTokenVerb()) {
case POST:
request.addBodyParameter(OAuthConstants.CLIENT_ID,
config.getApiKey());
request.addBodyParameter(OAuthConstants.CLIENT_SECRET,
config.getApiSecret());
request.addBodyParameter(OAuthConstants.CODE,
verifier.getValue());
request.addBodyParameter(OAuthConstants.REDIRECT_URI,
config.getCallback());
request.addBodyParameter(GRANT_TYPE,
GRANT_TYPE_AUTHORIZATION_CODE);
break;
case GET:
default:
request.addQuerystringParameter(OAuthConstants.CLIENT_ID,
config.getApiKey());
request.addQuerystringParameter(OAuthConstants.CLIENT_SECRET,
config.getApiSecret());
request.addQuerystringParameter(OAuthConstants.CODE,
verifier.getValue());
request.addQuerystringParameter(OAuthConstants.REDIRECT_URI,
config.getCallback());
if (config.hasScope())
request.addQuerystringParameter(OAuthConstants.SCOPE,
config.getScope());
}
Response response = request.send();
return api.getAccessTokenExtractor().extract(response.getBody());
}
}
}
Erstellen Sie eine Klasse MyConstants für die Archievierung der Google Client ID und Client Secret , die Sie vorher in Google Developers Console erstellt haben
MyConstants.java
package org.o7planning.tutorial.scribegoogle;
public class MyConstants {
// Client ID
public static final String GOOGLE_CLIENT_ID = "884814088321-lfgc199ui38csrv75bf2fr3etksv0kpm.apps.googleusercontent.com";
// Client Secret
public static final String GOOGLE_CLIENT_SECRET = "yNHBZRDB-ThCIRa29pNJEyh-";
// Redirect URI
public static final String GOOGLE_REDIRECT_URL = "https://examplewebsite.com/mypath/oauth2callback";
}
Google2Example.java
package org.o7planning.tutorial.scribegoogle.test;
import java.util.Scanner;
import org.o7planning.tutorial.scribegoogle.MyConstants;
import org.scribe.builder.ServiceBuilder;
import org.scribe.builder.api.Google2Api;
import org.scribe.model.OAuthRequest;
import org.scribe.model.Response;
import org.scribe.model.Token;
import org.scribe.model.Verb;
import org.scribe.model.Verifier;
import org.scribe.oauth.OAuthService;
public class Google2Example {
private static final String NETWORK_NAME = "Google";
private static final String PROTECTED_RESOURCE_URL = "https://www.googleapis.com/oauth2/v2/userinfo?alt=json";
private static final String SCOPE = "https://mail.google.com/ https://www.googleapis.com/auth/userinfo.email";
private static final Token EMPTY_TOKEN = null;
public static void main(String[] args) {
String apiKey = MyConstants.GOOGLE_CLIENT_ID;
String apiSecret = MyConstants.GOOGLE_CLIENT_SECRET;
String callbackUrl = MyConstants.GOOGLE_REDIRECT_URL;
// Create OAuthService for Google OAuth 2.0
OAuthService service = new ServiceBuilder().provider(Google2Api.class)
.apiKey(apiKey).apiSecret(apiSecret).callback(callbackUrl)
.scope(SCOPE).build();
Scanner in = new Scanner(System.in);
System.out.println("=== " + NETWORK_NAME + "'s OAuth Workflow ===");
System.out.println();
Verifier verifier = null;
Token accessToken = null;
// Obtain the Authorization URL
System.out.println("Fetching the Authorization URL...");
String authorizationUrl = service.getAuthorizationUrl(EMPTY_TOKEN);
System.out.println("Got the Authorization URL!");
System.out.println("Now go and authorize Scribe here:");
System.out.println();
// Copy this URL and run in browser.
System.out.println(authorizationUrl);
System.out.println();
// Copy Authorization Code in browser URL and paste to Console
System.out.println("And paste the authorization code here");
System.out.print(">>");
verifier = new Verifier(in.nextLine());
System.out.println();
// Trade the Request Token and Verfier for the Access Token
System.out.println("Trading the Request Token for an Access Token...");
accessToken = service.getAccessToken(EMPTY_TOKEN, verifier);
System.out.println("Got the Access Token!");
System.out.println("(if your curious it looks like this: "
+ accessToken + " )");
System.out.println();
// Now let's go and ask for a protected resource!
System.out.println("Now we're going to access a protected resource...");
OAuthRequest request = new OAuthRequest(Verb.GET,
PROTECTED_RESOURCE_URL);
service.signRequest(accessToken, request);
Response response = request.send();
System.out.println("Got it! Lets see what we found...");
System.out.println();
System.out.println(response.getCode());
System.out.println(response.getBody());
System.out.println();
System.out
.println("Thats it man! Go and build something awesome with Scribe! :)");
in.close();
}
}
Die Klasse Google2Example durchführen:
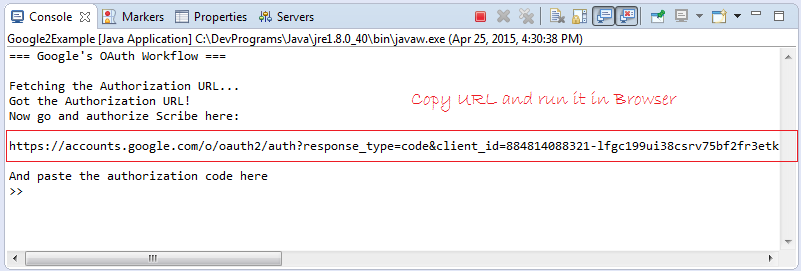
Kopieren Sie URL wie oben rot markiert und es auf dem Browser laufen
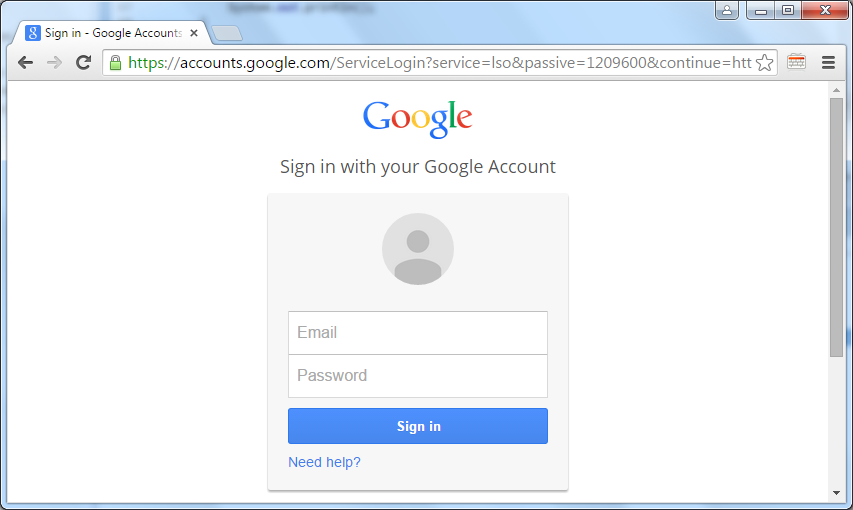
Nach der Anmeldung in dem Google Konto fordert Google den Benutzer, einige Information des Kontos zu sehen lassen. Klicken Sie auf Accept zur Genehmigung.
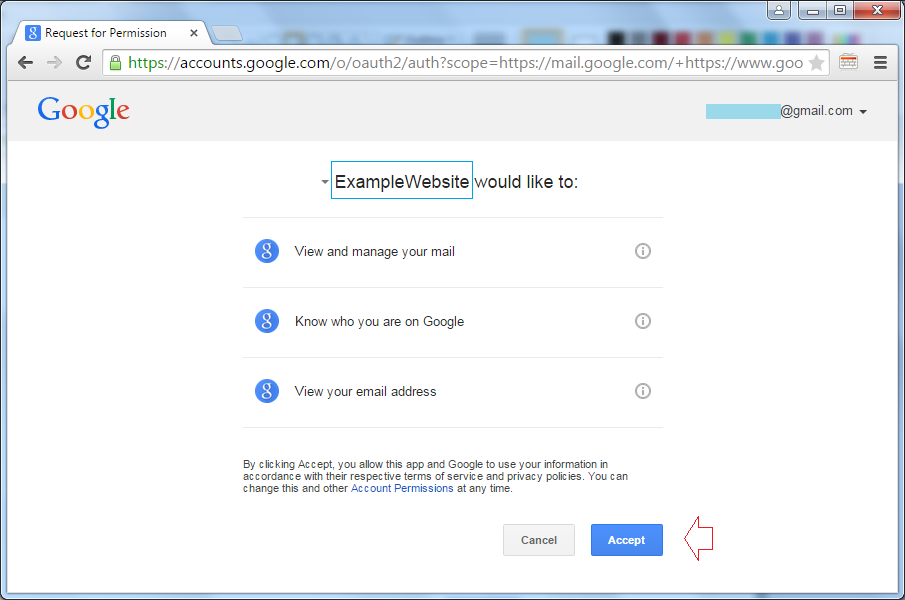
Die Website leitet (redirect) ins Callback-URL um, einschließend die Zugangskode. Sie sollen diese Zugangskode kopieren
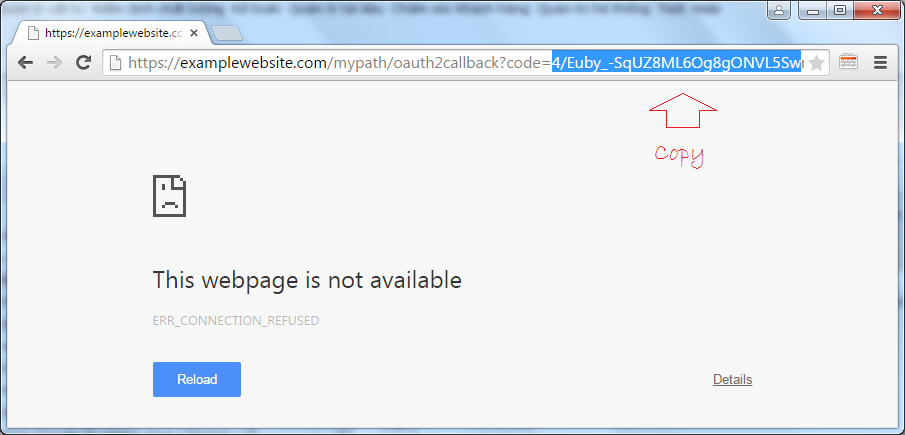
Die Kode kopieren und auf dem Fenster Java Console kleben:
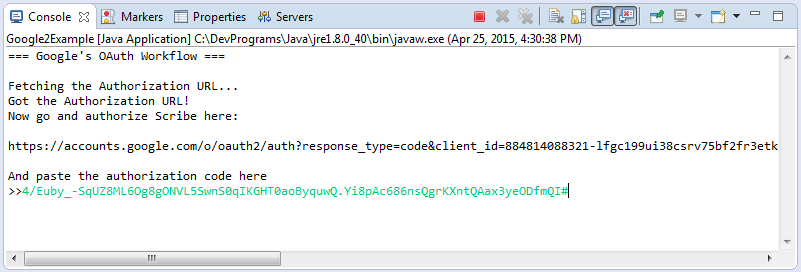
Sie erhalten die Information des Benutzer.
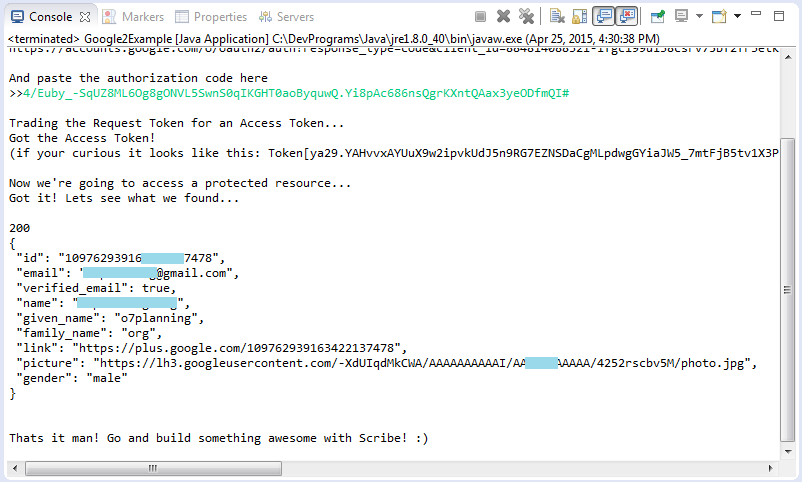
Java Open Source Bibliotheken
- Die Anleitung zu Java JSON Processing API (JSONP)
- Verwenden Sie Scribe OAuth Java API mit Google OAuth 2
- Hardware-Informationen in der Java-Anwendung abrufen
- Restfb Java API für Facebook
- Erstellen Sie Credentials für Google Drive API
- Die Anleitung zu Java JDOM2
- Die Anleitung zu Java XStream
- Verwenden Sie Java Jsoup Parsing HTML
- Rufen Sie geografische Informationen basierend auf der IP-Adresse mit GeoIP2JavaAPI ab
- Lesen und Schreiben von Excel-Dateien in Java mit Apache POI
- Entdecken Sie die Facebook Graph API
- Manipulieren von Dateien und Ordnern auf Google Drive mithilfe von Java
Show More