Rufen Sie geografische Informationen basierend auf der IP-Adresse mit GeoIP2JavaAPI ab
1. Was ist GeoIP2?
GeoIP2 ist ein Open Source Code Bibliothek Java. Es bietet GeoLite2 umsonst- ein Positionierungsdatum mit den angemessenen IP-Addresse- und API für den Umgang mit diesem Datum und API für den Umgang mit der Webservice von Positionierung
GeoLite2 ist eine umsonste Positionierungsdatum. Und das Datum wird regelmäßig in den ersten Dienstag des Monats aktuallisiert
GeoLite2 ist eine umsonste Positionierungsdatum. Und das Datum wird regelmäßig in den ersten Dienstag des Monats aktuallisiert
GeoIP2 unterstützt vielen verschiedenen Programmierungssprache wie: C#, C, Java, Perl, PHP, Python,...
Sie haben 2 Maßnahmen bei dem Arbeiten mit GeoIP2 API:
- GeoLite2 mit dem Positionierungsdaten herunterladen und es wie eine lokalen Databank benutzen. GeoIP2 API nimmt die geograhische Information nach der IP-Addresse in der Datensquellen
- GeoIP2 API mit der Webservice zur Informationversorgung durch die IP-Addresse verbinden. In der Maßnahme brauchst du ein License_Key ( Sie müssen bezahlen).
2. Download GeoIP2
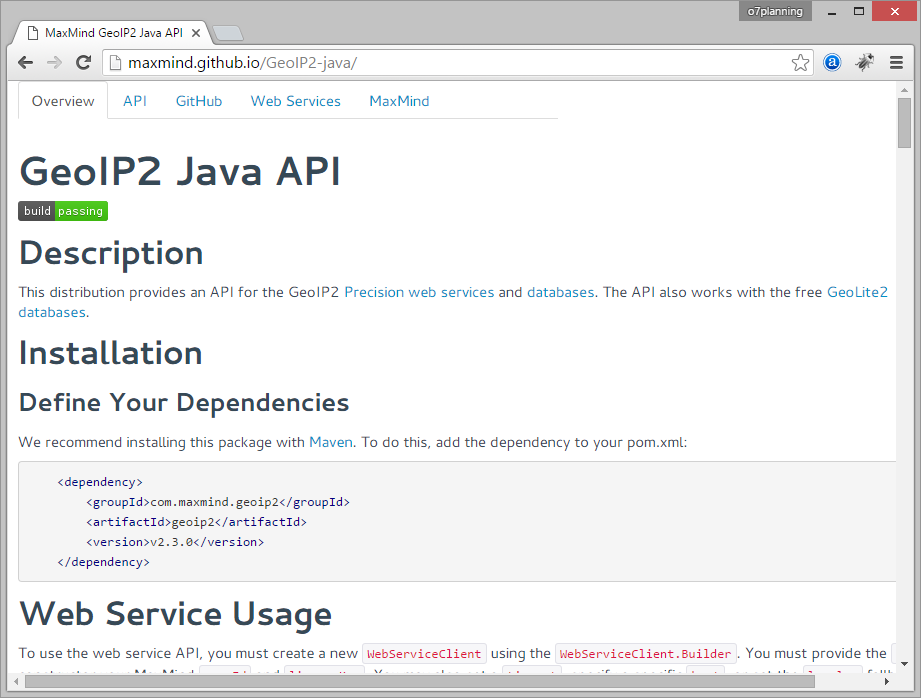
Wenn Sie Maven benutzen:
<!-- http://mvnrepository.com/artifact/com.maxmind.geoip2/geoip2 -->
<dependency>
<groupId>com.maxmind.geoip2</groupId>
<artifactId>geoip2</artifactId>
<version>2.15.0</version>
</dependency>
Oder die Bibliothek herunterladen
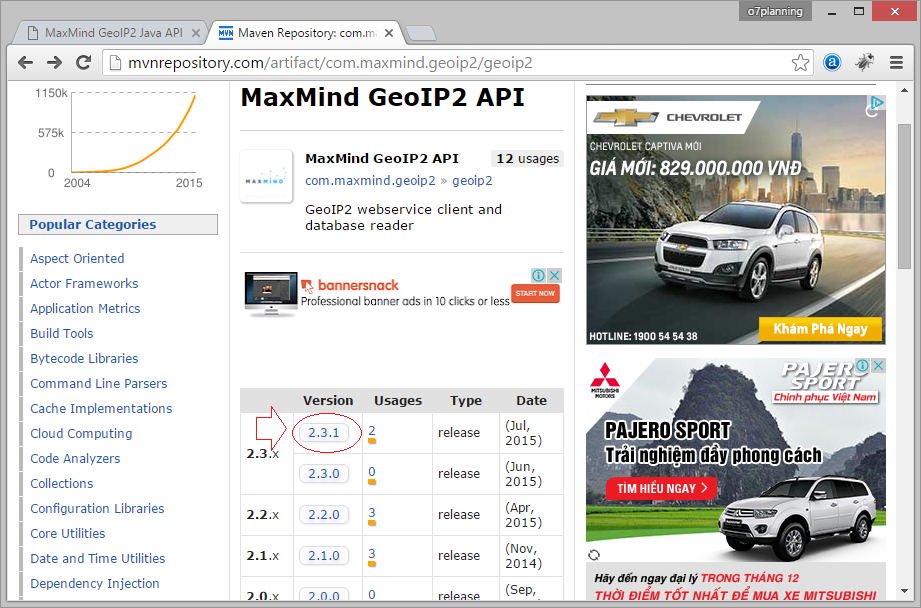
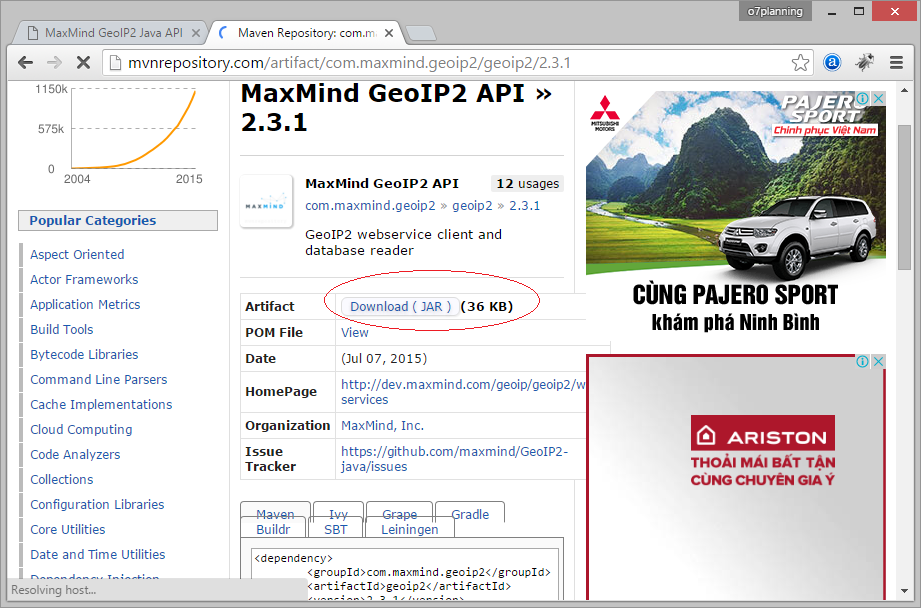
3. Download GeoLite2
To download GeoLite2 database you need to register for an account, which is completely free.
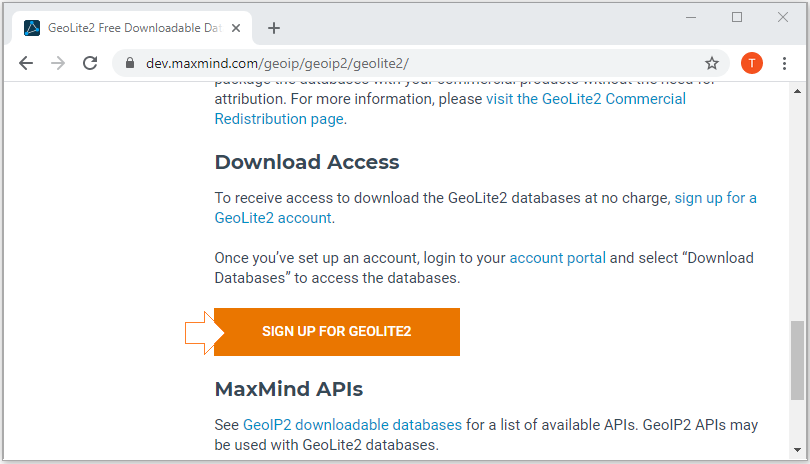
Then log in with your account:
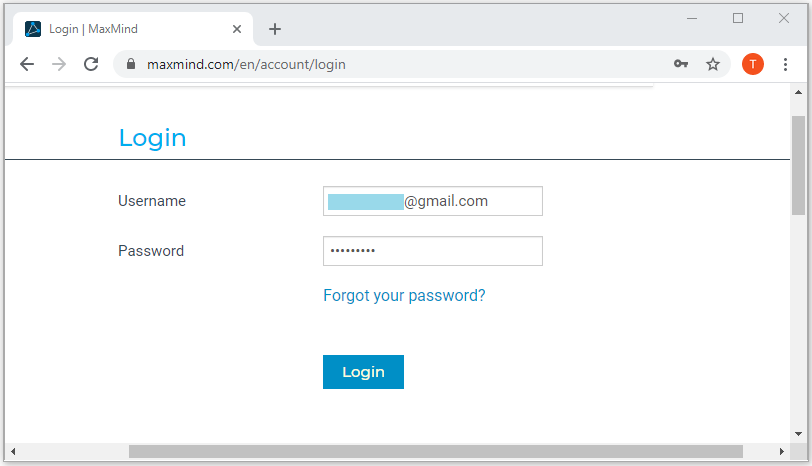
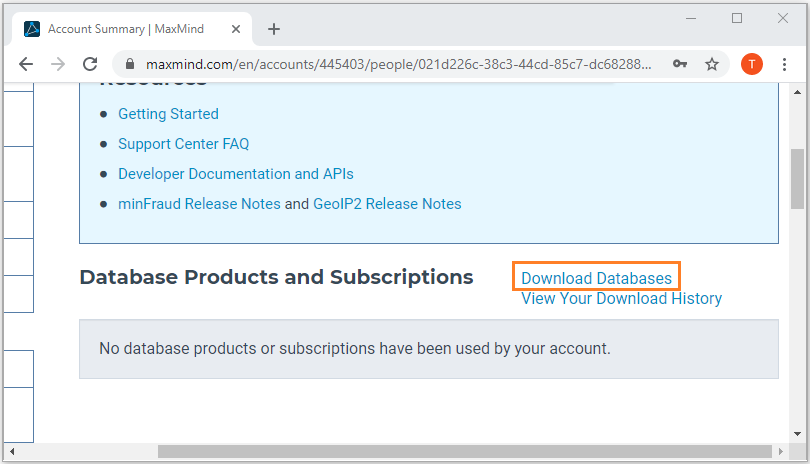
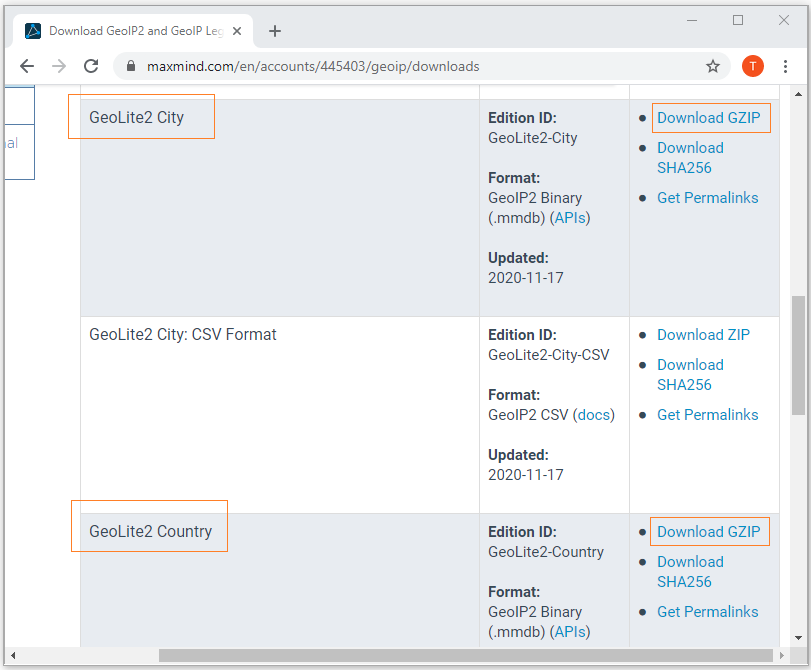
Das Ergebnis des Herunterladen
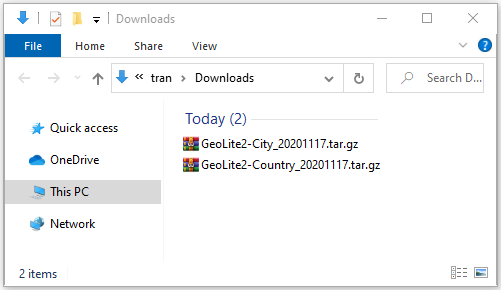
2 File diskomprimieren
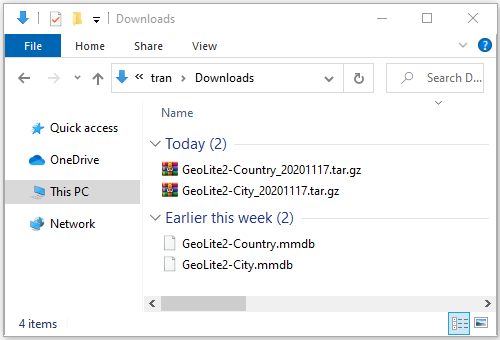
4. Die schnelle Erstellung eines Projekt zum Umgang mit GeoIP2
Ein Projekt Maven erstellen
- File/New/Other...
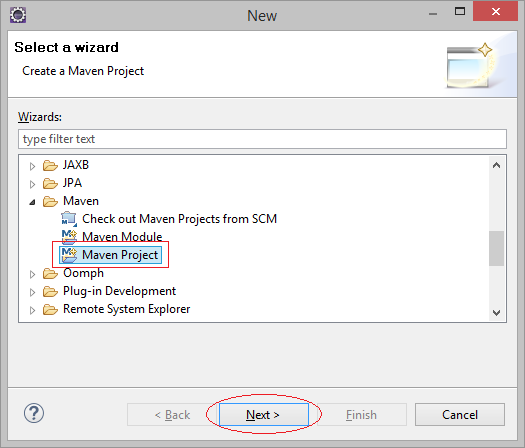
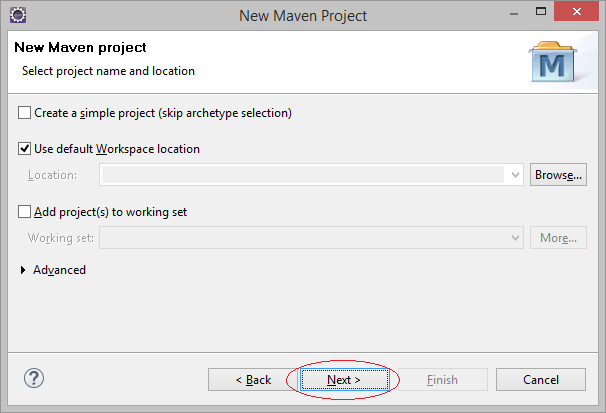
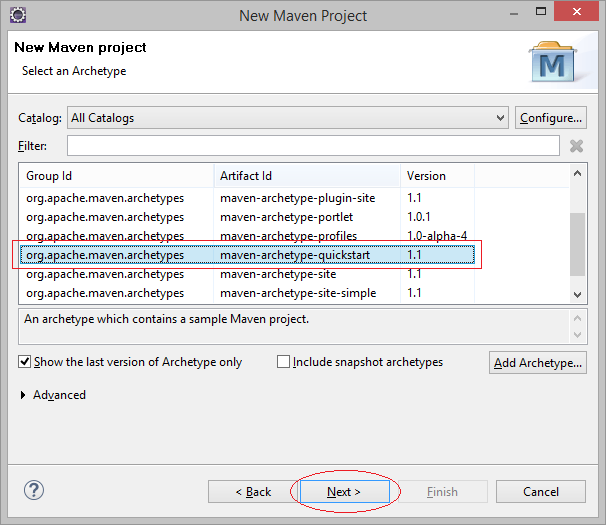
Geben Sie ein
- Group Id: org.o7planning
- Artifact Id: geoip2tutorial
- Package: org.o7planning.geoip2tutorial
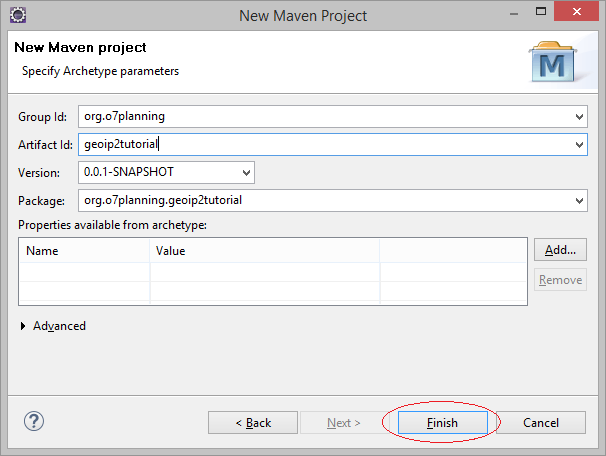
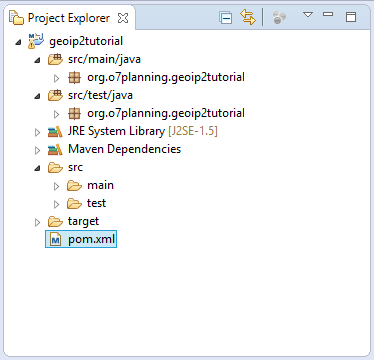
Melden Sie Maven
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>geoip2tutorial</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>geoip2tutorial</name>
<url>http://maven.apache.org</url>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
<!-- http://mvnrepository.com/artifact/com.maxmind.geoip2/geoip2 -->
<dependency>
<groupId>com.maxmind.geoip2</groupId>
<artifactId>geoip2</artifactId>
<version>2.15.0</version>
</dependency>
</dependencies>
</project>
5. Zum Beispiel über GeoIP2
Sie haben die Datenbank GeoLite2 heruntergeladet und sie in einem lokalen Ordner gelegt. Das Beispiel benutzt GeoIP2 Java API um die geographische Information mit der entsprechenden IP-Addresse zu nehmen
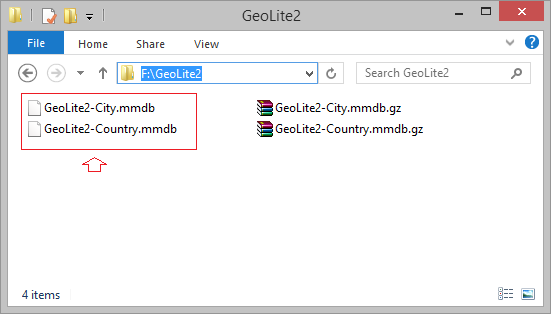
MyConstants.java
package org.o7planning.geoip2tutorial;
public class MyConstants {
// Country Data.
public static final String DATABASE_COUNTRY_PATH = "F:/GeoLite2/GeoLite2-Country.mmdb";
// City Data.
public static final String DATABASE_CITY_PATH = "F:/GeoLite2/GeoLite2-City.mmdb";
public static final int MY_USER_ID = 42;
public static final String MY_LICENSE_KEY = "license_key";
}
HelloGeoIP2.java
package org.o7planning.geoip2tutorial;
import java.io.File;
import java.io.IOException;
import java.net.InetAddress;
import com.maxmind.geoip2.DatabaseReader;
import com.maxmind.geoip2.exception.GeoIp2Exception;
import com.maxmind.geoip2.model.CityResponse;
import com.maxmind.geoip2.record.City;
import com.maxmind.geoip2.record.Country;
import com.maxmind.geoip2.record.Location;
import com.maxmind.geoip2.record.Postal;
import com.maxmind.geoip2.record.Subdivision;
public class HelloGeoIP2 {
public static void main(String[] args) throws IOException, GeoIp2Exception {
// A File object pointing to your GeoLite2 database
File dbFile = new File(MyConstants.DATABASE_CITY_PATH);
// This creates the DatabaseReader object,
// which should be reused across lookups.
DatabaseReader reader = new DatabaseReader.Builder(dbFile).build();
// A IP Address
InetAddress ipAddress = InetAddress.getByName("128.101.101.101");
// Get City info
CityResponse response = reader.city(ipAddress);
// Country Info
Country country = response.getCountry();
System.out.println("Country IsoCode: "+ country.getIsoCode()); // 'US'
System.out.println("Country Name: "+ country.getName()); // 'United States'
System.out.println(country.getNames().get("zh-CN")); // '美国'
Subdivision subdivision = response.getMostSpecificSubdivision();
System.out.println("Subdivision Name: " +subdivision.getName()); // 'Minnesota'
System.out.println("Subdivision IsoCode: "+subdivision.getIsoCode()); // 'MN'
// City Info.
City city = response.getCity();
System.out.println("City Name: "+ city.getName()); // 'Minneapolis'
// Postal info
Postal postal = response.getPostal();
System.out.println(postal.getCode()); // '55455'
// Geo Location info.
Location location = response.getLocation();
// Latitude
System.out.println("Latitude: "+ location.getLatitude()); // 44.9733
// Longitude
System.out.println("Longitude: "+ location.getLongitude()); // -93.2323
}
}
Das Beispiel durchführen
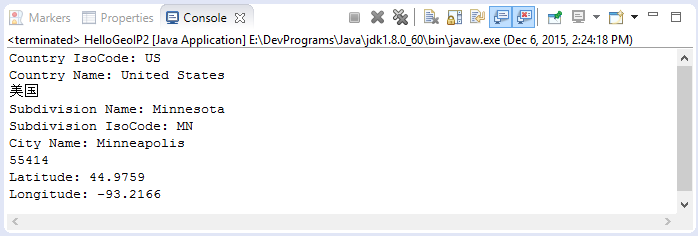
6. Zum Beispiel: GeoIP2 Web Service
Statt der Verbindung mit einem lokalen Datenbank zur Aufnahme der IP entsprechenden geographischen Information können Sie ein Webservice zur Datenversorgung benutzen. Sie sollen ein LICENSE_KEY kaufen. Das folgende Beispiel führt Sie bei der Aufnahme der geographischen Information mit der entsprechenden IP-Addresse durch die Web Service.
HelloGeoIP2Service.java
package org.o7planning.geoip2tutorial;
import java.io.IOException;
import java.net.InetAddress;
import com.maxmind.geoip2.WebServiceClient;
import com.maxmind.geoip2.exception.GeoIp2Exception;
import com.maxmind.geoip2.model.CountryResponse;
import com.maxmind.geoip2.record.Country;
public class HelloGeoIP2Service {
public static void main(String[] args) throws IOException, GeoIp2Exception {
WebServiceClient.Builder builder
= new WebServiceClient.Builder(MyConstants.MY_USER_ID, MyConstants.MY_LICENSE_KEY);
WebServiceClient client = builder.build();
// IP Address
InetAddress ipAddress = InetAddress.getByName("128.101.101.101");
// Do the lookup
CountryResponse response = client.country(ipAddress);
// Country Info .
Country country = response.getCountry();
System.out.println("Country Iso Code: "+ country.getIsoCode()); // 'US'
System.out.println("Country Name: "+ country.getName()); // 'United States'
System.out.println(country.getNames().get("zh-CN")); // '美国'
}
}
Java Open Source Bibliotheken
- Die Anleitung zu Java JSON Processing API (JSONP)
- Verwenden Sie Scribe OAuth Java API mit Google OAuth 2
- Hardware-Informationen in der Java-Anwendung abrufen
- Restfb Java API für Facebook
- Erstellen Sie Credentials für Google Drive API
- Die Anleitung zu Java JDOM2
- Die Anleitung zu Java XStream
- Verwenden Sie Java Jsoup Parsing HTML
- Rufen Sie geografische Informationen basierend auf der IP-Adresse mit GeoIP2JavaAPI ab
- Lesen und Schreiben von Excel-Dateien in Java mit Apache POI
- Entdecken Sie die Facebook Graph API
- Manipulieren von Dateien und Ordnern auf Google Drive mithilfe von Java
Show More