Lesen und Schreiben von Excel-Dateien in Java mit Apache POI
1. Was ist Apache POI?
Apache POI ist ein Open Source Bibliothek Java, wird von Apache gebieten. Dieser Bibliothek hilft Ihnen bei der Umgang mit der Microsoft Dockumente wie Word, Excel, Power point, Visio,...
POI ist die Kurzbezeichnung von "Poor Obfuscation Implementation". Die File Format der Microsoft werden versteckt. Der Ingenieur der Apache versuchten, es zu kennen und Sie fand, dass Microsoft die sehr komplizierten Format gemacht. Und der Name von Bibliothek kommt aus der Humor .
Poor Obfuscation Implementation: Die Durchführung der armen Verschleierung (nach der Übersetzter)
POI ist die Kurzbezeichnung von "Poor Obfuscation Implementation". Die File Format der Microsoft werden versteckt. Der Ingenieur der Apache versuchten, es zu kennen und Sie fand, dass Microsoft die sehr komplizierten Format gemacht. Und der Name von Bibliothek kommt aus der Humor .
Poor Obfuscation Implementation: Die Durchführung der armen Verschleierung (nach der Übersetzter)
In diesem Unterlagen gebe ich die Hinweise bei der Einführung von Apache POI für die Excel - Umgang
2. Die Überblick über Apache POI
Apache POI hilft Ihnen bei der Arbeiten mit Microsoft Format, seine Class haben die üblich Präfix HSSF, XSSF, HPSF, ... Wenn Sie die Präfix einer Class sehen, können Sie wissen, welche Format diese Class unterstützen
Zum Beispiel : Wenn Sie mit der Excel (XLS) arbeiten möchten, brauchen Sie die folgenden Class
- HSSFWorkbook
- HSSFSheet
- HSSFCellStyle
- HSSFDataFormat
- HSSFFont
- ...
Prefix | Description | |
1 | HSSF (Horrible SpreadSheet Format) | reads and writes Microsoft Excel (XLS) format files. |
2 | XSSF (XML SpreadSheet Format) | reads and writes Office Open XML (XLSX) format files. |
3 | HPSF (Horrible Property Set Format) | reads “Document Summary” information from Microsoft Office files. |
4 | HWPF (Horrible Word Processor Format) | aims to read and write Microsoft Word 97 (DOC) format files. |
5 | HSLF (Horrible Slide Layout Format) | a pure Java implementation for Microsoft PowerPoint files. |
6 | HDGF (Horrible DiaGram Format) | an initial pure Java implementation for Microsoft Visio binary files. |
7 | HPBF (Horrible PuBlisher Format) | a pure Java implementation for Microsoft Publisher files. |
8 | HSMF (Horrible Stupid Mail Format) | a pure Java implementation for Microsoft Outlook MSG files |
9 | DDF (Dreadful Drawing Format) | a package for decoding the Microsoft Office Drawing format. |
3. Die Überblick über Apache POI Excel
Beispiel unten bezeichnet ein Excel Struktur
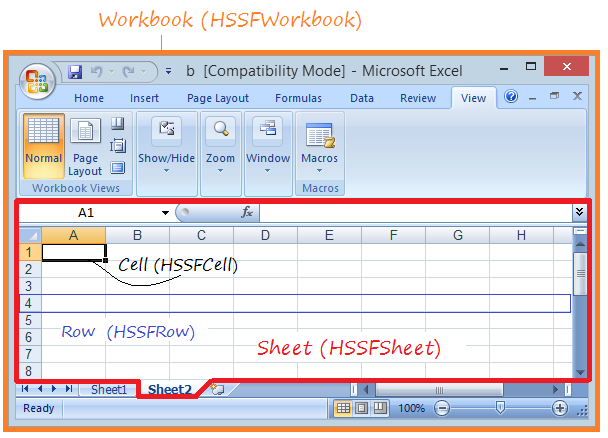
Apache POI bringt Sie die interface Workbook, Sheet, Row, Cell,... und die entsprechenden Durchführungsclass (Implementation) wie HSSFWorkbook, HSSFSheet, HSSFRow, HSSFCell,...
4. Die Bibliothek von Apache POI
Wenn Ihr Projekt Maven läuft, melden Sie einfach die Bibliothek in pom.xml an
<!-- https://mvnrepository.com/artifact/org.apache.poi/poi -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>3.17</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>3.17</version>
</dependency>
Beim Nichtanwendung der Maven können Sie die Bibliothek Apache POI herunterladen in ...
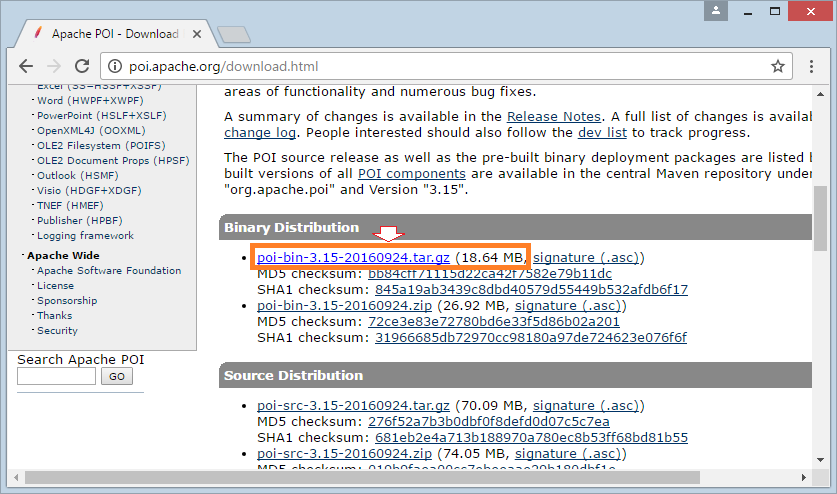
Herunterladen und entpacken: brauchen Sie mindesten 3 Jar file bei Umgang mit Excel
- poi-**.jar
- lib/commons-codec-**.jar
- lib/commons-collections4-**.jar
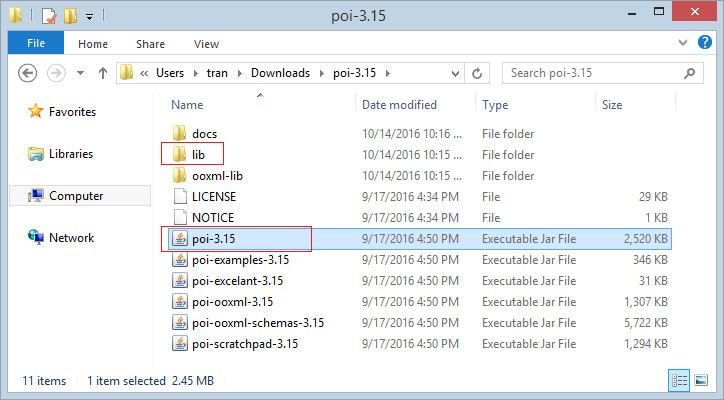
In diesem Unterlagen erstelle ich ein einfacher Projekt Maven mit der Name ApachePOIExcel
- Group ID: org.o7planning
- Artifact ID: ApachePOIExcel
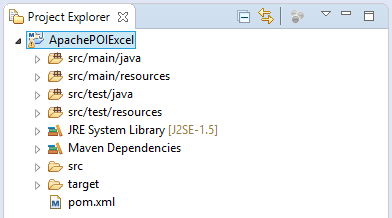
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>ApachePOIExcel</artifactId>
<version>0.0.1-SNAPSHOT</version>
<dependencies>
<!-- https://mvnrepository.com/artifact/org.apache.poi/poi -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>3.17</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>3.17</version>
</dependency>
</dependencies>
</project>
5. Die File Excel erstellen und aufschreiben
Microsoft Office von der vorherige Version (97-2003) hat die Excel file mit der XLS Format und die neuen Version mit XSLX Format. Um mit der XSL File zu arbeiten, brauchen Sie die Class mit der Präfix HSSF und die Class mit der Präfix XSSF für die XSLX File Format
Unten ist das einfache Beispiel für die Erstellung einer Exel File. Sie können mit Style in der Zelle (Cell) für die Erstellung einer schöner Excel Dokument verbinden. POI Style wird in Detail am Ende des Unterlagen gemeint.
CreateExcelDemo.java
package org.o7planning.apachepoiexcel.demo;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.List;
import org.apache.poi.hssf.usermodel.HSSFCellStyle;
import org.apache.poi.hssf.usermodel.HSSFFont;
import org.apache.poi.hssf.usermodel.HSSFSheet;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.CellType;
import org.apache.poi.ss.usermodel.Row;
import org.o7planning.apachepoiexcel.model.Employee;
import org.o7planning.apachepoiexcel.model.EmployeeDAO;
public class CreateExcelDemo {
private static HSSFCellStyle createStyleForTitle(HSSFWorkbook workbook) {
HSSFFont font = workbook.createFont();
font.setBold(true);
HSSFCellStyle style = workbook.createCellStyle();
style.setFont(font);
return style;
}
public static void main(String[] args) throws IOException {
HSSFWorkbook workbook = new HSSFWorkbook();
HSSFSheet sheet = workbook.createSheet("Employees sheet");
List<Employee> list = EmployeeDAO.listEmployees();
int rownum = 0;
Cell cell;
Row row;
//
HSSFCellStyle style = createStyleForTitle(workbook);
row = sheet.createRow(rownum);
// EmpNo
cell = row.createCell(0, CellType.STRING);
cell.setCellValue("EmpNo");
cell.setCellStyle(style);
// EmpName
cell = row.createCell(1, CellType.STRING);
cell.setCellValue("EmpNo");
cell.setCellStyle(style);
// Salary
cell = row.createCell(2, CellType.STRING);
cell.setCellValue("Salary");
cell.setCellStyle(style);
// Grade
cell = row.createCell(3, CellType.STRING);
cell.setCellValue("Grade");
cell.setCellStyle(style);
// Bonus
cell = row.createCell(4, CellType.STRING);
cell.setCellValue("Bonus");
cell.setCellStyle(style);
// Data
for (Employee emp : list) {
rownum++;
row = sheet.createRow(rownum);
// EmpNo (A)
cell = row.createCell(0, CellType.STRING);
cell.setCellValue(emp.getEmpNo());
// EmpName (B)
cell = row.createCell(1, CellType.STRING);
cell.setCellValue(emp.getEmpName());
// Salary (C)
cell = row.createCell(2, CellType.NUMERIC);
cell.setCellValue(emp.getSalary());
// Grade (D)
cell = row.createCell(3, CellType.NUMERIC);
cell.setCellValue(emp.getGrade());
// Bonus (E)
String formula = "0.1*C" + (rownum + 1) + "*D" + (rownum + 1);
cell = row.createCell(4, CellType.FORMULA);
cell.setCellFormula(formula);
}
File file = new File("C:/demo/employee.xls");
file.getParentFile().mkdirs();
FileOutputStream outFile = new FileOutputStream(file);
workbook.write(outFile);
System.out.println("Created file: " + file.getAbsolutePath());
}
}
Employee.java
package org.o7planning.apachepoiexcel.model;
public class Employee {
private String empNo;
private String empName;
private Double salary;
private int grade;
private Double bonus;
public Employee(String empNo, String empName,//
Double salary, int grade, Double bonus) {
this.empNo = empNo;
this.empName = empName;
this.salary = salary;
this.grade = grade;
this.bonus = bonus;
}
public String getEmpNo() {
return empNo;
}
public void setEmpNo(String empNo) {
this.empNo = empNo;
}
public String getEmpName() {
return empName;
}
public void setEmpName(String empName) {
this.empName = empName;
}
public Double getSalary() {
return salary;
}
public void setSalary(Double salary) {
this.salary = salary;
}
public int getGrade() {
return grade;
}
public void setGrade(int grade) {
this.grade = grade;
}
public Double getBonus() {
return bonus;
}
public void setBonus(Double bonus) {
this.bonus = bonus;
}
}
EmployeeDAO.java
package org.o7planning.apachepoiexcel.model;
import java.util.ArrayList;
import java.util.List;
public class EmployeeDAO {
public static List<Employee> listEmployees() {
List<Employee> list = new ArrayList<Employee>();
Employee e1 = new Employee("E01", "Tom", 200.0, 1, null);
Employee e2 = new Employee("E02", "Jerry", 100.2, 2, null);
Employee e3 = new Employee("E03", "Donald", 150.0, 2, null);
list.add(e1);
list.add(e2);
list.add(e3);
return list;
}
}
Beispiel
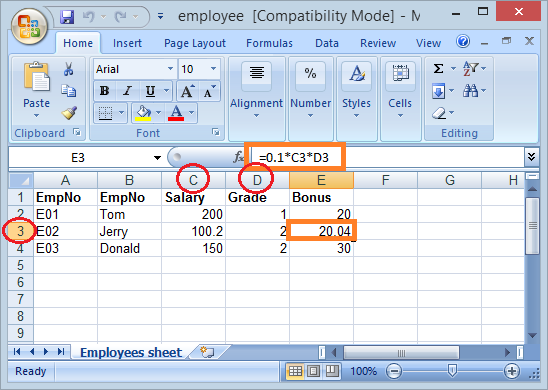
6. Die File xsl und xslx lesen
Das unten Beispiel liest ein einfache Excel File und schreiben in der Console. Diese File Excel ist die oben erstellte Excell File
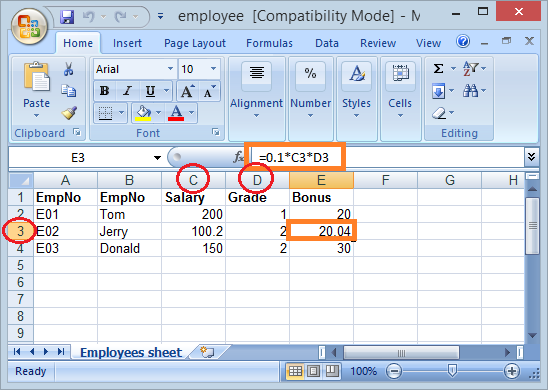
Notiz: In diesem Unterlagen benutze ich die Version Apache POI 3.15 und API hat mehr Veränderungen im Vergleich von der alten Version. Viele Methode wird in der nächsten Version (Apache POI 4.x) gelöscht werden. POI hat vor, die Enum statt die Konstante in API zu benutzen
ReadExcelDemo.java
package org.o7planning.apachepoiexcel.demo;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.util.Iterator;
import org.apache.poi.hssf.usermodel.HSSFSheet;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.CellType;
import org.apache.poi.ss.usermodel.FormulaEvaluator;
import org.apache.poi.ss.usermodel.Row;
public class ReadExcelDemo {
public static void main(String[] args) throws IOException {
// Read XSL file
FileInputStream inputStream = new FileInputStream(new File("C:/demo/employee.xls"));
// Get the workbook instance for XLS file
HSSFWorkbook workbook = new HSSFWorkbook(inputStream);
// Get first sheet from the workbook
HSSFSheet sheet = workbook.getSheetAt(0);
// Get iterator to all the rows in current sheet
Iterator<Row> rowIterator = sheet.iterator();
while (rowIterator.hasNext()) {
Row row = rowIterator.next();
// Get iterator to all cells of current row
Iterator<Cell> cellIterator = row.cellIterator();
while (cellIterator.hasNext()) {
Cell cell = cellIterator.next();
// Change to getCellType() if using POI 4.x
CellType cellType = cell.getCellTypeEnum();
switch (cellType) {
case _NONE:
System.out.print("");
System.out.print("\t");
break;
case BOOLEAN:
System.out.print(cell.getBooleanCellValue());
System.out.print("\t");
break;
case BLANK:
System.out.print("");
System.out.print("\t");
break;
case FORMULA:
// Formula
System.out.print(cell.getCellFormula());
System.out.print("\t");
FormulaEvaluator evaluator = workbook.getCreationHelper().createFormulaEvaluator();
// Print out value evaluated by formula
System.out.print(evaluator.evaluate(cell).getNumberValue());
break;
case NUMERIC:
System.out.print(cell.getNumericCellValue());
System.out.print("\t");
break;
case STRING:
System.out.print(cell.getStringCellValue());
System.out.print("\t");
break;
case ERROR:
System.out.print("!");
System.out.print("\t");
break;
}
}
System.out.println("");
}
}
}
Beispiel
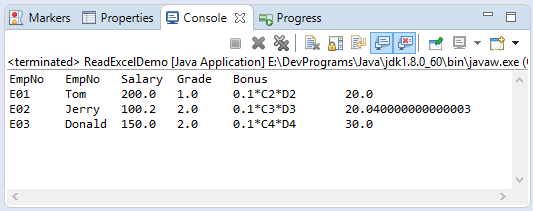
7. Die verfügbare File Excel aktualisieren (update)
In diesem Beispiel lese ich Excel file employee.xls und die Wert in der Spalte Salary doppelt updaten
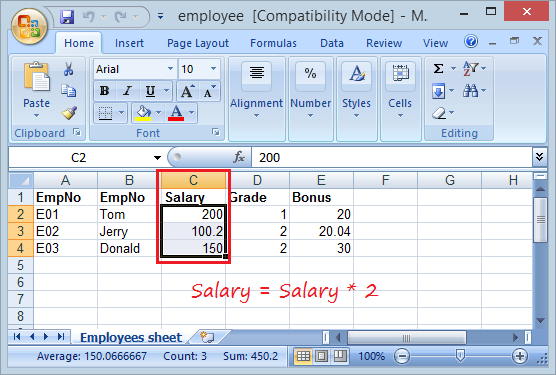
UpdateExcelDemo.java
package org.o7planning.apachepoiexcel.demo;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import org.apache.poi.hssf.usermodel.HSSFCell;
import org.apache.poi.hssf.usermodel.HSSFSheet;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
public class UpdateExcelDemo {
public static void main(String[] args) throws IOException {
File file = new File("C:/demo/employee.xls");
// Read XSL file
FileInputStream inputStream = new FileInputStream(file);
// Get the workbook instance for XLS file
HSSFWorkbook workbook = new HSSFWorkbook(inputStream);
// Get first sheet from the workbook
HSSFSheet sheet = workbook.getSheetAt(0);
HSSFCell cell = sheet.getRow(1).getCell(2);
cell.setCellValue(cell.getNumericCellValue() * 2);
cell = sheet.getRow(2).getCell(2);
cell.setCellValue(cell.getNumericCellValue() * 2);
cell = sheet.getRow(3).getCell(2);
cell.setCellValue(cell.getNumericCellValue() * 2);
inputStream.close();
// Write File
FileOutputStream out = new FileOutputStream(file);
workbook.write(out);
out.close();
}
}
Das Ergebnis nach der Aktualisierung
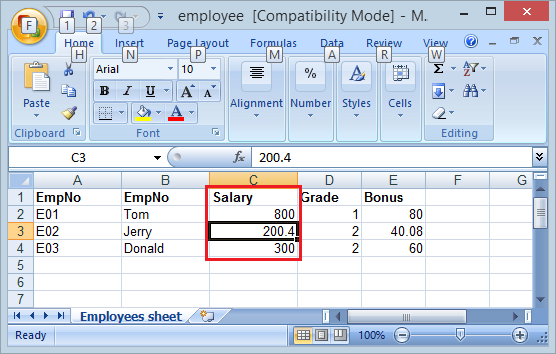
8. Das Formular und die Bewertung
Wenn Sie die Kenntnis über Excel haben. ist es Ihnen nicht schwierig eine Formel zu machen. Mit Apache POI können Sie ein Cell CellType.FORMULA, wird seine Wert auf der Formelbasic kalkuliert
SUM
Beispiel: Die Summe der Spalte C von Zeile 2 zu Zeile 4 kalkulieren
// Create Cell type of FORMULA
cell = row.createCell(rowIndex, CellType.FORMULA);
// Set formula
cell.setCellFormula("SUM(C2:C4)");
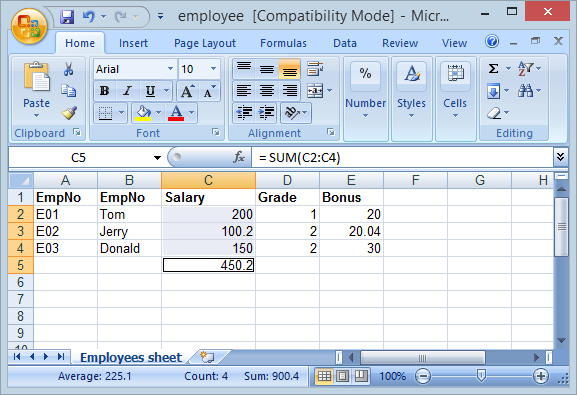
Formel für die Kalkulation jeder einzelnen Zelle
cell = row.createCell(rowIndex, CellType.FORMULA);
cell.setCellFormula("0.1*C2*D3");
Mit einer Zelle, die FORMULA hat, können Sie ihre Formel drücken und benutzen die FormulaEvaluator für Wertkalkulation der ZelleWert durch die Formel.
// Formula
String formula = cell.getCellFormula();
FormulaEvaluator evaluator
= workbook.getCreationHelper().createFormulaEvaluator();
// CellValue
CellValue cellValue = evaluator.evaluate(cell);
double value = cellValue.getNumberValue();
String value = cellValue.getStringValue();
boolean value = cellValue.getBooleanValue();
// ...
9. Das Stil (Style) anwenden
Beispiel
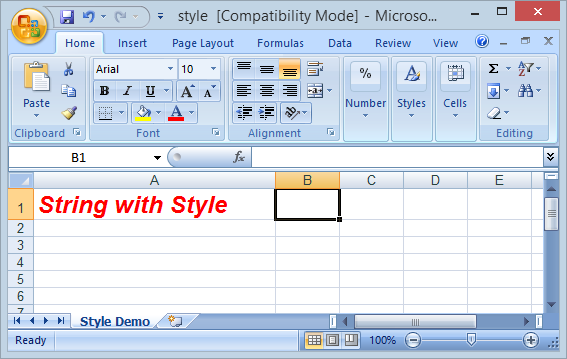
StyleDemo.java
package org.o7planning.apachepoiexcel.demo;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import org.apache.poi.hssf.usermodel.HSSFCell;
import org.apache.poi.hssf.usermodel.HSSFCellStyle;
import org.apache.poi.hssf.usermodel.HSSFFont;
import org.apache.poi.hssf.usermodel.HSSFRow;
import org.apache.poi.hssf.usermodel.HSSFSheet;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
import org.apache.poi.ss.usermodel.IndexedColors;
public class StyleDemo {
private static HSSFCellStyle getSampleStyle(HSSFWorkbook workbook) {
// Font
HSSFFont font = workbook.createFont();
font.setBold(true);
font.setItalic(true);
// Font Height
font.setFontHeightInPoints((short) 18);
// Font Color
font.setColor(IndexedColors.RED.index);
// Style
HSSFCellStyle style = workbook.createCellStyle();
style.setFont(font);
return style;
}
public static void main(String[] args) throws IOException {
HSSFWorkbook workbook = new HSSFWorkbook();
HSSFSheet sheet = workbook.createSheet("Style Demo");
HSSFRow row = sheet.createRow(0);
//
HSSFCell cell = row.createCell(0);
cell.setCellValue("String with Style");
HSSFCellStyle style = getSampleStyle(workbook);
cell.setCellStyle(style);
File file = new File("C:/demo/style.xls");
file.getParentFile().mkdirs();
FileOutputStream outFile = new FileOutputStream(file);
workbook.write(outFile);
System.out.println("Created file: " + file.getAbsolutePath());
}
}
Java Open Source Bibliotheken
- Die Anleitung zu Java JSON Processing API (JSONP)
- Verwenden Sie Scribe OAuth Java API mit Google OAuth 2
- Hardware-Informationen in der Java-Anwendung abrufen
- Restfb Java API für Facebook
- Erstellen Sie Credentials für Google Drive API
- Die Anleitung zu Java JDOM2
- Die Anleitung zu Java XStream
- Verwenden Sie Java Jsoup Parsing HTML
- Rufen Sie geografische Informationen basierend auf der IP-Adresse mit GeoIP2JavaAPI ab
- Lesen und Schreiben von Excel-Dateien in Java mit Apache POI
- Entdecken Sie die Facebook Graph API
- Manipulieren von Dateien und Ordnern auf Google Drive mithilfe von Java
Show More