Hardware-Informationen in der Java-Anwendung abrufen
1. Java-API zur Aufnahme der Information von PC-Hardware?
Manchmals brauchen Sie Java benutzen um die Information vom Hardware vom Computer zu haben, einschließend die Serial Nummer vom Mainboard, serial von der Festplatte, CPU ...
Leider hat Java keine solche API, oder vielleicht könnte es haben, aber es ist nicht umsonst für den Programmer. Allerdings können Sie auf Windows durch die Durchfürhung der VB Scripts diese Information nehmen
Leider hat Java keine solche API, oder vielleicht könnte es haben, aber es ist nicht umsonst für den Programmer. Allerdings können Sie auf Windows durch die Durchfürhung der VB Scripts diese Information nehmen
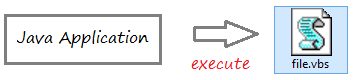
2. Die Aufnahme von Hardware-Information durch die File vbscript
OK, um einfach zu sein, können Sie eine File myscript.vbs mit der Inhalt erstellen
myscript.vbs
Set objWMIService = GetObject("winmgmts:\\.\root\cimv2")
Set colItems = objWMIService.ExecQuery _
("Select Name,UUID,Vendor,Version from Win32_ComputerSystemProduct")
For Each objItem in colItems
Wscript.Echo objItem.Name
Wscript.Echo objItem.UUID
Wscript.Echo objItem.Vendor
Wscript.Echo objItem.Version
Next
Auf Windows klicken Sie auf diese File um es zu starten
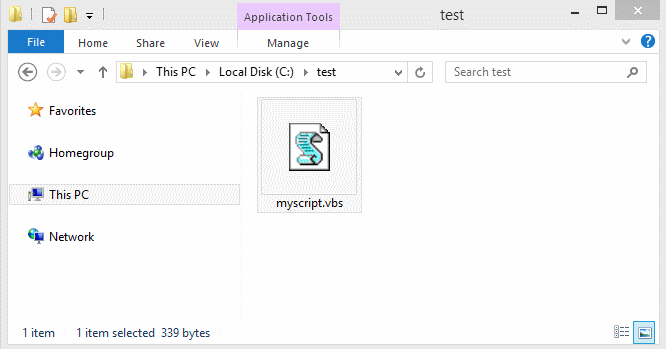
Davon ist Win32_ComputerSystemProduct eine Klasse von Visual Basic. Die Attribute von dieser Klasse sind
Properties |
string Caption |
string Description |
string IdentifyingNumber |
string Name |
string SKUNumber |
string UUID |
string Vendor |
string Version |
Sie können eine File mit einer vollen Script starten:
Win32_ComputerSystemProduct.vbs
Set objWMIService = GetObject("winmgmts:\\.\root\cimv2")
Set colItems = objWMIService.ExecQuery _
("Select * from Win32_ComputerSystemProduct")
For Each objItem in colItems
Wscript.Echo objItem.Caption
Wscript.Echo objItem.Description
Wscript.Echo objItem.IdentifyingNumber
Wscript.Echo objItem.Name
Wscript.Echo objItem.SKUNumber
Wscript.Echo objItem.UUID
Wscript.Echo objItem.Vendor
Wscript.Echo objItem.Version
Next
Die erhalteten Werte (In meinem Computer):
Property | Value (My Computer) |
Caption | Computer System Product |
Description | Computer System Product |
IdentifyingNumber | 3F027935U |
Name | Salellite S75B |
SKUNumber | null |
UUID | B09366C5-F0C7-E411-98E4-008CFA8C26DF |
Vendor | TOSHIBA |
Version | PSPPJU-07U051 |
Mehr Information der Klasse Win32_ComputerSystemProduct bei... sehen:
Enige Klasse von Visual Basic können Sie gucken
3. Benutzen Sie Java um die Hardware-Information aufzunehmen
OK, in der oben Teil haben Sie schon die VB Script gekannt um die Information vom Hardware des Computer zu haben. Jetzt brauchen Sie die Java zu benutzen um die File VB Scripts durchzuführen und die zurückgegebenen Werte zu nehmen
MyUtility.java
package org.o7planning.hardwareinfo;
public class MyUtility {
public static String makeVbScript(String vbClassName, String[] propNames) {
StringBuilder sb = new StringBuilder();
for (int i = 0; i < propNames.length; i++) {
if (i < propNames.length - 1) {
sb.append(propNames[i]).append(",");
} else {
sb.append(propNames[i]);
}
}
String colNameString = sb.toString();
sb.setLength(0);
sb.append("Set objWMIService = GetObject(\"winmgmts:\\\\.\\root\\cimv2\")").append("\n");
sb.append("Set colItems = objWMIService.ExecQuery _ ").append("\n");
sb.append("(\"Select ").append(colNameString).append(" from ").append(vbClassName).append("\") ").append("\n");
sb.append("For Each objItem in colItems ").append("\n");
for (String propName : propNames) {
sb.append(" Wscript.Echo objItem.").append(propName).append("\n");
}
sb.append("Next ").append("\n");
return sb.toString();
}
}
GetHardwareInfo.java
package org.o7planning.hardwareinfo;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileWriter;
import java.io.InputStreamReader;
import java.util.HashMap;
import java.util.Map;
public class GetHardwareInfo {
public static void printComputerSystemProductInfo() {
String vbClassName = "Win32_ComputerSystemProduct";
String[] propNames = new String[] { "Name", "UUID", "Vendor", "Version" };
String vbScript = MyUtility.makeVbScript(vbClassName, propNames);
System.out.println("----------------------------------------");
System.out.println(vbScript);
System.out.println("----------------------------------------");
try {
// Create temporary file.
File file = File.createTempFile("vbsfile", ".vbs");
System.out.println("Create File: " + file.getAbsolutePath());
System.out.println("------");
// Write script content to file.
FileWriter fw = new FileWriter(file);
fw.write(vbScript);
fw.close();
// Execute the file.
Process p = Runtime.getRuntime().exec("cscript //NoLogo " + file.getPath());
// Create Input stream to read data returned after execute vb script file.
BufferedReader input = new BufferedReader(new InputStreamReader(p.getInputStream()));
Map<String, String> map = new HashMap<String, String>();
String line;
int i = 0;
while ((line = input.readLine()) != null) {
if (i >= propNames.length) {
break;
}
String key = propNames[i];
map.put(key, line);
i++;
}
input.close();
//
for (String propName : propNames) {
System.out.println(propName + " : " + map.get(propName));
}
} catch (Exception e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
printComputerSystemProductInfo();
}
}
Das Beispiel starten
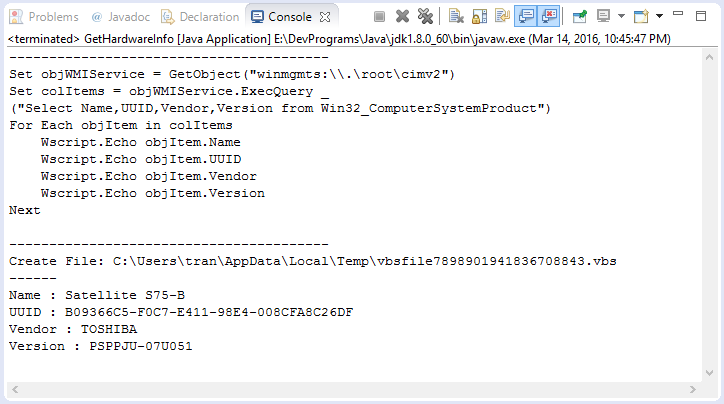
Java Open Source Bibliotheken
- Die Anleitung zu Java JSON Processing API (JSONP)
- Verwenden Sie Scribe OAuth Java API mit Google OAuth 2
- Hardware-Informationen in der Java-Anwendung abrufen
- Restfb Java API für Facebook
- Erstellen Sie Credentials für Google Drive API
- Die Anleitung zu Java JDOM2
- Die Anleitung zu Java XStream
- Verwenden Sie Java Jsoup Parsing HTML
- Rufen Sie geografische Informationen basierend auf der IP-Adresse mit GeoIP2JavaAPI ab
- Lesen und Schreiben von Excel-Dateien in Java mit Apache POI
- Entdecken Sie die Facebook Graph API
- Manipulieren von Dateien und Ordnern auf Google Drive mithilfe von Java
Show More