Konfigurieren Sie statische Ressourcen in Spring MVC
1. Das Zweck des Unterlagen
Der Unterlagen wird nach der Quelle von ... geschrieben
Eclipse 4.6 (NEON)
Spring 4 MVC
Im Unterlagen leite ich Sie bei der Konfiguration der static Data Sources im Spring MVC an, Die static Data sources sind oft die Foto-File, die css File und javascript,...
Spring MVC erlaubt Sie die Abbildung zwischen einen URL mit einem bestimmten Position von Data Source. Sie können das folgende Beispiel sehen
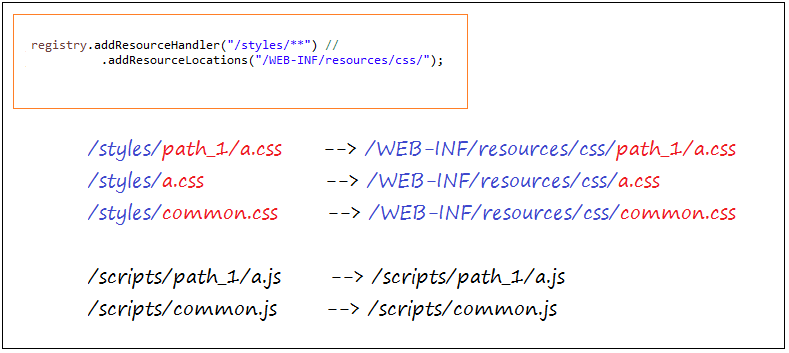
2. Maven Project erstellen
- File/New/Other..
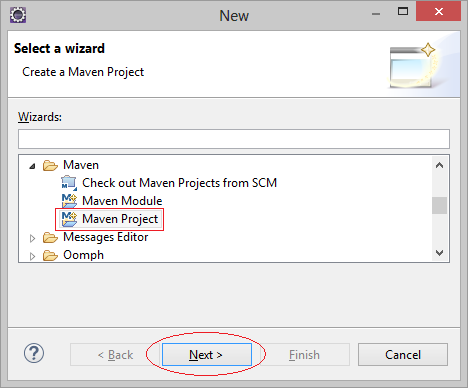
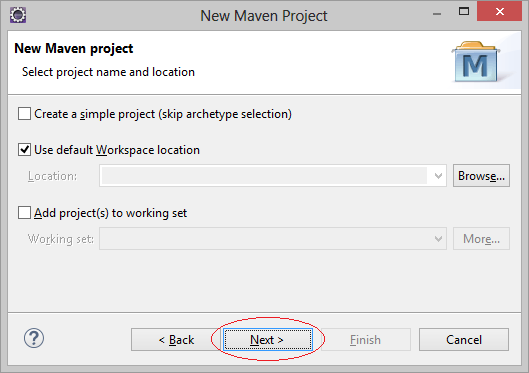
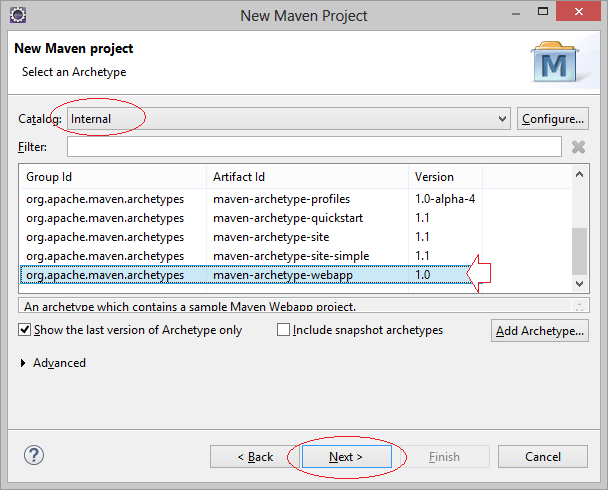
Geben Sie ein
- Group ID: org.o7planning
- Artifact ID: SpringMVCStaticResource
- Package: org.o7planning.tutorial.springmvcresource
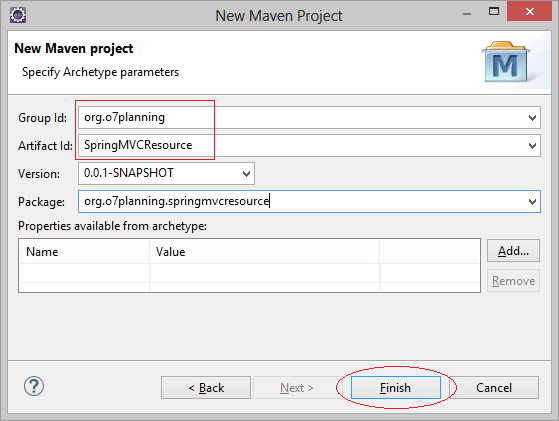
Das Projekt wird erstellt
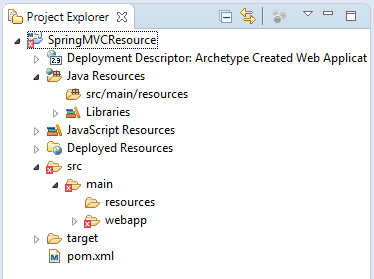
Do not worry with the error message when Project has been created. The reason is that you have not declared Servlet library.
Beachten Sie:
Eclipse erstellt das Projekt Maven, dessen Struktur falsch sein kann. Sie sollen prüfen und verändern
Eclipse erstellt das Projekt Maven, dessen Struktur falsch sein kann. Sie sollen prüfen und verändern
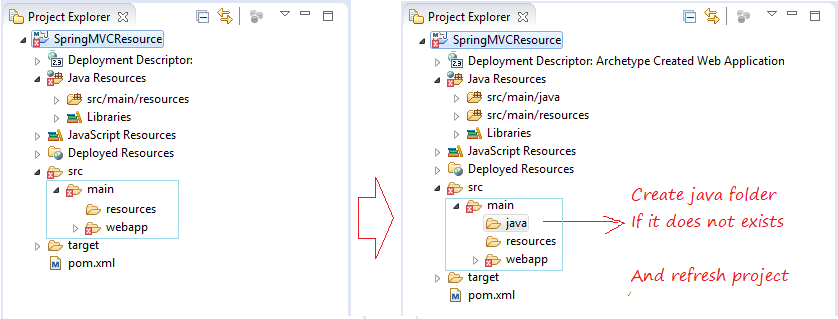
Stellen Sie sicher, dass Ihr Projekt Java >=6 benutzt
Project properties:
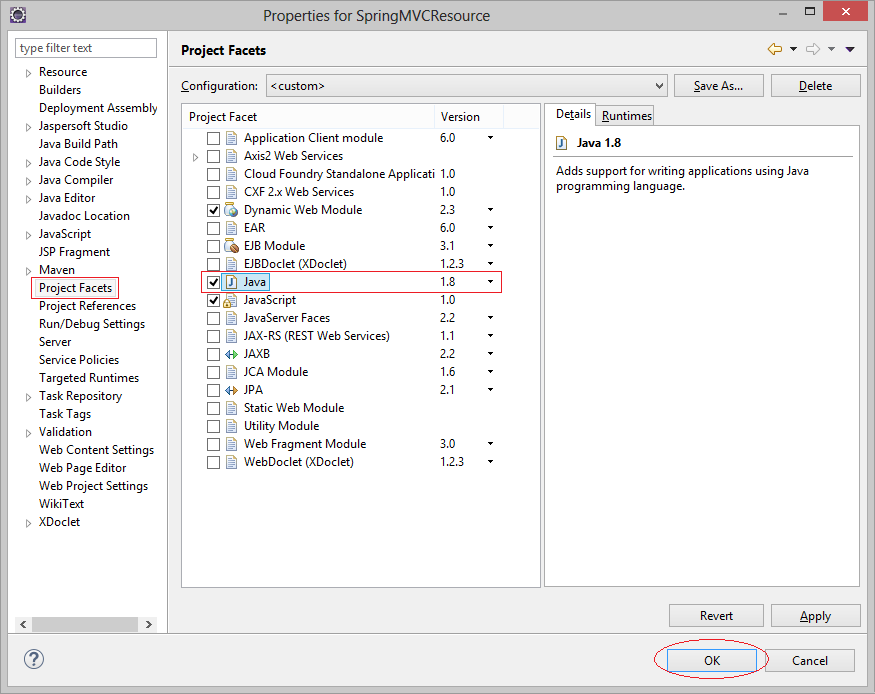
3. Maven & web.xml konfigurieren
web.xml durch die Benutzung von Web App >= 3 benutzen .
web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://java.sun.com/xml/ns/javaee"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd"
id="WebApp_ID" version="3.0">
<display-name>SpringMVCResource</display-name>
</web-app>
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>SpringMVCResource</artifactId>
<packaging>war</packaging>
<version>0.0.1-SNAPSHOT</version>
<name>SpringMVCResource Maven Webapp</name>
<url>http://maven.apache.org</url>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
<!-- Servlet API -->
<!-- http://mvnrepository.com/artifact/javax.servlet/javax.servlet-api -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
<scope>provided</scope>
</dependency>
<!-- Jstl for jsp page -->
<!-- http://mvnrepository.com/artifact/javax.servlet/jstl -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>jstl</artifactId>
<version>1.2</version>
</dependency>
<!-- JSP API -->
<!-- http://mvnrepository.com/artifact/javax.servlet.jsp/jsp-api -->
<dependency>
<groupId>javax.servlet.jsp</groupId>
<artifactId>jsp-api</artifactId>
<version>2.2</version>
<scope>provided</scope>
</dependency>
<!-- Spring dependencies -->
<!-- http://mvnrepository.com/artifact/org.springframework/spring-core -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>4.3.3.RELEASE</version>
</dependency>
<!-- http://mvnrepository.com/artifact/org.springframework/spring-web -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
<version>4.3.3.RELEASE</version>
</dependency>
<!-- http://mvnrepository.com/artifact/org.springframework/spring-webmvc -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>4.3.3.RELEASE</version>
</dependency>
</dependencies>
<build>
<finalName>SpringMVCResource</finalName>
<plugins>
<!-- Config: Maven Tomcat Plugin -->
<!-- http://mvnrepository.com/artifact/org.apache.tomcat.maven/tomcat7-maven-plugin -->
<plugin>
<groupId>org.apache.tomcat.maven</groupId>
<artifactId>tomcat7-maven-plugin</artifactId>
<version>2.2</version>
<!-- Config: contextPath and Port (Default - /SpringMVCResource : 8080) -->
<!--
<configuration>
<path>/</path>
<port>8899</port>
</configuration>
-->
</plugin>
</plugins>
</build>
</project>
4. Spring MVC konfigurieren
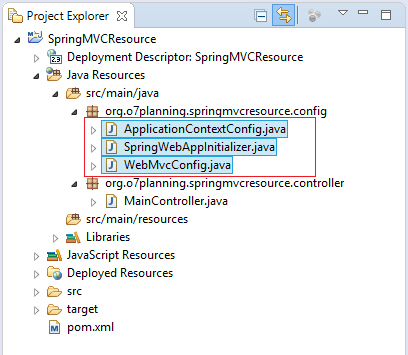
SpringWebAppInitializer.java
package org.o7planning.springmvcresource.config;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.ServletRegistration;
import org.springframework.web.WebApplicationInitializer;
import org.springframework.web.context.support.AnnotationConfigWebApplicationContext;
import org.springframework.web.servlet.DispatcherServlet;
public class SpringWebAppInitializer implements WebApplicationInitializer {
@Override
public void onStartup(ServletContext servletContext) throws ServletException {
AnnotationConfigWebApplicationContext appContext = new AnnotationConfigWebApplicationContext();
appContext.register(ApplicationContextConfig.class);
ServletRegistration.Dynamic dispatcher = servletContext.addServlet("SpringDispatcher",
new DispatcherServlet(appContext));
dispatcher.setLoadOnStartup(1);
dispatcher.addMapping("/");
}
}
ApplicationContextConfig.java
package org.o7planning.springmvcresource.config;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.view.InternalResourceViewResolver;
@Configuration
@ComponentScan("org.o7planning.springmvcresource.*")
public class ApplicationContextConfig {
@Bean(name = "viewResolver")
public InternalResourceViewResolver getViewResolver() {
InternalResourceViewResolver viewResolver = new InternalResourceViewResolver();
viewResolver.setPrefix("/WEB-INF/pages/");
viewResolver.setSuffix(".jsp");
return viewResolver;
}
}
WebMvcConfig.java
package org.o7planning.springmvcresource.config;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.DefaultServletHandlerConfigurer;
import org.springframework.web.servlet.config.annotation.EnableWebMvc;
import org.springframework.web.servlet.config.annotation.ResourceHandlerRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurerAdapter;
@Configuration
@EnableWebMvc
public class WebMvcConfig extends WebMvcConfigurerAdapter {
// Static Resource Config
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
// Css resource.
registry.addResourceHandler("/styles/**") //
.addResourceLocations("/WEB-INF/resources/css/").setCachePeriod(31556926);
}
@Override
public void configureDefaultServletHandling(DefaultServletHandlerConfigurer configurer) {
configurer.enable();
}
}
Achtung
Die Konfiguration von Static Resource:
// Css resource.
registry.addResourceHandler("/styles/**") //
.addResourceLocations("/WEB-INF/resources/css/");
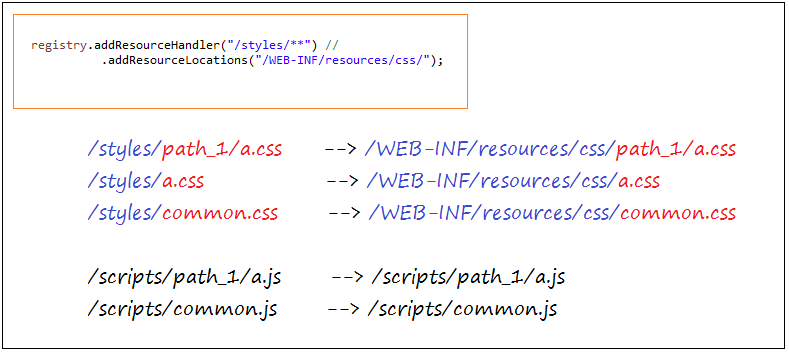
5. Spring Controllers
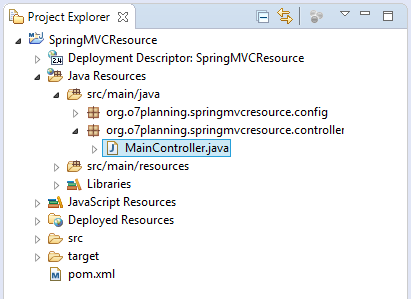
MyController.java
package org.o7planning.springmvcresource.controller;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class MainController {
@RequestMapping(value = "/staticResourceTest")
public String staticResource(Model model) {
return "staticResourceTest";
}
}
6. Static Resource & Views
Static Resource
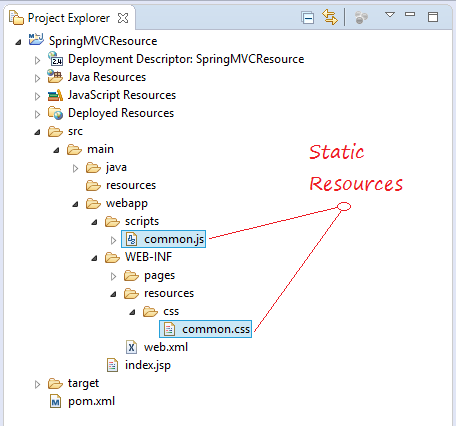
scripts/common.js
function sayHello() {
alert("Hello from JavaScript");
}
/WEB-INF/resource/css/commons.css
.button {
font-size: 20px;
background: #ccc;
}
.red-text {
color: red;
font-size: 30px;
}
.green-text {
color: green;
font-size: 20px;
}
Views
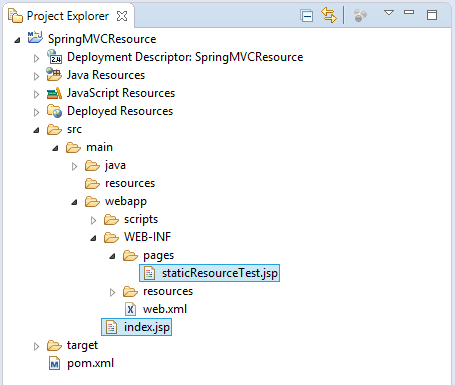
staticResourceTest.jsp
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Spring MVC Resource example</title>
<script type="text/javascript"
src="${pageContext.request.contextPath}/scripts/common.js"></script>
<link rel="stylesheet" type="text/css"
href="${pageContext.request.contextPath}/styles/common.css">
</head>
<body>
<pre>
Config: /styles/** ==> /WEB-INF/resources/css/
----------------------------------------------
/styles/common.css ==> /WEB-INF/resources/css/common.css
/styles/path1/abc.css ==> /WEB-INF/resources/css/path1/abc.css
/styles/path1/path2/abc.css ==> /WEB-INF/resources/css/path1/path2/abc.css
</pre>
<div class="red-text">Red text</div>
<br>
<div class="green-text">Green text</div>
<br>
<input type="button" class="button" onclick="sayHello();"
value="Click me!">
</body>
</html>
index.jsp
<html>
<body>
<a href="staticResourceTest">staticResourceTest</a>
</body>
</html>
7. Die Applikation laufen
Zum ersten Mal sollen Sie vor dem Laufen der Applikation das ganze Projekt bauen ( build ).
Klicken Sie mit der rechten Maustaste auf dem Projekt und wählen Sie
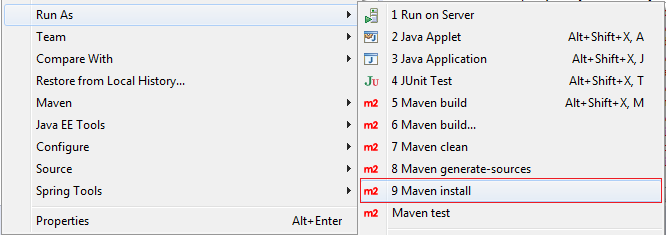
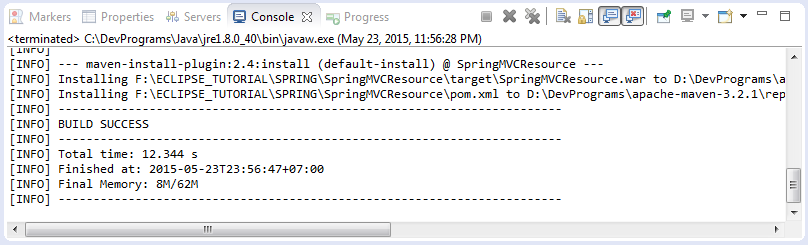
Konfigurieren zu laufen
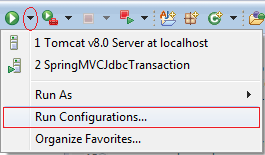
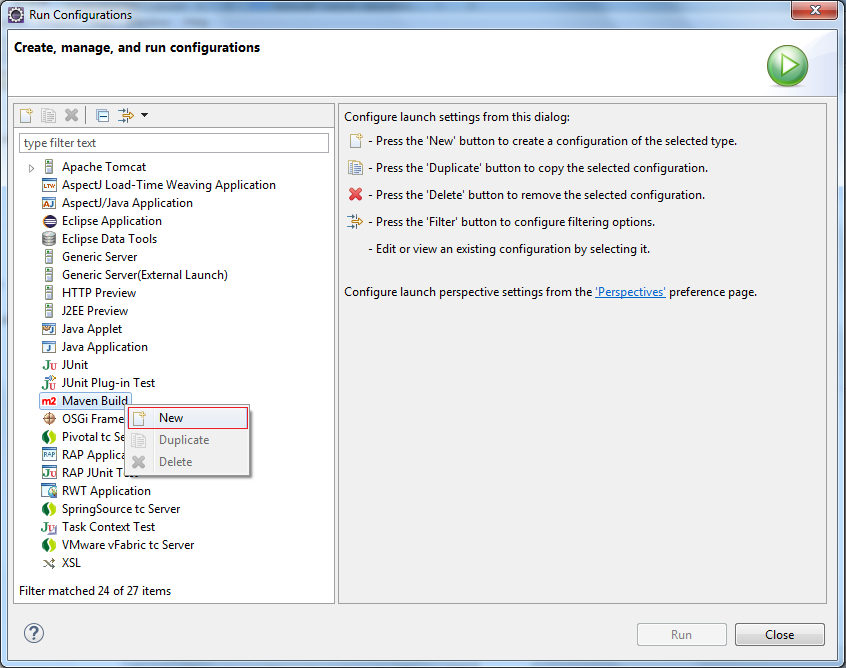
Geben Sie ein
- Name: Run SpringMVCResource
- Base directory: ${workspace_loc:/SpringMVCResource}
- Goals: tomcat7:run
No ADS
Anleitungen Spring MVC
- Die Anleitung zum Sping für den Anfänger
- Installieren Sie die Spring Tool Suite für Eclipse
- Die Anleitung zum Sping MVC für den Anfänger - Hello Spring 4 MVC
- Konfigurieren Sie statische Ressourcen in Spring MVC
- Die Anleitung zu Spring MVC Interceptor
- Erstellen Sie eine mehr Sprachen Web-Anwendung mit Spring MVC
- Datei hochladen und herunterladen mit Spring MVC
- Einfache Anmeldung Java Web Application mit Spring MVC, Spring Security und Spring JDBC
- Social Login in Spring MVC mit Spring Social Security
- Die Anleitung zu Spring MVC mit FreeMarker
- Verwenden Sie Template in Spring MVC mit Apache Tiles
- Hướng dẫn sử dụng Spring MVC và Spring JDBC Transaction
- Verwenden Sie in Spring MVC mehrere DataSources
- Die Anleitung zu Spring MVC Form und Hibernate
- Führen Sie geplante Hintergrundaufgaben in Spring aus
- Erstellen Sie eine Java Shopping Cart Web Application mit Spring MVC und Hibernate
- Einfache CRUD Beispiel mit Spring MVC RESTful Web Service
- Stellen Sie Spring MVC auf Oracle WebLogic Server bereit
Show More