Bedingte Anweisungen if, unless, switch in Thymeleaf
1. th:if, th:unless
In einige Situationen, wenn Sie ein konkretes Stück von Thymeleaf Template in das Ergebnis auftreten möchten wenn eine bestimmte Bedingung als true (richtig) bewertet wird. Um das zu machen, können Sie das Attribut th:if.
Achtung: Im Thymeleaf wird eine Variable oder eine Ausdruck als false (falsch) bewertet wenn ihre Wert null, false, 0, "false", "off", "no" ist. Die anderen Fällen werden als true (richtig) bewertet.
Die Syntax:
<someHtmlTag th:if="condition">
<!-- Other code -->
</someHtmlTag>
<!-- OR: -->
<th:block th:if="condition">
<!-- Other code -->
</th:block>
Ein anderes Attribut, das Sie verwenden können, ist th:unless, Es ist negativ mit th:if.
<someTag th:unless = "condition"> ... </someTag>
<!-- Same as -->
<someTag th:if = "!condition"> ... </someTag>
Example th:if
Das Beispiel mit th:if:
if-example1.html (Template)
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>If/Unless</title>
<style>
.product-container {
padding: 5px;
border: 1px solid #ccc;
margin-top: 10px;
height: 80px;
}
.img-container {
float: left;
margin-right: 5px;
}
</style>
</head>
<body>
<h1>th:if</h1>
<div class="product-container" th:each="product : ${products}">
<!--/* If the product has image, this code will be rendered. */-->
<div class="img-container" th:if="${product.image}">
<img th:src="@{|/${product.image}|}" height="70" />
</div>
<div>
<b>Code:</b> <span th:utext="${product.code}"></span>
</div>
<div>
<b>Name:</b> <span th:utext="${product.name}"></span>
</div>
</div>
</body>
</html>
(Java Spring)
@RequestMapping("/if-example1")
public String ifExample1(Model model) {
Product prod1 = new Product(1L, "SS-S9", "Sam Sung Galaxy S9", "samsung-s9.png");
Product prod2 = new Product(2L, "NK-5P", "Nokia 5.1 Plus", null);
Product prod3 = new Product(3L, "IP-7", "iPhone 7", "iphone-7.jpg");
List<Product> list = new ArrayList<Product>();
list.add(prod1);
list.add(prod2);
list.add(prod3);
model.addAttribute("products", list);
return "if-example1";
}
Product.java
package org.o7planning.thymeleaf.model;
public class Product {
private Long id;
private String code;
private String name;
private String image;
public Product() {
}
public Product(Long id, String code, String name, String image) {
this.id = id;
this.code = code;
this.name = name;
this.image = image;
}
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getCode() {
return code;
}
public void setCode(String code) {
this.code = code;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getImage() {
return image;
}
public void setImage(String image) {
this.image = image;
}
}
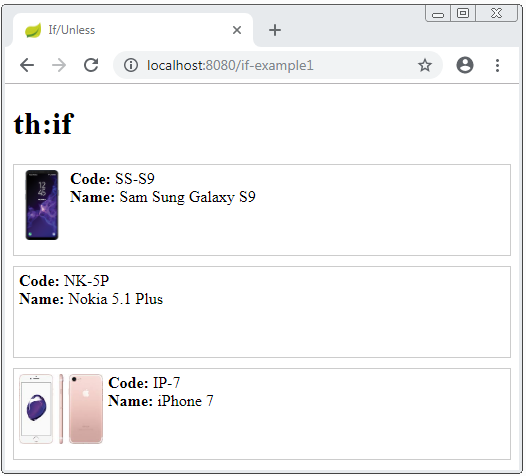
Example th:if, th:unless
Das Beispiel mit th:if und th:unless:
if-unless-example1.html (Template)
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>If/Unless</title>
<style>
.product-container {
padding: 5px;
border: 1px solid #ccc;
margin-top: 10px;
height: 80px;
}
.img-container {
float: left;
margin-right: 5px;
}
</style>
</head>
<body>
<h1>th:if, th:unless</h1>
<div class="product-container" th:each="product : ${products}">
<!--/* If the product has image, this code will be rendered. */-->
<div class="img-container" th:if="${product.image}">
<img th:src="@{|/${product.image}|}" height="70" />
</div>
<!--/* If the product has no image, display default Image. */-->
<div class="img-container" th:unless="${product.image}">
<img th:src="@{/no-image.png}" height="70" />
</div>
<div>
<b>Code:</b> <span th:utext="${product.code}"></span>
</div>
<div>
<b>Name:</b> <span th:utext="${product.name}"></span>
</div>
</div>
</body>
</html>
(Java Spring)
@RequestMapping("/if-unless-example1")
public String ifUnlessExample1(Model model) {
Product prod1 = new Product(1L, "SS-S9", "Sam Sung Galaxy S9", "samsung-s9.png");
Product prod2 = new Product(2L, "NK-5P", "Nokia 5.1 Plus", null);
Product prod3 = new Product(3L, "IP-7", "iPhone 7", "iphone-7.jpg");
List<Product> list = new ArrayList<Product>();
list.add(prod1);
list.add(prod2);
list.add(prod3);
model.addAttribute("products", list);
return "if-unless-example1";
}
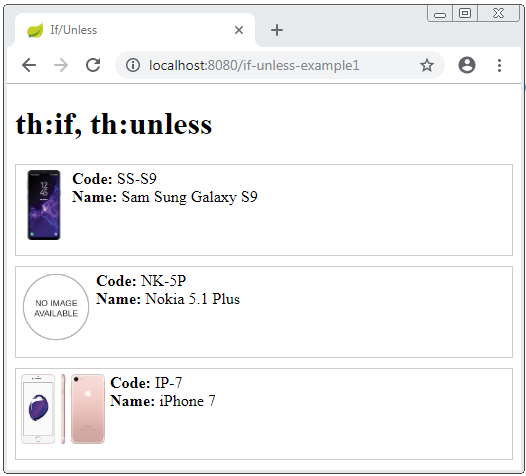
Den Operator Elvis mehr sehen:<!--/* Elvis Operator */--> <p>Age: <span th:utext="${person.age}?: '(no age specified)'">27</span>.</p>
2. th:switch, th:case
Im Java sind Sie an die Struktur switch/case gewöhnt. Thymeleaf hat auch eine ähnliche Struktur. Das ist th:swith/th:case.
<div th:switch="${user.role}">
<p th:case="'admin'">User is an administrator</p>
<p th:case="${roles.manager}">User is a manager</p>
<p th:case="'staff'">User is a staff</p>
</div>
<!-- th:switch/th:case with default case: -->
<div th:switch="${user.role}">
<p th:case="'admin'">User is an administrator</p>
<p th:case="${roles.manager}">User is a manager</p>
<p th:case="'staff'">User is a staff</p>
<p th:case="*">User is some other thing</p>
</div>
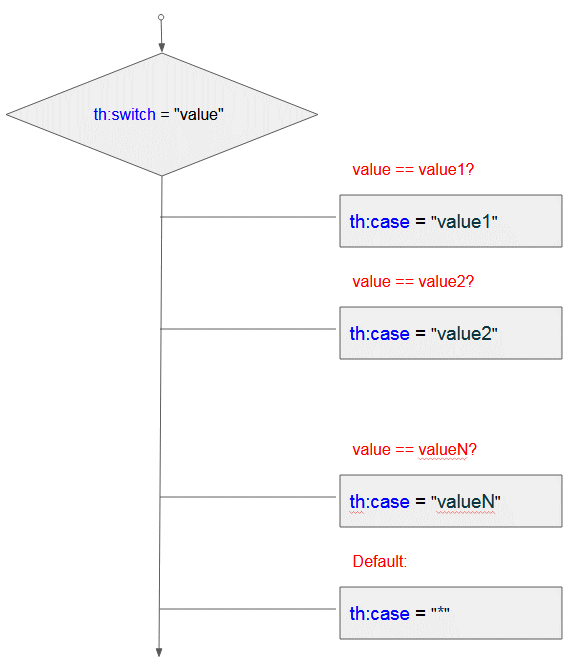
Das Programm werden die case (Fällen) vom unten nach hinten abwechselnd bewerten. Wenn ein case gefunden wird, als true (richtig) bewertet zu werden, wird es die Code in diese case "render" . Alle anderen case werden ignoriert.
th:case = "*" ist die Default case der Struktur th:swith/th:case. Wenn alle case oben als false gewertet werden, wird die Kode von Default case gerendert ("render") werden.
Example:
switch-example.html (Template)
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>th:witch/th:case</title>
</head>
<body>
<h1>tth:witch/th:case</h1>
<h4 th:utext="${user.userName}"></h4>
<div th:switch="${user.role}">
<p th:case="'admin'">User is an administrator</p>
<p th:case="'manager'">User is a manager</p>
<p th:case="'staff'">User is a staff</p>
<p th:case="*">User is some other thing</p>
</div>
</body>
</html>
(Java Spring)
@RequestMapping("/switch-example")
public String ifTestFalse(Model model) {
User user = new User("Administrator", "admin");
model.addAttribute("user", user);
return "switch-example";
}
User.java
package org.o7planning.thymeleaf.model;
public class User {
private String userName;
private String role;
public User(String userName, String role) {
this.userName = userName;
this.role = role;
}
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
public String getRole() {
return role;
}
public void setRole(String role) {
this.role = role;
}
}
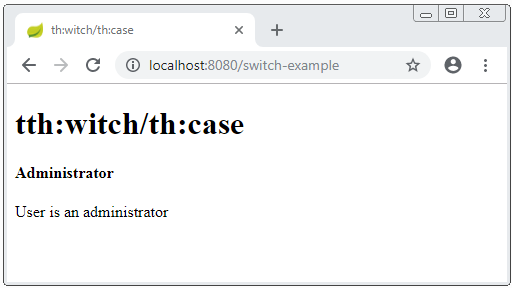
Anleitungen Thymeleaf
- Elvis-Betreiber in Thymeleaf
- Schleifen in Thymeleaf
- Bedingte Anweisungen if, unless, switch in Thymeleaf
- Vordefinierte Objekte in Thymeleaf
- Verwenden von Thymeleaf th:class, th:classappend, th:style, th:styleappend
- Einführung in Thymeleaf
- Variablen in Thymeleaf
- Verwenden Sie Fragment in Thymeleaf
- Verwenden Sie Layout in Thymeleaf
- Verwenden von Thymeleaf th:object und asterisk-syntax *{}
- Thymeleaf Form Select option Beispiel
Show More