Variablen in Thymeleaf
1. Die Variable (Variable)
Das Konzept von Variable in die Sprache Java ist Ihnen nicht fremd. Wie steht es jedoch im Thymeleaf ? Auf jeden Fall hat Thymeleaf das Konzept der Variable.
Ein Attribut des Objekt org.springframework.ui.Model, oder ein Attribut des Objekt HttpServletRequest ist ein Variable von Thymeleaf. Diese Variable kann überall in Template benutzt werden.
(Java Spring)
@RequestMapping("/variable-example1")
public String variableExample1(Model model, HttpServletRequest request) {
// variable1
model.addAttribute("variable1", "Value of variable1!");
// variable2
request.setAttribute("variable2", "Value of variable2!");
return "variable-example1";
}
variable-example1.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Variable</title>
</head>
<body>
<h1>Variables</h1>
<h4>${variable1}</h4>
<span th:utext="${variable1}"></span>
<h4>${variable2}</h4>
<span th:utext="${variable2}"></span>
</body>
</html>
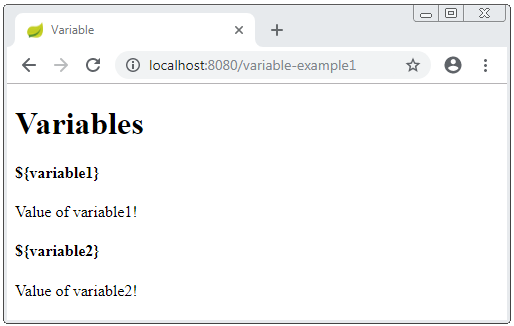
Local Variables
Sie können die Variable in Template definieren. Sie sind die lokalen Variables. Sie existieren und sind vorhanden in einer Sektion von Template.
In diesem Beispiel existieren 2 Variables flower, iter und sind vorhanden in die Schleife, die sie deklarieren.
(Java Spring)
@RequestMapping("/variable-in-loop")
public String objectServletContext(Model model, HttpServletRequest request) {
String[] flowers = new String[] {"Rose","Lily", "Tulip", "Carnation", "Hyacinth" };
model.addAttribute("flowers", flowers);
return "variable-in-loop";
}
variable-in-loop.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Variable</title>
</head>
<body>
<h1>Variable: flower, iter</h1>
<table border="1">
<tr>
<th>No</th>
<th>Flower Name</th>
</tr>
<!--
Local Variable: flower
Local Variable: iter (Iterator).
-->
<tr th:each="flower, iter : ${flowers}">
<td th:utext="${iter.count}">No</td>
<td th:utext="${flower}">Flower Name</td>
</tr>
</table>
</body>
</html>
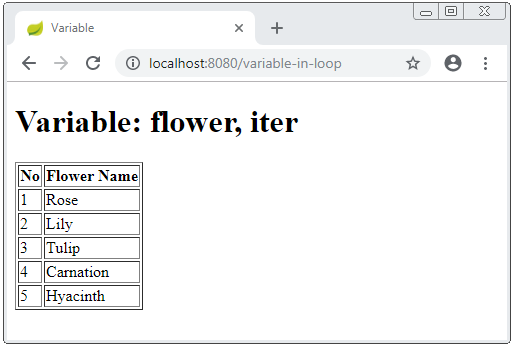
th:with
Sie können eine oder viele lokalen Variables durch das Attribut th:with. Seine Syntax ist ähnlich wie eine normale Wertzuweisung Ausdrück ( "value assignment expression").
(Java Spring)
@RequestMapping("/variable-example3")
public String variableExample3(Model model) {
String[] flowers = new String[] {"Rose","Lily", "Tulip", "Carnation", "Hyacinth" };
model.addAttribute("flowers", flowers);
return "variable-example3";
}
variable-example3.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Variable</title>
</head>
<body>
<h1>th:with</h1>
<!-- Local variable: flower0 -->
<div th:with="flower0 = ${flowers[0]}">
<h4>${flower0}</h4>
<span th:utext="${flower0}"></span>
</div>
<!-- Local variable: flower1, flower2 -->
<div th:with="flower1 = ${flowers[1]}, flower2 = ${flowers[2]}">
<h4>${flower1}, ${flower2}</h4>
<span th:utext="${flower1}"></span>
<br/>
<span th:utext="${flower2}"></span>
</div>
<hr>
<!-- Local variable: firstName, lastName, fullName -->
<div th:with="firstName = 'James', lastName = 'Smith', fullName = ${firstName} +' ' + ${lastName}">
First Name: <span th:utext="${firstName}"></span>
<br>
Last Name: <span th:utext="${lastName}"></span>
<br>
Full Name: <span th:utext="${fullName}"></span>
</div>
</body>
</html>
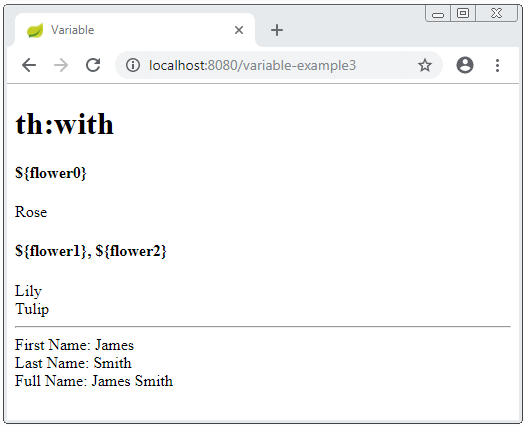
Zum Beispiel: Erstellen Sie ein Array im Thymeleaf:
variable-array-example.html (Template)
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Variable</title>
</head>
<body>
<h1>th:with (Array)</h1>
<!-- Create an Array: -->
<th:block th:with="flowers = ${ {'Rose', 'Lily', 'Tulip'} }">
<table border="1">
<tr>
<th>No</th>
<th>Flower</th>
</tr>
<tr th:each="flower, state : ${flowers}">
<td th:utext="${state.count}">No</td>
<td th:utext="${flower}">Flower</td>
</tr>
</table>
</th:block>
</body>
</html>
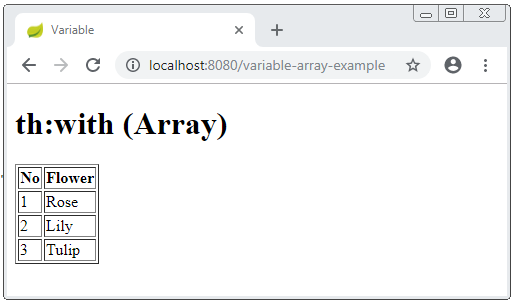
Anleitungen Thymeleaf
- Elvis-Betreiber in Thymeleaf
- Schleifen in Thymeleaf
- Bedingte Anweisungen if, unless, switch in Thymeleaf
- Vordefinierte Objekte in Thymeleaf
- Verwenden von Thymeleaf th:class, th:classappend, th:style, th:styleappend
- Einführung in Thymeleaf
- Variablen in Thymeleaf
- Verwenden Sie Fragment in Thymeleaf
- Verwenden Sie Layout in Thymeleaf
- Verwenden von Thymeleaf th:object und asterisk-syntax *{}
- Thymeleaf Form Select option Beispiel
Show More