Die Anleitung zu Java ByteArrayOutputStream
1. ByteArrayOutputStream
ByteArrayOutputStream ist eine Unterklasse von OutputStream. Wie genau der Name sagt, ByteArrayOutputStream wird verwendet um die bytes in ein Array byte zu schreiben, das es nach der Weise eines OutputStream verwaltet.
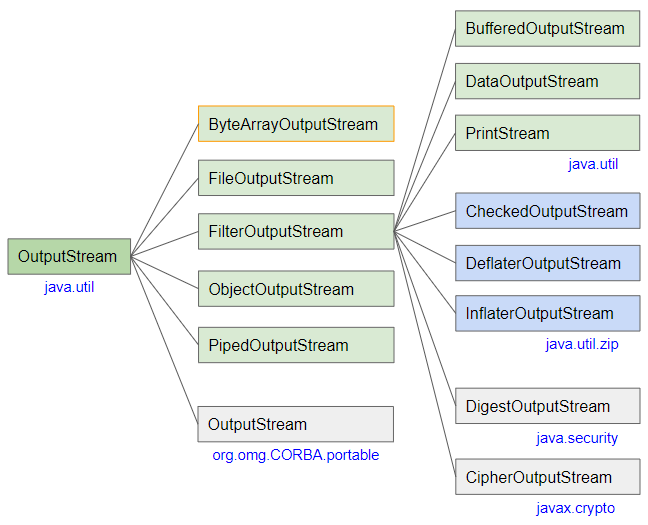
- OutputStream
- BufferedOutputStream
- ObjectOutputStream
- PrintStream
- FileOutputStream
- FilterOutputStream
- PipedOutputStream
- DataOutputStream
- InflaterOutputStream
- DigestOutputStream
- DeflaterOutputStream
- CipherOutputStream
- CheckedOutputStream
Die in ByteArrayOutputStream geschriebene bytes werden Elemente des Arraysbyte zugewiesen, das ByteArrayOutputStream verwaltet.
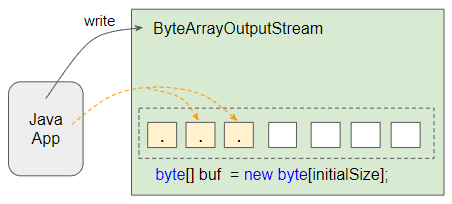
Wenn die Anzahl der in ByteArrayOutputStream geschriebenen bytes größer als die Länge des Array ist, erstellt ByteArrayOutputStream ein neues Array mit größerer Länge und kopiert die bytes aus dem alten Array.
2. Constructors
Constructors
public ByteArrayOutputStream()
public ByteArrayOutputStream(int initialSize)
- Der Konstructor ByteArrayOutputStream(int) erstellt ein Objekt ByteArrayOutputStream mit dem Array byte , das eine bestimmte Anfangsgröße hat.
- Der Konstructor ByteArrayOutputStream() erstellt ein Objekt ByteArrayOutputStream mit dem Array byte , das die Defaultgröße hat (32).
3. Methods
Alle Methode von ByteArrayOutputStream werden aus ihre Vaterklasse - OutputStream geerbt.
void close()
void reset()
int size()
byte[] toByteArray()
String toString()
String toString(String charsetName)
String toString(Charset charset)
void write(byte[] b, int off, int len)
void write(int b)
void writeBytes(byte[] b)
void writeTo(OutputStream out)
Schauen Sie den Artikel über OutputStream an um mehrere Beispiele über diese Methoden zu erhalten.
4. Examples
ByteArrayOutputStreamEx1.java
package org.o7planning.bytearrayoutputstream.ex;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
public class ByteArrayOutputStreamEx1 {
public static void main(String[] args) throws IOException {
ByteArrayOutputStream baos = new ByteArrayOutputStream(1024);
byte[] bytes1 = new byte[] {'H', 'e'};
baos.write(bytes1);
baos.write(108); // 'l'
baos.write('l'); // code: 108
baos.write('o'); // code: 111
byte[] buffer = baos.toByteArray();
for(byte b: buffer) {
System.out.println(b + " --> " + (char)b);
}
}
}
Output:
72 --> H
101 --> e
108 --> l
108 --> l
111 --> o
Z.B: Fügen Sie beispielweise 2 Arrays byte hinzu, um ein neues Array zu erstellen.
ByteArrayOutputStreamEx3.java
package org.o7planning.bytearrayoutputstream.ex;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
public class ByteArrayOutputStreamEx3 {
public static void main(String[] args) throws IOException {
byte[] arr1 = "Hello ".getBytes();
byte[] arr2 = new byte[] {'W', 'o', 'r', 'l', 'd', '!'};
byte[] result = add(arr1, arr2);
for(byte b: result) {
System.out.println(b + " " + (char)b);
}
}
public static byte[] add(byte[] arr1, byte[] arr2) {
if (arr1 == null) {
return arr2;
}
if (arr2 == null) {
return arr1;
}
ByteArrayOutputStream baos = new ByteArrayOutputStream();
try {
baos.write(arr1);
baos.write(arr2);
} catch (Exception e) {
// Never happened!
}
return baos.toByteArray();
}
}
Output:
72 H
101 e
108 l
108 l
111 o
32
87 W
111 o
114 r
108 l
100 d
33 !
Grundsätzlich erben die meisten Methode von ByteArrayOutputStream von OutputStream. Deshalb können Sie mehrere Beispiele zur Verwendung dieser Methode im folgenden Artikel finden.
Die Anleitungen Java IO
- Die Anleitung zu Java CharArrayWriter
- Die Anleitung zu Java FilterReader
- Die Anleitung zu Java FilterWriter
- Die Anleitung zu Java PrintStream
- Die Anleitung zu Java BufferedReader
- Die Anleitung zu Java BufferedWriter
- Die Anleitung zu Java StringReader
- Die Anleitung zu Java StringWriter
- Die Anleitung zu Java PipedReader
- Die Anleitung zu Java LineNumberReader
- Die Anleitung zu Java PushbackReader
- Die Anleitung zu Java PrintWriter
- Die Anleitung zu Java IO Binary Streams
- Die Anleitung zu Java IO Character Streams
- Die Anleitung zu Java BufferedOutputStream
- Die Anleitung zu Java ByteArrayOutputStream
- Die Anleitung zu Java DataOutputStream
- Die Anleitung zu Java PipedInputStream
- Die Anleitung zu Java OutputStream
- Die Anleitung zu Java ObjectOutputStream
- Die Anleitung zu Java PushbackInputStream
- Die Anleitung zu Java SequenceInputStream
- Die Anleitung zu Java BufferedInputStream
- Die Anleitung zu Java Reader
- Die Anleitung zu Java Writer
- Die Anleitung zu Java FileReader
- Die Anleitung zu Java FileWriter
- Die Anleitung zu Java CharArrayReader
- Die Anleitung zu Java ByteArrayInputStream
- Die Anleitung zu Java DataInputStream
- Die Anleitung zu Java ObjectInputStream
- Die Anleitung zu Java InputStreamReader
- Die Anleitung zu Java OutputStreamWriter
- Die Anleitung zu Java InputStream
- Die Anleitung zu Java FileInputStream
Show More
- Anleitungen Java Servlet/JSP
- Die Anleitungen Java New IO
- Anleitungen Spring Cloud
- Die Anleitungen Oracle Java ADF
- Die Anleitungen Java Collections Framework
- Java Grundlagen
- Die Anleitungen Java Date Time
- Java Open Source Bibliotheken
- Anleitungen Java Web Services
- Die Anleitungen Struts2 Framework
- Anleitungen Spring Boot