Die Anleitung zu Java DataInputStream
1. DataInputStream
DataInputStream wird verwendet, um die primitiven Daten aus einer Datenquelle zu lesen, insbesondere Datenquellen, die von DataOutputStream geschrieben wurden.
public class DataInputStream extends FilterInputStream implements DataInput
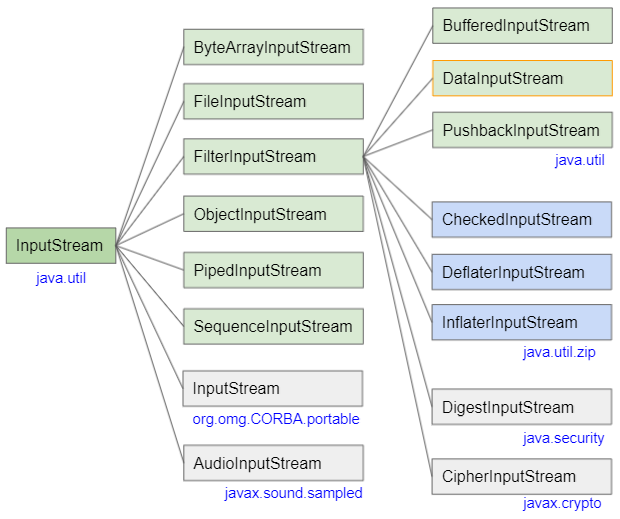
- ByteArrayInputStream
- FileInputStream
- FilterInputStream
- BufferedInputStream
- InputStream
- PushbackInputStream
- ObjectInputStream
- PipedInputStream
- SequenceInputStream
- AudioInputStream
- CheckedInputStream
- DeflaterInputStream
- InflaterInputStream
- CipherInputStream
- DigestInputStream
3. Methods
public final boolean readBoolean() throws IOException
public final byte readByte() throws IOException
public final int readUnsignedByte() throws IOException
public final short readShort() throws IOException
public final int readUnsignedShort() throws IOException
public final char readChar() throws IOException
public final int readInt() throws IOException
public final long readLong() throws IOException
public final float readFloat() throws IOException
public final double readDouble() throws IOException
public final String readUTF() throws IOException
public static final String readUTF(DataInput in) throws IOException
public final void readFully(byte[] b) throws IOException
public final void readFully(byte[] b, int off, int len) throws IOException
@Deprecated
public String readLine() throws IOException
Andere Methoden, die von Elternklassen geerbt wurden:
public int read() throws IOException
public int read(byte[] b) throws IOException
public int read(byte[] b, int off, int len) throws IOException
public byte[] readAllBytes() throws IOException
public byte[] readNBytes(int len) throws IOException
public int readNBytes(byte[] b, int off, int len) throws IOException
public long skip(long n) throws IOException
public int available() throws IOException
public void close() throws IOException
public synchronized void mark(int readlimit)
public synchronized void reset() throws IOException
public boolean markSupported()
public long transferTo(OutputStream out) throws IOException
public static InputStream nullInputStream()
4. Examples
DataInputStream wird häufig verwendet, um von DataOutputStream geschriebene Datenquellen zu lesen. In diesem Beispiel verwenden wir DataOutputStream, um eine Datentabelle mit einer Excel-ähnlichen Struktur in eine Datei zu schreiben, und verwenden dann DataInputStream, um diese Datei zu lesen.
OrderDate | Finished | Item | Units | UnitCost | Total |
2020-01-06 | Pencil | 95 | 1.99 | 189.05 | |
2020-01-23 | Binder | 50 | 19.99 | 999.50 | |
2020-02-09 | Pencil | 36 | 4.99 | 179.64 | |
2020-02-26 | Pen | 27 | 19.99 | 539.73 | |
2020-03-15 | Pencil | 56 | 2.99 | 167.44 |
Zuerst schreiben wir die Klasse Order, die die Daten einer Tabellenzeile simuliert:
Order.java
package org.o7planning.datainputstream.ex;
import java.time.LocalDate;
public class Order {
private LocalDate orderDate;
private boolean finished;
private String item;
private int units;
private float unitCost;
private float total;
public Order(LocalDate orderDate, boolean finished, //
String item, int units, float unitCost, float total) {
this.orderDate = orderDate;
this.finished = finished;
this.item = item;
this.units = units;
this.unitCost = unitCost;
this.total = total;
}
public LocalDate getOrderDate() {
return orderDate;
}
public boolean isFinished() {
return finished;
}
public String getItem() {
return item;
}
public int getUnits() {
return units;
}
public float getUnitCost() {
return unitCost;
}
public float getTotal() {
return total;
}
}
Verwenden Sie DataOutputStream, um die obige Datentabelle in eine Datei zu schreiben:
WriteDataFile_example1.java
package org.o7planning.datainputstream.ex;
import java.io.DataOutputStream;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
import java.time.LocalDate;
public class WriteDataFile_example1 {
// Windows: C:/somepath/data-file.txt
private static final String filePath = "/Volumes/Data/test/data-file.txt";
public static void main(String[] args) throws IOException {
Order[] orders = new Order[] { //
new Order(LocalDate.of(2020, 1, 6), true, "Pencil", 95, 1.99f, 189.05f),
new Order(LocalDate.of(2020, 1, 23), false, "Binder", 50, 19.99f, 999.50f),
new Order(LocalDate.of(2020, 2, 9), true, "Pencil", 36, 4.99f, 179.64f),
new Order(LocalDate.of(2020, 2, 26), false, "Pen", 27, 19.99f, 539.73f),
new Order(LocalDate.of(2020, 3, 15), true, "Pencil", 56, 2.99f, 167.44f) //
};
File outFile = new File(filePath);
outFile.getParentFile().mkdirs();
OutputStream outputStream = new FileOutputStream(outFile);
DataOutputStream dataOutputStream = new DataOutputStream(outputStream);
for (Order order : orders) {
dataOutputStream.writeUTF(order.getOrderDate().toString());
dataOutputStream.writeBoolean(order.isFinished());
dataOutputStream.writeUTF(order.getItem());
dataOutputStream.writeInt(order.getUnits());
dataOutputStream.writeFloat(order.getUnitCost());
dataOutputStream.writeFloat(order.getTotal());
}
dataOutputStream.close();
}
}
Nachdem wir das obige Beispiel ausgeführt haben, haben wir eine Datendatei mit ziemlich verwirrendem Inhalt:
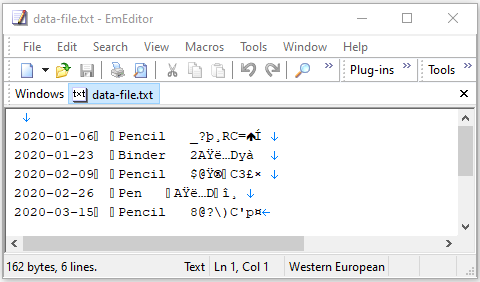
Als nächstes verwenden wir DataInputStream, um die obige Datei zu lesen.
ReadDataFile_example1.java
package org.o7planning.datainputstream.ex;
import java.io.DataInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
public class ReadDataFile_example1 {
// Windows: C:/somepath/data-file.txt
private static final String filePath = "/Volumes/Data/test/data-file.txt";
public static void main(String[] args) throws IOException {
File file = new File(filePath);
InputStream inputStream = new FileInputStream(file);
DataInputStream dataInputStream = new DataInputStream(inputStream);
int row = 0;
System.out.printf("|%3s | %-10s | %10s | %-15s | %8s| %10s | %10s |%n", //
"No", "Order Date", "Finished?", "Item", "Units", "Unit Cost", "Total");
System.out.printf("|%3s | %-10s | %10s | %-15s | %8s| %10s | %10s |%n", //
"--", "---------", "----------", "----------", "------", "---------", "---------");
while (dataInputStream.available() > 0) {
row++;
String orderDate = dataInputStream.readUTF();
boolean finished = dataInputStream.readBoolean();
String item = dataInputStream.readUTF();
int units = dataInputStream.readInt();
float unitCost = dataInputStream.readFloat();
float total = dataInputStream.readFloat();
System.out.printf("|%3d | %-10s | %10b | %-15s | %8d| %,10.2f | %,10.2f |%n", //
row, orderDate, finished, item, units, unitCost, total);
}
dataInputStream.close();
}
}
Output:
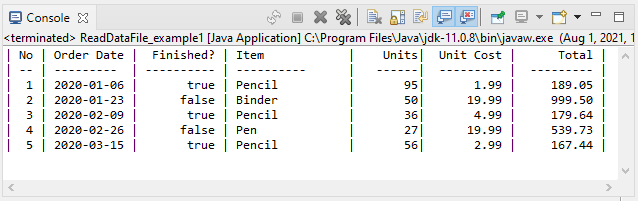
5. readUTF()
Die Methode readUTF() wird verwendet, um einen mit "Modified UTF-8" codierten String zu lesen (UTF-8 wurde geändert). Die Leseregeln sind symmetrisch zu den Schreibregeln der Methode DataOutput.writeUTF(String).
public final String readUTF() throws IOException
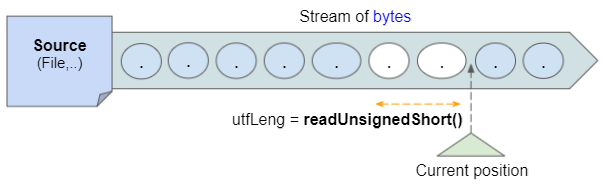
An der Cursorposition im Strom von bytes (stream of bytes)liest der DataInputStream die nächsten 2 bytes mit der Methode readUnsignedShort(), um die Anzahl der bytes zu bestimmen, die zum Speichern des Strings UTF-8 verwendet werden, und erhält das Ergebnis utfLeng.
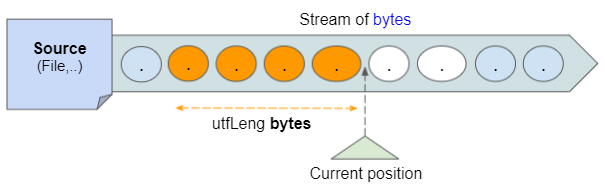
Dann liest der DataInputStream die nächsten utfLengbytes und wandelt sie in einen String UTF-8 um.
- UTF-8
- DataOutputStream
6. readUTF(DataInput in)
Die statische Methode readUTF(DataInput) wird verwendet, um einen String "Modified UTF-8" aus einem angegebenen Objekt DataInput zu lesen.
public static final String readUTF(DataInput in) throws IOException
7. readFully(byte[] buffer)
Liest so viele bytes wie möglich aus diesem DataInputStream und weist ihn dem angegebenen Array buffer zu.
public final void readFully(byte[] buffer) throws IOException
Diese Methode blockiert, bis eine der folgenden Bedingungen eintritt:
- buffer.lengthbytes lesen.
- Das Ende des Streams ist erreicht.
- Ein IOException passiert (anders als EOFException).
8. readFully(byte[] buffer, int off, int len)
Liest so viele bytes wie möglich aus diesem DataInputStream und weist ihn dem angegebenen Array buffer von index off bis index off+len-1 zu.
public final void readFully(byte[] buffer, int off, int len) throws IOException
Diese Methode blockiert, bis eine der folgenden Bedingungen eintritt:
- lenbytes lesen.
- Das Ende von Stream ist erreicht.
- Eine IOException auftritt (unterschiedlich von EOFException).
9. readBoolean()
Die Methode readBoolean() liest ein byte aus diesem DataInputStream. Wenn das Ergebnis nicht 0 ist, gibt die Methode true zurück, andernfalls gibt sie false zurück.
public final boolean readBoolean() throws IOException
10. readByte()
Die Methode readByte() liest ein byte aus diesem DataInputStream, der zurückgegebene Wert liegt zwischen -128 und 127.
Es eignet sich zum Lesen von Daten, die mit der Methode DataOutput.writeByte(byte) geschrieben wurden.
public final byte readByte() throws IOException
11. readUnsignedByte()
Die Methode readUnsignedShort() liest 1 byte aus diesem DataInputStream und wandelt es in eine vorzeichenlose 4-bytes-Integer mit Werten von 0 bis 255 um.
public final int readUnsignedByte() throws IOException
12. readShort()
Die Methode readShort() liest 2 bytes aus diesem DataInputStream und wandelt ihn in einen Datentyp short um.
public final short readShort() throws IOException
Der Umrechnungsregel:
return (short)((firstByte << 8) | (secondByte & 0xff));
13. readUnsignedShort()
Die Methode readUnsignedShort() liest 2 bytes aus diesem DataInputStream und wandelt sie in eine vorzeichenlose 4-bytes-Integer mit Werten von 0 bis 65535 um.
public final int readUnsignedShort() throws IOException
Der Umrechnungsregel:
return (((firstByte & 0xff) << 8) | (secondByte & 0xff))
14. readChar()
Die Methode eadChar() liest 2 bytes aus diesem DataInputStream und konvertiert ihn in den Datentyp char.
public final char readChar() throws IOException
Der Umrechnungsregel:
return (char)((firstByte << 8) | (secondByte & 0xff));
15. readInt()
Die Methode readInt() liest 4 bytes aus diesem DataInputStream und konvertiert ihn in den Datentyp int.
public final int readInt() throws IOException
Der Umrechnungsregel:
return (((firstByte & 0xff) << 24) | ((secondByte & 0xff) << 16)
| ((thirdByte & 0xff) << 8) | (fourthByte & 0xff));
16. readLong()
Die Methode readLong() liest 8 bytes aus diesem DataInputStream und wandelt ihn in einen Datentyp long um.
public final long readLong() throws IOException
Der Umrechnungsregel:
return (((long)(byte1 & 0xff) << 56) |
((long)(byte2 & 0xff) << 48) |
((long)(byte3 & 0xff) << 40) |
((long)(byte4 & 0xff) << 32) |
((long)(byte5 & 0xff) << 24) |
((long)(byte6 & 0xff) << 16) |
((long)(byte7 & 0xff) << 8) |
((long)(byte8 & 0xff)));
Die Anleitungen Java IO
- Die Anleitung zu Java CharArrayWriter
- Die Anleitung zu Java FilterReader
- Die Anleitung zu Java FilterWriter
- Die Anleitung zu Java PrintStream
- Die Anleitung zu Java BufferedReader
- Die Anleitung zu Java BufferedWriter
- Die Anleitung zu Java StringReader
- Die Anleitung zu Java StringWriter
- Die Anleitung zu Java PipedReader
- Die Anleitung zu Java LineNumberReader
- Die Anleitung zu Java PushbackReader
- Die Anleitung zu Java PrintWriter
- Die Anleitung zu Java IO Binary Streams
- Die Anleitung zu Java IO Character Streams
- Die Anleitung zu Java BufferedOutputStream
- Die Anleitung zu Java ByteArrayOutputStream
- Die Anleitung zu Java DataOutputStream
- Die Anleitung zu Java PipedInputStream
- Die Anleitung zu Java OutputStream
- Die Anleitung zu Java ObjectOutputStream
- Die Anleitung zu Java PushbackInputStream
- Die Anleitung zu Java SequenceInputStream
- Die Anleitung zu Java BufferedInputStream
- Die Anleitung zu Java Reader
- Die Anleitung zu Java Writer
- Die Anleitung zu Java FileReader
- Die Anleitung zu Java FileWriter
- Die Anleitung zu Java CharArrayReader
- Die Anleitung zu Java ByteArrayInputStream
- Die Anleitung zu Java DataInputStream
- Die Anleitung zu Java ObjectInputStream
- Die Anleitung zu Java InputStreamReader
- Die Anleitung zu Java OutputStreamWriter
- Die Anleitung zu Java InputStream
- Die Anleitung zu Java FileInputStream
Show More
- Anleitungen Java Servlet/JSP
- Die Anleitungen Java New IO
- Anleitungen Spring Cloud
- Die Anleitungen Oracle Java ADF
- Die Anleitungen Java Collections Framework
- Java Grundlagen
- Die Anleitungen Java Date Time
- Java Open Source Bibliotheken
- Anleitungen Java Web Services
- Die Anleitungen Struts2 Framework
- Anleitungen Spring Boot