Die Anleitung zu Java InputStream
1. InputStream
InputStream ist eine Klasse im Paket java.io. Es ist eine Basisklasse zur Darstellung eines Strom von bytes (stream of bytes), der beim Lesen einer bestimmten Datenquelle, z.B einer Datei, abgerufen wird.
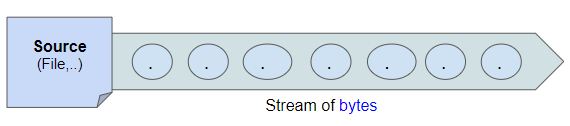
public abstract class InputStream implements Closeable
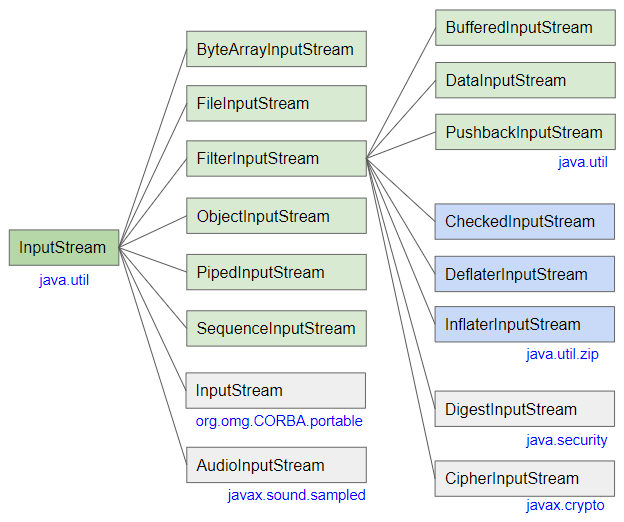
- ByteArrayInputStream
- FileInputStream
- FilterInputStream
- BufferedInputStream
- DataInputStream
- PushbackInputStream
- ObjectInputStream
- PipedInputStream
- SequenceInputStream
- AudioInputStream
- CheckedInputStream
- DeflaterInputStream
- InflaterInputStream
- CipherInputStream
- DigestInputStream
Grundsätzlich können Sie die Klasse InputStream nicht direkt verwenden, da es sich um eine abstrakte Klasse handelt. In einem bestimmten Fall können Sie jedoch eine ihrer Unterklasse verwenden.
Sehen wir uns ein Beispiel für eine UTF-8 codierte Textdatei an:
utf8-file-without-bom.txt
JP日本-八洲
UTF-8 verwendet 1, 2, 3 oder 4 bytes zum Speichern eines Zeichens. Das Bild unten zeigt die bytes in der oben genannten Datei.
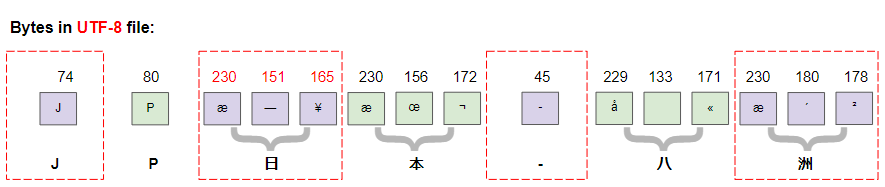
FileInputStream ist eine Unterklasse von InputStream, die üblicherweise zum Lesen von Dateien verwendet wird und wir erhalten einen Strom von bytes (stream of bytes).
InputStream Methods
public static InputStream nullInputStream()
public abstract int read() throws IOException
public int read(byte[] b) throws IOException
public int read(byte[] b, int off, int len) throws IOException
public byte[] readAllBytes() throws IOException
public byte[] readNBytes(int len) throws IOException
public int readNBytes(byte[] b, int off, int len) throws IOException
public long skip(long n) throws IOException
public int available() throws IOException
public void close() throws IOException
public synchronized void mark(int readlimit)
public synchronized void reset() throws IOException
public boolean markSupported()
public long transferTo(OutputStream out) throws IOException
2. read()
public int read() throws IOException
Die Methode read() wird zum Lesen eines byte verwendet. Der Wert des zurückgegebenen byte ist ein Integer zwischen 0 und 255, oder gibt -1 zurück, wenn es das Ende des Streams erreicht hat.
Diese Methode wird blockiert, bis das byte zum Lesen verfügbar ist oder ein Fehler IO auftritt oder das Ende des Streams erreicht hat.
utf8-file-without-bom.txt
JP日本-八洲
Zum Beispiel:
InputStream_read_ex1.java
package org.o7planning.inputstream.ex;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
public class InputStream_read_ex1 {
// Windows path: C:/somepath/utf8-file-without-bom.txt"
private static final String filePath = "/Volumes/Data/test/utf8-file-without-bom.txt";
public static void main(String[] args) throws IOException {
// FileInputStream is a subclass of InputStream.
InputStream is = new FileInputStream(filePath);
int code;
while((code = is.read()) != -1) {
System.out.println(code + " " + (char)code);
}
is.close();
}
}
Output:
74 J
80 P
230 æ
151
165 ¥
230 æ
156
172 ¬
45 -
229 å
133
171 «
230 æ
180 ´
178 ²
3. read(byte[])
public int read(byte[] b) throws IOException
Die Methode read(byte[]) liest bytes aus InputStream und weist den Elementen des Arrays zu und gibt die Anzahl der gelesenen bytes zurück. Diese Methode gibt -1 zurück wenn das Ende des Streams erreicht ist.
Diese Methode wird blockiert, bis die bytes zum Lesen verfügbar sind oder ein Fehler IO auftritt oder das Ende des Streams erreicht hat.
Grundsätzlich hat die Verwendung der Methode read(byte[]) die erhöhere Leistung als die Methode read(), da dadurch weniger häufig aus dem Stream gelesen werden muss.
InputStream_read_ex2.java
package org.o7planning.inputstream.ex;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
public class InputStream_read_ex2 {
public static void main(String[] args) throws IOException {
String url = "https://s3.o7planning.com/txt/utf8-file-without-bom.txt";
InputStream is = new URL(url).openStream();
// Create a temporary byte array.
byte[] tempByteArray = new byte[10];
int byteCount = -1;
int nth = 0;
while ((byteCount = is.read(tempByteArray)) != -1) {
nth++;
System.out.println("--- Read th: " + nth + " ---");
System.out.println(" >> Number of bytes read: " + byteCount +"\n");
for(int i= 0; i < byteCount; i++) {
// bytes are in range [-128,127]
// Convert byte to unsigned byte. [0, 255].
int code = tempByteArray[i] & 0xff;
System.out.println(tempByteArray[i] + " " + code + " " + (char)code);
}
}
is.close();
}
}
Output:
--- Read th: 1 ---
>> Number of bytes read: 10
74 74 J
80 80 P
-26 230 æ
-105 151
-91 165 ¥
-26 230 æ
-100 156
-84 172 ¬
45 45 -
-27 229 å
--- Read th: 2 ---
>> Number of bytes read: 5
-123 133
-85 171 «
-26 230 æ
-76 180 ´
-78 178 ²
Hinweis: Der Datentyp byte besteht aus den Integer im Bereich von -128 bis 127. Sie können ihn in den Integer ohne Vorzeichen (unsigned integer) im Bereich von 0 bis 255 konvertieren.
- Convert byte to unsigned byte
4. read(byte[], int, int)
public int read(byte[] b, int offset, int len) throws IOException
Die Methode read(byte[],int,int) liest bytes und weist Elementen des Arrays von Index offset zu Index offset+len zu und gibt die Anzahl der gelesenen bytes zurück. Diese Methode gibt -1 zurück wenn das Ende des Streams erreicht ist.
Diese Methode wird blockiert, bis die bytes zum Lesen verfügbar sind oder ein Fehler IO auftritt oder das Ende des Streams erreicht hat.
5. readAllBytes()
public byte[] readAllBytes() throws IOException
Z.B
InputStream_readAllBytes_ex1.java
package org.o7planning.inputstream.ex;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
public class InputStream_readAllBytes_ex1 {
public static void main(String[] args) throws IOException {
String url = "https://s3.o7planning.com/txt/utf8-file-without-bom.txt";
InputStream is = new URL(url).openStream();
byte[] allBytes = is.readAllBytes();
String content = new String(allBytes, "UTF-8");
System.out.println(content);
is.close();
}
}
Output:
JP日本-八洲
6. readNBytes(int len)
public byte[] readNBytes(int len) throws IOException
Die Methode readNBytes(int) liest bis zu "len"bytes aus InputStream, und gibt ein gelesenes Array byte zurück. Wenn das zurückgegebene Array leer ist, befindet sich bereits am Ende des Streams.
Diese Methode wird blockiert, bis "len"bytes gelesen wurde oder ein Fehler IO auftritt oder das Ende des Stream erreicht ist.
Zum Beispiel:
InputStream_readNBytes_ex1.java
package org.o7planning.inputstream.ex;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
public class InputStream_readNBytes_ex1 {
// Windows path: C:/somepath/utf8-file-without-bom.txt"
private static final String filePath = "/Volumes/Data/test/utf8-file-without-bom.txt";
public static void main(String[] args) throws IOException {
// FileInputStream is a subclass of InputStream.
InputStream is = new FileInputStream(filePath);
byte[] bytes = null;
int nth = 0;
while( true) {
nth++;
bytes = is.readNBytes(10);
System.out.println("--- Read th: " + nth + " ---");
System.out.println(" >> Number of bytes read: " + bytes.length +"\n");
if(bytes.length == 0) {
break;
}
for(int i= 0; i< bytes.length; i++) {
// bytes are in range [-128,127]
// Convert byte to unsigned byte. [0, 255].
int code = bytes[i] & 0xff;
System.out.println(bytes[i] + " " + code + " " + (char)code);
}
}
is.close();
}
}
Output:
--- Read th: 1 ---
>> Number of bytes read: 10
74 74 J
80 80 P
-26 230 æ
-105 151
-91 165 ¥
-26 230 æ
-100 156
-84 172 ¬
45 45 -
-27 229 å
--- Read th: 2 ---
>> Number of bytes read: 5
-123 133
-85 171 «
-26 230 æ
-76 180 ´
-78 178 ²
--- Read th: 3 ---
>> Number of bytes read: 0
7. readNBytes(byte[] b, int off, int len)
public int readNBytes(byte[] b, int offset, int len) throws IOException
Die Methode readNBytes(byte[],int,int) liest bis "len"bytes aus InputStream, und weißt den Elemente von Arrays von Index offset bis zu Index offset+len die gelesenen Bytes zu und gibt die Anzahl der gelesenen bytes zurück. Gibt -1 zurück wenn das Ende des Streams erreicht ist.
read(byte[],int,int) vs readNBytes(byte[],int,int)
public int read(byte[] b, int offset, int len) throws IOException
public int readNBytes(byte[] b, int offset, int len) throws IOException
Die beiden Methoden read(byte[],int,int) und readNBytes(byte[],int,int) sind ziemlich gleich. In der folgenden Situation gibt es jedoch einen Unterschied:
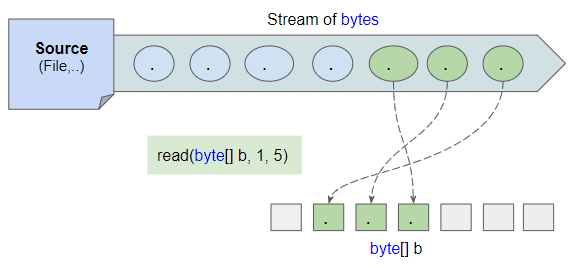
Die Methode read(byte[] b,int offset, int len) garantiert nicht, dass "len" bytes aus dem Stream gelesen werden, obwohl sie das Ende des Streams noch nicht erreicht haben.
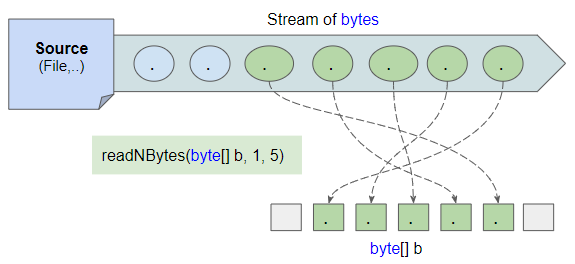
Die Methode readNBytes(byte[] b,int offset, int len) garantiert, dass "len" bytes aus dem Stream gelesen werden, wenn sie das Ende des Streams noch nicht erreicht haben.
8. close()
public void close() throws IOException
Schließt den Stream und gibt alle damit verbundenen Systemressourcen frei. Sobald der Stream geschlossen wurde, wird durch die Aufrüfen von read(), mark(), reset() oder skip() eine IOException ausgelöst. Das Schließen eines vorher geschlossenen Stream hat keine Auswirkung.
public interface Closeable extends AutoCloseable
Die Klasse InputStream implementiert die Interface Closeable. Wenn Sie die Code nach den Regel von AutoCloseable schreiben, schließt das System den Stream automatisch für Sie, ohne die Methode close() direkt aufrufen zu müssen.
InputStream_close_ex1.java
package org.o7planning.inputstream.ex;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
public class InputStream_close_ex1 {
// Or Windows path: C:/Somefolder/utf8-file-without-bom.txt
private static final String file_path = "/Volumes/Data/test/utf8-file-without-bom.txt";
public static void main(String[] args) throws IOException {
// (InputStream class implements Closeable)
// (Closeable interface extends AutoCloseable)
// try block will automatically close stream for you.
try (InputStream fileInputStream= new FileInputStream(file_path)) {
int code;
while((code = fileInputStream.read()) != -1) {
System.out.println(code +" " + (char)code);
}
} // end try
}
}
- Die Anleitung zu Java Closeable
9. skip(long)
public long skip(long n) throws IOException
Die Methode skip(long) ignoriert "n"bytes.
Diese Methode wird blockiert, bis bytes verfügbar sind oder ein Fehler IO auftritt oder das Ende des Streams erreicht ist.
InputStream_skip_ex1.java
package org.o7planning.inputstream.ex;
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.InputStream;
public class InputStream_skip_ex1 {
public static void main(String[] args) throws IOException {
String s = "123456789-987654321-ABCDE";
byte[] bytes = s.getBytes();
// ByteArrayInputStream is a subclass of InputStream.
InputStream is = new ByteArrayInputStream(bytes);
int firstByteCode = is.read();
int secondByteCode = is.read();
System.out.println("First byte: " + (char) firstByteCode);
System.out.println("Second byte: " + (char) secondByteCode);
is.skip(18); // Skips 18 bytes.
int code;
while ((code = is.read()) != -1) {
System.out.println(code +" " + (char) code);
}
is.close();
}
}
Output:
First byte: 1
Second byte: 2
65 A
66 B
67 C
68 D
69 E
10. transferTo(OutputStream)
// Java 10+
public long transferTo(OutputStream out) throws IOException
Mit der Methode transferTo(OutputStream) werden alle bytes aus dem aktuellen InputStream gelesen, in das angegebene Objekt OutputStream geschrieben und die Anzahl der bytes, die an OutputStream übertragen werden. Nach der Erledigung befindet sich das aktuelle Objekt InputStream am Ende des Stream. Diese Methode schließt das aktuelle Objekt InputStream nicht, sowie das Objekt OutputStream.
InputStream_transferTo_ex1.java
package org.o7planning.inputstream.ex;
import java.io.ByteArrayInputStream;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
public class InputStream_transferTo_ex1 {
public static void main(String[] args) throws IOException {
String s = "123456789-987654321-ABCDE";
byte[] bytes = s.getBytes();
// ByteArrayInputStream is a subclass of InputStream.
InputStream reader = new ByteArrayInputStream(bytes);
// Or Windows path: C:/Somepath/out-file.txt
File file = new File("/Volumes/Data/test/out-file.txt");
// Create parent folder.
file.getParentFile().mkdirs();
OutputStream writer = new FileOutputStream(file);
reader.skip(10); // Skips 10 bytes.
reader.transferTo(writer);
reader.close();
writer.close();
}
}
Output:
out-file.txt
987654321-ABCDE
- OutputStream
- FileOutputStream
11. markSupported()
public boolean markSupported()
Mit der Methode markSupported() wird überprüft, ob das aktuelle Objekt InputStream die Operation mark(int) unterstützt oder nicht. (Sehen Sie mehr die Methode mark(int))
12. mark(int)
public void mark(int readAheadLimit) throws IOException
Mit der Methode mark (int) können Sie die aktuelle Position im Stream markieren. Sie können die nächsten bytes lesen und die Methode reset() aufrufen, um zu der zuvor markierten Position zurückzukehren. Dabei ist readAheadLimit die maximale Anzahl von bytes, die nach dem Markieren gelesen werden können, ohne die markierte Position zu verlieren.
Hinweis: Nicht alle InputStreams unterstützen die Operation mark(int). Um sicherzugehen, dass Sie die Methode markSupported() aufrufen müssen, um zu überprüfen, ob das aktuelle Objekt InputStream diesen Vorgang unterstützt.
InputStream_mark_ex1.java
package org.o7planning.inputstream.ex;
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.InputStream;
public class InputStream_mark_ex1 {
public static void main(String[] args) throws IOException {
String s = "123456789-987654321-ABCDE";
byte[] bytes = s.getBytes(); // byte[]{'1','2', .... 'E'}
// ByteArrayInputStream is a subclass of InputStream.
InputStream is = new ByteArrayInputStream(bytes);
is.skip(10); // Skips 10 bytes.
System.out.println("ByteArrayInputStream markSupported? " + is.markSupported()); // true
is.mark(9);
int code1 = is.read();
int code2 = is.read();
System.out.println(code1 + " " + (char) code1); // '9'
System.out.println(code2 + " " + (char) code2); // '8'
is.skip(5);
System.out.println("Reset");
is.reset(); // Return to the marked position.
int code;
while((code = is.read())!= -1) {
System.out.println(code + " " + (char)code);
}
is.close();
}
}
Output:
ByteArrayInputStream markSupported? true
57 9
56 8
Reset
57 9
56 8
55 7
54 6
53 5
52 4
51 3
50 2
49 1
45 -
65 A
66 B
67 C
68 D
69 E
No ADS
Die Anleitungen Java IO
- Die Anleitung zu Java CharArrayWriter
- Die Anleitung zu Java FilterReader
- Die Anleitung zu Java FilterWriter
- Die Anleitung zu Java PrintStream
- Die Anleitung zu Java BufferedReader
- Die Anleitung zu Java BufferedWriter
- Die Anleitung zu Java StringReader
- Die Anleitung zu Java StringWriter
- Die Anleitung zu Java PipedReader
- Die Anleitung zu Java LineNumberReader
- Die Anleitung zu Java PushbackReader
- Die Anleitung zu Java PrintWriter
- Die Anleitung zu Java IO Binary Streams
- Die Anleitung zu Java IO Character Streams
- Die Anleitung zu Java BufferedOutputStream
- Die Anleitung zu Java ByteArrayOutputStream
- Die Anleitung zu Java DataOutputStream
- Die Anleitung zu Java PipedInputStream
- Die Anleitung zu Java OutputStream
- Die Anleitung zu Java ObjectOutputStream
- Die Anleitung zu Java PushbackInputStream
- Die Anleitung zu Java SequenceInputStream
- Die Anleitung zu Java BufferedInputStream
- Die Anleitung zu Java Reader
- Die Anleitung zu Java Writer
- Die Anleitung zu Java FileReader
- Die Anleitung zu Java FileWriter
- Die Anleitung zu Java CharArrayReader
- Die Anleitung zu Java ByteArrayInputStream
- Die Anleitung zu Java DataInputStream
- Die Anleitung zu Java ObjectInputStream
- Die Anleitung zu Java InputStreamReader
- Die Anleitung zu Java OutputStreamWriter
- Die Anleitung zu Java InputStream
- Die Anleitung zu Java FileInputStream
Show More
- Anleitungen Java Servlet/JSP
- Die Anleitungen Java New IO
- Anleitungen Spring Cloud
- Die Anleitungen Oracle Java ADF
- Die Anleitungen Java Collections Framework
- Java Grundlagen
- Die Anleitungen Java Date Time
- Java Open Source Bibliotheken
- Anleitungen Java Web Services
- Die Anleitungen Struts2 Framework
- Anleitungen Spring Boot