Die Anleitung zu Java PipedInputStream
1. PipedInputStream
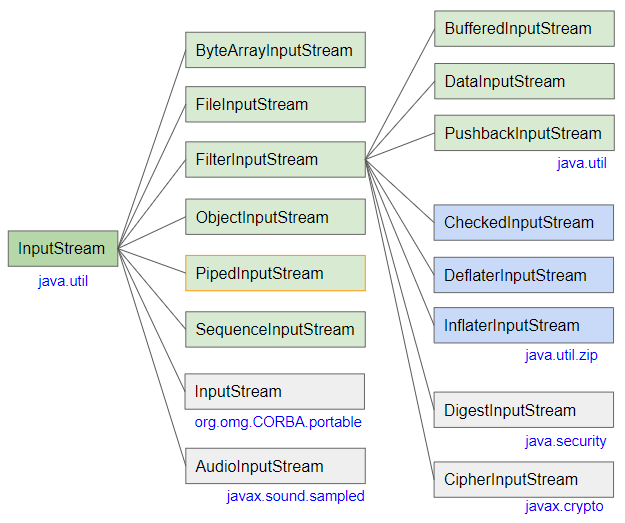
- InputStream
- BufferedInputStream
- SequenceInputStream
- FileInputStream
- ByteArrayInputStream
- ObjectInputStream
- PushbackInputStream
- FilterInputStream
- AudioInputStream
- DataInputStream
- InflaterInputStream
- DigestInputStream
- DeflaterInputStream
- CipherInputStream
- CheckedInputStream
Um PipedInputStream leicht zu verstehen, gebe ich eine Situation wie folgt an:
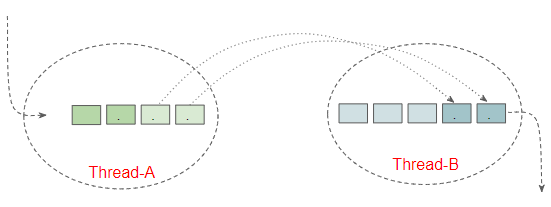
Angenommen, Sie entwickeln eine Anwendung Multithreading, und Sie haben zwei unabhängige Thread als Thread-A und Thread-B. Die Frage ist:
- Was ist zu tun, damit bytes jedes Mal, wenn sie in Thread-A auftreten, automatisch an Thread-B übertragen werden?
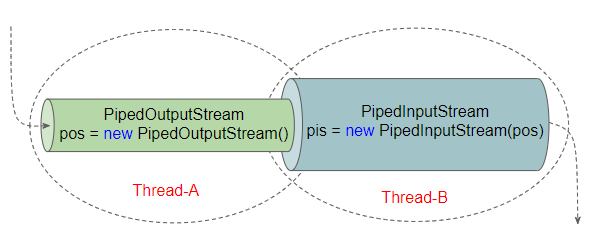
PipedOutputStream und PipedInputStream werden erstellt, damit Sie die oben erwähnte Situation umgehen. Jedes Mal, wenn die Daten in PipedOutputStream geschrieben werden, werden sie automatisch im PipedInputStream erscheinen.
PipedInputStream constructors
PipedInputStream()
PipedInputStream(int pipeSize)
PipedInputStream(PipedOutputStream src)
PipedInputStream(PipedOutputStream src, int pipeSize)
Damit die im PipedOutputStream geschriebenen Daten auf PipedInputStream auftreten, sollen Sie diese zwei Objekte verbinden (connect).
PipedOutputStream pipedOS = new PipedOutputStream();
PipedInputStream pipedIS = new PipedInputStream();
pipedOS.connect(pipedIS);
Die Code oben ist äquivalent mit der folgenden Wege:
PipedOutputStream pipedOS = new PipedOutputStream();
PipedInputStream pipedIS = new PipedInputStream();
pipedIS.connect(pipedOS);
PipedOutputStream pipedOS = new PipedOutputStream();
PipedInputStream pipedIS = new PipedInputStream(pipedOS);
PipedInputStream pipedIS = new PipedInputStream();
PipedOutputStream pipedOS = new PipedOutputStream(pipedIS);
- Die Anleitung zu Java PipedOutputStream
- Die Anleitung zu Java PipedWriter
2. Example 1
PipedInputStreamEx1.java
package org.o7planning.pipedinputstream.ex;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.PipedInputStream;
import java.io.PipedOutputStream;
public class PipedInputStreamEx1 {
private PipedInputStream pipedIS;
private PipedOutputStream pipedOS;
public static void main(String[] args) throws IOException, InterruptedException {
new PipedInputStreamEx1().test();
}
private void test() throws IOException, InterruptedException {
// Create a PipedInputStream
pipedIS = new PipedInputStream();
// Data written to 'pipedOS'
// will appear automatically at 'pipedIS'.
pipedOS = new PipedOutputStream(pipedIS);
new ThreadB().start();
new ThreadA().start();
}
//
class ThreadA extends Thread {
@Override
public void run() {
try {
byte[] bytes = new byte[] { 'a', 97, 'b', 'c', 101 };
for (byte b : bytes) {
pipedOS.write(b);
Thread.sleep(1000);
}
} catch (Exception e) {
e.printStackTrace();
} finally {
closeQuietly(pipedOS);
}
}
}
//
class ThreadB extends Thread {
@Override
public void run() {
try {
int b = 0;
while ((b = pipedIS.read()) != -1) {
System.out.println(b + " " + (char) b);
}
} catch (Exception e) {
e.printStackTrace();
} finally {
closeQuietly(pipedIS);
}
}
}
private void closeQuietly(InputStream in) {
if (in != null) {
try {
in.close();
} catch (IOException e) {
}
}
}
private void closeQuietly(OutputStream out) {
if (out != null) {
try {
out.close();
} catch (IOException e) {
}
}
}
}
Output:
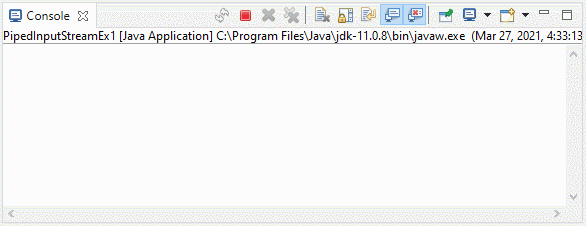
3. Example 2
Z.B: Verwenden Sie PipedInputStream, PipedOutputStream mit BufferedInputStream und BufferedOutputStream um die Leistung des Programm zu verbessern.
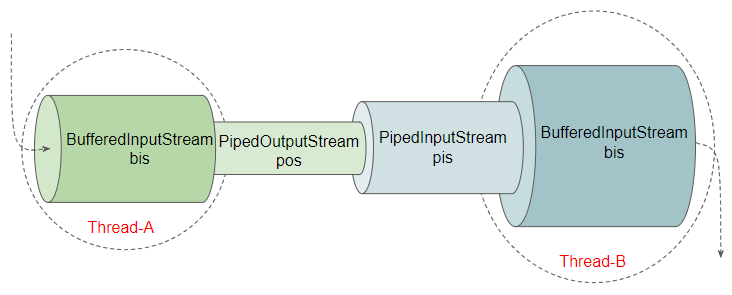
PipedInputStreamEx2.java
package org.o7planning.pipedinputstream.ex;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.PipedInputStream;
import java.io.PipedOutputStream;
public class PipedInputStreamEx2 {
private BufferedInputStream bufferedIS;
private BufferedOutputStream bufferedOS;
public static void main(String[] args) throws IOException, InterruptedException {
new PipedInputStreamEx2().test();
}
private void test() throws IOException, InterruptedException {
PipedInputStream pipedIS = new PipedInputStream();
PipedOutputStream pipedOS = new PipedOutputStream();
pipedIS.connect(pipedOS);
this.bufferedIS = new BufferedInputStream(pipedIS);
this.bufferedOS = new BufferedOutputStream(pipedOS);
new ThreadB().start();
new ThreadA().start();
}
//
class ThreadA extends Thread {
@Override
public void run() {
try {
byte[] bytes = new byte[] { 'a', 97, 'b', 'c', 101 };
for (byte b : bytes) {
bufferedOS.write(b);
}
} catch (Exception e) {
e.printStackTrace();
} finally {
closeQuietly(bufferedOS);
}
}
}
//
class ThreadB extends Thread {
@Override
public void run() {
try {
int code;
while ((code = bufferedIS.read()) != -1) {
System.out.println(code + " " + (char)code);
}
} catch (Exception e) {
e.printStackTrace();
} finally {
closeQuietly(bufferedIS);
}
}
}
private void closeQuietly(InputStream in) {
if (in != null) {
try {
in.close();
} catch (IOException e) {
}
}
}
private void closeQuietly(OutputStream out) {
if (out != null) {
try {
out.close();
} catch (IOException e) {
}
}
}
}
Die Anleitungen Java IO
- Die Anleitung zu Java CharArrayWriter
- Die Anleitung zu Java FilterReader
- Die Anleitung zu Java FilterWriter
- Die Anleitung zu Java PrintStream
- Die Anleitung zu Java BufferedReader
- Die Anleitung zu Java BufferedWriter
- Die Anleitung zu Java StringReader
- Die Anleitung zu Java StringWriter
- Die Anleitung zu Java PipedReader
- Die Anleitung zu Java LineNumberReader
- Die Anleitung zu Java PushbackReader
- Die Anleitung zu Java PrintWriter
- Die Anleitung zu Java IO Binary Streams
- Die Anleitung zu Java IO Character Streams
- Die Anleitung zu Java BufferedOutputStream
- Die Anleitung zu Java ByteArrayOutputStream
- Die Anleitung zu Java DataOutputStream
- Die Anleitung zu Java PipedInputStream
- Die Anleitung zu Java OutputStream
- Die Anleitung zu Java ObjectOutputStream
- Die Anleitung zu Java PushbackInputStream
- Die Anleitung zu Java SequenceInputStream
- Die Anleitung zu Java BufferedInputStream
- Die Anleitung zu Java Reader
- Die Anleitung zu Java Writer
- Die Anleitung zu Java FileReader
- Die Anleitung zu Java FileWriter
- Die Anleitung zu Java CharArrayReader
- Die Anleitung zu Java ByteArrayInputStream
- Die Anleitung zu Java DataInputStream
- Die Anleitung zu Java ObjectInputStream
- Die Anleitung zu Java InputStreamReader
- Die Anleitung zu Java OutputStreamWriter
- Die Anleitung zu Java InputStream
- Die Anleitung zu Java FileInputStream
Show More
- Anleitungen Java Servlet/JSP
- Die Anleitungen Java New IO
- Anleitungen Spring Cloud
- Die Anleitungen Oracle Java ADF
- Die Anleitungen Java Collections Framework
- Java Grundlagen
- Die Anleitungen Java Date Time
- Java Open Source Bibliotheken
- Anleitungen Java Web Services
- Die Anleitungen Struts2 Framework
- Anleitungen Spring Boot