Die Anleitung zu TypeScript Arrays
1. Was ist Array?
In TypeScript ist Array ein spezieller Datentyp, der zum Speichern vieler Werte vieler verschiedener Datentypen verwendet wird. Im Gegensatz zu Java kann Array in TypeScript seine Länge bei Bedarf automatisch erweitern.
Ein Type[]-Array in TypeScript hat dieselbe Methode zum Speichern von Daten wie Map<Integer,Type> in Java. Wir werden dies im Artikel klären.
Ähnlich wie JavaScript unterstützt TypeScript zwei Syntaxen zum Erstellen eines Arrays:
Die Syntax Type[]
Die Syntax Type[] ist die gängige Syntax zum Deklarieren eines Arrays, die in vielen verschiedenen Sprachen verwendet wird und natürlich TypeScript beinhaltet:
Beispiel: Deklarieren Sie ein Array, nur um string-Elemente zu speichern. Der TypeScript-Compiler meldet einen Fehler, wenn Sie versehentlich ein Element eines anderen Typs zum Array hinzufügen.
let fruits: string[];
fruits = ['Apple', 'Orange', 'Banana'];
// Or:
let fruits: string[] = ['Apple', 'Orange', 'Banana'];
Beispiel: Deklarieren Sie ein Array mit Elementen unterschiedlicher Datentypen:
let arr = [1, 3, 'Apple', 'Orange', 'Banana', true, false];
Beispiel: Deklarieren Sie ein Array mit dem union data type - number und string:
let values: (string | number)[] = ['Apple', 'Orange', 1, 2, 'Banana', 3];
Syntax Array<Type>
Das Deklarieren eines Arrays basierend auf der Syntax Array<Type> entspricht auch der Syntax Type[]. Es gibt keinen Unterschied.
let fruits: Array<string>;
fruits = ['Apple', 'Orange', 'Banana'];
// Or:
let fruits: Array<string> = ['Apple', 'Orange', 'Banana'];
In TypeScript ist die Syntax Type[] nur eine Kurzform der Syntax Array<Type>. Arrays in TypeScript sind Objekte der Interface Array<T>, entsprechen also den in dieser Interface definierten Standards (sehen Sie mehr unten).
Beispiel: Deklarieren Sie ein Array mit dem union data type - number und string:
let values: Array<string | number> = ['Apple', 'Orange', 1, 2, 'Banana', 3];
2. Auf Array-Elemente zugreifen
Auf die Array-Elemente kann über den Index eines Elements zugegriffen werden, z.B. myArray[index]. Der Array-Index beginnt bei 0, also ist der Index des ersten Elements 0, der Index des zweiten Elements ist eins und so weiter.
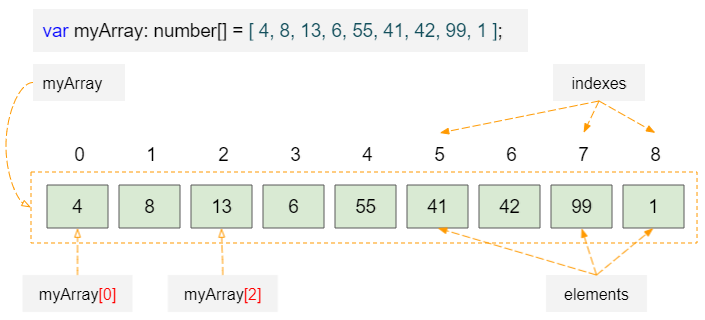
Zum Beispiel:
array_ex1.ts
function array_ex1_test() {
var myArray: number[] = [4, 8, 13, 6, 55, 41, 42, 99, 1];
console.log("Length of myArray: " + myArray.length); // 9 Elements
console.log("Element at index 0: " + myArray[0]); // 4
console.log("Element at index 1: " + myArray[1]); // 8
console.log("Element at index 4: " + myArray[4]); // 55
}
array_ex1_test(); // Call the function
Output:
Length of myArray: 9
Element at index 0: 4
Element at index 1: 8
Element at index 4: 55
Sie können den Elementen eines Arrays auch über ihren Index neue Werte zuweisen:
array_ex2.ts
function array_ex2_test() {
var fruits: string[] = ["Acerola", "Apple", "Banana" ];
console.log(`fruits[0] = ${fruits[0]}`);
console.log(`fruits[1] = ${fruits[1]}`);
console.log("--- Assign new values to elements ... --- ")
fruits[0] = "Breadfruit";
fruits[1] = "Carambola";
console.log(`fruits[0] = ${fruits[0]}`);
console.log(`fruits[1] = ${fruits[1]}`);
}
array_ex2_test(); // Call the function.
Output:
fruits[0] = Acerola
fruits[1] = Apple
--- Assign new values to elements ... ---
fruits[0] = Breadfruit
fruits[1] = Carambola
Im Gegensatz zu Java können Arrays in TypeScript bei Bedarf automatisch länger werden. Im folgenden Beispiel haben wir ein Array mit der Anfangslänge 3. Wir weisen den Elementen an Index 6 und 7 Werte zu. Die Länge des Arrays beträgt nun 8.
array_ex3.ts
function array_ex3_test() {
var fruits: string[] = ["Acerola", "Apple", "Banana" ];
console.log(`Array length is ${fruits.length}`); // 3
console.log(" --- Set the value for the elements at index 6 and 7. ---");
// Add more elements to the array.
fruits[6] = "Breadfruit";
fruits[7] = "Carambola";
console.log(`Now Array length is ${fruits.length}`); // 8 indexes: [0,1,2,3,4,5,6,7]
console.log(`Element at index 4: ${fruits[4]}`); // undefined
console.log(`Element at index 5: ${fruits[5]}`); // undefined
}
array_ex3_test(); // Call the function.
Output:
Array length is 3
--- Set the value for the elements at index 6 and 7. ---
Now Array length is 8
Element at index 4: undefined
Element at index 5: undefined
3. Nicht-integer Index
Im Gegensatz zu Java und C#. TypeScript-Arrays akzeptieren Nicht-Integer Indizes, die auch negative Indizes akzeptieren. Solche Elemente zählen jedoch nicht zur Länge des Arrays.
array_idx_ex1.ts
function array_idx_ex1_test() {
var fruits: string[] = ["Acerola", "Apple", "Banana" ];
console.log(`Array length is ${fruits.length}`); // 3
console.log(" --- Set the value for the elements at indexes 6, 10.5 and -100 ---");
// Add more elements to the array.
fruits[6] = "Breadfruit";
fruits[10.5] = "Carambola"; // !!!!!!!!!!
fruits[-100] = "Grapefruit"; // !!!!!!!!!!
console.log(`Now Array length is ${fruits.length}`); // 7 indexes: [0,1,2,3,4,5,6]
console.log(`Element at index 4: ${fruits[4]}`); // undefined
console.log(`Element at index 5: ${fruits[5]}`); // undefined
console.log(`Element at index 10.5: ${fruits[10.5]}`); // Carambola
console.log(`Element at index -100: ${fruits[-100]}`); // Grapefruit
}
array_idx_ex1_test(); // Call the function.
Output:
Array length is 3
--- Set the value for the elements at indexes 6, 10.5 and -100 ---
Now Array length is 7
Element at index 4: undefined
Element at index 5: undefined
Element at index 10.5: Carambola
Element at index -100: Grapefruit
4. Schleife über Array
Grundsätzlich gibt es zwei Schleifensyntaxen for für den Zugriff auf die Elemente eines Arrays.
Syntax 1:
Diese Syntax erlaubt nur den Zugriff auf Elemente mit einem nicht negativen Integer-Index.
for(let idx =0; idx < fruits.length; idx++) {
console.log(`Element at index ${idx} is ${fruits[idx]}`);
}
Zum Beispiel:
array_for_loop_ex1.ts
function array_for_loop_ex1_test() {
var fruits: string[] = ["Acerola", "Apple", "Banana" ];
console.log(`Array length is ${fruits.length}`); // 3 indexes [0,1,2]
console.log(" --- Set the value for the elements at indexes 6 and 10.5 ---");
// Add more elements to the array.
fruits[6] = "Breadfruit";
fruits[10.5] = "Carambola"; // !!!!!!!!!!
for(let idx =0; idx < fruits.length; idx++) {
console.log(`Element at index ${idx} is ${fruits[idx]}`);
}
}
array_for_loop_ex1_test(); // Call the function.
Output:
Element at index 0 is Acerola
Element at index 1 is Apple
Element at index 2 is Banana
Element at index 3 is undefined
Element at index 4 is undefined
Element at index 5 is undefined
Element at index 6 is Breadfruit
Syntax 2:
Die folgende Schleifensyntax for greift nur auf Indizes zu, die tatsächlich im Array vorhanden sind, einschließlich Indizes, die keinen Integer oder negative Zahlen sind.
for(let idx in fruits) {
console.log(`Element at index ${idx} is ${fruits[idx]}`);
}
Zum Beispiel:
array_for_loop_ex2.ts
function array_for_loop_ex2_test() {
var fruits: string[] = ["Acerola", "Apple", "Banana" ];
console.log(`Array length is ${fruits.length}`); // 3 indexes [0,1,2]
console.log(" --- Set the value for the elements at indexes 6 and 10.5 ---");
// Add more elements to the array.
fruits[6] = "Breadfruit";
fruits[10.5] = "Carambola"; // !!!!!!!!!!
for(let idx in fruits) {
console.log(`Element at index ${idx} is ${fruits[idx]}`);
}
}
array_for_loop_ex2_test(); // Call the function.
Output:
Element at index 0 is Acerola
Element at index 1 is Apple
Element at index 2 is Banana
Element at index 6 is Breadfruit
Element at index 10.5 is Carambola
Other Examples:
Beispiel: Ein Array, das Elemente vom Typ string enthält, wandelt alle seine Elemente in Großbuchstaben um.
array_for_loop_ex3.ts
function array_for_loop_ex3_test() {
var fruits: string[] = ["Acerola", "Apple", "Banana" ];
for(let idx in fruits) {
let v = fruits[idx];
if(v) {
fruits[idx] = v.toUpperCase();
}
}
// Print elements:
for(let idx in fruits) {
console.log(`Element at index ${idx} is ${fruits[idx]}`);
}
}
array_for_loop_ex3_test(); // Call the function.
Output:
Element at index 0 is ACEROLA
Element at index 1 is APPLE
Element at index 2 is BANANA
5. Schreibgeschütztes Array
Verwenden Sie das Schlüsselwort readonly in einer Array-Deklaration, um ein schreibgeschütztes Array zu erstellen. Das bedeutet, dass Sie keine Array-Elemente hinzufügen, entfernen oder aktualisieren können
- Hinweis: Das Schlüsselwort readonly ist nur in der Syntax Type[] zulässig, nicht in der Syntax Array<Type>.
let okArray1 : readonly string[] = ["Acerola", "Apple", "Banana" ]; // OK
let okArray2 : readonly string[];
okArray2 = ["Acerola", "Apple", "Banana" ]; // OK
let errorArray1 : readonly Array<String>; // Compile Error!!!
Ohne das Schlüsselwort readonly zu verwenden, können Sie auch mit der Interface ReadonlyArray<T> ein schreibgeschütztes Array deklarieren:
let okArray3 : ReadonlyArray<String>; // OK
Zum Beispiel:
let fruits: readonly string[] = ["Acerola", "Apple", "Banana" ]; // OK
fruits[1] = "Breadfruit"; // Compile Error!!!!!!
let years: ReadonlyArray<number> = [2001, 2010, 2020 ]; // OK
years[1] = 2021; // Compile Error!!!!!!
6. Array-Methoden
In TypeScript ist ein Array ein Objekt der Interface Array<T>, daher sind in dieser Interface Methoden definiert.
interface Array<T> {
length: number;
toString(): string;
toLocaleString(): string;
pop(): T | undefined;
push(...items: T[]): number;
concat(...items: ConcatArray<T>[]): T[];
concat(...items: (T | ConcatArray<T>)[]): T[];
join(separator?: string): string;
reverse(): T[];
shift(): T | undefined;
slice(start?: number, end?: number): T[];
sort(compareFn?: (a: T, b: T) => number): this;
splice(start: number, deleteCount?: number): T[];
splice(start: number, deleteCount: number, ...items: T[]): T[];
unshift(...items: T[]): number;
indexOf(searchElement: T, fromIndex?: number): number;
lastIndexOf(searchElement: T, fromIndex?: number): number;
every<S extends T>(predicate: (value: T, index: number, array: T[]) => value is S, thisArg?: any): this is S[];
every(predicate: (value: T, index: number, array: T[]) => unknown, thisArg?: any): boolean;
some(predicate: (value: T, index: number, array: T[]) => unknown, thisArg?: any): boolean;
forEach(callbackfn: (value: T, index: number, array: T[]) => void, thisArg?: any): void;
map<U>(callbackfn: (value: T, index: number, array: T[]) => U, thisArg?: any): U[];
filter<S extends T>(predicate: (value: T, index: number, array: T[]) => value is S, thisArg?: any): S[];
filter(predicate: (value: T, index: number, array: T[]) => unknown, thisArg?: any): T[];
reduce(callbackfn: (previousValue: T, currentValue: T, currentIndex: number, array: T[]) => T): T;
reduce(callbackfn: (previousValue: T, currentValue: T, currentIndex: number, array: T[]) => T, initialValue: T): T;
reduce<U>(callbackfn: (previousValue: U, currentValue: T, currentIndex: number, array: T[]) => U, initialValue: U): U;
reduceRight(callbackfn: (previousValue: T, currentValue: T, currentIndex: number, array: T[]) => T): T;
reduceRight(callbackfn: (previousValue: T, currentValue: T, currentIndex: number, array: T[]) => T, initialValue: T): T;
reduceRight<U>(callbackfn: (previousValue: U, currentValue: T, currentIndex: number, array: T[]) => U, initialValue: U): U;
[n: number]: T;
}
7. forEach(..)
forEach(callbackfn: (value: T, index: number, array: T[]) => void, thisArg?: any): void;
Führt die angegebene Aktion für jedes Element in einem Array aus.
Zum Beispiel:
array_forEach_ex1.ts
function array_forEach_ex1_test() {
let fruits: string[] = ["Acerola", "Apple", "Banana" ]; // OK
// A callback function.
var callback = function(value:string, index:number, thisArray:string[]) {
if(index % 2 == 0) {
console.log(value);
} else {
console.log(value.toUpperCase());
}
}
fruits.forEach(callback);
}
array_forEach_ex1_test(); // Call the function.
Output:
Acerola
APPLE
Banana
8. every(..)
every(predicate: (value: T, index: number, array: T[]) => unknown, thisArg?: any): boolean;
Überprüft, ob alle Elemente eines Arrays den Test einer angegebenen Funktion bestehen.
predicate: Eine Funktion zum Überprüfen jedes Elements des Arrays.
Beispiel: Ein Array besteht aus Jahren. Prüfen Sie, ob alle Jahre größer als 1990 sind.
array_every_ex1.ts
function array_every_ex1_test() {
let years: number[] = [2001, 1995, 2007, 2010, 2020];
// Arrow function.
var predicate = (value: number, index: number, thisArray: number[]) => {
return value > 1990;
}
var ok: boolean = years.every(predicate);
console.log(`All years > 1990? ${ok}`); // true
}
array_every_ex1_test(); // Call the function.
9. sort(..)
sort(compareFn?: (a: T, b: T) => number): this;
Sortiert dieses Array basierend auf der angegebenen Funktion und gibt dieses Array zurück. Wenn keine Funktion bereitgestellt wird, werden die Elemente des Arrays nach natürlichen Regeln sortiert.
Beispiel: Sortieren eines Arrays string:
array_sort_ex1.ts
function array_sort_ex1_test() {
let fruits: string[] = ["Banana", "Acerola", "Apple", "Carambola", "Breadfruit"];
// Compare Function:
var compareFn = (a: string, b: string) => {
// v > 0 --> a > b
// v = 0 --> a == b
// v < 0 --> a < b
let v: number = a.localeCompare(b);
return v;
}
fruits.sort(compareFn);
console.log("--- after sorting --- ");
console.log(fruits);
}
array_sort_ex1_test(); // Call the function.
Output:
--- after sorting ---
[ 'Acerola', 'Apple', 'Banana', 'Breadfruit', 'Carambola' ]
10. filter(..)
filter(predicate: (value: T, index: number, array: T[]) => unknown, thisArg?: any): T[];
filter<S extends T>(predicate: (value: T, index: number, array: T[]) => value is S, thisArg?: any): S[];
Gibt ein neues Array von Elementen zurück, die den Test einer angegebenen Funktion bestehen.
Beispiel: Ein Array besteht aus Jahren, filtern Sie ein neues Array heraus, das nur aus geraden Jahren besteht.
array_filter_ex1.ts
function array_filter_ex1_test() {
let years: number[] = [2001, 1995, 2007, 2010, 2020];
// Filter even value.
var predicate = (value: number, index: number, thisArray: number[]) => {
return value % 2 == 0;
}
var evenYears: number[] = years.filter(predicate);
console.log(" --- Even Years: --- ");
console.log(evenYears);
}
array_filter_ex1_test(); // Call the function.
Output:
--- Even Years: ---
[ 2010, 2020 ]
Anleitungen Typescript
- Führen Sie Ihr erstes TypeScript-Beispiel in Visual Studio Code aus
- Die Anleitung zu TypeScript Namespaces
- Die Anleitung zu TypeScript Module
- Typeof-Operator in der TypeScript-Sprache
- Schleifen in TypeScript
- Installieren Sie das TypeScript unter Windows
- Funktionen in TypeScript
- Die Anleitung zu TypeScript Tuples
- Schnittstellen in TypeScript
- Die Anleitung zu TypeScript Arrays
- Operator instanceof in der TypeScript-Sprache
- Methoden in TypeScript
- Die Anleitung zu TypeScript Closures
- Konstruktoren in TypeScript
- Eigenschaften in TypeScript
- Analysieren von JSON in TypeScript
- Analysieren von JSON in TypeScript mit der json2typescript-Bibliothek
- Was ist Transpiler?
Show More