Analysieren von JSON in TypeScript mit der json2typescript-Bibliothek
1. json2typescript
json2typescript ist eine Bibliothek, die Hilfsklassen (helper classes) enthält, um eine Mapping zwischen einem JSON-Dokument und einem TypeScript-Objekt zu definieren. Diese Bibliothek ist von Java Jackson inspiriert und hat ähnliche Funktionen.
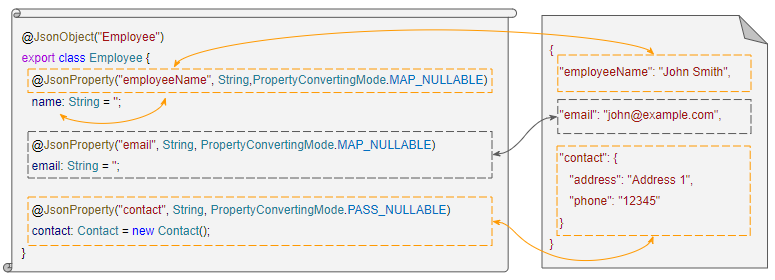
Grundsätzlich eignet sich json2typescript für die großen Projekte, die wenige Konfigurationsschritte erfordern. Wenn Sie nur mit JSON in einem kleinen TypeScript-Projekt arbeiten, sollten Sie die Methoden JSON.parse(..) und JSON.stringify(..) verwenden, die einfach sind und keine zusätzliche Konfiguration erfordern.
2. Die Installation
Installieren Sie zuerst die Bibliothek json2typescript im Projekt:
npm install json2typescript
Fügen Sie als Nächstes die folgenden Eigenschaften zur Datei tsconfig.json hinzu:
tsconfig.json
{
"compilerOptions": {
...
"experimentalDecorators": true,
"allowJs": true
}
}
3. Grundsätzliches Beispiel:
In diesem Beispiel erstellen wir 2 Dateien model:
- model_file1.ts
- model_file2.ts.
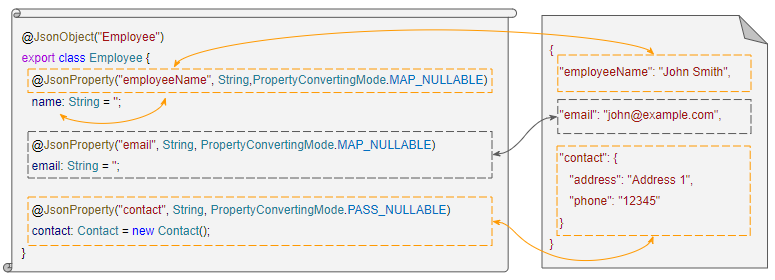
Klassen werden in den Dateien model definiert. Verwenden Sie @JsonObject und @JsonProperty, um die Klasse und ihre Eigenschaften zu kommentieren, um dem Tool vorzuschlagen, wie TypeScript-Objekte in JSON-Text konvertiert werden und umgekehrt.
@JsonObject
Eine Klasse wird mit @JsonObject annotiert, dann kann ihr Objekt in einen JSON-Text konvertiert werden und umgekehrt.
@JsonProperty
Eine Eigenschaft eines TypeScript-Objekts kann in eine JSON-Eigenschaft konvertiert werden, wenn sie mit @JsonProperty kommentiert wird. Andernfalls wird es ignoriert.
Alle mit @JsonProperty annotierten Eigenschaften der TypeScript-Klasse müssen auf Standardwerte gesetzt werden. Andernfalls entspricht das Ergebnis möglicherweise nicht Ihren Erwartungen.
model_file1.ts
import {
JsonObject, JsonProperty, JsonConvert, OperationMode,
ValueCheckingMode, PropertyConvertingMode
} from "json2typescript";
@JsonObject("Contact")
export class Contact {
// PropertyConvertingMode.MAP_NULLABLE is default.
// MAP_NULLABLE: Throw Exception if JSON value is undefined
// IGNORE_NULLABLE: Using Java property default value if JSON value is undefined
// PASS_NULLABLE: Set Java property value to null if JSON value is undefined
@JsonProperty("address", String, PropertyConvertingMode.MAP_NULLABLE)
address: string = '';
@JsonProperty("phone", String, PropertyConvertingMode.MAP_NULLABLE)
phone: string = '';
}
@JsonObject("Employee")
export class Employee {
@JsonProperty("employeeName", String, PropertyConvertingMode.MAP_NULLABLE)
name: string = '';
@JsonProperty("email", String, PropertyConvertingMode.MAP_NULLABLE)
email: string = '';
@JsonProperty("contact", Contact, PropertyConvertingMode.PASS_NULLABLE)
contact?: Contact = new Contact();
}
PropertyConvertingMode.MAP_NULLABLE:
Dies ist der Standardkonvertierungsmodus. Eine Ausnahme wird ausgelöst, wenn dieser Eigenschaft der TypeScript-Klasse keine JSON-Eigenschaft entspricht.
PropertyConvertingMode.IGNORE_NULLABLE:
Die Eigenschaft des TypeScript-Objekts erhält den Standardwert, wenn es keine entsprechende Eigenschaft in JSON gibt.
PropertyConvertingMode.PASS_NULLABLE:
Die Eigenschaft des TypeScript-Objekts ist null, wenn es keine entsprechende Eigenschaft in JSON gibt.
model_file2.ts
import {
JsonObject, JsonProperty, JsonConvert, OperationMode,
ValueCheckingMode, PropertyConvertingMode
} from "json2typescript";
import { Contact } from './model_file1';
@JsonObject("Company")
class Company {
@JsonProperty("companyName", String, PropertyConvertingMode.MAP_NULLABLE)
name: string = '';
@JsonProperty("contact", Contact, PropertyConvertingMode.PASS_NULLABLE)
contact?: Contact = new Contact();
}
Wenn Sie Visual Studio Code zum Schreiben von Code verwenden, erhalten Sie möglicherweise eine Fehlermeldung wie unten. Sehen Sie, wie Sie den auf stackoverflow geteilten Fehler beheben:Experimental support for decorators is a feature that is subject to change in a future release. Set the 'experimentalDecorators' option in your 'tsconfig' or 'jsconfig' to remove this warning.ts(1219)
json2typescript_ex1.ts
import {
JsonObject, JsonConvert, OperationMode, ValueCheckingMode
} from "json2typescript";
import { Employee, Contact } from './model_file1';
// @see Employee class in model_file1.ts
let jsonStr = ` {
"employeeName": "John Smith",
"email": "john@example.com",
"contact": {
"address": "Address 1",
"phone": "12345"
}
} `;
let jsonConvert: JsonConvert = new JsonConvert();
// ----------- JSON Text to TypeScript Object ------------------
let jsonObj: any = JSON.parse(jsonStr);
let emp: Employee = jsonConvert.deserializeObject(jsonObj, Employee);
console.log('JSON to TypeScript:');
console.log(emp);
console.log(' ------------- ');
// ----------- TypeScript Object to JSON Text ------------------
let jsonText = jsonConvert.serialize(emp);
console.log('TypeScript to JSON:');
console.log(jsonText);
Output:
JSON to TypeScript:
Employee {
name: 'John Smith',
email: 'john@example.com',
contact: Contact { address: 'Address 1', phone: '12345' }
}
-------------
TypeScript to JSON:
{
employeeName: 'John Smith',
email: 'john@example.com',
contact: { address: 'Address 1', phone: '12345' }
}
json2typescript_ex2.ts
import {
JsonObject, JsonConvert, OperationMode, ValueCheckingMode
} from "json2typescript";
import { Employee, Contact } from './model_file1';
// @see Employee class in model_file1.ts
let jsonStr = ` {
"employeeName": "John Smith",
"email": "john@example.com"
} `;
let jsonConvert: JsonConvert = new JsonConvert();
// ----------- JSON Text to TypeScript Object ------------------
let jsonObj: any = JSON.parse(jsonStr);
let emp: Employee = jsonConvert.deserializeObject(jsonObj, Employee);
console.log('JSON to TypeScript:');
console.log(emp);
console.log(' ------------- ');
// ----------- TypeScript Object to JSON Text ------------------
let jsonText = jsonConvert.serialize(emp);
console.log('TypeScript to JSON:');
console.log(jsonText);
Output:
JSON to TypeScript:
Employee {
name: 'John Smith',
email: 'john@example.com',
contact: undefined
}
-------------
TypeScript to JSON:
{
employeeName: 'John Smith',
email: 'john@example.com',
contact: undefined
}
4. @JsonProperty
Eine Eigenschaft eines TypeScript-Objekts kann in eine JSON-Eigenschaft konvertiert werden, wenn sie mit @JsonProperty kommentiert wird. Andernfalls wird es ignoriert.
Alle mit @JsonProperty annotierten Eigenschaften der TypeScript-Klasse müssen auf Standardwerte gesetzt werden. Andernfalls entspricht das Ergebnis möglicherweise nicht Ihren Erwartungen.
Zum Beispiel:
// Model class:
@JsonObject("User")
export class User {
@JsonProperty("userName", String, PropertyConvertingMode.MAP_NULLABLE)
userName: string = '';
@JsonProperty("joinDate", DateConverter)
joinDate: Date = new Date();
}
Die Syntax:
@JsonProperty("JSON_Property_Name", JSON_Type, Custom_Converter, PropertyConvertingMode)
JSON_Property_Name
Der Name des Property in JSON.
JSON_Type (Optional)
Ein JSON-Datentyp.
- String, [String]
- Number, [Number]
- Boolean, [Boolean]
- Any, [Any]
Custom_Converter (Optional)
Sehen Sie die Beispiele im Abschnitt @JsonConverter.
PropertyConvertingMode (Optional)
PropertyConvertingMode.MAP_NULLABLE:
- Dies ist der Standardkonvertierungsmodus. Eine Ausnahme wird ausgelöst, wenn dieser Eigenschaft der TypeScript-Klasse keine JSON-Eigenschaft entspricht.
PropertyConvertingMode.IGNORE_NULLABLE:
- Die Eigenschaft des TypeScript-Objekts erhält den Standardwert, wenn es keine entsprechende Eigenschaft in JSON gibt.
PropertyConvertingMode.PASS_NULLABLE:
- Die Eigenschaft des TypeScript-Objekts ist null, wenn es keine entsprechende Eigenschaft in JSON gibt.
5. @JsonConverter
In einigen Fällen müssen Sie möglicherweise eine benutzerdefinierte Konvertierung zwischen JSON-Objekten und TypeScript-Objekten vornehmen. Sie können benutzerdefinierte Konverter wie folgt definieren:
model_file3.ts
import {
JsonObject, JsonProperty, JsonConvert, OperationMode,
ValueCheckingMode, PropertyConvertingMode, JsonConverter, JsonCustomConvert
} from "json2typescript";
// Custom Converter:
@JsonConverter
class DateConverter implements JsonCustomConvert<Date> {
serialize(date: Date): any {
return date.getFullYear() + "-" + (date.getMonth() + 1) + "-" + date.getDate();
}
deserialize(date: any): Date {
return new Date(date);
}
}
// Model class:
@JsonObject("User")
export class User {
@JsonProperty("userName", String, PropertyConvertingMode.MAP_NULLABLE)
userName: string = '';
@JsonProperty("joinDate", DateConverter)
joinDate: Date = new Date();
}
json2typescript_ex3.ts
import {
JsonObject, JsonConvert, OperationMode, ValueCheckingMode
} from "json2typescript";
import { User } from './model_file3';
// @see User class in model_file3.ts
let jsonStr = ` {
"userName": "tom",
"joinDate": "2021-12-15"
} `;
let jsonConvert: JsonConvert = new JsonConvert();
// ----------- JSON Text to TypeScript Object ------------------
let jsonObj: any = JSON.parse(jsonStr);
let user: User = jsonConvert.deserializeObject(jsonObj, User);
console.log('JSON to TypeScript:');
console.log(user);
console.log(' ------------- ');
// ----------- TypeScript Object to JSON Text ------------------
let jsonText = jsonConvert.serialize(user);
console.log('TypeScript to JSON:');
console.log(jsonText);
Output:
JSON to TypeScript:
User { userName: 'tom', joinDate: 2021-12-15T00:00:00.000Z }
-------------
TypeScript to JSON:
{ userName: 'tom', joinDate: '2021-12-15' }
Anleitungen Typescript
- Führen Sie Ihr erstes TypeScript-Beispiel in Visual Studio Code aus
- Die Anleitung zu TypeScript Namespaces
- Die Anleitung zu TypeScript Module
- Typeof-Operator in der TypeScript-Sprache
- Schleifen in TypeScript
- Installieren Sie das TypeScript unter Windows
- Funktionen in TypeScript
- Die Anleitung zu TypeScript Tuples
- Schnittstellen in TypeScript
- Die Anleitung zu TypeScript Arrays
- Operator instanceof in der TypeScript-Sprache
- Methoden in TypeScript
- Die Anleitung zu TypeScript Closures
- Konstruktoren in TypeScript
- Eigenschaften in TypeScript
- Analysieren von JSON in TypeScript
- Analysieren von JSON in TypeScript mit der json2typescript-Bibliothek
- Was ist Transpiler?
Show More