Analysieren von JSON in TypeScript
1. TypeScript JSON
Wie Sie wissen, kann TypeScript als erweiterte Version von TypeScript betrachtet werden. Schließlich wird TypeScript auch in JavaScript-Code umgewandelt, der im Browser oder anderen TypeScript-Umgebungen wie NodeJS ausgeführt werden kann. TypeScript unterstützt alle JavaScript-Bibliotheken und API-Dokumentation.
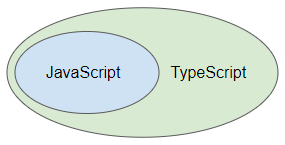
Die einfachste Methode zum Analysieren von JSON-Daten ist die Verwendung der Methoden JSON.parse(..) und JSON.stringify(..). Es gibt keinen Unterschied in der Verwendung in JavaScript und TypeScript.
JSON
// Static methods:
parse(text: string, reviver?: (this: any, key: string, value: any) => any): any;
stringify(value: any, replacer?: (this: any, key: string, value: any) => any, space?: string | number): string;
stringify(value: any, replacer?: (number | string)[] | null, space?: string | number): string;
Erwägen Sie die Verwendung einer der folgenden JSON-Bibliotheken, wenn Sie ein großes TypeScript-Projekt entwickeln.
2. JSON.parse(..)
parse(text: string, reviver?: (this: any, key: string, value: any) => any): any;
Konvertiert einen JSON-Text in ein TypeScript-Objekt.
- text: Ein JSON-Text.
- reviver: Eine optionale Funktion, die für jede Eigenschaft dieses JSON aufgerufen wird. Der Parameter [key=''] entspricht dem globalen JSON-Objekt. Eigenschaften auf Blattebene werden zuerst ausgeführt, Eigenschaften auf Stammebene werden zuletzt ausgeführt.
Beispiel: Verwenden Sie die JSON.parse(..)-Methode, um einen JSON-Text in ein Objekt umzuwandeln.
json_parse_ex1.ts
function json_parse_ex1_test() {
let jsonString = ` {
"name": "John Smith",
"email": "john@example.com",
"contact": {
"address": "Address 1",
"phone": "12345"
}
}
`;
// Return an object.
let empObj = JSON.parse(jsonString);
console.log(empObj);
console.log(`Address: ${empObj.contact.address}`);
}
json_parse_ex1_test(); // Call the function.
Output:
{
name: 'John Smith',
email: 'john@example.com',
contact: { address: 'Address 1', phone: '12345' }
}
Address: Address 1
Beispiel: Verwenden Sie die Methode JSON.parse(..) unter Beteiligung der revive-Funktion, um einen JSON-Text in ein Objekt einer Klasse zu konvertieren.
json_parse_ex2.ts
class Employee {
employeeName: string;
employeeEmail: string;
contact: Contact;
constructor(employeeName: string, employeeEmail: string, contact: Contact) {
this.employeeName = employeeName;
this.employeeEmail = employeeEmail;
this.contact = contact;
}
}
class Contact {
address: string;
phone: string;
constructor(address: string, phone: string) {
this.address = address;
this.phone = phone;
}
}
function json_parse_ex2_test() {
let jsonString = ` {
"name": "John Smith",
"email": "john@example.com",
"contact": {
"address": "Address 1",
"phone": "12345"
}
}
`;
// Return an object.
let empObj = JSON.parse(jsonString, function (key: string, value: any) {
if(key == 'name') {
return (value as string).toUpperCase(); // String.
} else if (key == 'contact') {
return new Contact(value.address, value.phone);
} else if (key == '') { // Entire JSON
return new Employee(value.name, value.email, value.contact);
}
return value;
});
console.log(empObj);
console.log(`Employee Name: ${empObj.employeeName}`);
console.log(`Employee Email: ${empObj.employeeEmail}`);
console.log(`Phone: ${empObj.contact.phone}`);
}
json_parse_ex2_test(); // Call the function.
Output:
Employee {
employeeName: 'JOHN SMITH',
employeeEmail: 'john@example.com',
contact: Contact { address: 'Address 1', phone: '12345' }
}
Employee Name: JOHN SMITH
Employee Email: john@example.com
Phone: 12345
3. JSON.stringify(..) *
stringify(value: any, replacer?: (this: any, key: string, value: any) => any, space?: string | number): string;
Konvertiert einen Wert oder ein Objekt in einen JSON-Text.
- value: Ein Wert, normalerweise ein Objekt oder ein Array, der in JSON-Text konvertiert werden soll.
- replacer: Eine optionale Funktion, die verwendet wird, um die Ergebnisse anzupassen.
- space: Fügen Sie Einzüge, Leerzeichen oder Zeilenumbruchzeichen zu JSON-Text hinzu, um ihn leichter lesbar zu machen.
Beispiel: Konvertieren von Werten in JSON-Text:
json_stringify_ex1a.ts
console.dir(JSON.stringify(1));
console.dir(JSON.stringify(5.9));
console.dir(JSON.stringify(true));
console.dir(JSON.stringify(false));
console.dir(JSON.stringify('falcon'));
console.dir(JSON.stringify("sky"));
console.dir(JSON.stringify(null));
Output:
'1'
'5.9'
'true'
'false'
'"falcon"'
'"sky"'
'null'
Beispiel: Konvertieren eines Objekts in einen JSON-Text:
json_stringify_ex1b.ts
function json_stringify_ex1b_test() {
// An Object:
let emp = {
name: 'John Smith',
email: 'john@example.com',
contact: { address: 'Address 1', phone: '12345' }
};
let jsonString = JSON.stringify(emp);
console.log(jsonString);
}
json_stringify_ex1b_test(); // Call the function.
Output:
{"name":"John Smith","email":"john@example.com","contact":{"address":"Address 1","phone":"12345"}}
Beispiel: Verwendung der JSON.stringify(..)-Methode mit Parameter replacer.
json_stringify_replacer_ex1a.ts
function json_stringify_replacer_ex1a_test() {
// An Object:
let emp = {
name: 'John Smith',
email: 'john@example.com',
contact: { address: 'Address 1', phone: '12345' }
};
let jsonString = JSON.stringify(emp, function (key: string, value: any) {
if (key == 'contact') {
return undefined;
} else if (key == 'name') {
return (value as string).toUpperCase();
}
return value;
});
console.log(jsonString);
}
json_stringify_replacer_ex1a_test(); // Call the function.
Output:
{"name":"JOHN SMITH","email":"john@example.com"}
4. JSON.stringify(..) **
stringify(value: any, replacer?: (number | string)[] | null, space?: string | number): string;
Konvertiert einen Wert oder ein Objekt in einen JSON-Text.
- value: Ein Wert, normalerweise ein Objekt oder ein Array, der in JSON-Text konvertiert werden soll.
- replacer: Ein optionales Array aus Strings oder Zahlen. Als Liste genehmigter Eigenschaften werden sie im zurückgegebenen JSON-Text angezeigt.
- space: Fügen Sie Einzüge, Leerzeichen oder Zeilenumbruchzeichen zu JSON-Text hinzu, um ihn leichter lesbar zu machen.
json_stringify_replacer_ex2a.ts
function json_stringify_replacer_ex2a_test() {
// An Object:
let emp = {
name: 'John Smith',
email: 'john@example.com',
contact: { address: 'Address 1', phone: '12345' }
};
let replacer = ['email', 'contact', 'address'];
let jsonString = JSON.stringify(emp, replacer, ' ');
console.log(jsonString);
}
json_stringify_replacer_ex2a_test(); // Call the function.
Output:
{
"email": "john@example.com",
"contact": {
"address": "Address 1"
}
}
Anleitungen Typescript
- Führen Sie Ihr erstes TypeScript-Beispiel in Visual Studio Code aus
- Die Anleitung zu TypeScript Namespaces
- Die Anleitung zu TypeScript Module
- Typeof-Operator in der TypeScript-Sprache
- Schleifen in TypeScript
- Installieren Sie das TypeScript unter Windows
- Funktionen in TypeScript
- Die Anleitung zu TypeScript Tuples
- Schnittstellen in TypeScript
- Die Anleitung zu TypeScript Arrays
- Operator instanceof in der TypeScript-Sprache
- Methoden in TypeScript
- Die Anleitung zu TypeScript Closures
- Konstruktoren in TypeScript
- Eigenschaften in TypeScript
- Analysieren von JSON in TypeScript
- Analysieren von JSON in TypeScript mit der json2typescript-Bibliothek
- Was ist Transpiler?
Show More