Die Anleitung zu Java Commons Email
1. Die Vorstellung

Commons Email ist eine Java Bibliothek von Apache, die auf Email bezieht. In der Praxis vom Umgang mit Java Email können Sie JavaMailAPI verwenden, die im JDK6 bereit integriert wird. Commons Email hilfts Ihnen bei dem Umgang mit JavaMail API einfacher, Sie ist kein Ersatz von JavaMail API.
Sowie ist Commons IO kein Ersatz von JavaIO , es enthaltet sondern viele Utility- Method und - Class, damit Sie mit Java IO einfacher arbeiten und die Zeit bei der Code sparen
2. Die Bibliothek
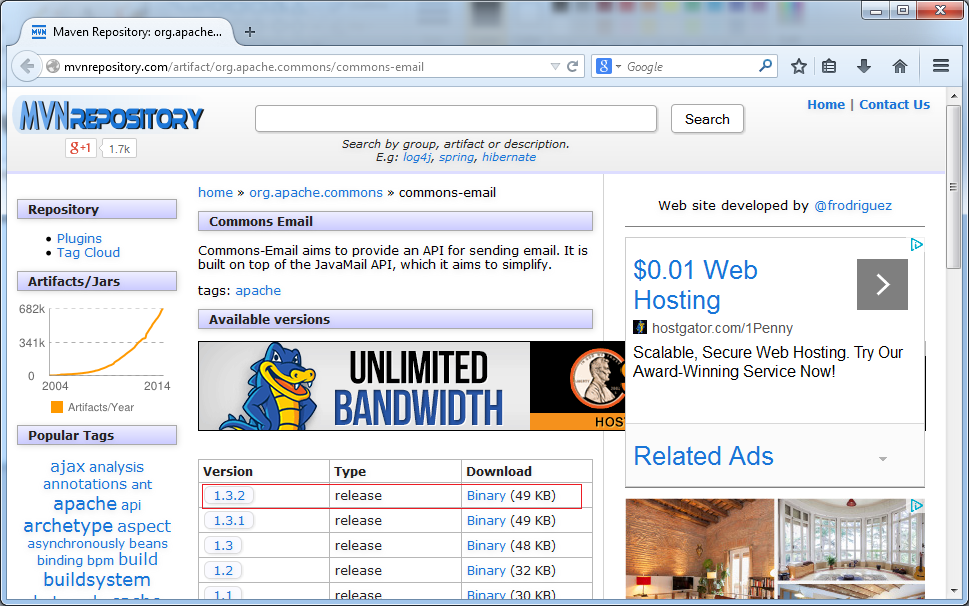
Maven:
** pom.xml **
<!-- http://mvnrepository.com/artifact/org.apache.commons/commons-email -->
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-email</artifactId>
<version>1.3.2</version>
</dependency>
See Also:
3. Die Achtung für Gmail
Wenn Sie die Email aus der Java Applikation benutzend Gmail schicken möchten, brauchen Sie die Applikation weniger sicher genehmigen (Allow less secure apps):
Auf dem Browser melden Sie Ihre Gmail an und greifen den folgenden Pfad zu:
Sie werden eine Benachrichtigung von Google bekommenEinige Applikation und das Gerät benuzt die weniger sichere Sign-in Technologie, das Ihr Konto mehr gefährdet macht. Sie können den Zugang für die Appliaktion schließen (Turn Off) , die wir empfahlen oder den Zugang öffnen (Turn On) wenn Sie die Risiken akzeptieren
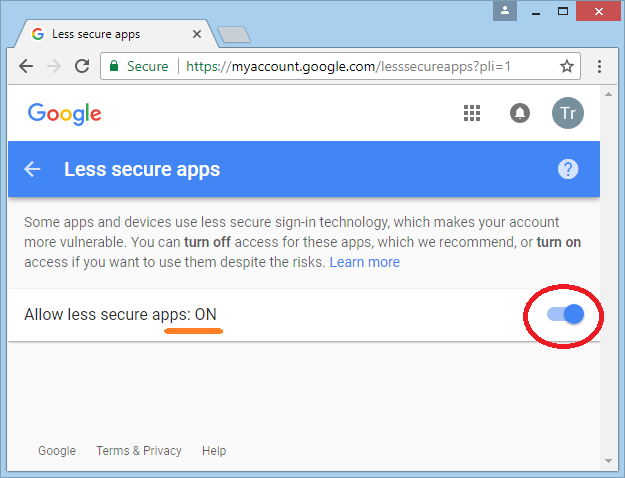
4. Zum Beispiel: die Email einfach schicken
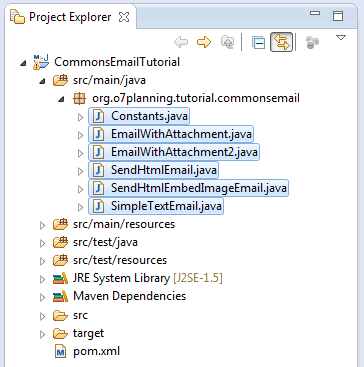
Zuerst ist das ein Beispiel zum Email Schicken mit Commons-Email
Constants.java
package org.o7planning.tutorial.commonsemail;
public class Constants {
public static final String MY_EMAIL = "yourEmail@gmail.com";
public static final String MY_PASSWORD ="your password";
public static final String FRIEND_EMAIL = "friendEmail@gmail.com";
}
SimpleTextEmail.java
package org.o7planning.tutorial.commonsemail;
import org.apache.commons.mail.DefaultAuthenticator;
import org.apache.commons.mail.Email;
import org.apache.commons.mail.SimpleEmail;
public class SimpleTextEmail {
public static void main(String[] args) {
try {
Email email = new SimpleEmail();
// Configuration
email.setHostName("smtp.googlemail.com");
email.setSmtpPort(465);
email.setAuthenticator(new DefaultAuthenticator(Constants.MY_EMAIL,
Constants.MY_PASSWORD));
// Required for gmail
email.setSSLOnConnect(true);
// Sender
email.setFrom(Constants.MY_EMAIL);
// Email title
email.setSubject("Test Email");
// Email message.
email.setMsg("This is a test mail ... :-)");
// Receiver
email.addTo(Constants.FRIEND_EMAIL);
email.send();
System.out.println("Sent!!");
} catch (Exception e) {
e.printStackTrace();
}
}
}
Das Ergebnis vom Laufen des oben Beispiel
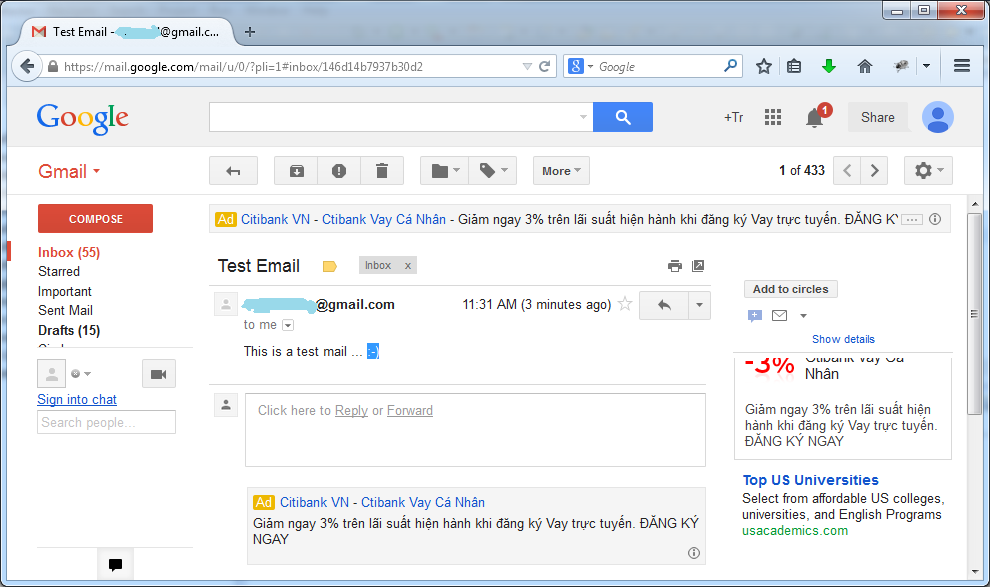
5. Ein beigefügten Email schicken
Das Beispiel : Ein Email schicken, in der eine File auf dem Festplatte beigefügt wird.
EmailWithAttachment.java
package org.o7planning.tutorial.commonsemail;
import org.apache.commons.mail.DefaultAuthenticator;
import org.apache.commons.mail.EmailAttachment;
import org.apache.commons.mail.MultiPartEmail;
public class EmailWithAttachment {
public static void main(String[] args) {
try {
// Create the attachment
EmailAttachment attachment = new EmailAttachment();
attachment.setPath("C:/mypictures/map-vietnam.png");
attachment.setDisposition(EmailAttachment.ATTACHMENT);
attachment.setDescription("Vietnam Map");
attachment.setName("Map");
// Create the email message
MultiPartEmail email = new MultiPartEmail();
// Configuration
email.setHostName("smtp.googlemail.com");
email.setSmtpPort(465);
email.setSSLOnConnect(true);
email.setAuthenticator(new DefaultAuthenticator(Constants.MY_EMAIL,
Constants.MY_PASSWORD));
email.setFrom(Constants.MY_EMAIL, "TRAN");
email.addTo(Constants.FRIEND_EMAIL, "Hong");
email.setSubject("The Map");
email.setMsg("Here is the map you wanted");
// Add the attachment
email.attach(attachment);
// Send the email
email.send();
System.out.println("Sent!");
} catch (Exception e) {
e.printStackTrace();
}
}
}
Das Ergebnis
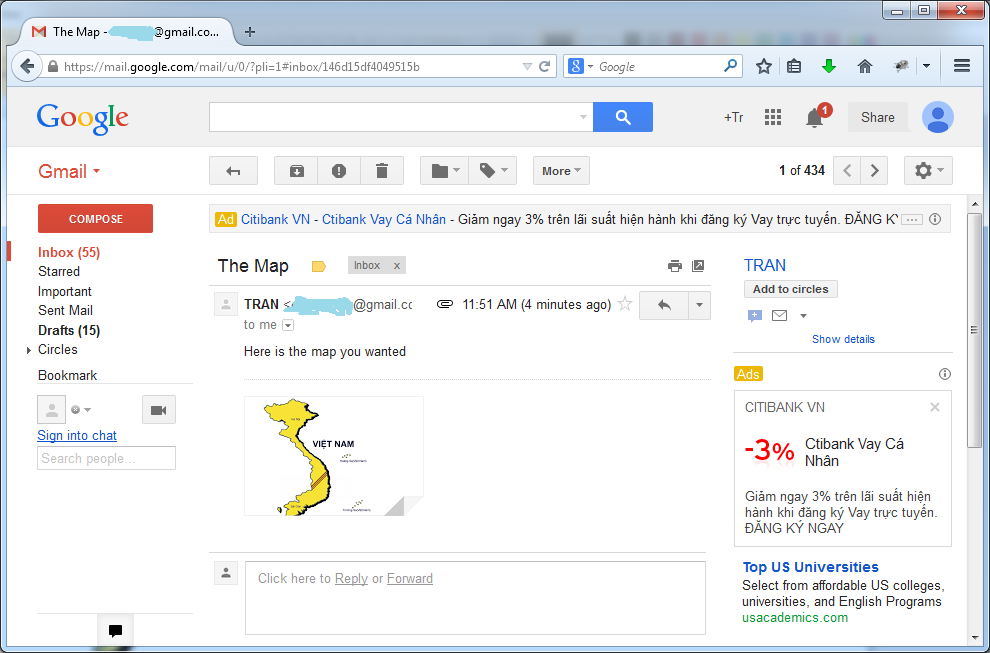
Ein anderes Beispiel: Ein Email schicken, in der ein Pfad aufs Internet beigefügt wird
EmailWithAttachment2.java
package org.o7planning.tutorial.commonsemail;
import java.net.URL;
import org.apache.commons.mail.DefaultAuthenticator;
import org.apache.commons.mail.EmailAttachment;
import org.apache.commons.mail.MultiPartEmail;
public class EmailWithAttachment2 {
public static void main(String[] args) {
try {
// Create the attachment
EmailAttachment attachment = new EmailAttachment();
attachment.setURL(new URL(
"http://www.apache.org/images/asf_logo_wide.gif"));
attachment.setDisposition(EmailAttachment.ATTACHMENT);
attachment.setDescription("Apache logo");
attachment.setName("Apache logo");
// Create the email message
MultiPartEmail email = new MultiPartEmail();
// Configuration
email.setHostName("smtp.googlemail.com");
email.setSmtpPort(465);
email.setSSLOnConnect(true);
email.setAuthenticator(new DefaultAuthenticator(
Constants.MY_EMAIL, Constants.MY_PASSWORD));
email.setFrom(Constants.MY_EMAIL, "TRAN");
email.addTo(Constants.FRIEND_EMAIL, "Hong");
email.setSubject("The logo");
email.setMsg("Here is Apache's logo");
// Add the attachment
email.attach(attachment);
// Send the email
email.send();
System.out.println("Sent!");
} catch (Exception e) {
e.printStackTrace();
}
}
}
Das Ergebnis vom Laufen des Beispiel
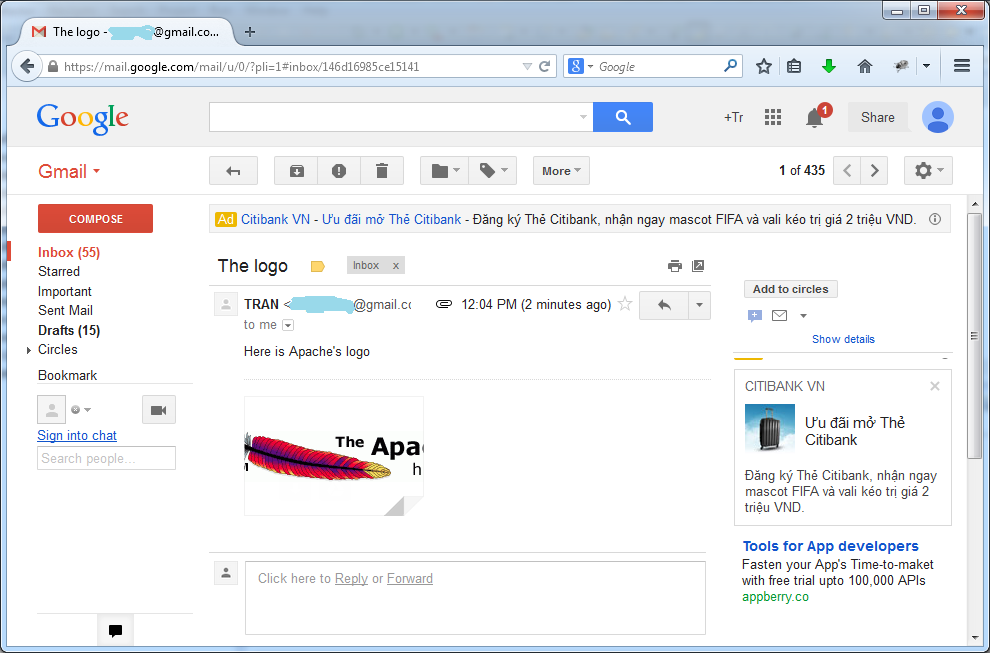
6. Das Email in der HTML Format schicken
SendHtmlEmail.java
package org.o7planning.tutorial.commonsemail;
import java.net.URL;
import org.apache.commons.mail.DefaultAuthenticator;
import org.apache.commons.mail.HtmlEmail;
public class SendHtmlEmail {
public static void main(String[] args) {
try {
// Create the email message
HtmlEmail email = new HtmlEmail();
// Configuration
email.setHostName("smtp.googlemail.com");
email.setSmtpPort(465);
email.setAuthenticator(new DefaultAuthenticator(
Constants.MY_EMAIL,Constants.MY_PASSWORD));
email.setSSLOnConnect(true);
email.setFrom(Constants.MY_EMAIL, "TRAN");
// Receiver
email.addTo(Constants.FRIEND_EMAIL);
// Title
email.setSubject("Test Sending HTML formatted email");
// Embed the image and get the content id
URL url = new URL("http://www.apache.org/images/asf_logo_wide.gif");
String cid = email.embed(url, "Apache logo");
// Set the html message
email.setHtmlMsg("<html><h2>The apache logo</h2> <img src=\"cid:"
+ cid + "\"></html>");
// Set the alternative message
email.setTextMsg("Your email client does not support HTML messages");
// Send email
email.send();
System.out.println("Sent!!");
} catch (Exception e) {
e.printStackTrace();
}
}
}
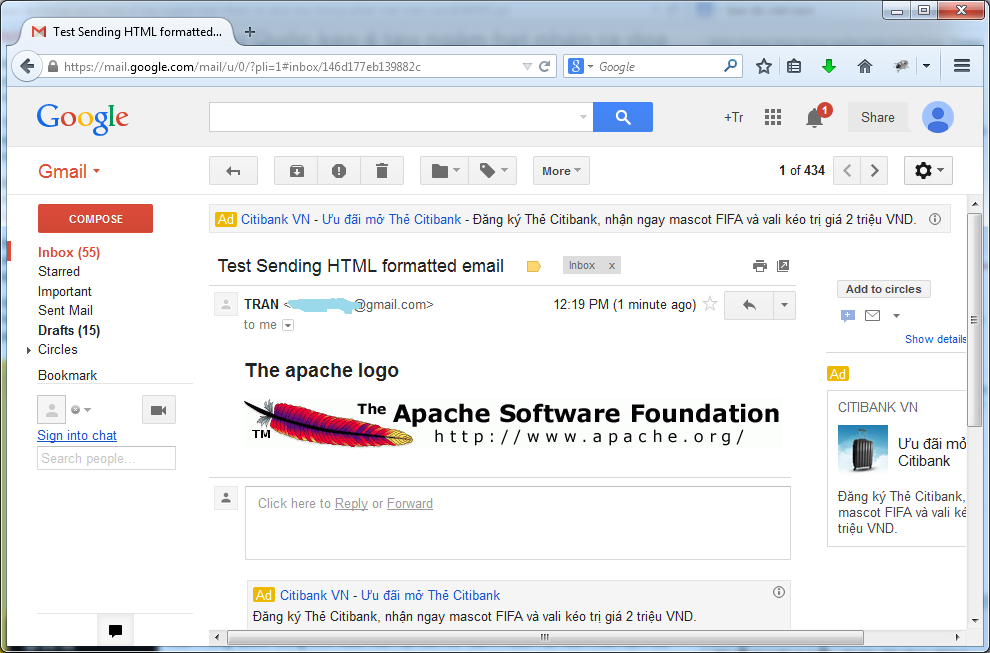
7. Ein Email in der HTML Format mit dem eingebettenen Foto schicken
SendHtmlEmbedImageEmail.java
package org.o7planning.tutorial.commonsemail;
import java.net.URL;
import org.apache.commons.mail.DefaultAuthenticator;
import org.apache.commons.mail.ImageHtmlEmail;
import org.apache.commons.mail.resolver.DataSourceUrlResolver;
public class SendHtmlEmbedImageEmail {
public static void main(String[] args) {
try {
// Load your HTML email template
// Here, Img has relative location (**)
String htmlEmailTemplate = "<h2>Hello!</h2>"
+"This is Apache Logo <br/>"
+"<img src='proper/commons-email/images/commons-logo.png'/>";
// Create the email message
ImageHtmlEmail email = new ImageHtmlEmail();
// Configuration
email.setHostName("smtp.googlemail.com");
email.setSmtpPort(465);
email.setAuthenticator(new DefaultAuthenticator(
Constants.MY_EMAIL, Constants.MY_PASSWORD));
email.setSSLOnConnect(true);
email.setFrom(Constants.MY_EMAIL, "TRAN");
email.addTo(Constants.FRIEND_EMAIL);
email.setSubject("Sending HTML formatted email with embedded images");
// Define you base URL to resolve relative resource locations
// (Example - Img you see above ** )
URL url = new URL("http://commons.apache.org");
email.setDataSourceResolver(new DataSourceUrlResolver(url) );
// Set the html message
email.setHtmlMsg(htmlEmailTemplate);
// Set the alternative message
email.setTextMsg("Your email client does not support HTML messages");
// Send the email
email.send();
System.out.println("Sent!!");
} catch (Exception e) {
e.printStackTrace();
}
}
}
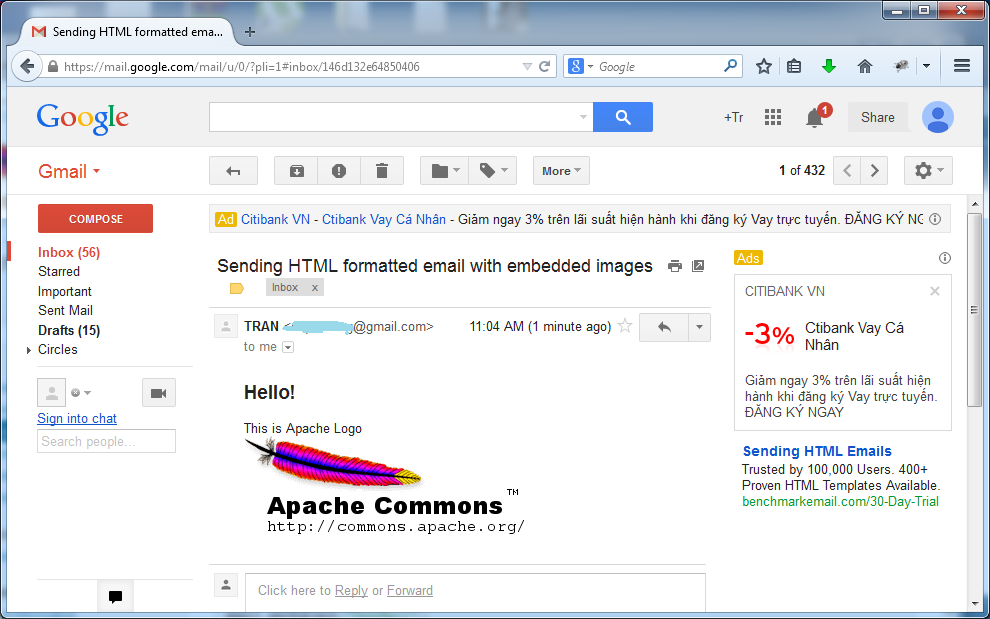
8. Der Anhang: Die Bibliothek Commons Email herunterladen
Wenn Sie Commons Email in einem normalen Projekt benutzen, sollen Sie die notwendigen Bibliotheke herunterladen.Commons Email basiert auf Email API so sollen Sie 2 Bibliotheke.
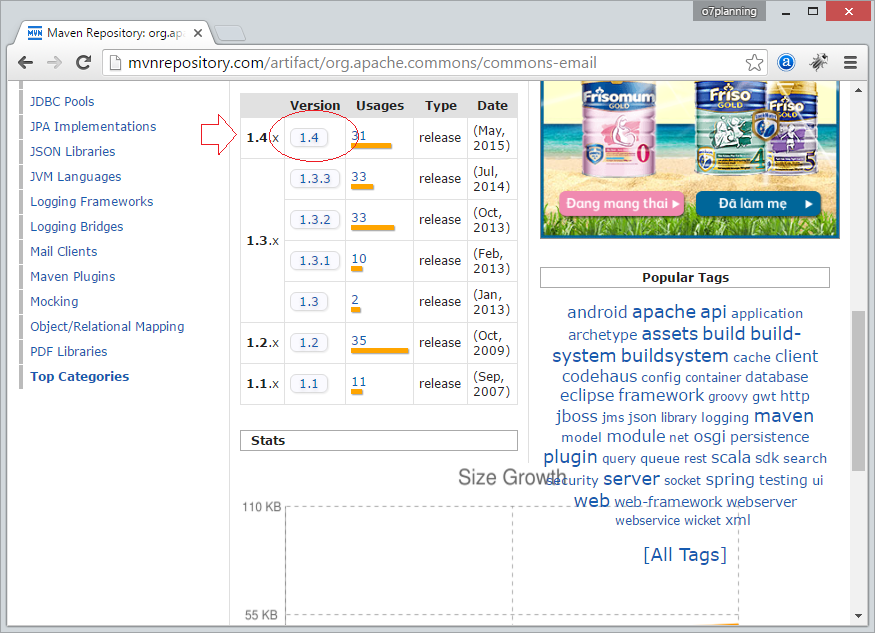
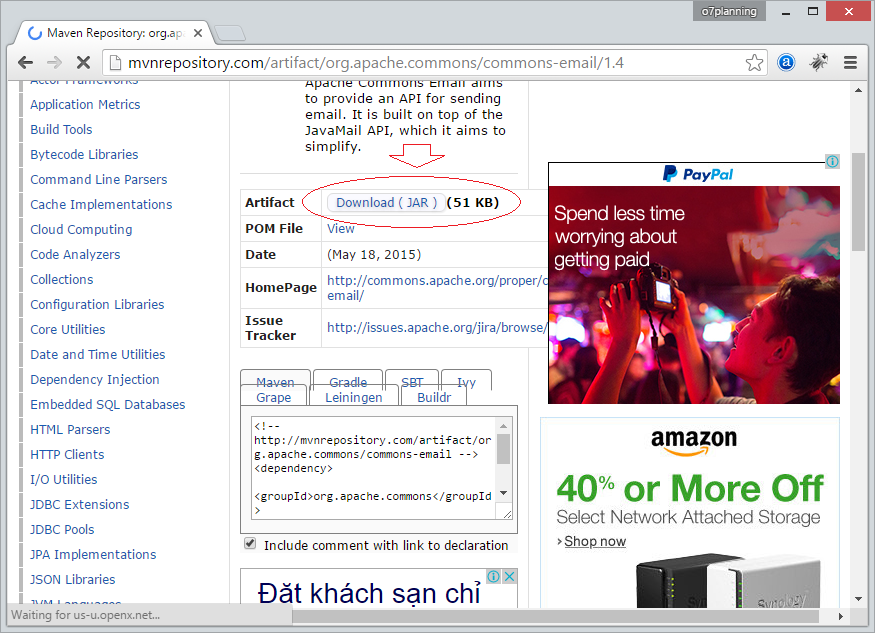
Nächste laden Sie die Bibliothek Email API:herunter
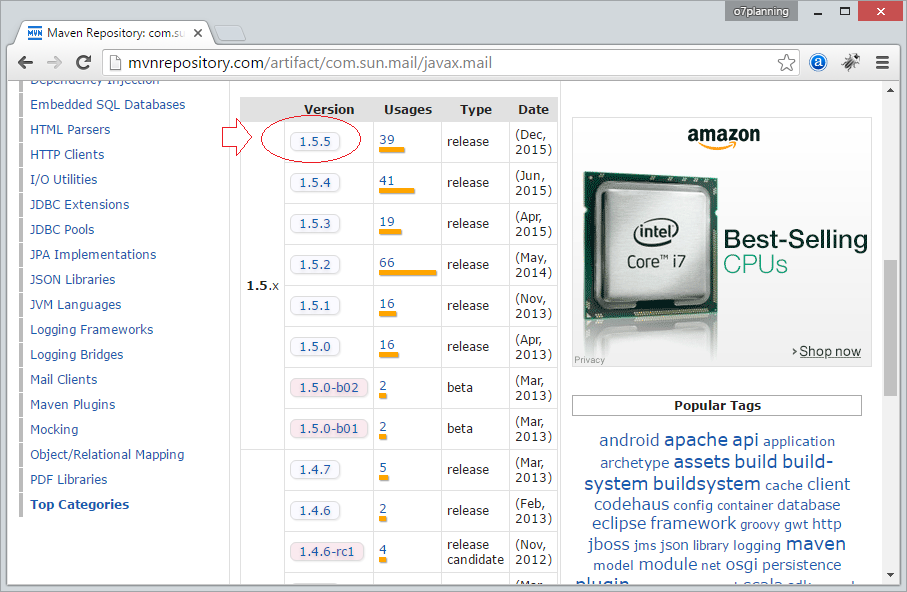
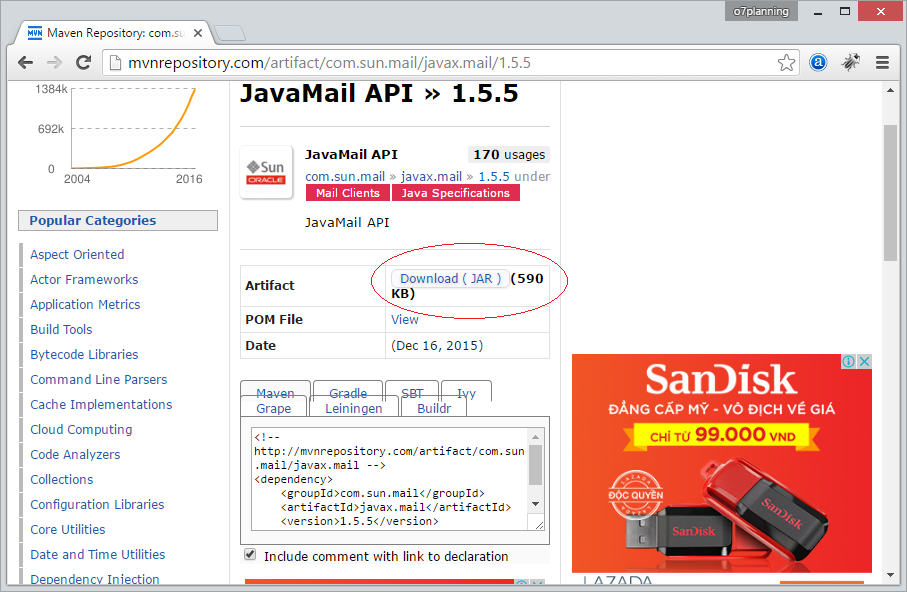
In den letzten laden Sie 2 File jar herunter
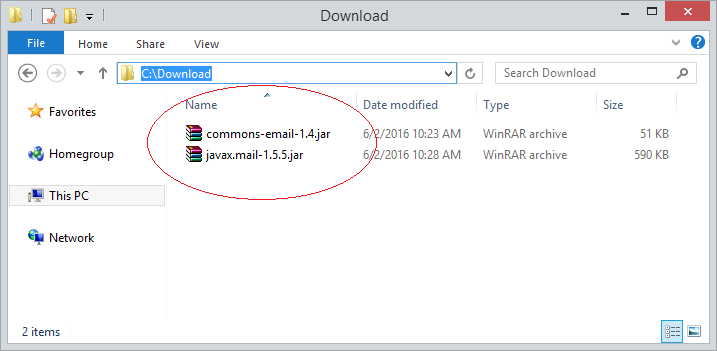
Java Grundlagen
- Anpassen von Java-Compiler, der Ihre Annotation verarbeitet (Annotation Processing Tool)
- Java Programmierung für Team mit Eclipse und SVN
- Die Anleitung zu Java WeakReference
- Die Anleitung zu Java PhantomReference
- Komprimierung und Dekomprimierung in Java
- Konfigurieren von Eclipse zur Verwendung des JDK anstelle von JRE
- Java-Methoden String.format() und printf()
- Syntax und neue Funktionen in Java 8
- Die Anleitung zu Java Reguläre Ausdrücke
- Die Anleitung zu Java Multithreading Programming
- JDBC Driver Bibliotheken für verschiedene Arten von Datenbank in Java
- Die Anleitung zu Java JDBC
- Holen Sie sich die automatisch erhöhenden Wert der Spalte bei dem Insert eines Rekord, der JDBC benutzt
- Die Anleitung zu Java Stream
- Die Anleitung zu Java Functional Interface
- Einführung in Raspberry Pi
- Die Anleitung zu Java Predicate
- Abstrakte Klasse und Interface in Java
- Zugriffsmodifikatoren (Access modifiers) in Java
- Die Anleitung zu Java Enum
- Die Anleitung zu Java Annotation
- Vergleichen und Sortieren in Java
- Die Anleitung zu Java String, StringBuffer und StringBuilder
- Die Anleitung zu Java Exception
- Die Anleitung zu Java Generics
- Manipulieren von Dateien und Verzeichnissen in Java
- Die Anleitung zu Java BiPredicate
- Die Anleitung zu Java Consumer
- Die Anleitung zu Java BiConsumer
- Was ist erforderlich, um mit Java zu beginnen?
- Geschichte von Java und der Unterschied zwischen Oracle JDK und OpenJDK
- Installieren Sie Java unter Windows
- Installieren Sie Java unter Ubuntu
- Installieren Sie OpenJDK unter Ubuntu
- Installieren Sie Eclipse
- Installieren Sie Eclipse unter Ubuntu
- Schnelle lernen Java für Anfänger
- Geschichte von Bits und Bytes in der Informatik
- Datentypen in Java
- Bitweise Operationen
- if else Anweisung in Java
- Switch Anweisung in Java
- Schleifen in Java
- Die Anleitung zu Java Array
- JDK Javadoc im CHM-Format
- Vererbung und Polymorphismus in Java
- Die Anleitung zu Java Function
- Die Anleitung zu Java BiFunction
- Beispiel für Java Encoding und Decoding mit Apache Base64
- Die Anleitung zu Java Reflection
- Java-Remote-Methodenaufruf - Java RMI
- Die Anleitung zu Java Socket
- Welche Plattform sollten Sie wählen für Applikationen Java Desktop entwickeln?
- Die Anleitung zu Java Commons IO
- Die Anleitung zu Java Commons Email
- Die Anleitung zu Java Commons Logging
- Java System.identityHashCode, Object.hashCode und Object.equals verstehen
- Die Anleitung zu Java SoftReference
- Die Anleitung zu Java Supplier
- Java Aspect Oriented Programming mit AspectJ (AOP)
Show More
- Anleitungen Java Servlet/JSP
- Die Anleitungen Java Collections Framework
- Java API für HTML & XML
- Die Anleitungen Java IO
- Die Anleitungen Java Date Time
- Anleitungen Spring Boot
- Anleitungen Maven
- Anleitungen Gradle
- Anleitungen Java Web Services
- Anleitungen Java SWT
- Die Anleitungen JavaFX
- Die Anleitungen Oracle Java ADF
- Die Anleitungen Struts2 Framework
- Anleitungen Spring Cloud