Verwenden Sie Twitter Bootstrap im Spring Boot
1. Das Zweck des Artikel
Vor dem Lernen sollen Sie zuerst Bootstrap kennenlernen :
Bootstrap ist ein HTML, CSS & JavaScript Framework erlaubt bei der Entwurf und Entwicklung der Applikation "Responsive Web Mobile". Bootstrap fasst HTML templates, CSS templates & Javascript und die grundlegenden vorhandenen Dinge wie: typography, forms, buttons, tables, navigation, modals, image carousels und die sonstigen Dingen um. Im Bootstrap gibt es Javascript Plugin, damit Ihr Design von Web Reponsive mehr einfach und leichter ist.
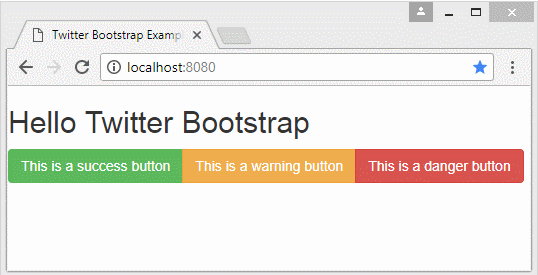
Bootstrap wird durch Mark Otto und Jacob Thornton in Twitter entwickelt. Es wird wie eine Open-Source-Code am August 2011 auf GitHub veröffentlicht.
Mehr sehen
Im Artikel leite ich Sie bei der Erstellung einer Applikation Spring Boot mit der Verwendung von Bootstrap. Und weil Bootstrap ein Html, Css, Javascript Framework ist, können Sie deshalb irgendeine Technologie für View Layer (JSP, Thymeleaf, Freemarker,...).
Die Inhalt werden im Artikel gemeint:
- Eine Applikation von Spring Boot erstellen.
- Die notwendigen Bibliotheken deklarieren damit Sie Bootstrap verwenden können.
- Eine einfache Seite mit der Verwendung von UI Component durch Bootstrap erstellen.
3. pom.xml konfigurieren
Bootstrap wurde in einer kleinen und schönen File Jar eingepackt. Um es zu benutzen, können Sie es in pom.xml deklarieren und deshalb können Sie bereit mit Bootstrap Framework arbeiten.
** Bootstrap **
<!-- https://mvnrepository.com/artifact/org.webjars/jquery -->
<dependency>
<groupId>org.webjars</groupId>
<artifactId>jquery</artifactId>
<version>3.3.1-1</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.webjars/popper.js -->
<dependency>
<groupId>org.webjars</groupId>
<artifactId>popper.js</artifactId>
<version>1.14.1</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.webjars/bootstrap -->
<dependency>
<groupId>org.webjars</groupId>
<artifactId>bootstrap</artifactId>
<version>4.1.1</version>
</dependency>
Die vollen Inhalt von der File pom.xml:
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>SpringBootBootstrap</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>SpringBootBootstrap</name>
<description>Spring Boot + Bootstrap</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.0.RELEASE</version>
<relativePath /> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<!-- https://mvnrepository.com/artifact/org.webjars/jquery -->
<dependency>
<groupId>org.webjars</groupId>
<artifactId>jquery</artifactId>
<version>3.3.1-1</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.webjars/popper.js -->
<dependency>
<groupId>org.webjars</groupId>
<artifactId>popper.js</artifactId>
<version>1.14.1</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.webjars/bootstrap -->
<dependency>
<groupId>org.webjars</groupId>
<artifactId>bootstrap</artifactId>
<version>4.1.1</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
4. Resource Handler
Die Datenquelle vom Bootstrap wird in die File Jar eingepackt. Sie sollen sie aussetzen (expose) damit sie durch den Pfad zugriffen werden. Zum Beispiel:
- http://somedomain/SomeContextPath/jquery/jquery.min.css
- http://somedomain/SomeContextPath/popper/popper.min.js
- http://somedomain/SomeContextPath/bootstrap/css/bootstrap.min.css
- http://somedomain/SomeContextPath/bootstrap/js/bootstrap.min.js
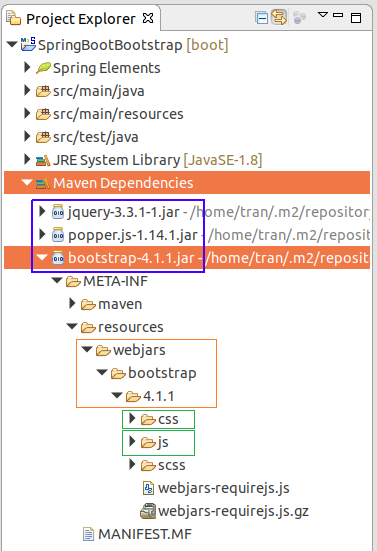
WebMvcConfig.java
package org.o7planning.sbbootstrap.config;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.ResourceHandlerRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class WebMvcConfig implements WebMvcConfigurer {
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
//
// Access Bootstrap static resource:
//
//
// http://somedomain/SomeContextPath/jquery/jquery.min.css
//
registry.addResourceHandler("/jquery/**") //
.addResourceLocations("classpath:/META-INF/resources/webjars/jquery/3.3.1-1/");
//
// http://somedomain/SomeContextPath/popper/popper.min.js
//
registry.addResourceHandler("/popper/**") //
.addResourceLocations("classpath:/META-INF/resources/webjars/popper.js/1.14.1/umd/");
// http://somedomain/SomeContextPath/bootstrap/css/bootstrap.min.css
// http://somedomain/SomeContextPath/bootstrap/js/bootstrap.min.js
//
registry.addResourceHandler("/bootstrap/**") //
.addResourceLocations("classpath:/META-INF/resources/webjars/bootstrap/4.1.1/");
}
}
5. Controller
HelloWorldController.java
package org.o7planning.sbbootstrap.controller;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class HelloWorldController {
@RequestMapping("/")
public String helloWorld(Model model) {
return "index";
}
}
6. Views
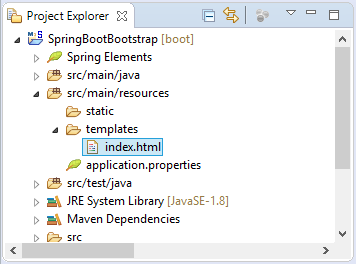
index.html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8"/>
<title>Twitter Bootstrap Example</title>
<meta name="viewport" content="width=device-width, initial-scale=1.0"/>
<!-- See configuration in WebMvConfig.java -->
<link th:href="@{/bootstrap/css/bootstrap.min.css}"
rel="stylesheet" media="screen"/>
<script th:src="@{/jquery/jquery.min.js}"></script>
<script th:src="@{/popper/popper.min.js}"></script>
<script th:src="@{/bootstrap/js/bootstrap.min.js}"></script>
</head>
<body>
<h2>Hello Twitter Bootstrap</h2>
<div class="btn-group">
<button type="button" class="btn btn-success">This is a success button</button>
<button type="button" class="btn btn-warning">This is a warning button</button>
<button type="button" class="btn btn-danger">This is a danger button</button>
</div>
</body>
</html>
Und hier ist die Image von Webseit und UI Component vom Bootstrap:
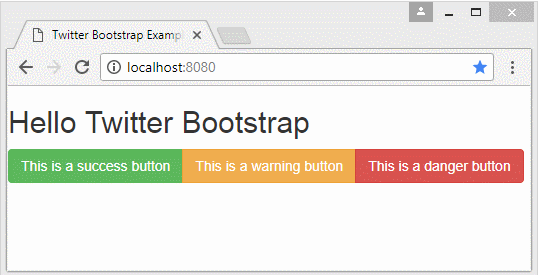
Anleitungen Spring Boot
- Installieren Sie die Spring Tool Suite für Eclipse
- Die Anleitung zum Sping für den Anfänger
- Die Anleitung zum Spring Boot für den Anfänger
- Gemeinsame Eigenschaften von Spring Boot
- Die Anleitung zu Spring Boot und Thymeleaf
- Die Anleitung zu Spring Boot und FreeMarker
- Die Anleitung zu Spring Boot und Groovy
- Die Anleitung zu Spring Boot und Mustache
- Die Anleitung zu Spring Boot und JSP
- Die Anleitung zu Spring Boot, Apache Tiles, JSP
- Verwenden Sie Logging im Spring Boot
- Anwendungsüberwachung mit Spring Boot Actuator
- Erstellen Sie eine mehrsprachige Webanwendung mit Spring Boot
- Verwenden Sie im Spring Boot mehrere ViewResolver
- Verwenden Sie Twitter Bootstrap im Spring Boot
- Die Anleitung zu Spring Boot Interceptor
- Die Anleitung zu Spring Boot, Spring JDBC und Spring Transaction
- Die Anleitung zu Spring JDBC
- Die Anleitung zu Spring Boot, JPA und Spring Transaction
- Die Anleitung zu Spring Boot und Spring Data JPA
- Die Anleitung zu Spring Boot, Hibernate und Spring Transaction
- Spring Boot, JPA und H2-Datenbank integrieren
- Die Anleitung zu Spring Boot und MongoDB
- Verwenden Sie mehrere DataSource mit Spring Boot und JPA
- Verwenden Sie mehrere DataSource mit Spring Boot und RoutingDataSource
- Erstellen Sie eine Login-Anwendung mit Spring Boot, Spring Security, Spring JDBC
- Erstellen Sie eine Login-Anwendung mit Spring Boot, Spring Security, JPA
- Erstellen Sie eine Benutzerregistrierungsanwendung mit Spring Boot, Spring Form Validation
- Beispiel für OAuth2 Social Login im Spring Boot
- Führen Sie geplante Hintergrundaufgaben in Spring aus
- CRUD Restful Web Service Beispiel mit Spring Boot
- Beispiel Spring Boot Restful Client mit RestTemplate
- CRUD-Beispiel mit Spring Boot, REST und AngularJS
- Sichere Spring Boot RESTful Service mit Basic Authentication
- Sicherer Spring Boot RESTful Service mit Auth0 JWT
- Beispiel Upload file mit Spring Boot
- Beispiel Download File mit Spring Boot
- Das Beispiel: Spring Boot File Upload mit jQuery Ajax
- Das Beispiel File Upload mit Spring Boot und AngularJS
- Erstellen Sie eine Warenkorb-Webanwendung mit Spring Boot, Hibernate
- Die Anleitung zu Spring Email
- Erstellen Sie eine einfache Chat-Anwendung mit Spring Boot und Websocket
- Stellen Sie die Spring Boot-Anwendung auf Tomcat Server bereit
- Stellen Sie die Spring Boot-Anwendung auf Oracle WebLogic Server bereit
- Installieren Sie ein kostenloses Let's Encrypt SSL-Zertifikat für Spring Boot
- Konfigurieren Sie Spring Boot so, dass HTTP zu HTTPS umgeleitet wird
Show More