Das Beispiel: Spring Boot File Upload mit jQuery Ajax
1. Das Zweck der Unterricht
In der Unterricht werde ich eine Applikation Spring Boot upload file mit jQuery Ajax erstellen. Unten ist das die Vorschau der Applikation, die wir implementieren werden
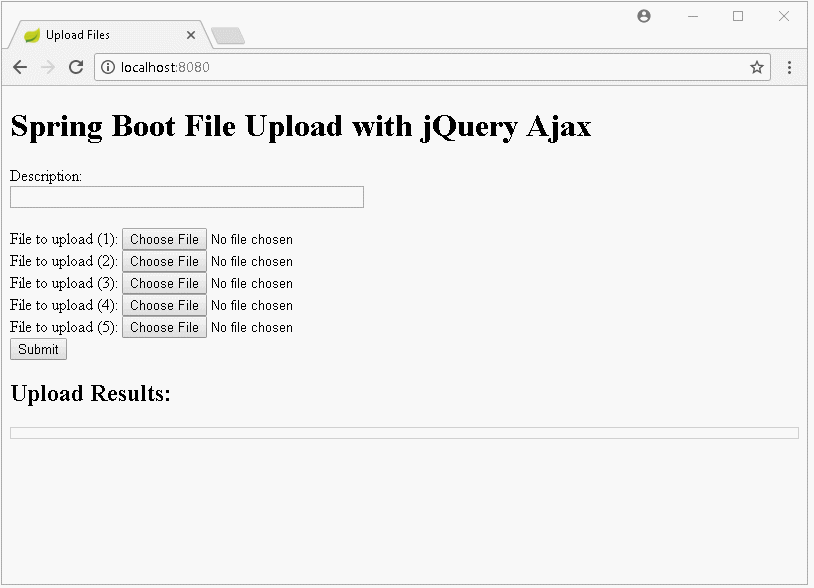
Das ist der Fehleranmeldung
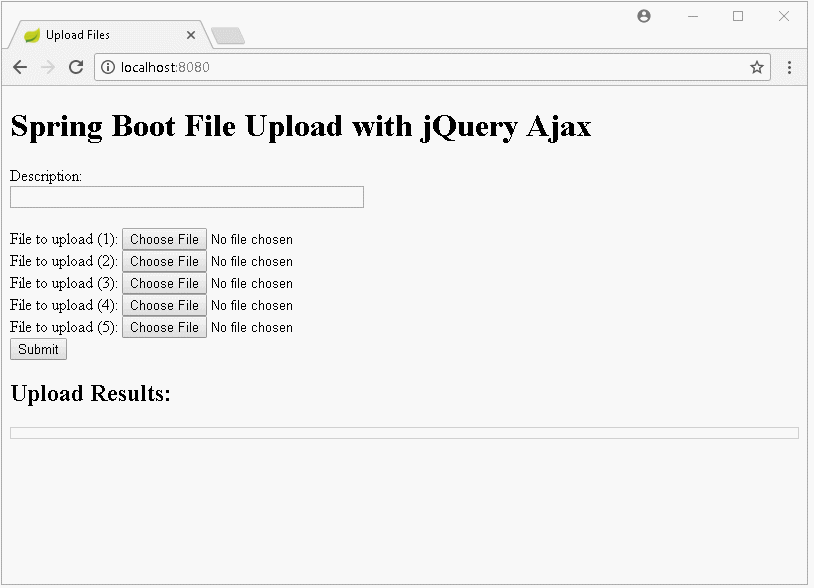
Sehen Sie mehr das Beispiel vom Upload file mit Spring Boot ohne die Verwendung von AJAX:File Upload + AngularJS:
2. Das Projekt Spring Boot erstellen
Auf Eclipse erstellen Sie das Projekt Spring Boot:
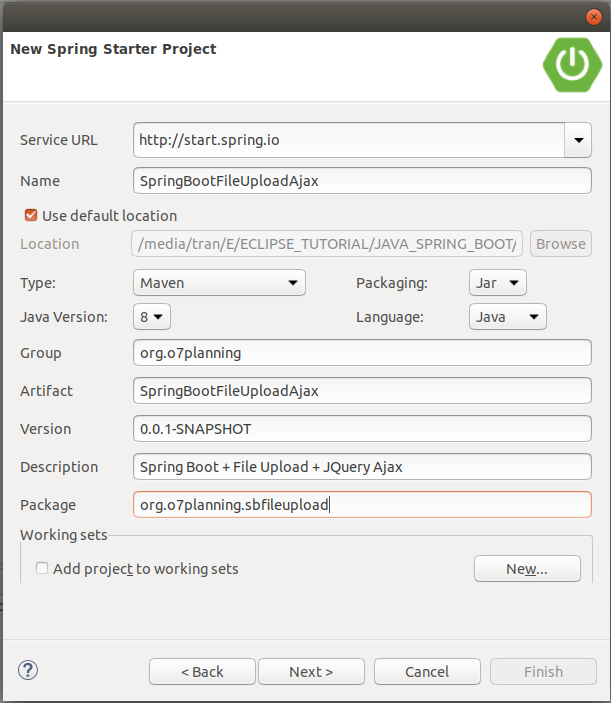
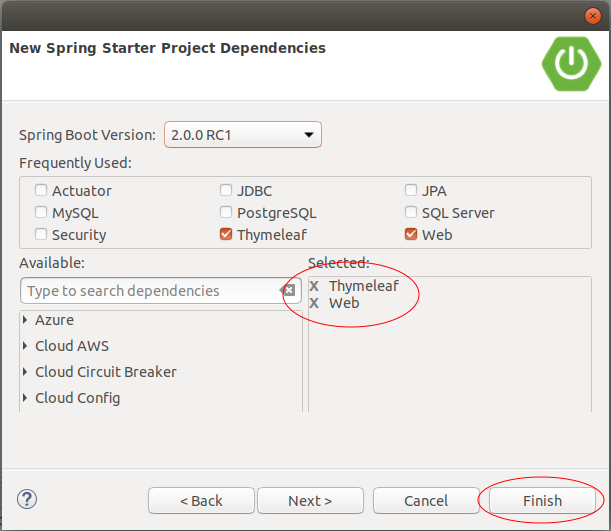
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>SpringBootFileUploadAjax</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>SpringBootFileUploadAjax</name>
<description>Spring Boot + File Upload + JQuery Ajax</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.0.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
SpringBootFileUploadAjaxApplication.java
package org.o7planning.sbfileupload;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class SpringBootFileUploadAjaxApplication {
public static void main(String[] args) {
SpringApplication.run(SpringBootFileUploadAjaxApplication.class, args);
}
}
3. Form, Controller, Exception Handler
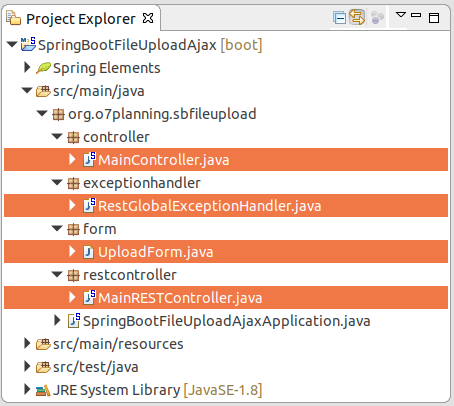
Die Klasse UploadForm vertritt die HTML Form Daten
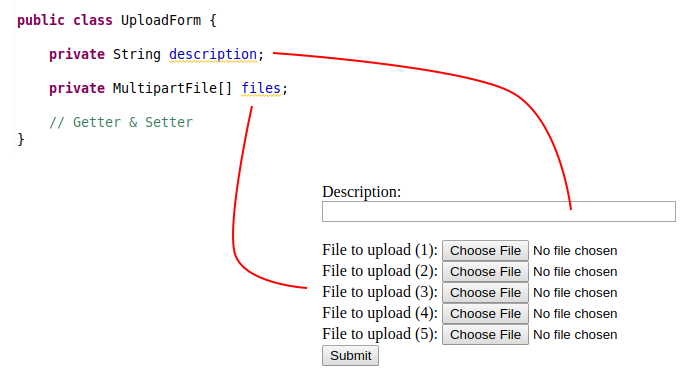
UploadForm.java
package org.o7planning.sbfileupload.form;
import org.springframework.web.multipart.MultipartFile;
public class UploadForm {
private String description;
private MultipartFile[] files;
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public MultipartFile[] getFiles() {
return files;
}
public void setFiles(MultipartFile[] files) {
this.files = files;
}
}
MainController.java
package org.o7planning.sbfileupload.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
@Controller
public class MainController {
@GetMapping("/")
public String index() {
return "upload";
}
}
Die Klasse MainRESTController definiert ein REST API um die Daten der vom Benutzer hochgeladeten File zu behandeln. REST API wird durch jQuery Ajax aufgeruft (in main.js sehen).
MainRESTController.java
package org.o7planning.sbfileupload.restcontroller;
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import org.o7planning.sbfileupload.form.UploadForm;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
@RestController
public class MainRESTController {
// Linux: /home/{user}/test
// Windows: C:/Users/{user}/test
private static String UPLOAD_DIR = System.getProperty("user.home") + "/test";
@PostMapping("/rest/uploadMultiFiles")
public ResponseEntity<?> multiUploadFileModel(@ModelAttribute UploadForm form) {
System.out.println("Description:" + form.getDescription());
String result = null;
try {
result = this.saveUploadedFiles(form.getFiles());
}
// Here Catch IOException only.
// Other Exceptions catch by RestGlobalExceptionHandler class.
catch (IOException e) {
e.printStackTrace();
return new ResponseEntity<>("Error: " + e.getMessage(), HttpStatus.BAD_REQUEST);
}
return new ResponseEntity<String>("Uploaded to: <br/>" + result, HttpStatus.OK);
}
// Save Files
private String saveUploadedFiles(MultipartFile[] files) throws IOException {
// Make sure directory exists!
File uploadDir = new File(UPLOAD_DIR);
uploadDir.mkdirs();
StringBuilder sb = new StringBuilder();
for (MultipartFile file : files) {
if (file.isEmpty()) {
continue;
}
String uploadFilePath = UPLOAD_DIR + "/" + file.getOriginalFilename();
byte[] bytes = file.getBytes();
Path path = Paths.get(uploadFilePath);
Files.write(path, bytes);
sb.append(uploadFilePath).append("<br/>");
}
return sb.toString();
}
}
Nach dem Default ist die Größe die auf dem Server hochgeladete File nicht höher als 1MB. Und wenn der Benutzer vielen Files gleichzeitig hochladen, wird die gesamten Größe der Files höher nicht höher als 1 MB. Allerdings können Sie konfigurieren um die Parameter zu ändern.
application.properties
spring.servlet.multipart.max-file-size=1MB
spring.servlet.multipart.max-request-size=5MB
spring.thymeleaf.cache=false
RestGlobalExceptionHandler ist eine benutzerdefinierte Klasse, aus der Klasse ResponseEntityExceptionHandler verlängert. In dieser Klasse können Sie die aus der Methode REST geworfenen Ausnahmen behandeln. Das hilft Ihnen bei der Behandlung der Ausnahmen in einer Position statt in jeder Methode REST.
RestGlobalExceptionHandler.java
package org.o7planning.sbfileupload.exceptionhandler;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.multipart.MultipartException;
import org.springframework.web.servlet.mvc.method.annotation.ResponseEntityExceptionHandler;
import javax.servlet.http.HttpServletRequest;
@ControllerAdvice
public class RestGlobalExceptionHandler extends ResponseEntityExceptionHandler {
// Catch max file size Exception.
@ExceptionHandler(MultipartException.class)
@ResponseBody
public ResponseEntity<?> handleControllerException(HttpServletRequest request, Throwable ex) {
HttpStatus status = this.getStatus(request);
return new ResponseEntity<String>("(Message in RestGlobalExceptionHandler *): " + ex.getMessage(), status);
}
// Cache Other Exception
@ExceptionHandler(Exception.class)
@ResponseBody
public ResponseEntity<?> handleControllerRootException(HttpServletRequest request, Throwable ex) {
HttpStatus status = this.getStatus(request);
return new ResponseEntity<String>("(Message in RestGlobalExceptionHandler **): " + ex.getMessage(), status);
}
private HttpStatus getStatus(HttpServletRequest request) {
Integer statusCode = (Integer) request.getAttribute("javax.servlet.error.status_code");
if (statusCode == null) {
return HttpStatus.INTERNAL_SERVER_ERROR;
}
return HttpStatus.valueOf(statusCode);
}
}
4. Javascript, View
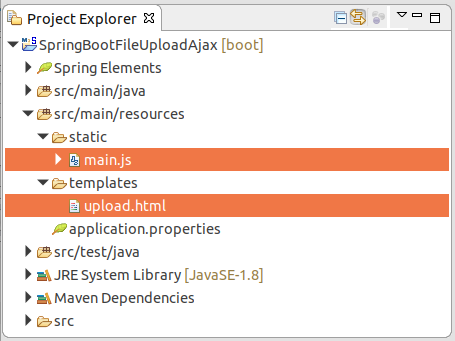
upload.html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Upload Files</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<script type="text/javascript" th:src="@{/main.js}"></script>
</head>
<body>
<h1>Spring Boot File Upload with jQuery Ajax</h1>
<form method="POST" enctype="multipart/form-data" id="fileUploadForm">
Description: <br/>
<input type="text" name="description" style="width:350px;"/>
<br/><br/>
File to upload (1): <input type="file" name="files"/><br />
File to upload (2): <input type="file" name="files"/><br />
File to upload (3): <input type="file" name="files"/><br />
File to upload (4): <input type="file" name="files"/><br />
File to upload (5): <input type="file" name="files"/><br />
<input type="submit" value="Submit" id="submitButton"/>
</form>
<h2>Upload Results:</h2>
<div style="border:1px solid #ccc;padding: 5px;">
<span id="result"></span>
</div>
</body>
</html>
main.js
$(document).ready(function() {
$("#submitButton").click(function(event) {
// Stop default form Submit.
event.preventDefault();
// Call Ajax Submit.
ajaxSubmitForm();
});
});
function ajaxSubmitForm() {
// Get form
var form = $('#fileUploadForm')[0];
var data = new FormData(form);
$("#submitButton").prop("disabled", true);
$.ajax({
type: "POST",
enctype: 'multipart/form-data',
url: "/rest/uploadMultiFiles",
data: data,
// prevent jQuery from automatically transforming the data into a query string
processData: false,
contentType: false,
cache: false,
timeout: 1000000,
success: function(data, textStatus, jqXHR) {
$("#result").html(data);
console.log("SUCCESS : ", data);
$("#submitButton").prop("disabled", false);
$('#fileUploadForm')[0].reset();
},
error: function(jqXHR, textStatus, errorThrown) {
$("#result").html(jqXHR.responseText);
console.log("ERROR : ", jqXHR.responseText);
$("#submitButton").prop("disabled", false);
}
});
}
Anleitungen Spring Boot
- Installieren Sie die Spring Tool Suite für Eclipse
- Die Anleitung zum Sping für den Anfänger
- Die Anleitung zum Spring Boot für den Anfänger
- Gemeinsame Eigenschaften von Spring Boot
- Die Anleitung zu Spring Boot und Thymeleaf
- Die Anleitung zu Spring Boot und FreeMarker
- Die Anleitung zu Spring Boot und Groovy
- Die Anleitung zu Spring Boot und Mustache
- Die Anleitung zu Spring Boot und JSP
- Die Anleitung zu Spring Boot, Apache Tiles, JSP
- Verwenden Sie Logging im Spring Boot
- Anwendungsüberwachung mit Spring Boot Actuator
- Erstellen Sie eine mehrsprachige Webanwendung mit Spring Boot
- Verwenden Sie im Spring Boot mehrere ViewResolver
- Verwenden Sie Twitter Bootstrap im Spring Boot
- Die Anleitung zu Spring Boot Interceptor
- Die Anleitung zu Spring Boot, Spring JDBC und Spring Transaction
- Die Anleitung zu Spring JDBC
- Die Anleitung zu Spring Boot, JPA und Spring Transaction
- Die Anleitung zu Spring Boot und Spring Data JPA
- Die Anleitung zu Spring Boot, Hibernate und Spring Transaction
- Spring Boot, JPA und H2-Datenbank integrieren
- Die Anleitung zu Spring Boot und MongoDB
- Verwenden Sie mehrere DataSource mit Spring Boot und JPA
- Verwenden Sie mehrere DataSource mit Spring Boot und RoutingDataSource
- Erstellen Sie eine Login-Anwendung mit Spring Boot, Spring Security, Spring JDBC
- Erstellen Sie eine Login-Anwendung mit Spring Boot, Spring Security, JPA
- Erstellen Sie eine Benutzerregistrierungsanwendung mit Spring Boot, Spring Form Validation
- Beispiel für OAuth2 Social Login im Spring Boot
- Führen Sie geplante Hintergrundaufgaben in Spring aus
- CRUD Restful Web Service Beispiel mit Spring Boot
- Beispiel Spring Boot Restful Client mit RestTemplate
- CRUD-Beispiel mit Spring Boot, REST und AngularJS
- Sichere Spring Boot RESTful Service mit Basic Authentication
- Sicherer Spring Boot RESTful Service mit Auth0 JWT
- Beispiel Upload file mit Spring Boot
- Beispiel Download File mit Spring Boot
- Das Beispiel: Spring Boot File Upload mit jQuery Ajax
- Das Beispiel File Upload mit Spring Boot und AngularJS
- Erstellen Sie eine Warenkorb-Webanwendung mit Spring Boot, Hibernate
- Die Anleitung zu Spring Email
- Erstellen Sie eine einfache Chat-Anwendung mit Spring Boot und Websocket
- Stellen Sie die Spring Boot-Anwendung auf Tomcat Server bereit
- Stellen Sie die Spring Boot-Anwendung auf Oracle WebLogic Server bereit
- Installieren Sie ein kostenloses Let's Encrypt SSL-Zertifikat für Spring Boot
- Konfigurieren Sie Spring Boot so, dass HTTP zu HTTPS umgeleitet wird
Show More