CRUD Restful Web Service Beispiel mit Spring Boot
1. Das Zweck des Beispiel
Der Unterlagen wird nach ... geschrieben
Spring Boot 2.x
Eclipse 4.7 Oxygen
Mehr sehen
Im Unterlagen leite ich Sie bei der Erstellung einer Applikation Restful Web Service , die Spring Boot verwendet und 4 Funktionen Create, Read, Update, Delete (CRUD) hat.
Read (GET method)
We will build an URI that is assigned to return the user an employee list and defines another URI that returns the user the information of a particular employee. The data that the user will be received is in XML or JSON format. These URIs only accept the requests with GET method.
- GET http://localhost:8080/employees
- GET http://localhost:8080/employee/E01
Update (PUT method).
Build an URI to process the request for changing an employee's information. This URI accepts only the requests with PUT method. The data attached with the request is the new information of the employee, which is in XML or JSON format.
- PUT http://localhost:8080/employee
Create (POST method)
Ein URI bauen um die Anforderung (request) zur Erstellung eines Mitarbeiter (employee) zu behandeln. URI akzeptiert die Anforderung mit der Methode POST. Die Daten wird mit der Anforderung geschickt, dass die Information des erstellten Mitarbeiter in Format XML oder JSON ist.
- POST http://localhost:8080/employee
Delete (DELETE method).
Ein URI bauen um die Anforderung der Löschung eines Mitarbeiter zu behandeln. URI akzeptiert nur die Anforderung mit der Methode DELETE.
Achtung: Keine Daten werden in die Anforderung in diesem Fall beigefügt (Wie die beigefügten Daten in der Methode POST), denn die Anforderung (request) mit der Methode DELETE kann die Daten nicht beifügen. Die Information des Mitarbeiter zu löschen liegt in URI oder in QueryString vom URL.
- DELETE http://localhost:8080/employee/{empNo}
2. Spring Boot Projekt erstellen
Auf die Eclipse wählen Sie:
- File/New/Other..
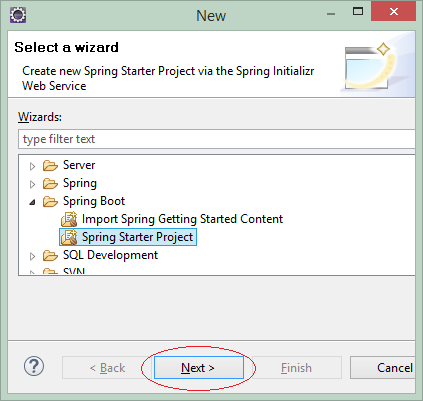
Geben Sie ein
- Name: SpringBootCrudRestful
- Group: org.o7planning
- Package: org.o7planning.sbcrudrestful
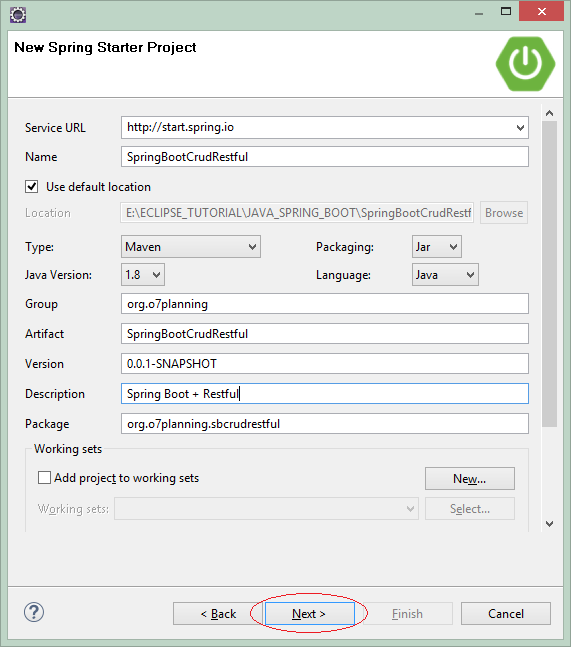
Zunächst sollen sie die Technologie zu verwenden wählen
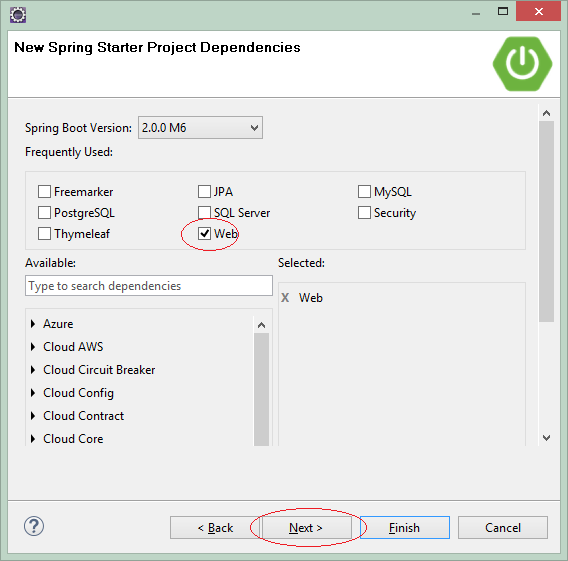
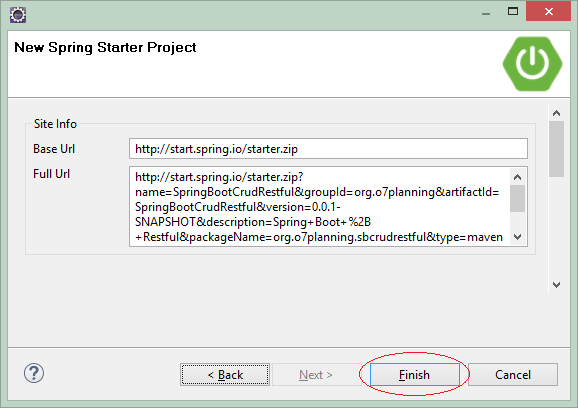
OK, Das Project wird erstellt
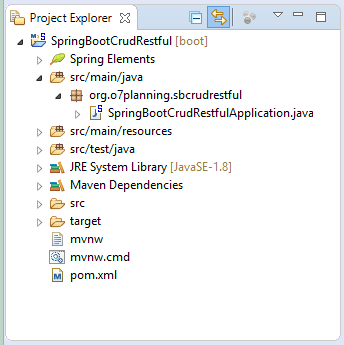
3. pom.xml konfigurieren
In diesem Beispiel brauchen Sie eine Bibliothek um XML zu Java umzuwandeln (convert) und umgekehrt und eine Bibliothek um JSON zu Java umzuwandeln und umgekehrt
JSON <==> Java
spring-boot-starter-web hat die Bibliothek jackson-databind schon integriert. Diese Bibliothek hilft JSON zu Java umzuwandeln und umgekehrt
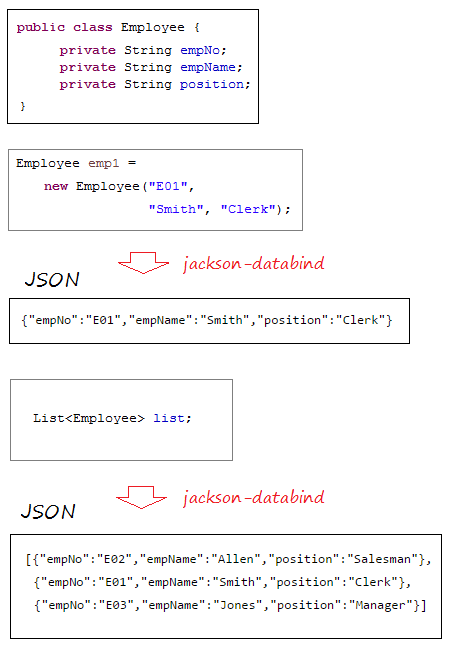
XML <==> Java
Spring Boot verwendet JAXB (in JDK verfügbar) als eine Standard-Bibliothek um XML und Java umzuwandeln. Allerdings brauchen die Klasse von Ihrem Java durch @XmlRootElement,... annotiert zu werden. Deshalb sollen Sie meiner Meinung nach jackson-dataformat-xml wie eine Bibliothek der Umwandlung XML und Java verwenden. Um jackson-dataformat-xml zu benutzen sollen Sie es in die File pom.xml deklarieren:
** pom.xml **
...
<dependencies>
...
<dependency>
<groupId>com.fasterxml.jackson.dataformat</groupId>
<artifactId>jackson-dataformat-xml</artifactId>
</dependency>
...
</dependencies>
...
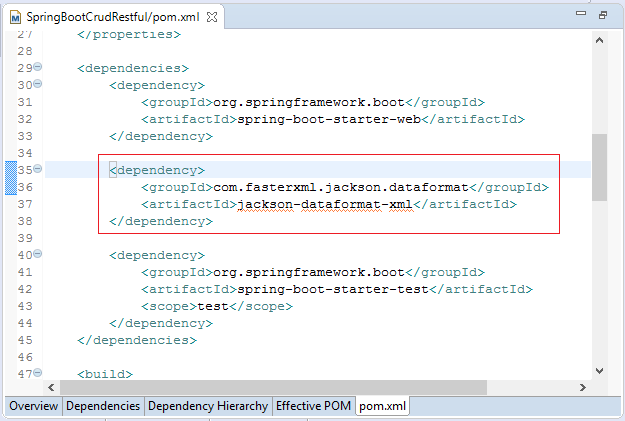
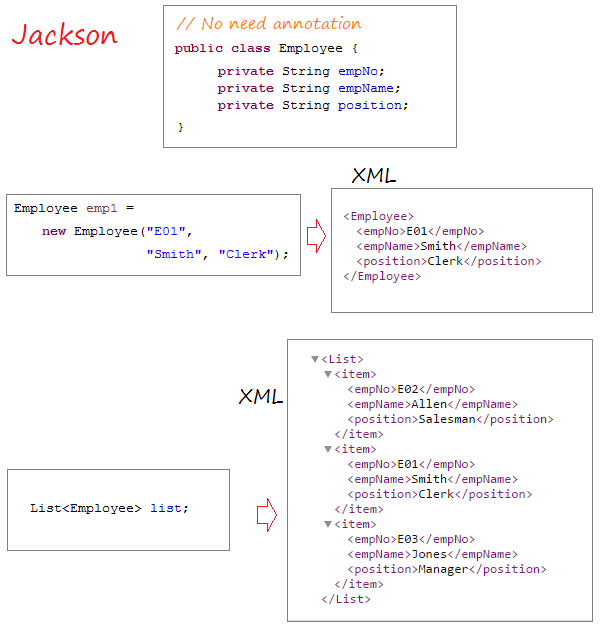
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>SpringBootCrudRestful</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>SpringBootCrudRestful</name>
<description>Spring Boot + Restful</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.0.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.dataformat</groupId>
<artifactId>jackson-dataformat-xml</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
4. Die Kode der Applikation
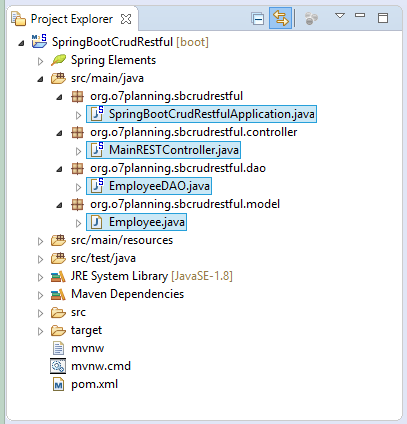
SpringBootCrudRestfulApplication.java
package org.o7planning.sbcrudrestful;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class SpringBootCrudRestfulApplication {
public static void main(String[] args) {
SpringApplication.run(SpringBootCrudRestfulApplication.class, args);
}
}
Die Klasse Employee vertritt einen Mitarbeiter
Employee.java
package org.o7planning.sbcrudrestful.model;
public class Employee {
private String empNo;
private String empName;
private String position;
public Employee() {
}
public Employee(String empNo, String empName, String position) {
this.empNo = empNo;
this.empName = empName;
this.position = position;
}
public String getEmpNo() {
return empNo;
}
public void setEmpNo(String empNo) {
this.empNo = empNo;
}
public String getEmpName() {
return empName;
}
public void setEmpName(String empName) {
this.empName = empName;
}
public String getPosition() {
return position;
}
public void setPosition(String position) {
this.position = position;
}
}
Die Klasse EmployeeDAO wird durch @Repository annotiert um mit Spring zu informieren, dass es Spring BEAN ist. Diese Klasse schließt die Methode zum Abfragen der Liste der Mitarbeiter (employee), der Erstellung des Mitarbeiter, der Änderung und der Löschung der Information ein.
EmployeeDAO.java
package org.o7planning.sbcrudrestful.dao;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.o7planning.sbcrudrestful.model.Employee;
import org.springframework.stereotype.Repository;
@Repository
public class EmployeeDAO {
private static final Map<String, Employee> empMap = new HashMap<String, Employee>();
static {
initEmps();
}
private static void initEmps() {
Employee emp1 = new Employee("E01", "Smith", "Clerk");
Employee emp2 = new Employee("E02", "Allen", "Salesman");
Employee emp3 = new Employee("E03", "Jones", "Manager");
empMap.put(emp1.getEmpNo(), emp1);
empMap.put(emp2.getEmpNo(), emp2);
empMap.put(emp3.getEmpNo(), emp3);
}
public Employee getEmployee(String empNo) {
return empMap.get(empNo);
}
public Employee addEmployee(Employee emp) {
empMap.put(emp.getEmpNo(), emp);
return emp;
}
public Employee updateEmployee(Employee emp) {
empMap.put(emp.getEmpNo(), emp);
return emp;
}
public void deleteEmployee(String empNo) {
empMap.remove(empNo);
}
public List<Employee> getAllEmployees() {
Collection<Employee> c = empMap.values();
List<Employee> list = new ArrayList<Employee>();
list.addAll(c);
return list;
}
}
Die Klasse MainRESTController wird durch @RestController annotiert um mit Spring zu informieren, dass es Spring Restful Controller ist,
MainRESTController.java
package org.o7planning.sbcrudrestful.controller;
import java.util.List;
import org.o7planning.sbcrudrestful.dao.EmployeeDAO;
import org.o7planning.sbcrudrestful.model.Employee;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.MediaType;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class MainRESTController {
@Autowired
private EmployeeDAO employeeDAO;
@RequestMapping("/")
@ResponseBody
public String welcome() {
return "Welcome to RestTemplate Example.";
}
// URL:
// http://localhost:8080/SomeContextPath/employees
// http://localhost:8080/SomeContextPath/employees.xml
// http://localhost:8080/SomeContextPath/employees.json
@RequestMapping(value = "/employees", //
method = RequestMethod.GET, //
produces = { MediaType.APPLICATION_JSON_VALUE, //
MediaType.APPLICATION_XML_VALUE })
@ResponseBody
public List<Employee> getEmployees() {
List<Employee> list = employeeDAO.getAllEmployees();
return list;
}
// URL:
// http://localhost:8080/SomeContextPath/employee/{empNo}
// http://localhost:8080/SomeContextPath/employee/{empNo}.xml
// http://localhost:8080/SomeContextPath/employee/{empNo}.json
@RequestMapping(value = "/employee/{empNo}", //
method = RequestMethod.GET, //
produces = { MediaType.APPLICATION_JSON_VALUE, //
MediaType.APPLICATION_XML_VALUE })
@ResponseBody
public Employee getEmployee(@PathVariable("empNo") String empNo) {
return employeeDAO.getEmployee(empNo);
}
// URL:
// http://localhost:8080/SomeContextPath/employee
// http://localhost:8080/SomeContextPath/employee.xml
// http://localhost:8080/SomeContextPath/employee.json
@RequestMapping(value = "/employee", //
method = RequestMethod.POST, //
produces = { MediaType.APPLICATION_JSON_VALUE, //
MediaType.APPLICATION_XML_VALUE })
@ResponseBody
public Employee addEmployee(@RequestBody Employee emp) {
System.out.println("(Service Side) Creating employee: " + emp.getEmpNo());
return employeeDAO.addEmployee(emp);
}
// URL:
// http://localhost:8080/SomeContextPath/employee
// http://localhost:8080/SomeContextPath/employee.xml
// http://localhost:8080/SomeContextPath/employee.json
@RequestMapping(value = "/employee", //
method = RequestMethod.PUT, //
produces = { MediaType.APPLICATION_JSON_VALUE, //
MediaType.APPLICATION_XML_VALUE })
@ResponseBody
public Employee updateEmployee(@RequestBody Employee emp) {
System.out.println("(Service Side) Editing employee: " + emp.getEmpNo());
return employeeDAO.updateEmployee(emp);
}
// URL:
// http://localhost:8080/SomeContextPath/employee/{empNo}
@RequestMapping(value = "/employee/{empNo}", //
method = RequestMethod.DELETE, //
produces = { MediaType.APPLICATION_JSON_VALUE, MediaType.APPLICATION_XML_VALUE })
@ResponseBody
public void deleteEmployee(@PathVariable("empNo") String empNo) {
System.out.println("(Service Side) Deleting employee: " + empNo);
employeeDAO.deleteEmployee(empNo);
}
}
Erklären:
- produces = { MediaType.APPLICATION_JSON_VALUE, MediaType.APPLICATION_XML_VALUE }
- produces = { "application/json" , "application/xml" }
Das Attribut produces wird verwendet um zu regeln, ein URL erstellt (den Benutzer zurückgeben) die Daten mit einer Format. Z.B "application/json", "application/xml".
5. Die Applikation durchführen
Um die Applikation durchzuführen, klicken Sie die Rechtmaustaste aufs Project und wählen:
- Run As/Spring Boot App
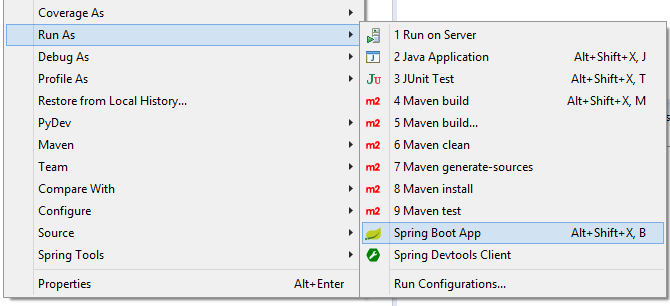
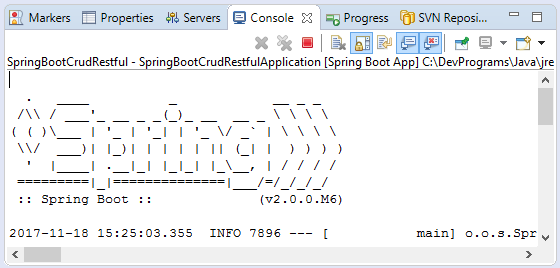
Nach der Durchführung können Sie ihre Applikation checken
Test GET:
If ERROR==> Please use Spring Boot 2.0.0.M5 temporarily.(And wait for the official version of Spring Boot 2).<parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.0.0.M5</version> <relativePath/> <!-- lookup parent from repository --> </parent>
Um die Liste der Mitarbeiter (employee) zu holen, brauch der Benutzer request (die Anforderung) mit der Methode GET schicken. Sie können durch die Verwendung des Browser diese Funktion checken
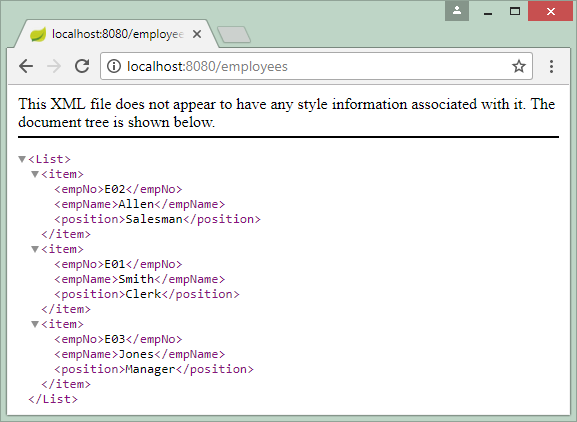
Oder
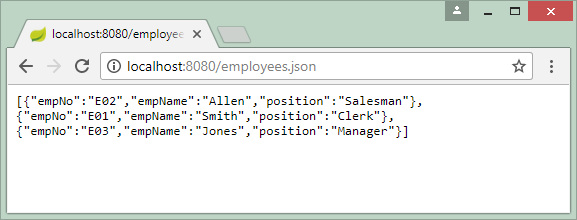
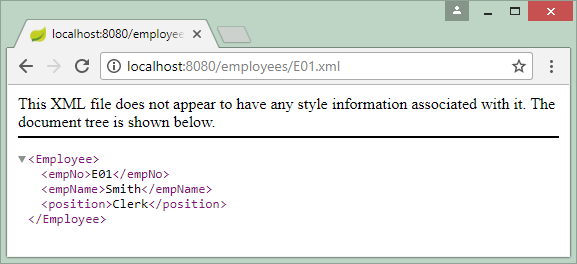
Wie erstellt man ein Request verwendend die Methode POST, PUT oder DELETE?Um Request (die Anforderung) mit der Methode POST, PUT oder DELETE zu erstellen, sollen Sie ein Tool wie RestClient, cURL,..verwenden oder eine Applikation Rest Client von Ihnen schreibenMehr sehen
Test POST
Um einen Mitarbeiter (employee) zu erstellen, sollen Sie eine Anforderung (request) mit der Methode POST erstellen, und die Information des Mitarbeiter mitschicken. Die geschickten Daten liegt in die Format JSON oder XML:
POST http://localhost:8080/employee
Acept: application/xml
<Employee>
<empNo>E11</empNo>
<empName>New Employee</empName>
<position>Clerk</position>
</Employee>
POST http://localhost:8080/employee
Acept: application/json
{"empNo":"E11","empName":"New Employee","position":"Clerk"}
Anleitungen Java Web Services
- Was ist RESTful Web Service?
- Die Anleitung zum Java RESTful Web Services für den Anfänger
- Zum Beispiel CRUD einfach mit Java Restful Web Service
- Erstellen Sie einen Java RESTful Client mit Jersey Client
- RESTClient Ein Debugger für RESTful Web Services
- Einfache CRUD Beispiel mit Spring MVC RESTful Web Service
- CRUD Restful Web Service Beispiel mit Spring Boot
- Beispiel Spring Boot Restful Client mit RestTemplate
- Sichere Spring Boot RESTful Service mit Basic Authentication
Show More
Anleitungen Spring Boot
- Installieren Sie die Spring Tool Suite für Eclipse
- Die Anleitung zum Sping für den Anfänger
- Die Anleitung zum Spring Boot für den Anfänger
- Gemeinsame Eigenschaften von Spring Boot
- Die Anleitung zu Spring Boot und Thymeleaf
- Die Anleitung zu Spring Boot und FreeMarker
- Die Anleitung zu Spring Boot und Groovy
- Die Anleitung zu Spring Boot und Mustache
- Die Anleitung zu Spring Boot und JSP
- Die Anleitung zu Spring Boot, Apache Tiles, JSP
- Verwenden Sie Logging im Spring Boot
- Anwendungsüberwachung mit Spring Boot Actuator
- Erstellen Sie eine mehrsprachige Webanwendung mit Spring Boot
- Verwenden Sie im Spring Boot mehrere ViewResolver
- Verwenden Sie Twitter Bootstrap im Spring Boot
- Die Anleitung zu Spring Boot Interceptor
- Die Anleitung zu Spring Boot, Spring JDBC und Spring Transaction
- Die Anleitung zu Spring JDBC
- Die Anleitung zu Spring Boot, JPA und Spring Transaction
- Die Anleitung zu Spring Boot und Spring Data JPA
- Die Anleitung zu Spring Boot, Hibernate und Spring Transaction
- Spring Boot, JPA und H2-Datenbank integrieren
- Die Anleitung zu Spring Boot und MongoDB
- Verwenden Sie mehrere DataSource mit Spring Boot und JPA
- Verwenden Sie mehrere DataSource mit Spring Boot und RoutingDataSource
- Erstellen Sie eine Login-Anwendung mit Spring Boot, Spring Security, Spring JDBC
- Erstellen Sie eine Login-Anwendung mit Spring Boot, Spring Security, JPA
- Erstellen Sie eine Benutzerregistrierungsanwendung mit Spring Boot, Spring Form Validation
- Beispiel für OAuth2 Social Login im Spring Boot
- Führen Sie geplante Hintergrundaufgaben in Spring aus
- CRUD Restful Web Service Beispiel mit Spring Boot
- Beispiel Spring Boot Restful Client mit RestTemplate
- CRUD-Beispiel mit Spring Boot, REST und AngularJS
- Sichere Spring Boot RESTful Service mit Basic Authentication
- Sicherer Spring Boot RESTful Service mit Auth0 JWT
- Beispiel Upload file mit Spring Boot
- Beispiel Download File mit Spring Boot
- Das Beispiel: Spring Boot File Upload mit jQuery Ajax
- Das Beispiel File Upload mit Spring Boot und AngularJS
- Erstellen Sie eine Warenkorb-Webanwendung mit Spring Boot, Hibernate
- Die Anleitung zu Spring Email
- Erstellen Sie eine einfache Chat-Anwendung mit Spring Boot und Websocket
- Stellen Sie die Spring Boot-Anwendung auf Tomcat Server bereit
- Stellen Sie die Spring Boot-Anwendung auf Oracle WebLogic Server bereit
- Installieren Sie ein kostenloses Let's Encrypt SSL-Zertifikat für Spring Boot
- Konfigurieren Sie Spring Boot so, dass HTTP zu HTTPS umgeleitet wird
Show More