Beispiel Spring Boot Restful Client mit RestTemplate
1. Das Zweck des Beispiel
Der Unterlagen wird nach ... geschrieben
- Spring Boot 2.x
- RestTemplate
- Eclipse 3.7
Im Unterlagen leite ich Ihnen bei der Erstellung einer Applikation Restful Client mit der Verwendung von Spring Boot. Und es gibt 4 Funktion :
- Eine Anforderung mit der Methode GET erstellen,und nach Restful Web Service schicken um die Liste der Mitarbeiter (employee), oder die Information eines Mitarbeiter zu holen. Die erhalteten Daten hat die Format XML oder JSON.
- Eine Anforderung mit der Methode PUT erstellen,und nach Restful Web Service schicken um die Information eines Mitarbeiter zu ändern. Die beigefügten Daten in Request hat die Format XML oder JSON.
- Eine Anforderung mit der Methode POST erstellen,und nach Restful Web Service schicken um einen Mitarbeiter einzufügen. Die beigefügten Daten in Request hat die Format XML oder JSON.
- Eine Anforderung mit der Methode DELETE erstellen, und nach Restful Web Service schicken um einen Mitarbeiter zu löschen
Der Unterlagen benutzt die vom folgenden Beispiel erstellten Restful Web Service:
Die Klasse RestTemplate ist eine Zentrumsklasse im Spring Framework für synchronous calls durch Client um in RESTful Web Service zuzugreifen. Diese Klasse bietet die Funktionen um REST Services einfach zu verbrauchen. Bei der Verwendung der oben gemeinten Klasse bietet der Benutzer URL, den Parameter (wenn ja) und extrahiert die erhalteten Ergebnisse. RestTemplate verwaltet die Verbindung HTTP (HTTP Connection).
2. Spring Boot Projekt erstellen
Auf Eclipse erstellen Sie Spring Boot Projekt
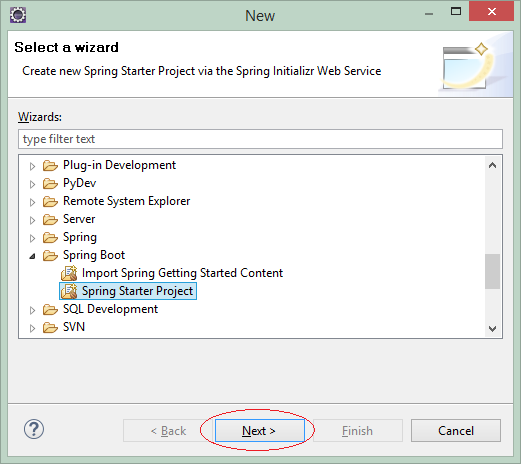
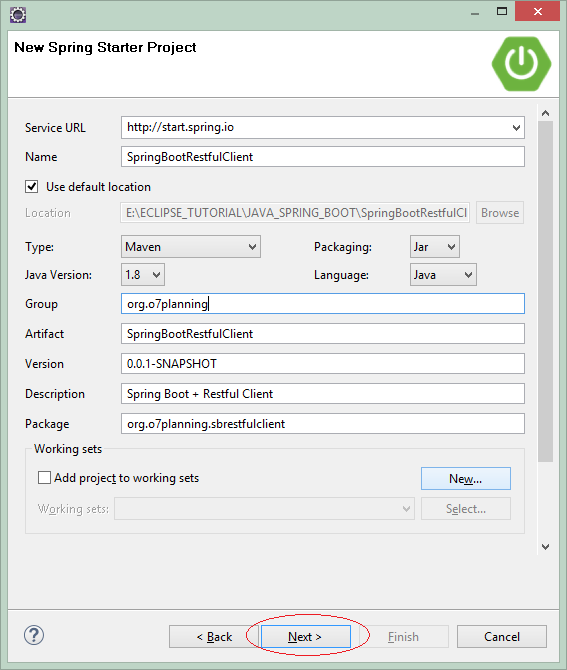
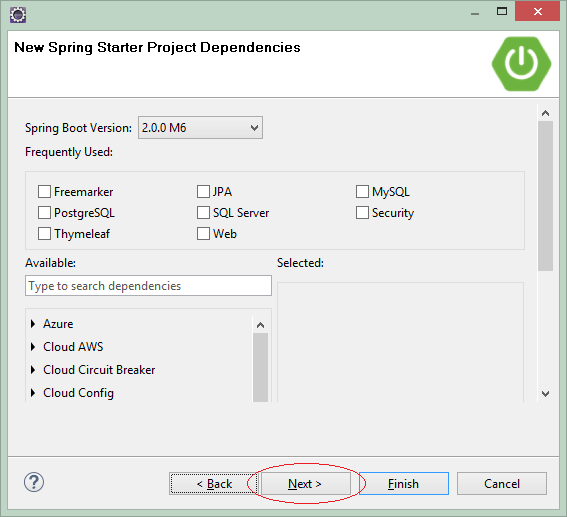
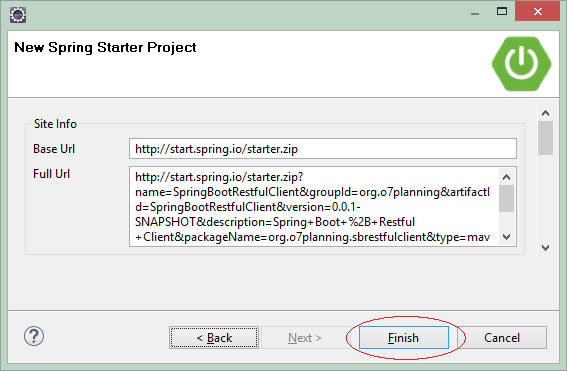
OK, Das Projekt wird erstellt
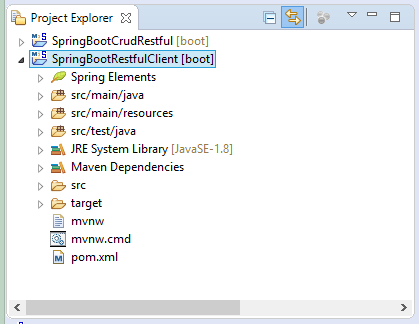
3. pom.xml konfigurieren
Das Projekt brauch die Bibliothek Spring Restful Client verwenden. Deshalb haben Sie 2 Auswählen
- spring-boot-starter-web
- spring-boot-starter-data-rest
spring-boot-starter-web
spring-boot-starter-web fasst die Bibliothek zur Aufbau einer Web- Applikation benutzend Spring MVC um, und verwendet tomcat wie ein standard eingebetter Web Container . Es fasst die Bibliothek für die Applikation RESTful um.
spring-boot-starter-web
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
spring-boot-starter-data-rest
spring-boot-starter-data-rest fasst die Bibliothek zum Umgang mit Spring RESTful um.
spring-boot-starter-data-rest
<!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-starter-data-rest -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-rest</artifactId>
</dependency>
Java <==> JSON
Die beiden "Starter" oben fasst die Bibliothek jackson-databind um um ein Objekt Java zu JSON umzuwandeln und umgekehrt
Java <==> XML
Spring verwendet JAXB (in JDK verfügbar) um das Objekt Java zu XML umzuwandeln und umgekehrt. Allerdings werden die Klasse Java durch @XmlRootElement... annotiert. Deshalb sollen Sie meiner Meinung nach jackson-dataformat-xml als eine Bibliothek zum Umwandlung von XML und Java verwenden. Um jackson-dataformat-xml zu verwenden, sollen Sie es in die File n pom.xml deklarieren:
jackson-dataformat-xml
<dependency>
<groupId>com.fasterxml.jackson.dataformat</groupId>
<artifactId>jackson-dataformat-xml</artifactId>
</dependency>
OK, Sie haben 2 Auswählen um in pom.xml zu deklarieren:
** pom.xml (Option 1) **
<dependencies>
......
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-rest</artifactId>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.dataformat</groupId>
<artifactId>jackson-dataformat-xml</artifactId>
</dependency>
.....
</dependencies>
** pom.xml (Option 2) **
<dependencies>
......
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.dataformat</groupId>
<artifactId>jackson-dataformat-xml</artifactId>
</dependency>
.....
</dependencies>
Die Bibliothek Apache Commons Codec ist notwendig um username/password zu enkodieren (encode) falls Sie Rest Client zum Zugang der von Basic Authentication gesicherten Datensource verwenden.
<!-- https://mvnrepository.com/artifact/commons-codec/commons-codec -->
<dependency>
<groupId>commons-codec</groupId>
<artifactId>commons-codec</artifactId>
</dependency>
Die Inhalt der Datei pom.xml:
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>SpringBootRestfulClient</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>SpringBootRestfulClient</name>
<description>Spring Boot + Restful Client</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.0.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-rest</artifactId>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.dataformat</groupId>
<artifactId>jackson-dataformat-xml</artifactId>
</dependency>
<!-- https://mvnrepository.com/artifact/commons-codec/commons-codec -->
<dependency>
<groupId>commons-codec</groupId>
<artifactId>commons-codec</artifactId>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
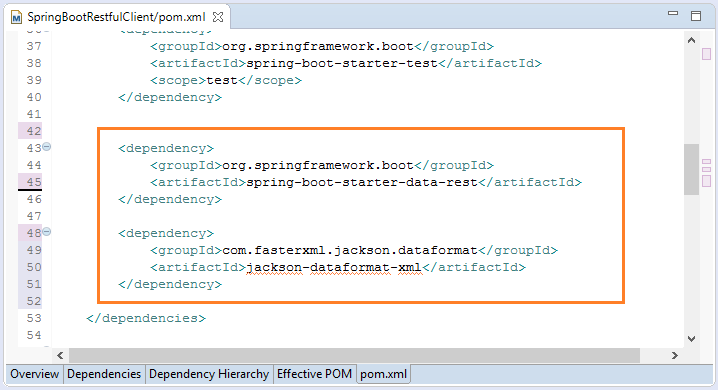
4. GET - getForObject
Die Methode getForObject verwendet um eine Anforderung (request) nach Restful Service zu schicken und die Ergebnis zu erhalten. Unter ist es das meist einfache Beispiel, die zurückgegebene Ergebnis ist String.
SimplestGetExample.java
package org.o7planning.sbrestfulclient.get;
import org.springframework.web.client.RestTemplate;
public class SimplestGetExample {
static final String URL_EMPLOYEES = "http://localhost:8080/employees";
static final String URL_EMPLOYEES_XML = "http://localhost:8080/employees.xml";
static final String URL_EMPLOYEES_JSON = "http://localhost:8080/employees.json";
public static void main(String[] args) {
RestTemplate restTemplate = new RestTemplate();
// Send request with GET method and default Headers.
String result = restTemplate.getForObject(URL_EMPLOYEES, String.class);
System.out.println(result);
}
}
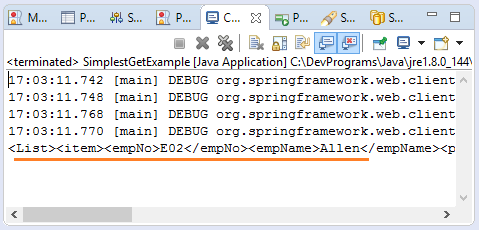
Die Anforderungen, die nach Restful Service geschickt werden, sollen die Information Headers anpassen um Restful Service die gewünschten Datensformat zu informieren (JSON, XML, ...)
GetWithHeaderExample.java
package org.o7planning.sbrestfulclient.get;
import java.util.Arrays;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpMethod;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.web.client.RestTemplate;
public class GetWithHeaderExample {
static final String URL_EMPLOYEES = "http://localhost:8080/employees";
public static void main(String[] args) {
// HttpHeaders
HttpHeaders headers = new HttpHeaders();
headers.setAccept(Arrays.asList(new MediaType[] { MediaType.APPLICATION_JSON }));
// Request to return JSON format
headers.setContentType(MediaType.APPLICATION_JSON);
headers.set("my_other_key", "my_other_value");
// HttpEntity<String>: To get result as String.
HttpEntity<String> entity = new HttpEntity<String>(headers);
// RestTemplate
RestTemplate restTemplate = new RestTemplate();
// Send request with GET method, and Headers.
ResponseEntity<String> response = restTemplate.exchange(URL_EMPLOYEES, //
HttpMethod.GET, entity, String.class);
String result = response.getBody();
System.out.println(result);
}
}
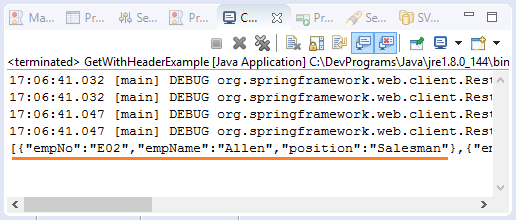
Die aus Restful Serivce zurückgegebenen Daten in die Format XML oder JSON kann zum Objekt Java umgewandelt werden.
Employee.java
package org.o7planning.sbrestfulclient.model;
public class Employee {
private String empNo;
private String empName;
private String position;
public Employee() {
}
public Employee(String empNo, String empName, String position) {
this.empNo = empNo;
this.empName = empName;
this.position = position;
}
public String getEmpNo() {
return empNo;
}
public void setEmpNo(String empNo) {
this.empNo = empNo;
}
public String getEmpName() {
return empName;
}
public void setEmpName(String empName) {
this.empName = empName;
}
public String getPosition() {
return position;
}
public void setPosition(String position) {
this.position = position;
}
}
SimplestGetPOJOExample.java
package org.o7planning.sbrestfulclient.get;
import org.o7planning.sbrestfulclient.model.Employee;
import org.springframework.web.client.RestTemplate;
public class SimplestGetPOJOExample {
static final String URL_EMPLOYEES = "http://localhost:8080/employees";
static final String URL_EMPLOYEES_XML = "http://localhost:8080/employees.xml";
static final String URL_EMPLOYEES_JSON = "http://localhost:8080/employees.json";
public static void main(String[] args) {
RestTemplate restTemplate = new RestTemplate();
// Send request with GET method and default Headers.
Employee[] list = restTemplate.getForObject(URL_EMPLOYEES, Employee[].class);
if (list != null) {
for (Employee e : list) {
System.out.println("Employee: " + e.getEmpNo() + " - " + e.getEmpName());
}
}
}
}
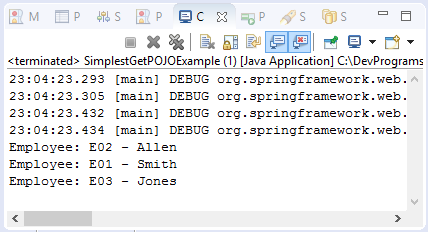
5. GET - exchange
Die Methode exchange verwendet hilft Ihnen bei dem Schicken eines Request nach Restful Service, das zurückgegebene Ergebnis ist ResponseEntity. Das Objekt enthaltet viele bemerkenswerten Informationen, zum Beispiel HttpStatus,...
GetPOJOWithHeaderExample.java
package org.o7planning.sbrestfulclient.get;
import java.util.Arrays;
import org.o7planning.sbrestfulclient.model.Employee;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpMethod;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.web.client.RestTemplate;
public class GetPOJOWithHeaderExample {
static final String URL_EMPLOYEES = "http://localhost:8080/employees";
public static void main(String[] args) {
// HttpHeaders
HttpHeaders headers = new HttpHeaders();
headers.setAccept(Arrays.asList(new MediaType[] { MediaType.APPLICATION_XML }));
// Request to return XML format
headers.setContentType(MediaType.APPLICATION_XML);
headers.set("my_other_key", "my_other_value");
// HttpEntity<Employee[]>: To get result as Employee[].
HttpEntity<Employee[]> entity = new HttpEntity<Employee[]>(headers);
// RestTemplate
RestTemplate restTemplate = new RestTemplate();
// Send request with GET method, and Headers.
ResponseEntity<Employee[]> response = restTemplate.exchange(URL_EMPLOYEES, //
HttpMethod.GET, entity, Employee[].class);
HttpStatus statusCode = response.getStatusCode();
System.out.println("Response Satus Code: " + statusCode);
// Status Code: 200
if (statusCode == HttpStatus.OK) {
// Response Body Data
Employee[] list = response.getBody();
if (list != null) {
for (Employee e : list) {
System.out.println("Employee: " + e.getEmpNo() + " - " + e.getEmpName());
}
}
}
}
}
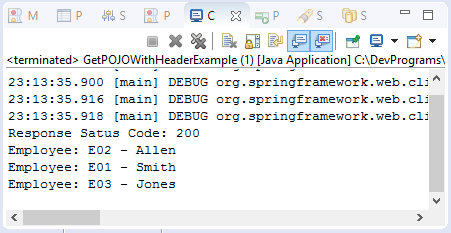
6. GET - Basic Authentication
Mit der durch Basic Authentication gesicherten Datensource (resource) wird die Anforderungen, die Sie nach REST Service gesichert, mit username/password mitschicken. Die Information username/password soll das Algorithmus Base64 enkodiert werden (encode) vor dem Anfügen vom request.
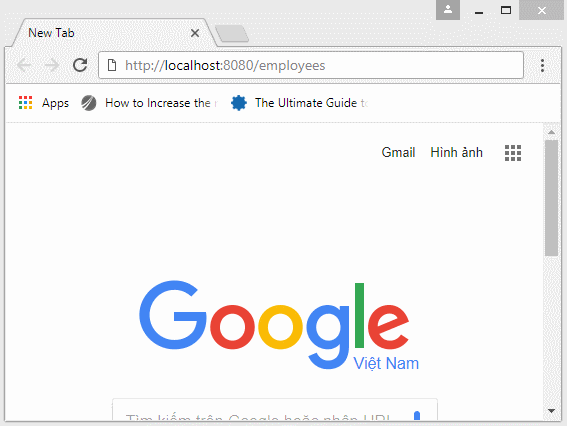
GetWithBasicAuthExample.java
package org.o7planning.sbrestfulclient.get;
import java.nio.charset.Charset;
import java.util.Arrays;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpMethod;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.web.client.RestTemplate;
import org.apache.commons.codec.binary.Base64;
public class GetWithBasicAuthExample {
public static final String USER_NAME = "tom";
public static final String PASSWORD = "123";
static final String URL_EMPLOYEES = "http://localhost:8080/employees";
public static void main(String[] args) {
// HttpHeaders
HttpHeaders headers = new HttpHeaders();
//
// Authentication
//
String auth = USER_NAME + ":" + PASSWORD;
byte[] encodedAuth = Base64.encodeBase64(auth.getBytes(Charset.forName("US-ASCII")));
String authHeader = "Basic " + new String(encodedAuth);
headers.set("Authorization", authHeader);
//
headers.setAccept(Arrays.asList(new MediaType[] { MediaType.APPLICATION_JSON }));
// Request to return JSON format
headers.setContentType(MediaType.APPLICATION_JSON);
headers.set("my_other_key", "my_other_value");
// HttpEntity<String>: To get result as String.
HttpEntity<String> entity = new HttpEntity<String>(headers);
// RestTemplate
RestTemplate restTemplate = new RestTemplate();
// Send request with GET method, and Headers.
ResponseEntity<String> response = restTemplate.exchange(URL_EMPLOYEES, //
HttpMethod.GET, entity, String.class);
String result = response.getBody();
System.out.println(result);
}
}
7. POST - postForObject
Die Methode postForObject wird verwendet um eine Anforderung nach Restful Service zur Erstellung einer Ressourcen zu schicken und gleichzeitig die erstellten Ressourcen zurückzugeben
Post_postForObject_Example.java
package org.o7planning.sbrestfulclient.post;
import org.o7planning.sbrestfulclient.model.Employee;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.http.MediaType;
import org.springframework.web.client.RestTemplate;
public class Post_postForObject_Example {
static final String URL_CREATE_EMPLOYEE = "http://localhost:8080/employee";
public static void main(String[] args) {
String empNo = "E11";
Employee newEmployee = new Employee(empNo, "Tom", "Cleck");
HttpHeaders headers = new HttpHeaders();
headers.add("Accept", MediaType.APPLICATION_XML_VALUE);
headers.setContentType(MediaType.APPLICATION_XML);
RestTemplate restTemplate = new RestTemplate();
// Data attached to the request.
HttpEntity<Employee> requestBody = new HttpEntity<>(newEmployee, headers);
// Send request with POST method.
Employee e = restTemplate.postForObject(URL_CREATE_EMPLOYEE, requestBody, Employee.class);
if (e != null && e.getEmpNo() != null) {
System.out.println("Employee created: " + e.getEmpNo());
} else {
System.out.println("Something error!");
}
}
}
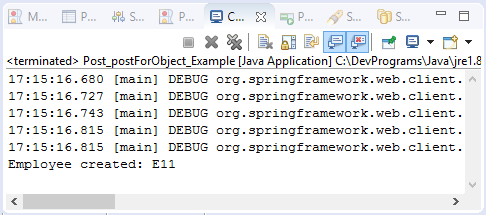
8. POST - postForEntity
Die Methode postForEntity wird verwendet um eine Anforderung nach Restful Service zur Erstellung einer Ressourcen zu schicken . Die Methode gibt das Objekt ResponseEntity zurückgeben. Das Objekt enthaltet die neu erstellte Ressourcen und die beziehenden Informationen, z.B HttpStatus, ...
Post_postForEntity_Example.java
package org.o7planning.sbrestfulclient.post;
import org.o7planning.sbrestfulclient.model.Employee;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.client.RestTemplate;
public class Post_postForEntity_Example {
static final String URL_CREATE_EMPLOYEE = "http://localhost:8080/employee";
public static void main(String[] args) {
Employee newEmployee = new Employee("E11", "Tom", "Cleck");
RestTemplate restTemplate = new RestTemplate();
// Data attached to the request.
HttpEntity<Employee> requestBody = new HttpEntity<>(newEmployee);
// Send request with POST method.
ResponseEntity<Employee> result
= restTemplate.postForEntity(URL_CREATE_EMPLOYEE, requestBody, Employee.class);
System.out.println("Status code:" + result.getStatusCode());
// Code = 200.
if (result.getStatusCode() == HttpStatus.OK) {
Employee e = result.getBody();
System.out.println("(Client Side) Employee Created: "+ e.getEmpNo());
}
}
}
9. PUT - Das einfache Beispiel
Die Methode put der Klasse RestTemplate wird verwendet um eine Anforderung nach Restful Service zur Änderung einer Ressourcen zu schicken. Diese Methode gibt nichts zurück
PutSimpleExample.java
package org.o7planning.sbrestfulclient.put;
import org.o7planning.sbrestfulclient.model.Employee;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.http.MediaType;
import org.springframework.web.client.RestTemplate;
public class PutSimpleExample {
static final String URL_UPDATE_EMPLOYEE = "http://localhost:8080/employee";
static final String URL_EMPLOYEE_PREFIX = "http://localhost:8080/employee";
public static void main(String[] args) {
String empNo = "E01";
Employee updateInfo = new Employee(empNo, "Tom", "Cleck");
HttpHeaders headers = new HttpHeaders();
headers.add("Accept", MediaType.APPLICATION_JSON_VALUE);
RestTemplate restTemplate = new RestTemplate();
// Data attached to the request.
HttpEntity<Employee> requestBody = new HttpEntity<>(updateInfo, headers);
// Send request with PUT method.
restTemplate.put(URL_UPDATE_EMPLOYEE, requestBody, new Object[] {});
String resourceUrl = URL_EMPLOYEE_PREFIX + "/" + empNo;
Employee e = restTemplate.getForObject(resourceUrl, Employee.class);
if (e != null) {
System.out.println("(Client side) Employee after update: ");
System.out.println("Employee: " + e.getEmpNo() + " - " + e.getEmpName());
}
}
}
10. PUT - exchange
Das Beispiel über die Verwendung der Methode exchange der Klasse RestTemplate um eine Anforderung nach Restful Service zur Änderung der Ressourcen zu schicken
PutWithExchangeExample.java
package org.o7planning.sbrestfulclient.put;
import org.o7planning.sbrestfulclient.model.Employee;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpMethod;
import org.springframework.http.MediaType;
import org.springframework.web.client.RestTemplate;
public class PutWithExchangeExample {
static final String URL_UPDATE_EMPLOYEE = "http://localhost:8080/employee";
static final String URL_EMPLOYEE_PREFIX = "http://localhost:8080/employee";
public static void main(String[] args) {
String empNo = "E01";
Employee updateInfo = new Employee(empNo, "Tom", "Cleck");
HttpHeaders headers = new HttpHeaders();
headers.add("Accept", MediaType.APPLICATION_JSON_VALUE);
RestTemplate restTemplate = new RestTemplate();
// Data attached to the request.
HttpEntity<Employee> requestBody = new HttpEntity<>(updateInfo, headers);
// Send request with PUT method.
restTemplate.exchange(URL_UPDATE_EMPLOYEE, HttpMethod.PUT, requestBody, Void.class);
String resourceUrl = URL_EMPLOYEE_PREFIX + "/" + empNo;
Employee e = restTemplate.getForObject(resourceUrl, Employee.class);
if (e != null) {
System.out.println("(Client side) Employee after update: ");
System.out.println("Employee: " + e.getEmpNo() + " - " + e.getEmpName());
}
}
}
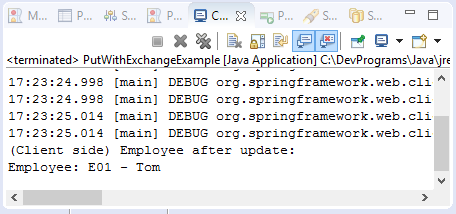
11. DELELE
Die Methode delete der Klasse RestTemplate verwenden um eine Anforderung nach Restful Service zur Löschung einer Ressourcen
DeleteSimpleExample.java
package org.o7planning.sbrestfulclient.delete;
import org.o7planning.sbrestfulclient.model.Employee;
import org.springframework.web.client.RestTemplate;
public class DeleteSimpleExample {
public static void main(String[] args) {
RestTemplate restTemplate = new RestTemplate();
// empNo="E01"
String resourceUrl = "http://localhost:8080/employee/E01";
// Send request with DELETE method.
restTemplate.delete(resourceUrl);
// Get
Employee e = restTemplate.getForObject(resourceUrl, Employee.class);
if (e != null) {
System.out.println("(Client side) Employee after delete: ");
System.out.println("Employee: " + e.getEmpNo() + " - " + e.getEmpName());
} else {
System.out.println("Employee not found!");
}
}
}
DeleteExample2.java
package org.o7planning.sbrestfulclient.delete;
import org.o7planning.sbrestfulclient.model.Employee;
import org.springframework.web.client.RestTemplate;
public class DeleteExample2 {
public static void main(String[] args) {
RestTemplate restTemplate = new RestTemplate();
// URL with URI-variable
String resourceUrl = "http://localhost:8080/employee/{empNo}";
Object[] uriValues = new Object[] { "E01" };
// Send request with DELETE method.
restTemplate.delete(resourceUrl, uriValues);
Employee e = restTemplate.getForObject(resourceUrl, Employee.class);
if (e != null) {
System.out.println("(Client side) Employee after delete: ");
System.out.println("Employee: " + e.getEmpNo() + " - " + e.getEmpName());
} else {
System.out.println("Employee not found!");
}
}
}
Anleitungen Java Web Services
- Was ist RESTful Web Service?
- Die Anleitung zum Java RESTful Web Services für den Anfänger
- Zum Beispiel CRUD einfach mit Java Restful Web Service
- Erstellen Sie einen Java RESTful Client mit Jersey Client
- RESTClient Ein Debugger für RESTful Web Services
- Einfache CRUD Beispiel mit Spring MVC RESTful Web Service
- CRUD Restful Web Service Beispiel mit Spring Boot
- Beispiel Spring Boot Restful Client mit RestTemplate
- Sichere Spring Boot RESTful Service mit Basic Authentication
Show More
Anleitungen Spring Boot
- Installieren Sie die Spring Tool Suite für Eclipse
- Die Anleitung zum Sping für den Anfänger
- Die Anleitung zum Spring Boot für den Anfänger
- Gemeinsame Eigenschaften von Spring Boot
- Die Anleitung zu Spring Boot und Thymeleaf
- Die Anleitung zu Spring Boot und FreeMarker
- Die Anleitung zu Spring Boot und Groovy
- Die Anleitung zu Spring Boot und Mustache
- Die Anleitung zu Spring Boot und JSP
- Die Anleitung zu Spring Boot, Apache Tiles, JSP
- Verwenden Sie Logging im Spring Boot
- Anwendungsüberwachung mit Spring Boot Actuator
- Erstellen Sie eine mehrsprachige Webanwendung mit Spring Boot
- Verwenden Sie im Spring Boot mehrere ViewResolver
- Verwenden Sie Twitter Bootstrap im Spring Boot
- Die Anleitung zu Spring Boot Interceptor
- Die Anleitung zu Spring Boot, Spring JDBC und Spring Transaction
- Die Anleitung zu Spring JDBC
- Die Anleitung zu Spring Boot, JPA und Spring Transaction
- Die Anleitung zu Spring Boot und Spring Data JPA
- Die Anleitung zu Spring Boot, Hibernate und Spring Transaction
- Spring Boot, JPA und H2-Datenbank integrieren
- Die Anleitung zu Spring Boot und MongoDB
- Verwenden Sie mehrere DataSource mit Spring Boot und JPA
- Verwenden Sie mehrere DataSource mit Spring Boot und RoutingDataSource
- Erstellen Sie eine Login-Anwendung mit Spring Boot, Spring Security, Spring JDBC
- Erstellen Sie eine Login-Anwendung mit Spring Boot, Spring Security, JPA
- Erstellen Sie eine Benutzerregistrierungsanwendung mit Spring Boot, Spring Form Validation
- Beispiel für OAuth2 Social Login im Spring Boot
- Führen Sie geplante Hintergrundaufgaben in Spring aus
- CRUD Restful Web Service Beispiel mit Spring Boot
- Beispiel Spring Boot Restful Client mit RestTemplate
- CRUD-Beispiel mit Spring Boot, REST und AngularJS
- Sichere Spring Boot RESTful Service mit Basic Authentication
- Sicherer Spring Boot RESTful Service mit Auth0 JWT
- Beispiel Upload file mit Spring Boot
- Beispiel Download File mit Spring Boot
- Das Beispiel: Spring Boot File Upload mit jQuery Ajax
- Das Beispiel File Upload mit Spring Boot und AngularJS
- Erstellen Sie eine Warenkorb-Webanwendung mit Spring Boot, Hibernate
- Die Anleitung zu Spring Email
- Erstellen Sie eine einfache Chat-Anwendung mit Spring Boot und Websocket
- Stellen Sie die Spring Boot-Anwendung auf Tomcat Server bereit
- Stellen Sie die Spring Boot-Anwendung auf Oracle WebLogic Server bereit
- Installieren Sie ein kostenloses Let's Encrypt SSL-Zertifikat für Spring Boot
- Konfigurieren Sie Spring Boot so, dass HTTP zu HTTPS umgeleitet wird
Show More