Die Anleitung zu ReactJS Event
In dieser Unterricht werde ich Sie "Wie Event im ReactJS zu verwenden" anleiten. Es gibt nicht viele Unterschiede wenn Sie die event im ReactJS im Vergleich von event im Javascript benutzen
- Im Javascript werden Sie Event in einer Funktion behandeln während Sie im React Event in einer Methode vom Component.
1. ReactJS Events
React ist eine Bibliothek, die auf Javascript basiert. Im Grunde gibt es nicht viele Unterschiede in der Behandlung des Event zwischen ReactJS und Javascript. Mit Javascript , wenn Event passiert, wird eine Funktion zu implementieren aufgeruft. Aber mit React, wenn Event passiert, wird eine Methode vom Component aufgeruft.
OK, zuerst sehen Sie ein einfaches Beispiel:
onClick-example.html
class CurrentTime extends React.Component {
constructor(props) {
super(props);
var now = new Date();
this.state = {
currentTime: now.toString()
};
}
// A method of CurrentTime component
refreshCurrentTime() {
var now = new Date();
this.setState({ currentTime: now.toString() });
}
render() {
return (
<div>
<h4>Current Time:</h4>
<p>{this.state.currentTime}</p>
<button onClick={() => this.refreshCurrentTime()}>
Refresh Current Time
</button>
</div>
);
}
}
// Render
ReactDOM.render(<CurrentTime />, document.getElementById("currenttime1"));
onClick-example.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>ReactJS Event</title>
<script src="https://unpkg.com/react@16.4.2/umd/react.production.min.js"></script>
<script src="https://unpkg.com/react-dom@16.4.2/umd/react-dom.production.min.js"></script>
<script src="https://unpkg.com/babel-standalone@6.26.0/babel.min.js"></script>
<style>
#currenttime1 {
border:1px solid blue;
padding: 5px;
margin-top: 20px;
}
</style>
</head>
<body>
<h3>ReactJS Event: onClick</h3>
<div id="currenttime1"></div>
<script src="onClick-example.jsx" type="text/babel"></script>
</body>
</html>
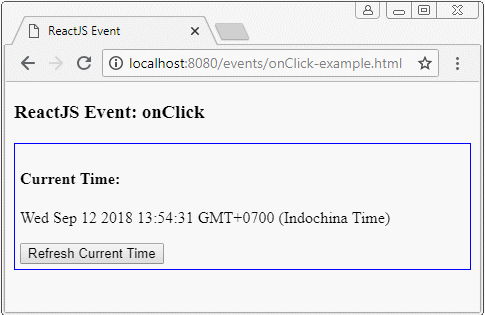
Arrow Function
Mit der Syntax Javascript ES6 können Sie ein arrow function ,die sehr kurz und einfach zu verstehen ist:
** arrow function **
// Normal function with parameters
var myfunc = function(param1, param2) {
// Statements
};
// Arrow function with parameters.
var arrfunc = (param1, param2) => {
// Statements
};
// Normal function without paramters.
var myfunc2 = function( ) {
// Statements
};
// Arrow function without parameters.
var arrfunc2 = ( ) => {
// Statements
};
// If function have only one statement.
var arrfunc2 = ( ) => singleStatement;
onClick, onChange,..
onClick muss auf einer Funktion Javascript aufrufen, Es kann auf einer Methode vom Component direkt nicht aufrufen. Deshalb soll onClick auf einer anonymen Funktion aufrufen und innerhalb dieser Funktion können Sie auf die Methode vom Component aufrufen:
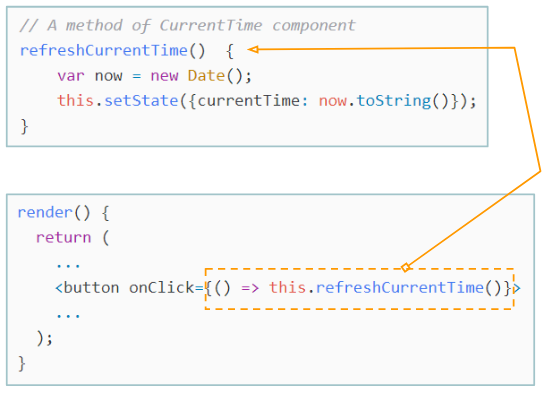
Arrow function mit dem Parameter event:
// A method of Component with event parameter.
refreshCurrentTime(event) {
var now = new Date();
this.setState({ currentTime: now.toString() });
}
render() {
return (
<div>
<h4>Current Time:</h4>
<p>{this.state.currentTime}</p>
<button onClick={(event) => this.refreshCurrentTime(event)}>
Refresh Current Time
</button>
</div>
);
}
Mit Javascript ES6 können Sie auf die Methode vom Component nach der folgenden Weise aufrufen:
.jsx
// A method of this Component.
refreshCurrentTime(event) {
var now = new Date();
this.setState({ currentTime: now.toString() });
}
render() {
return (
<div>
<h4>Current Time:</h4>
<p>{this.state.currentTime}</p>
<button onClick={this.refreshCurrentTime.bind(this,event)}>
Refresh Current Time
</button>
</div>
);
}
Oder
onClick-example3.jsx
class CurrentTime extends React.Component {
constructor(props) {
super(props);
var now = new Date();
this.state = {
currentTime: now.toString()
};
// (***)
this.refreshCurrentTime = this.refreshCurrentTime.bind(this);
}
// A method of this Component
refreshCurrentTime( ) {
var now = new Date();
this.setState({ currentTime: now.toString() });
}
render() {
return (
<div>
<h4>Current Time:</h4>
<p>{this.state.currentTime}</p>
<button onClick={this.refreshCurrentTime}>
Refresh Current Time
</button>
</div>
);
}
}
// Render
ReactDOM.render(<CurrentTime />, document.getElementById("currenttime1"));
2. Event vom Sub-Component
Ein Event passiert bei einem Sub- Component , aber Sie möchten auf einer Methode vom Vater-Component aufrufen. Unter ist es ein solches Beispiel.
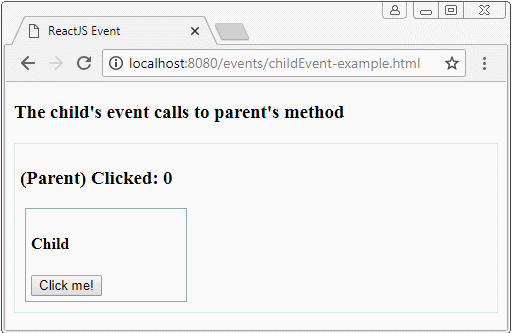
childEvent-example.jsx
// <Child> with 1 attribute: myClickHandler
// <Child myClickHandler=.. />
class Child extends React.Component {
render() {
return (
<div className="child">
<h4>Child</h4>
<button onClick={this.props.myClickHandler}>Click me!</button>
</div>
);
}
}
class Parent extends React.Component {
constructor(props) {
super(props);
this.state = {
count: 0
};
}
myMethodOfParent() {
this.setState((prevState, props) => {
return {
count: prevState.count + 1
};
});
}
render() {
return (
<div className="parent">
<h3>(Parent) Clicked: {this.state.count} </h3>
<Child myClickHandler={() => this.myMethodOfParent()} />
</div>
);
}
}
// Render
ReactDOM.render(<Parent />, document.getElementById("placeholder1"));
childEvent-example.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>ReactJS Event</title>
<script src="https://unpkg.com/react@16.4.2/umd/react.production.min.js"></script>
<script src="https://unpkg.com/react-dom@16.4.2/umd/react-dom.production.min.js"></script>
<script src="https://unpkg.com/babel-standalone@6.26.0/babel.min.js"></script>
<style>
.parent {
border:1px solid #d9ebe8;
padding: 5px;
margin-top: 20px;
}
.child {
width:150px;
border:1px solid #8cb0aa;
margin: 5px;
padding: 5px;
}
</style>
</head>
<body>
<h3>The child's event calls to parent's method</h3>
<div id="placeholder1"></div>
<script src="childEvent-example.jsx" type="text/babel"></script>
</body>
</html>
3. Ein kompliziertes Beispiel
OK, und jetzt ist es ein mehr kompliziertes Beispiel. Es fordert Sie, die Kenntnisse im ReactJS, wie Lists, Props, State, Event.. zu beherrschen
Die Kenntnisse im ReactJS , die Sie kenn brauchen
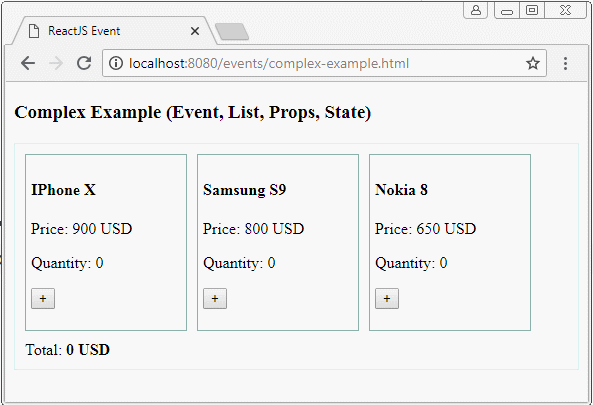
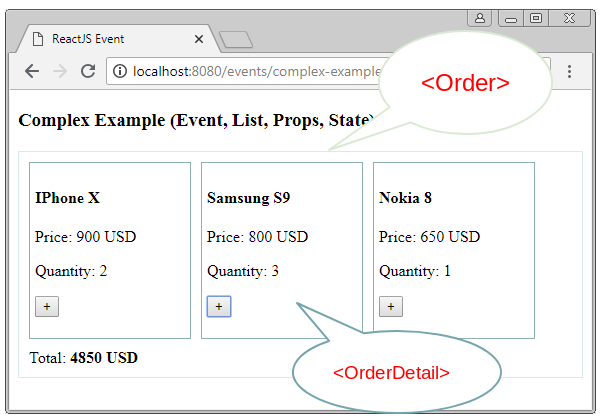
complex-example.jsx
// <OrderDetail> with 4 attributes:
// productName, price, quantity, addHandler
class OrderDetail extends React.Component {
render() {
return (
<div className="order-detail">
<h4>{this.props.productName}</h4>
<p>Price: {this.props.price} USD</p>
<p>Quantity: {this.props.quantity}</p>
<p>
<button onClick={this.props.addHandler}>+</button>
</p>
</div>
);
}
}
class Order extends React.Component {
constructor(props) {
super(props);
this.state = {
amount: 0,
details: [
{ id: 1, productName: "IPhone X", price: 900, quantity: 0 },
{ id: 2, productName: "Samsung S9", price: 800, quantity: 0 },
{ id: 3, productName: "Nokia 8", price: 650, quantity: 0 }
]
};
}
updateOrder(index) {
this.setState((prevState, props) => {
console.log(this.state.details);
var newQty = prevState.details[index].quantity + 1;
this.state.details[index].quantity = newQty;
this.state.amount = prevState.amount + 1 * prevState.details[index].price;
return {
amount: this.state.amount,
details: this.state.details
};
});
}
render() {
// Array of <OrderDetail ../>
var detailTags = this.state.details.map((e, index) => (
<OrderDetail
key={e.id}
addHandler={() => this.updateOrder(index)}
productName={e.productName}
price={e.price}
quantity={e.quantity}
/>
));
return (
<div className="order">
{detailTags}
<div className="clear" />
<p className="total">Total: <b>{this.state.amount} USD</b></p>
</div>
);
}
}
// Render
ReactDOM.render(<Order />, document.getElementById("order1"));
complex-example.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>ReactJS Event</title>
<script src="https://unpkg.com/react@16.4.2/umd/react.production.min.js"></script>
<script src="https://unpkg.com/react-dom@16.4.2/umd/react-dom.production.min.js"></script>
<script src="https://unpkg.com/babel-standalone@6.26.0/babel.min.js"></script>
<style>
.order {
border:1px solid #d9ebe8;
padding: 5px;
margin-top: 20px;
}
.order-detail {
float : left;
width:150px;
border:1px solid #8cb0aa;
margin: 5px;
padding: 5px;
}
.clear {
clear: both;
}
.total {
margin: 5px;
}
</style>
</head>
<body>
<h3>Complex Example (Event, List, Props, State)</h3>
<div id="order1"></div>
<script src="complex-example.jsx" type="text/babel"></script>
</body>
</html>
Anleitungen ReactJS
- Die Anleitung zu ReactJS props und state
- Die Anleitung zu ReactJS Event
- Die Anleitung zu ReactJS Component API
- Methoden in den Lebenskreis ReactJS Component
- Die Anleitung zu ReactJS Refs
- Die Anleitung zu ReactJS Lists und Keys
- Die Anleitung zu ReactJS Form
- Verstehen React Router mit Beispiel auf der Client-Seite
- Einführung in Redux
- Einfaches Beispiel mit React und Redux auf der Client-Seite
- Die Anleitung zu React-Transition-Group API
- Schnellstart mit ReactJS in die Umwelt NodeJS
- Verstehen ReactJS Router mit einem grundlegenden Beispiel (NodeJS)
- Beispiel React-Transition-Group Transition (NodeJS)
- Beispiel React-Transition-Group CSSTransition (NodeJS)
- Einführung in ReactJS
- Installieren Sie das React Plugin für Atom Editor
- Erstellen Sie einen einfachen HTTP-Server mit NodeJS
- Schnellstart mit ReactJS - Hallo ReactJS
Show More