Die Anleitung zu JavaFX ProgressBar und ProgressIndicator
1. ProgressBar und ProgressIndicator
ProgressBar und ProgressIndicator bezeichnen die Progress einer Task in der Applikation JavaFX
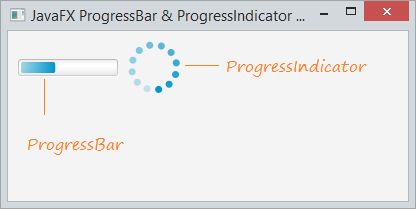
ProgressBar und ProgressIndicator mit der bestimmten Volume einer Task
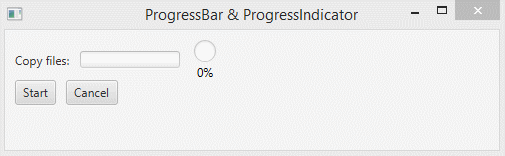
2. Das Beispiel mit ProgressBar & ProgressIndicator
Beispiel: ProgressBar und ProgressIndicator bezeichnen ein Progress mit der unbestimmten Endpunkt
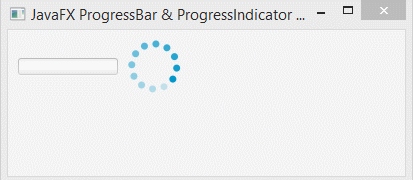
ProgressDemo.java
package org.o7planning.javafx.progress;
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.ProgressBar;
import javafx.scene.control.ProgressIndicator;
import javafx.scene.layout.FlowPane;
import javafx.stage.Stage;
public class ProgressDemo extends Application {
@Override
public void start(Stage stage) {
ProgressBar progressBar = new ProgressBar();
ProgressIndicator progressIndicator = new ProgressIndicator();
FlowPane root = new FlowPane();
root.setPadding(new Insets(10));
root.setHgap(10);
root.getChildren().addAll(progressBar, progressIndicator);
Scene scene = new Scene(root, 400, 300);
stage.setTitle("JavaFX ProgressBar & ProgressIndicator (o7planning.org)");
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
3. Progress und Task
Erstellung ein Task wie die Files kopieren. Die Kopie Task kostet die Zeit und Sie sollen die ProgressBar oder ProgressIndicator benutzen um die Prozent der erledigten Task darzustellen.
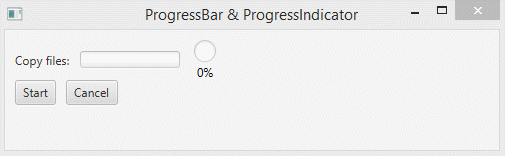
ProgressAndTaskDemo.java
package org.o7planning.javafx.progress;
import java.io.File;
import java.util.List;
import javafx.application.Application;
import javafx.concurrent.WorkerStateEvent;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.control.ProgressBar;
import javafx.scene.control.ProgressIndicator;
import javafx.scene.layout.FlowPane;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
public class ProgressAndTaskDemo extends Application {
private CopyTask copyTask;
@Override
public void start(Stage primaryStage) {
final Label label = new Label("Copy files:");
final ProgressBar progressBar = new ProgressBar(0);
final ProgressIndicator progressIndicator = new ProgressIndicator(0);
final Button startButton = new Button("Start");
final Button cancelButton = new Button("Cancel");
final Label statusLabel = new Label();
statusLabel.setMinWidth(250);
statusLabel.setTextFill(Color.BLUE);
// Start Button.
startButton.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
startButton.setDisable(true);
progressBar.setProgress(0);
progressIndicator.setProgress(0);
cancelButton.setDisable(false);
// Create a Task.
copyTask = new CopyTask();
// Unbind progress property
progressBar.progressProperty().unbind();
// Bind progress property
progressBar.progressProperty().bind(copyTask.progressProperty());
// Hủy bỏ kết nối thuộc tính progress
progressIndicator.progressProperty().unbind();
// Bind progress property.
progressIndicator.progressProperty().bind(copyTask.progressProperty());
// Unbind text property for Label.
statusLabel.textProperty().unbind();
// Bind the text property of Label
// with message property of Task
statusLabel.textProperty().bind(copyTask.messageProperty());
// When completed tasks
copyTask.addEventHandler(WorkerStateEvent.WORKER_STATE_SUCCEEDED, //
new EventHandler<WorkerStateEvent>() {
@Override
public void handle(WorkerStateEvent t) {
List<File> copied = copyTask.getValue();
statusLabel.textProperty().unbind();
statusLabel.setText("Copied: " + copied.size());
}
});
// Start the Task.
new Thread(copyTask).start();
}
});
// Cancel
cancelButton.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
startButton.setDisable(false);
cancelButton.setDisable(true);
copyTask.cancel(true);
progressBar.progressProperty().unbind();
progressIndicator.progressProperty().unbind();
statusLabel.textProperty().unbind();
//
progressBar.setProgress(0);
progressIndicator.setProgress(0);
}
});
FlowPane root = new FlowPane();
root.setPadding(new Insets(10));
root.setHgap(10);
root.getChildren().addAll(label, progressBar, progressIndicator, //
statusLabel, startButton, cancelButton);
Scene scene = new Scene(root, 500, 120, Color.WHITE);
primaryStage.setTitle("ProgressBar & ProgressIndicator");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
Application.launch(args);
}
}
CopyTask.java
package org.o7planning.javafx.progress;
import java.io.File;
import java.util.ArrayList;
import java.util.List;
import javafx.concurrent.Task;
// Copy all file in C:/Windows
public class CopyTask extends Task<List<File>> {
@Override
protected List<File> call() throws Exception {
File dir = new File("C:/Windows");
File[] files = dir.listFiles();
int count = files.length;
List<File> copied = new ArrayList<File>();
int i = 0;
for (File file : files) {
if (file.isFile()) {
this.copy(file);
copied.add(file);
}
i++;
this.updateProgress(i, count);
}
return copied;
}
private void copy(File file) throws Exception {
this.updateMessage("Copying: " + file.getAbsolutePath());
Thread.sleep(500);
}
}
Die Anleitungen JavaFX
- Öffnen Sie ein neues Fenster (window) in JavaFX
- Die Anleitung zu JavaFX ChoiceDialog
- Die Anleitung zu JavaFX Alert Dialog
- Die Anleitung zu JavaFX TextInputDialog
- Installieren Sie e(fx)clipse für Eclipse (JavaFX Tooling)
- Installieren Sie JavaFX Scene Builder für Eclipse
- Die Anleitung zum JavaFX für den Anfänger - Hello JavaFX
- Die Anleitung zu JavaFX FlowPane Layout
- Die Anleitung zu JavaFX TilePane Layout
- Die Anleitung zu JavaFX HBox, VBox Layout
- Die Anleitung zu JavaFX BorderPane Layout
- Die Anleitung zu JavaFX AnchorPane Layout
- Die Anleitung zu JavaFX TitledPane
- Die Anleitung zu JavaFX Accordion
- Die Anleitung zu JavaFX ListView
- Die Anleitung zu JavaFX Group
- Die Anleitung zu JavaFX ComboBox
- Die Anleitung zu JavaFX Transformation
- Effekte (Effects) in JavaFX
- Die Anleitung zu JavaFX GridPane Layout
- Die Anleitung zu JavaFX StackPane Layout
- Die Anleitung zu JavaFX ScrollPane
- Die Anleitung zu JavaFX WebView und WebEngine
- Die Anleitung zu JavaFX HTMLEditor
- Die Anleitung zu JavaFX TableView
- Die Anleitung zu JavaFX TreeView
- Die Anleitung zu JavaFX TreeTableView
- Die Anleitung zu JavaFX Menu
- Die Anleitung zu JavaFX ContextMenu
- Die Anleitung zu JavaFX Image und ImageView
- Die Anleitung zu JavaFX Label
- Die Anleitung zu JavaFX Hyperlink
- Die Anleitung zu JavaFX Button
- Die Anleitung zu JavaFX ToggleButton
- Die Anleitung zu JavaFX RadioButton
- Die Anleitung zu JavaFX MenuButton und SplitMenuButton
- Die Anleitung zu JavaFX TextField
- Die Anleitung zu JavaFX PasswordField
- Die Anleitung zu JavaFX TextArea
- Die Anleitung zu JavaFX Slider
- Die Anleitung zu JavaFX Spinner
- Die Anleitung zu JavaFX ProgressBar und ProgressIndicator
- Die Anleitung zu JavaFX ChoiceBox
- Die Anleitung zu JavaFX Tooltip
- Die Anleitung zu JavaFX DatePicker
- Die Anleitung zu JavaFX ColorPicker
- Die Anleitung zu JavaFX FileChooser und DirectoryChooser
- Die Anleitung zu JavaFX PieChart
- Die Anleitung zu JavaFX AreaChart und StackedAreaChart
- Die Anleitung zu JavaFX BarChart und StackedBarChart
- Die Anleitung zu JavaFX Line
- Die Anleitung zu JavaFX Rectangle und Ellipse
Show More