Die Anleitung zu JavaScript String
1. ECMAScript String
Im ECMAScript ist String ein besonderes Typ. Der Grund ist, dass es in einem Programm regelmässig benutzt wird. Deshalb muss er die Leistung und die Flexibilität haben. Das ist der Grund, warum String beide objektiv und primitiv ist.
String Literal
Sie können ein string literal erstellen, string literal wird in dem Stapel (stack) gelagert, das den weniger Speicherungsraum auffordert und bei der Manipulation billig ist
let aStringLiteral = "Hello World";
string literal werden in einem Common Pool gelagert. Wenn Sie 2 Variable String Literal mit der gleichen Inhalt anmelden, zeigen Sie auf eine in dem Pool gelagerte gleiche Addresse.
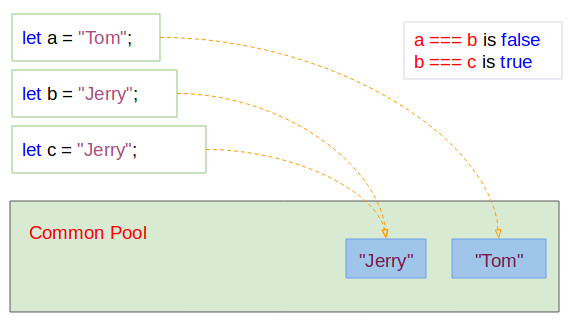
string-literal-example.js
let a = "Tom";
let b = "Jerry";
let c = "Jerry";
console.log(a); // Tom
console.log(b); // Jerry
console.log(c); // Jerry
// a, b: Same address?
console.log( a === b); // false
// b, c: Same address?
console.log( b === c); // true
String Object
Sie können durch constructor der Klasse String ein String erstellen. In dieser Situaltion bekommen Sie ein String object .
let myStringObj = new String(value);
Die Parameter:
- value: eine Wert, zu einem String umzuwandeln (convert).
string-object-example.js
let a = new String("Hello World");
console.log(a); // [String: 'Hello World']
let b = new String(true);
console.log(b); // [String: 'true']
let c = new String(100.20);
console.log(c); // [String: '100.2']
Der Operator new erstellt immer in die Speicherung heap eine neue Entität, das erklärt, warum es Sie bei der Verwendung von String Object mehr kostet.
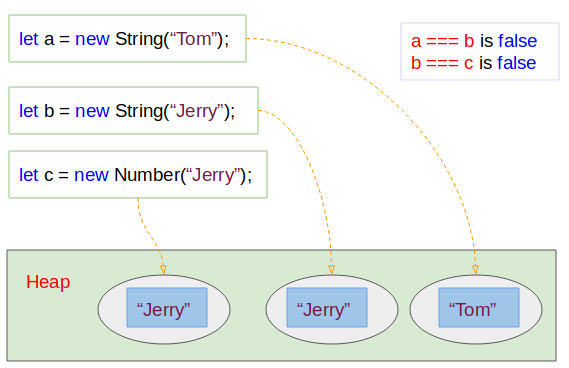
Wenn Sie eine neue Wert die Variable aa für die Variable bb zuweisen, wird die Variable bb auf die Addresse in die Speicherung zeigen, auf die die Variable aa gerade zeigt. In dieser Situation werden keine Entitäte in die Speicherung erstellt.
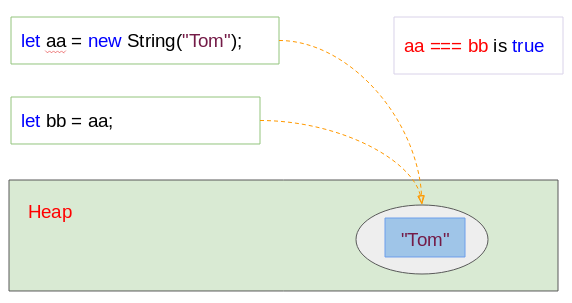
string-object-example2.js
let aa = new String("Tom");
let bb = aa;
// aa, bb: Same address?
var sameAddress = aa === bb;
console.log( sameAddress); // true
String object vs String Literal
Die String Literal werden in Stack gespeichert. Inzwischen werden String Object erstellt und in die Speicherung Heap gespeichert. Es fordert eine komplizierte Speicherung auf und kostet den Speicherungsraum. So sollen Sie String Literal anstatt von String Object irgendwann möglich benutzen.
typeof
Der Operator typeof(string_object) wird das String 'object' zurückgeben, inzwischend typeof(string_literal) gibt das String 'string' zurück:
typeof-example.js
let myStringObj = new String("Tom");
console.log(myStringObj); // [String: 'Tom']
console.log( typeof(myStringObj) ); // object
let myStringLiteral = "Jerry";
console.log(myStringLiteral); // Jerry
console.log( typeof(myStringLiteral) ); // string
Function - String(something)
Die Funktion String(something) wandelt etwas (something) zu einem String Literal um.
String-function-example.js
// String Object:
let s = new String("Hello");
console.log(s); // [String: 'Hello']
// String(value) function:
// Convert String Object to String Literal.
let s2 = String(s);
console.log(s2); // Hello
// A Number Object.
let number = new Number(300.20);
let n2 = String(number);
console.log( n2 ); // 300.2
2. String ist unveränderbar (Immutable)
Im ECMAScript ist das Datentyp String unveränderbar (imutable), d.h Alle Aktionen, die Sie in die String betätigen, wie das String einfügen,das String schneiden... erstellen ein neues String in die Speicherung oder gibt ein neues String zurück.
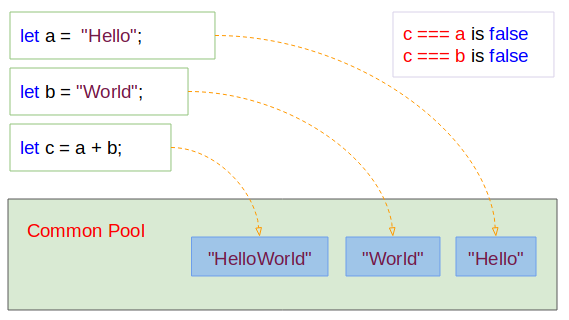
Das Method concat(string) wird benutzt um ein String zu einem momentanen String zu konkatenieren (concatenate) . Tatsächlich ändert das jetzige String aber nicht, sondern wird ein neues String erstellt (das Ergebnis des Konkaktenieren. Die folgende Figur bezeichnet das Verhalten des Programm:
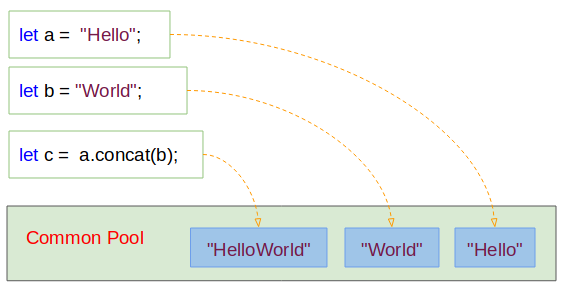
concat-example2.js
let a = "Hello";
let b = "World";
let c = a.concat(b);
console.log(c); // HelloWorld
console.log(a); // Hello
console.log( a === c); // false
3. Die Properties von String
- constructor
- length
- prototype
length
property gibt die Zeichen des String zurück.
length-example.js
let s1 = "Tom";
console.log(s1); // Tom
console.log("Length "+ s1.length); // 3
let s2 = "Tom & Jerry";
console.log(s2); // Tom & Jerry
console.log("Length "+ s2.length); // 11
4. Die Methode von String
charAt(index)
Die Zeichen in die bestimmten Index zurückgeben

charAt-example.js
let str = "This is a String";
console.log("str.charAt(0) is:" + str.charAt(0)); // T
console.log("str.charAt(1) is:" + str.charAt(1)); // h
console.log("str.charAt(2) is:" + str.charAt(2)); // i
console.log("str.charAt(3) is:" + str.charAt(3)); // s
console.log("str.charAt(4) is:" + str.charAt(4)); //
console.log("str.charAt(5) is:" + str.charAt(5)); // i
charCodeAt(index)

charCodeAt-example.js
let str = "This is a String";
console.log("str.charCodeAt(0) is:" + str.charCodeAt(0)); // 84
console.log("str.charCodeAt(1) is:" + str.charCodeAt(1)); // 104
console.log("str.charCodeAt(2) is:" + str.charCodeAt(2)); // 105
console.log("str.charCodeAt(3) is:" + str.charCodeAt(3)); // 115
console.log("str.charCodeAt(4) is:" + str.charCodeAt(4)); // 32
console.log("str.charCodeAt(5) is:" + str.charCodeAt(5)); // 105
concat(string2[,string3[, ..., stringN]]
indexOf(searchValue[, fromIndex])
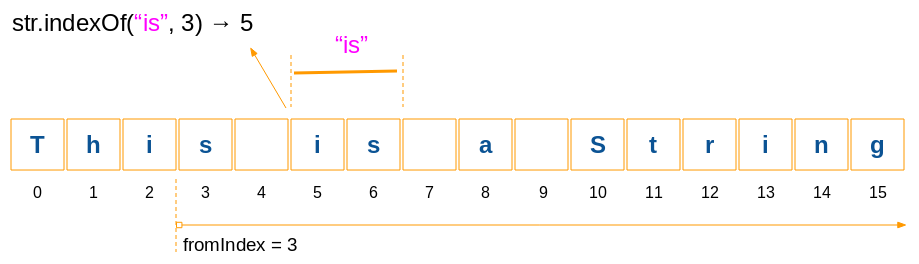
indexOf-example.js
let str = "This is a String";
let idx = str.indexOf("is", 3);
console.log( idx ); // 5
let idx2 = str.indexOf("is");
console.log( idx2 ); // 2
lastIndexOf(searchValue[, toIndex])
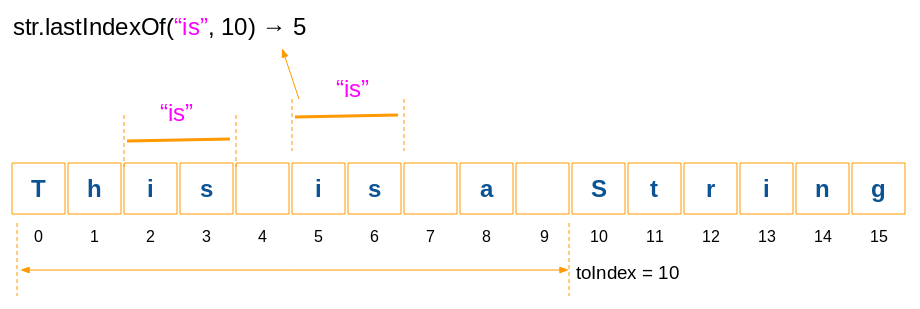
lastIndexOf-example.js
let str = "This is a String";
let idx = str.lastIndexOf("is", 10);
console.log( idx ); // 5
let idx2 = str.lastIndexOf("is", 5);
console.log( idx2 ); // 5
let idx3 = str.lastIndexOf("is", 4);
console.log( idx3 ); // 4
str.localeCompare( otherStr )
Das Method wird benutzt um die Inhalt des momentanen String mit einem anderen String zu vergleichen. Der Zweck ist zu bewerten, welches String vorn steht und welches String hinter steht. Das Method ist sehr nützlich wenn Sie die Reihenfolge der String arrangieren möchten. Der Vergleichgrunsatz basiert nach Locale der Umwelt.
returns:
- Das Method gibt 0 zurück, d.h 2 gleiche String.
- Das Method gibt eine Zahl größer als 0, d.h das momentane String ist größer als (nachfolgen) das andere String.
- Das Method gibt eine Zahl kleiner als 0, d.h das momentane String ist kleiner als (vorangehen) das andere String
localeCompare-example.js
// "A" - "B" - "C" ("A" < "B")
console.log( "A".localeCompare("B") ); // -1
// "B" > "A"
console.log( "B".localeCompare("A") ); // 1
// "Abc" < "bced"
console.log( "Abc".localeCompare("bced") ); // -1
str.replace(regexp/substr, newSubStr/function[, flags]);
Die Parameters
- regexp −Ein Objekt RegExp (reguläre Ausdruck). Das Substring, das der regulären Ausdruck entspricht, wird durch das zurückgegebene Ergebnis des 2.Parameter ersetzt.
- substr − Ein Substring wird durch newSubStr ersetzt.
- newSubStr − Das String wird für den Ersatz der Substring benutzt, die aus dem ersten String bekommen.
- function − Eine Funktion wird aufgeruft um ein neues Substring zu erstellen.
- flags − Ein String enthaltet die attributes, die für reguläre Ausdruck benutzt werden (der 1.Parameter).
Zum Beispiel
replace-example.js
let str = "Years: 2001, 2012, 2018";
// Replace first
let newStr = str.replace("20", "21");
console.log( newStr ); // Years: 2101, 2012, 2018
Zum Beispiel: Die Sub-String, die einer Regulär Ausdruck entsprechen suchen und sie mit einem anderen Substring ersetztn.
replace-example2.js
let str = "Mr Blue has a blue house and a blue car";
// Replace (g: Global/All)
var res = str.replace(/blue/g, "red");
console.log(res);// Mr Blue has a red house and a red car
Z.B: Die Substring, die einer Regulär Ausdruck entsprechen suchen und sie mit eine aus einer Funktion zurückgegebene Wert ersetzen
replace-example3.js
var str = "Mr Blue has a blue house and a blue car";
function myfunc(subStr) {
return subStr.toUpperCase();
}
// Replace (g: Global/All, i: Ignore Case)
var res = str.replace(/blue|house|car/gi, myfunc);
console.log(res); // Mr BLUE has a BLUE HOUSE and a BLUE CAR
str.search(searchValue)
Die Substring-Stelle, die einer Regulär Ausdruck entsprechen, suchen. Wenn nicht gefunden, wird die Wert von -1 zurückgegeben.
Die Parameters:
- searchValue - eine reguläre Ausdruck oder ein String. Wenn es ein String ist, wird es zu einer regulären Ausdruck automatisch umgewandelt.
search-example.js
// g: Global/All, i: Ignore Case
var regex = /apples/gi;
var str = "Apples are round, and apples are juicy.";
let idx = str.search(regex);
console.log(idx); // 0
str.slice( beginIndex [, endIndex] )
Das Method für die Rückgabe eines Substring wird durch 2 Stelle beginIndex und endIndex festgelegt. Wenn die Stelle beginIndex rechts liegt und endIndex links liegt, sind die Rückgabenstring immer leer
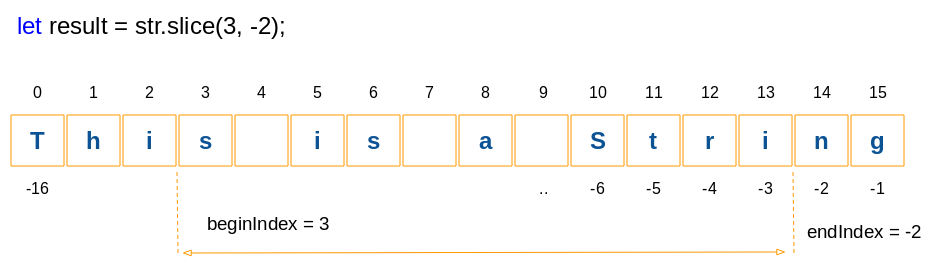
slice-example.js
var str = "This is a String";
var result = str.slice(3, -2);
console.log(result);// s is a Stri
var result2 = str.slice(3);
console.log(result2);// s is a String
// IMPORTANT!!
var result3 = str.slice(5, 1); // ==> Empty String
console.log(result3);//
// IMPORTANT!!
var result4 = str.slice(5, -13); // ==> Empty String
console.log(result4);//
str.substr(start[, length])
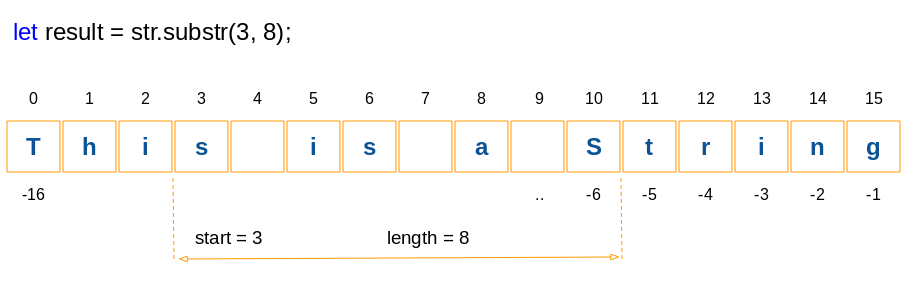
substr-example.js
var str = "This is a String";
var result = str.substr(3, 8);
console.log(result);// s is a S
var result2 = str.substr(3);
console.log(result2);// s is a String
str.substring(beginIndex[, endIndex])
Das Method für die Rückgabe eines Substring wird durch 2 Stelle beginIndex und endIndex festgelegt. Achtung: substring(a, b) & substring(b, a) werden ein gleiches Ergebnis zurückgeben
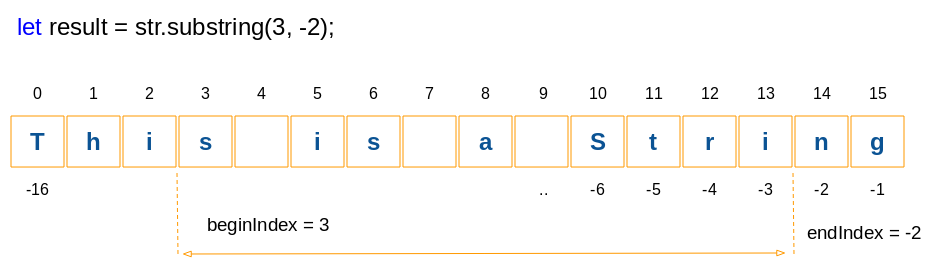
substring-example.js
var str = "This is a String";
var result = str.substring(3, -2);
console.log(result);// s is a Stri
var result2 = str.substring(3);
console.log(result2);// s is a String
// IMPORTANT:
// result3 same as result4:
var result3 = str.substring(4, 1);
var result4 = str.substring(1, 4);
console.log(result3);// his
console.log(result4);// his
toLocaleLowerCase()
toLocaleLowerCase-example.js
let str = "This is a String";
var result = str.toLocaleLowerCase( );
console.log( result ); // this is a string
toLocaleupperCase()
toLocaleUpperCase-example.js
let str = "This is a String";
var result = str.toLocaleUpperCase( );
console.log( result ); // THIS IS A STRING
toLowerCase()
let str = "This is a String";
var result = str.toLowerCase( );
console.log( result ); // this is a string
toUpperCase()
toUpperCase-example.js
let str = "This is a String";
var result = str.toUpperCase( );
console.log( result ); // THIS IS A STRING
valueOf()
Das Method gibt String literal von String object zurück.
valueOf-example.js
let str = new String("This is a String");
console.log( str );// [String: 'This is a String']
let literal = str.valueOf();
console.log( literal );// This is a String
Anleitungen ECMAScript, Javascript
- Einführung in Javascript und ECMAScript
- Schnellstart mit Javascript
- Dialogfeld Alert, Confirm, Prompt in Javascript
- Schnellstart mit JavaScript
- Die Anleitung zu JavaScript Variable
- Bitweise Operationen
- Die Anleitung zu JavaScript Array
- Schleifen in JavaScript
- Die Anleitung zu JavaScript Function
- Die Anleitung zu JavaScript Number
- Die Anleitung zu JavaScript Boolean
- Die Anleitung zu JavaScript String
- if else Anweisung in JavaScript
- Switch Anweisung in JavaScript
- Die Anleitung zu JavaScript Error
- Die Anleitung zu JavaScript Date
- Die Anleitung zu JavaScript Module
- Die Geschichte der Module in JavaScript
- Die Funktionen setTimeout und setInterval in JavaScript
- Die Anleitung zu Javascript Form Validation
- Die Anleitung zu JavaScript Web Cookie
- Schlüsselwort void in JavaScript
- Klassen und Objekte in JavaScript
- Klasse und Vererbung Simulationstechniken in JavaScript
- Vererbung und Polymorphismus in JavaScript
- Das Verständnis über Duck Typing in JavaScript
- Die Anleitung zu JavaScript Symbol
- Die Anleitung zu JavaScript Set Collection
- Die Anleitung zu JavaScript Map Collection
- Das Verständnis über JavaScript Iterable und Iterator
- Die Anleitung zu JavaScript Reguläre Ausdrücke
- Die Anleitung zu JavaScript Promise, Async Await
- Die Anleitung zu Javascript Window
- Die Anleitung zu Javascript Console
- Die Anleitung zu Javascript Screen
- Die Anleitung zu Javascript Navigator
- Die Anleitung zu Javascript Geolocation API
- Die Anleitung zu Javascript Location
- Die Anleitung zu Javascript History API
- Die Anleitung zu Javascript Statusbar
- Die Anleitung zu Javascript Locationbar
- Die Anleitung zu Javascript Scrollbars
- Die Anleitung zu Javascript Menubar
- Die Anleitung zu Javascript JSON
- Ereignisbehandlung in JavaScript
- Die Anleitung zu Javascript MouseEvent
- Die Anleitung zu Javascript WheelEvent
- Die Anleitung zu Javascript KeyboardEvent
- Die Anleitung zu Javascript FocusEvent
- Die Anleitung zu Javascript InputEvent
- Die Anleitung zu Javascript ChangeEvent
- Die Anleitung zu Javascript DragEvent
- Die Anleitung zu Javascript HashChangeEvent
- Die Anleitung zu Javascript URL Encoding
- Die Anleitung zu Javascript FileReader
- Die Anleitung zu Javascript XMLHttpRequest
- Die Anleitung zu Javascript Fetch API
- Analysieren Sie XML in Javascript mit DOMParser
- Einführung in Javascript HTML5 Canvas API
- Hervorhebung Code mit SyntaxHighlighter Javascript-Bibliothek
- Was sind Polyfills in der Programmierwissenschaft?
Show More