Die Anleitung zu JavaScript Promise, Async Await
1. Was ist Promise?
In der PC-Programmierung arbeiten Sie sehr oft mit der Funktionen , und bei der Aufruf einer Funktion bekommen Sie fast sofort das zurückgegebene Ergebnis.
function sum(a, b) {
return a + b;
}
// Call function:
let result = sum(10, 5);
console.log(result);
Angenommen erstellen Sie eine Funktion mit der Name von downloadFile(url) um eine File aus Internet herunterzuladen.
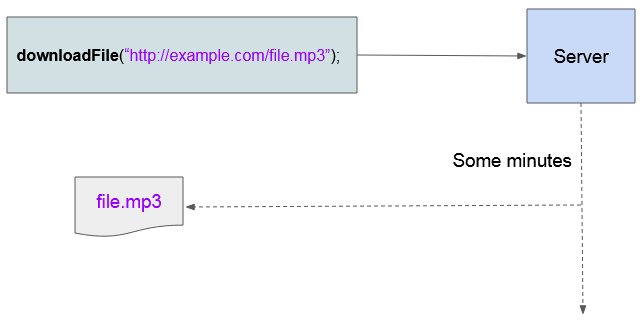
Das Herunterlade einer großeren File kostet einige Minuten oder länger. Bei der synchronousen Aufruf der Funktion downloadFile(url) wird sie alle Manipulationen des Benutzer bis zur Erledigung überwintern, so kann der Benutzer während des Herunterladen der File mit der Applikation nicht manipulieren.
Deshalb können Sie auf eine Funktion wie folgend nicht erwarten:
// Call download:
var myfile = downloadFile("http://example.com/file.mp3");
Promise?
- Die Anwort ist, dass diese Funktion eine Promise (das Versprechen) statt einer File zurückgeben soll.
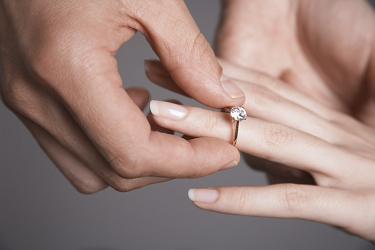
Stellen Sie sich vor, Ihre schöne Freundin sagt Ihnen "Heirate mich bitte!". Okey, natürlich, aber Sie können das sofort nicht tun weil Sie die Zeit für die Vorbereitung brauchen . Aber sie wartet auf Ihre Anwort und es ist am besten, dass Sie auf eine Versprechung warten und sofort sind die beide freundlich.
Promise State?
Eine Promise hat 3 Zustände:
Zustand | Bezeichnung |
Pending | Promise ist im Prozess von Verarbeitung. Sie wissen nicht, ob sie gelingt oder versagt. Zum Beispiel, die File wird heruntergeladet.. |
Fulfilled | Die Aufgabe der Promise erledigt. Z.B die File wird erfolgreich heruntergeladet.. |
Rejected | Die Aufgabe der Promise versagt. Z.B, ein Fehler passiert be idem Herunterladen der File. |
Die Syntax zur Erstellung eines Objekt Promise:
new Promise (
function (resolve, reject) {
// Codes
}
);
Zum Beispiel:
// [ECMAScript 5 Syntax]
var isNetworkOK = true;
// A Promise
var willIGetAFile = new Promise (
function (resolve, reject) {
if (isNetworkOK) {
console.log("Complete the download process!"); // ***
var file = {
fileName: 'file.mp3',
fileContent: '...',
fileSize: '3 MB'
};
resolve(file); // fulfilled
} else {
console.log("File download process failed!"); // ***
var error = new Error('There is a problem with the network.');
reject(error); // reject
}
}
);
Die Funktion downloadFile(url) von Ihnen wird ein Objekt Promise zurückgeben, wie folgend:
// [ECMAScript 5 Syntax]
var isNetworkOK = true;
// This function return a Promise
function downloadFile(url) {
console.log("Start downloading file ..."); // ***
// A Promise
var willIGetAFile = new Promise (
function (resolve, reject) {
if (isNetworkOK) {
console.log("Complete the download process!"); // ***
var file = {
fileName: 'file.mp3',
fileContent: '...',
fileSize: '3 MB'
};
resolve(file); // fulfilled
} else {
console.log("File download process failed!"); // ***
var error = new Error('There is a problem with the network.');
reject(error); // reject
}
}
);
return willIGetAFile; // Return a Promise.
}
2. Promise verwenden
Oben hatten wir die Funktion downloadFile(url),diese Funktion gibt ein Objekt Promise zurück. Jetzt werden wir studieren, wie die Funktion verwendet wird.
// Call downloadFile(..) function:
// Returns a Promise object:
var willIGetAFile = downloadFile("http://example.com/file.mp3");
willIGetAFile
.then(function (fulfilled) {
// Get a File
// Output: {fileName: 'file.mp3', fileContent: '...', fileSize: '3 MB'}
console.log(fulfilled);
})
.catch(function (error) {
// Network Error!
// Output: There is a problem with the network.
console.log(error.message);
});
OK, Das ist die volle Kode des Beispiel:
promise-example.js
// [ECMAScript 5 Syntax]
var isNetworkOK = true;
// This function return a Promise
function downloadFile(url) {
console.log("Start downloading file ..."); // ***
// A Promise
var willIGetAFile = new Promise (
function (resolve, reject) {
if (isNetworkOK) {
console.log("Complete the download process!"); // ***
var file = {
fileName: 'file.mp3',
fileContent: '...',
fileSize: '3 MB'
};
resolve(file); // fulfilled
} else {
console.log("File download process failed!"); // ***
var error = new Error('There is a problem with the network.');
reject(error); // reject
}
}
);
return willIGetAFile; // Return a Promise.
}
// Call downloadFile(..) function:
// Returns a Promise object:
var willIGetAFile = downloadFile("http://example.com/file.mp3");
willIGetAFile
.then(function (fulfilled) {
// Get a File
// Output: {fileName: 'file.mp3', fileContent: '...', fileSize: '3 MB'}
console.log(fulfilled);
})
.catch(function (error) {
// Network Error!
// Output: There is a problem with the network.
console.log(error.message);
});
Wenn isNetworkOK = true, wenn das Beispiel durchgeführt wird, bekommen Sie das Ergebnis:
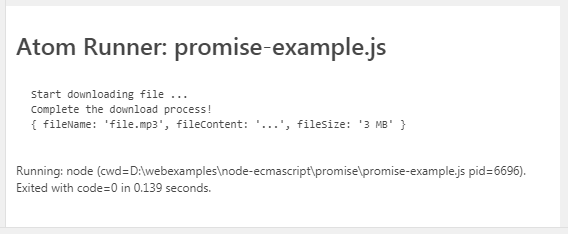
Wenn isNetworkOK = false, wenn das Beispiel durchgeführt wird, bekommen Sie das Ergebnis:
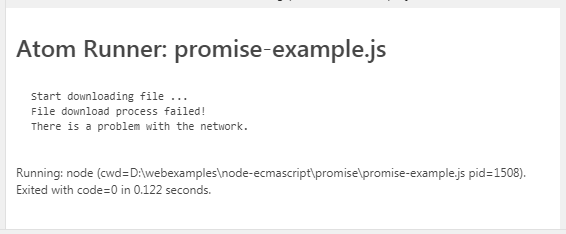
3. Chaining Promise
Chaining Promise (die Versprechenskette) ist eine Reihe aufeinanderfolgender Promise zusammengeführt.
Stellen Sie sich vor, Sie brauchen 2 Manipulationen durchführen, einschließend die Aufruf auf die Funktion downloadFile(url) um eine File aus Internet herunterzuladen, danach die Aufruf auf die Funktion openFile(file) um die heruntergeladete File zu öffnen.

Das Herunterladen einer File aus Internet kostet einen Zeitraum. Die Funktion downloadFile(url) wird entworfen, dass sie Sie ein Versprechen (Promise) zurückgibt. Sie können die File haben zu öffen wenn das Herunterladen erfolgreich ist. Deshalb sollen Sie die Funktion openFile(file) zur Zurückgabe eines Versprechen (Promise) entwerfen.
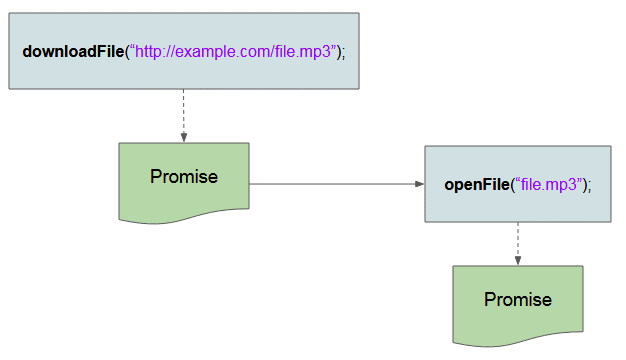
Die Funktion openFile(file) für die Zurückgabe eines Objekt Promise schreiben.
function openFile(file) {
console.log("Start opening file ..."); // ***
var willFileOpen = new Promise(
function (resolve, reject) {
var message = "File " + file.fileName + " opened!"
resolve(message);
}
);
return willFileOpen; // Return a Promise.
}
Statt der Erstellung eines Objekt Promise durch den Operator new können Sie das static Method Promise.resolve(value) oder Promise.reject(error). 2 Methode gibt ein Objekt Promise zurück..
Sie können die Funktion openFile(file) oben kürzer wieder schreiben
// Shorter:
function openFile(file) {
console.log("Start opening file ..."); // ***
var message = "File " + file.fileName + " opened!"
// Create a Promise
var willFileOpen = Promise.resolve(message);
return willFileOpen;
}
Die Aufruf auf die Funktion downloadFile(url) und openFile(file) nach dem Stil Promise
console.log("Start app.."); // ***
// Call downloadFile(..) function:
// Returns a Promise object:
var willIGetAFile = downloadFile("http://example.com/file.mp3");
willIGetAFile
.then(openFile) // Chain it!
.then(function (fulfilled) { // If successful fileOpen.
// Get a message after file opened!
// Output: File file.mp3 opened!
console.log(fulfilled);
})
.catch(function (error) {
// Network Error!
// Output: There is a problem with the network.
console.log(error.message);
});
console.log("End app.."); // ***
Die volle Code des Beispiel sehen:
chaining-promise-example.js
// [ECMAScript 5 Syntax]
var isNetworkOK = true;
// This function return a Promise
function downloadFile(url) {
console.log("Start downloading file ..."); // ***
// A Promise
var willIGetAFile = new Promise (
function (resolve, reject) {
if (isNetworkOK) {
console.log("Complete the download process!"); // ***
var file = {
fileName: 'file.mp3',
fileContent: '...',
fileSize: '3 MB'
};
resolve(file); // fulfilled
} else {
console.log("File download process failed!"); // ***
var error = new Error('There is a problem with the network.');
reject(error); // reject
}
}
);
return willIGetAFile; // Return a Promise.
}
function openFile(file) {
console.log("Start opening file ..."); // ***
var willFileOpen = new Promise(
function (resolve, reject) {
var message = "File " + file.fileName + " opened!"
resolve(message);
}
);
return willFileOpen; // Return a Promise.
}
// Call downloadFile(..) function:
// Returns a Promise object:
var willIGetAFile = downloadFile("http://example.com/file.mp3");
willIGetAFile
.then(openFile) // Chain it!
.then(function (fulfilled) { // If successful fileOpen.
// Get a message after file opened!
// Output: File file.mp3 opened!
console.log(fulfilled);
})
.catch(function (error) {
// Network Error!
// Output: There is a problem with the network.
console.log(error.message);
});
Das Beispiel durchführen und dann das Ergebnis bekommen
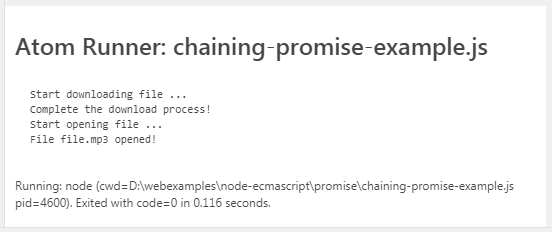
4. Promise ist asynchron
Promise ist asynchron. D.h wenn Sie auf eine Funktion Promise aufrufen (die Funktion gibt eine Promise zurück), wird sie während der Durchführung des Versprechen Ihre Applikation nicht überwintern.
Um das Problem zu erklären, korrigieren Sie das Beispiel oben einbisschen. Die Verwendung der Funktion setTimeout() zur Simulation des Herunterladen kostet einen Zeitraum.
chaining-promise-example-2.js
// [ECMAScript 5 Syntax]
var isNetworkOK = true;
// This function return a Promise
function downloadFile(url) {
console.log("Start downloading file ..."); // ***
// A Promise
var willIGetAFile = new Promise (
function (resolve, reject) {
if (isNetworkOK) {
setTimeout( function() {
console.log("Complete the download process!"); // ***
var file = {
fileName: 'file.mp3',
fileContent: '...',
fileSize: '3 MB'
};
resolve(file); // fulfilled
}, 5 * 1000); // 5 Seconds
} else {
var error = new Error('There is a problem with the network.');
reject(error); // reject
}
}
);
return willIGetAFile; // Return a Promise.
}
function openFile(file) {
console.log("Start opening file ..."); // ***
var willFileOpen = new Promise(
function (resolve, reject) {
var message = "File " + file.fileName + " opened!"
resolve(message);
}
);
return willFileOpen; // Return a Promise.
}
console.log("Start app.."); // ***
// Call downloadFile(..) function:
// Returns a Promise object:
var willIGetAFile = downloadFile("http://example.com/file.mp3");
willIGetAFile
.then(openFile) // Chain it!
.then(function (fulfilled) { // If successful fileOpen.
// Get a message after file opened!
// Output: File file.mp3 opened!
console.log(fulfilled);
})
.catch(function (error) {
// Network Error!
// Output: There is a problem with the network.
console.log(error.message);
});
console.log("End app.."); // ***
Das ist das Ergebnis, das Sie bekommen wenn Sie das Beispiel oben laufen:
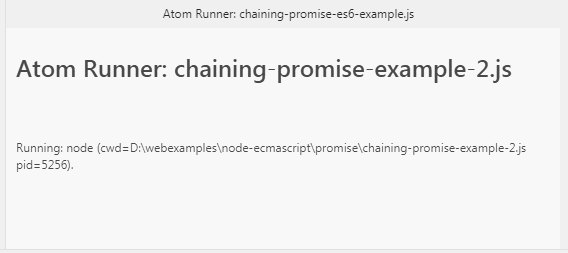
Achten Sie bitte auf die Reihenfolge der Nachrichten, die in dem Bildschirm Console gedruck werden:
Start app..
Start downloading file ...
End app..
Complete the download process!
Start opening file ...
File file.mp3 opened!
5. Promise im ES6
ECMAScript-6 führt die Syntax Pfeil (Narrow Function) ein, so können Sie das Beispiel oben nach der Syntax ES6 wieder schreiben::
chaining-promise-es6-example.js
// [ECMAScript 6 Syntax]
var isNetworkOK = true;
// This function return a Promise
downloadFile = function(url) {
console.log("Start downloading file ..."); // ***
// A Promise
var willIGetAFile = new Promise (
(resolve, reject) => {
if (isNetworkOK) {
setTimeout( function() {
console.log("Complete the download process!"); // ***
var file = {
fileName: 'file.mp3',
fileContent: '...',
fileSize: '3 MB'
};
resolve(file); // fulfilled
}, 5 * 1000); // 5 Seconds
} else {
var error = new Error('There is a problem with the network.');
reject(error); // reject
}
}
);
return willIGetAFile; // Return a Promise.
}
openFile = function (file) {
console.log("Start opening file ..."); // ***
var willFileOpen = new Promise(
(resolve, reject) => {
var message = "File " + file.fileName + " opened!"
resolve(message);
}
);
return willFileOpen; // Return a Promise.
}
console.log("Start app.."); // ***
// Call downloadFile(..) function:
// Returns a Promise object:
var willIGetAFile = downloadFile("http://example.com/file.mp3");
willIGetAFile
.then(openFile) // Chain it!
.then(function (fulfilled) { // If successful fileOpen.
// Get a message after file opened!
// Output: File file.mp3 opened!
console.log(fulfilled);
})
.catch(function (error) {
// Network Error!
// Output: There is a problem with the network.
console.log(error.message);
});
console.log("End app.."); // ***
Sehen Sie die Funktion und die Funktion Pfeil in ECMAScript mehr:
6. ES7 - Async Await
ECMAScript-7 führt die Syntax async und await ein, das bei der leichteren und einfacher-zu-verstehen Verwendung vonPromise hilft
chaining-promise-es7-example.js
// [ECMAScript 7 Syntax]
var isNetworkOK = true;
// An Asynchronous function return a Promise
async function downloadFile(url) {
console.log("Start downloading file ..."); // ***
// A Promise
var willIGetAFile = new Promise (
(resolve, reject) => {
if (isNetworkOK) {
setTimeout( function() {
console.log("Complete the download process!"); // ***
var file = {
fileName: 'file.mp3',
fileContent: '...',
fileSize: '3 MB'
};
resolve(file); // fulfilled
}, 5 * 1000); // 5 Seconds
} else {
var error = new Error('There is a problem with the network.');
reject(error); // reject
}
}
);
return willIGetAFile; // Return a Promise.
}
// An Asynchronous function return a Promise
async function openFile(file) {
console.log("Start opening file ..."); // ***
var willFileOpen = new Promise(
(resolve, reject) => {
var message = "File " + file.fileName + " opened!"
resolve(message);
}
);
return willFileOpen; // Return a Promise.
}
// Main Function (Asynchronous function)
async function mainFunction() {
try {
console.log("Start app.."); // ***
// Call downloadFile(..) function with 'await' keyword:
// It returns a File (Not Promise)
var file = await downloadFile("http://example.com/file.mp3");
console.log(file);
// Call openFile(..) function with 'await' keyword:
// It returns a String (Not Promise)
var message = await openFile(file);
console.log(message);
console.log("End app.."); // ***
} catch(e) {
console.log(e.message);
}
}
// Call Main Function:
(async () => {
await mainFunction();
})();
Anleitungen ECMAScript, Javascript
- Einführung in Javascript und ECMAScript
- Schnellstart mit Javascript
- Dialogfeld Alert, Confirm, Prompt in Javascript
- Schnellstart mit JavaScript
- Die Anleitung zu JavaScript Variable
- Bitweise Operationen
- Die Anleitung zu JavaScript Array
- Schleifen in JavaScript
- Die Anleitung zu JavaScript Function
- Die Anleitung zu JavaScript Number
- Die Anleitung zu JavaScript Boolean
- Die Anleitung zu JavaScript String
- if else Anweisung in JavaScript
- Switch Anweisung in JavaScript
- Die Anleitung zu JavaScript Error
- Die Anleitung zu JavaScript Date
- Die Anleitung zu JavaScript Module
- Die Geschichte der Module in JavaScript
- Die Funktionen setTimeout und setInterval in JavaScript
- Die Anleitung zu Javascript Form Validation
- Die Anleitung zu JavaScript Web Cookie
- Schlüsselwort void in JavaScript
- Klassen und Objekte in JavaScript
- Klasse und Vererbung Simulationstechniken in JavaScript
- Vererbung und Polymorphismus in JavaScript
- Das Verständnis über Duck Typing in JavaScript
- Die Anleitung zu JavaScript Symbol
- Die Anleitung zu JavaScript Set Collection
- Die Anleitung zu JavaScript Map Collection
- Das Verständnis über JavaScript Iterable und Iterator
- Die Anleitung zu JavaScript Reguläre Ausdrücke
- Die Anleitung zu JavaScript Promise, Async Await
- Die Anleitung zu Javascript Window
- Die Anleitung zu Javascript Console
- Die Anleitung zu Javascript Screen
- Die Anleitung zu Javascript Navigator
- Die Anleitung zu Javascript Geolocation API
- Die Anleitung zu Javascript Location
- Die Anleitung zu Javascript History API
- Die Anleitung zu Javascript Statusbar
- Die Anleitung zu Javascript Locationbar
- Die Anleitung zu Javascript Scrollbars
- Die Anleitung zu Javascript Menubar
- Die Anleitung zu Javascript JSON
- Ereignisbehandlung in JavaScript
- Die Anleitung zu Javascript MouseEvent
- Die Anleitung zu Javascript WheelEvent
- Die Anleitung zu Javascript KeyboardEvent
- Die Anleitung zu Javascript FocusEvent
- Die Anleitung zu Javascript InputEvent
- Die Anleitung zu Javascript ChangeEvent
- Die Anleitung zu Javascript DragEvent
- Die Anleitung zu Javascript HashChangeEvent
- Die Anleitung zu Javascript URL Encoding
- Die Anleitung zu Javascript FileReader
- Die Anleitung zu Javascript XMLHttpRequest
- Die Anleitung zu Javascript Fetch API
- Analysieren Sie XML in Javascript mit DOMParser
- Einführung in Javascript HTML5 Canvas API
- Hervorhebung Code mit SyntaxHighlighter Javascript-Bibliothek
- Was sind Polyfills in der Programmierwissenschaft?
Show More