Klassen und Objekte in JavaScript
1. Ihre erste Klasse
ECMAScript 5 hat kein expliziter Begriff über die Klasse , stattdessen simuliert es eine Klasse nach den 2 Begriffe function & prototype. Denn ES5 wurd im Jahr 2011 eingeführt. Bisher ist seine Syntax sehr populär. Allerdings können wir nicht bestreiten, dass die Erstellung einer Klasse in den solche Weg dem Programmer schwer zu verstehen ist. Inzwischen haben die Klasse wie Java, C#,.. den mehr moderne Weg zur Erstellung einer Klasse. Die Version ES6 im 2015 löst rechzeitig dieses Problem. Sie gibt eine moderne Version um eine Klasse zu definieren.
Die neue Syntax ECMAScript 6 hilft dem Programmer bei der Verringerung der Komplizität in die Erstellung einer Klasse und in die Erstellung einer Sub-Klasse. In die technische Seite gibt es keinen Unterschied im Vergleich von der vorherigen Version.
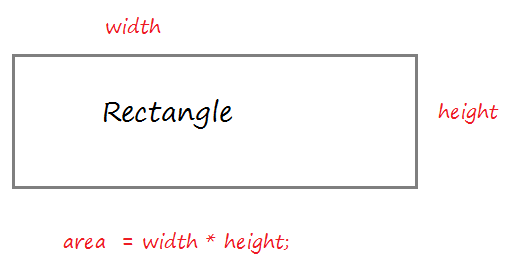
// Define a class.
class Rectangle {
// Ein Constructor hat 2 Parameter.
// (die werden benutzt um das Objekt zu erstellen)
// this.width zeigt nach property width der Klasse.
constructor (width = 5 , height = 10) {
this.width = width;
this.height = height;
}
// Das Method ist benutzt um die Oberfläche des Rechsteck zu rechnen.
getArea() {
var area = this.width * this.height
return area
}
}
// Ein Objekt der Klasse Rectangle durch Constructor erstellen.
var rect = new Rectangle(3, 5);
console.log("Height "+ rect.height);
console.log("Width "+ rect.width);
// Das Method aufrufen
let area = rect.getArea();
console.log("Area "+ area );
Height 5
Width 3
Area 15
Was passiert wenn Sie ein Objekt aus constructor der Klasse erstellen?
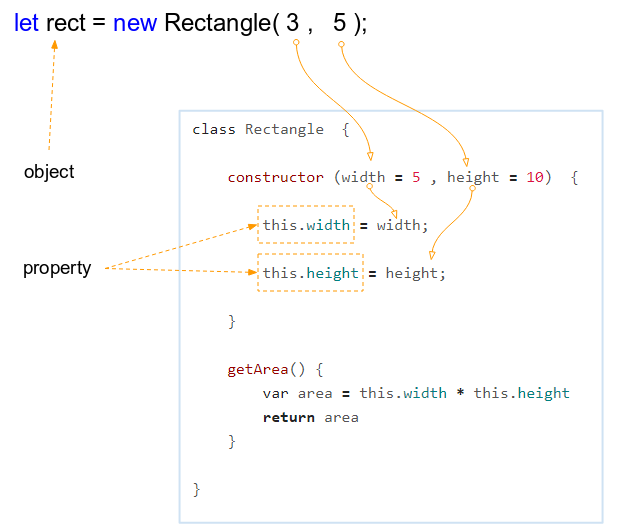
// width = 3, height = 5
let rect = new Rectangle(3, 5);
// width = 15, height = 10 (Default)
let rect2 = new Rectangle(15);
// width = 5 (Default), height = 50
let rect3 = new Rectangle(undefined, 50);
// width = 5 (Default), height = 10 (Default)
let rect4 = new Rectangle();
2. Getter & Setter
Angenommen, wir haben eine Klasse Person, die Klasse hat ein property name.
class Person {
constructor(name) {
this.name = name;
}
}
// Create an object
let person = new Person("Tom");
// Access to property name.
console.log( person.name); // Tom
// Assign new value to property name.
person.name = "Jerry"; // !!!
console.log( person.name ); // Jerry
class Person {
constructor (name) {
// property: __name
this.__name = name;
}
// Getter of property name
get name() {
console.log("Call getter of property 'name'!!");
return this.__name;
}
}
// ------------ TEST -----------------
let person = new Person("Tom");
// Access to property 'name' ==> Call getter
console.log( person.name); // Tom
// Assign new value to property name.
person.name = "Jerry"; // Not Working!!!!
// Access to property 'name' ==> Call getter
console.log( person.name); // Tom
class Person {
constructor (name) {
// property: __name
this.__name = name;
}
// Setter of property name
set name(newName) {
console.log("Call setter of property 'name'!!");
this.__name = newName;
}
// A method
showInfo() {
console.log("Person: " + this.__name);
}
}
// ------------ TEST -----------------
let person = new Person("Tom");
// Can not access to property 'name'
console.log( person.name); // undefined
// Set new value to property 'name' ==> Call setter
person.name = "Jerry";
person.showInfo(); // Person: Tom
class Rectangle {
constructor (width = 5 , height = 10) {
this.__width = width;
this.height = height;
}
// Getter of property 'width'
get width() {
return this.__width;
}
// Setter of property 'width'
set width(newWidth) {
if(newWidth > 0) {
this.__width = newWidth;
} else {
console.log("Invalid width " + newWidth);
}
}
}
// ------------ TEST ------------------
var rect = new Rectangle(3, 5);
console.log("Height "+ rect.height); // Height: 5
console.log("Width "+ rect.width); // Width: 3
rect.width = -100;
console.log("Height "+ rect.height); // Height: 5
console.log("Width "+ rect.width); // Width: 3
rect.width = 100;
console.log("Height "+ rect.height); // Height: 5
console.log("Width "+ rect.width); // Width: 100
Height 5
Width 3
Invalid width -100
Height 5
Width 3
Height 5
Width 100
Die property mit der Präfix 2 Unterstrichen ( __ ) werden oft durch die Programmer vereinbart, außerhalb der Klassen nicht zu benutzen. Allerdings kann diese Vereinbarung gebrochen werden. Und deshalb sind diese solchen Property gefährlich..
class Something {
constructor(){
this.#someProperty = "test";
}
}
const instance = new Something();
console.log(instance.#someProperty); // undefined
class Person {
constructor (name) {
// Private property: #name
this.#name = name;
}
// Getter of property name
get name() {
console.log("Call getter of property 'name'!!");
return this.#name;
}
}
3. Static Field
Die static Felder (static field) mit der stabilen Wert (unveränderbar) werden constant static field genannt.
class Employee {
constructor (fullName, age) {
this.fullName = fullName;
if(age < Employee.MIN_AGE || age > Employee.MAX_AGE) {
throw "Invalid Age " + age;
}
this.age = age;
}
// A static field: MIN_AGE
static get MIN_AGE() {
return 18;
}
// A static field: MAX_AGE
static get MAX_AGE() {
if(!Employee.__MAXA) {
Employee.__MAXA = 60;
}
return Employee.__MAXA;
}
static set MAX_AGE(newMaxAge) {
Employee.__MAXA = newMaxAge;
}
}
// ---- TEST ---------
console.log("Mininum Age Allowed: " + Employee.MIN_AGE);
console.log("Maximum Age Allowed: " + Employee.MAX_AGE);
// Set new Maximum Age:
Employee.MAX_AGE = 65;
console.log("Maximum Age Allowed: " + Employee.MAX_AGE);
let baby = new Employee("Some Baby", 1); // Error!!
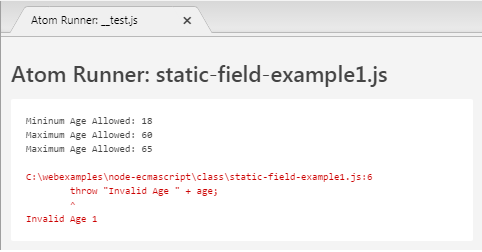
Sie haben eine andere Maßnahme um einen static Feld für die Klasse anzumelden. Allerdings werden die static Felder, die durch diese Maßnahme erstellt werden, kein Konstant ist denn ihre Wert kann ändern. Unter ist es ein Beispiel:
class Employee {
constructor (fullName, age) {
this.fullName = fullName;
if(age < Employee.MIN_AGE || age > Employee.MAX_AGE) {
throw "Invalid Age " + age;
}
this.age = age;
}
}
Employee.MIN_AGE = 18;
Employee.MAX_AGE = 60;
// ---- TEST ---------
console.log("Mininum Age Allowed: " + Employee.MIN_AGE);
console.log("Maximum Age Allowed: " + Employee.MAX_AGE);
// Set new Maximum Age:
Employee.MAX_AGE = 65;
console.log("Maximum Age Allowed: " + Employee.MAX_AGE);
let baby = new Employee("Some Baby", 1); // Error!!
4. Das static Method
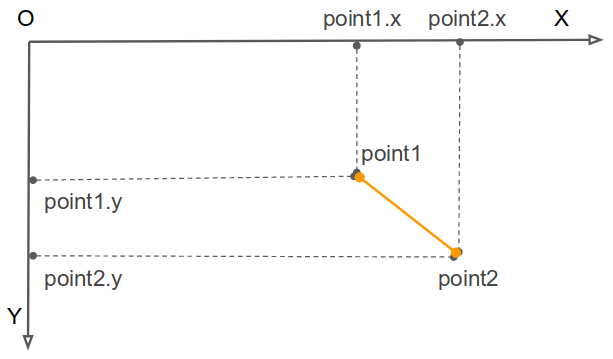
class Point {
constructor(x, y) {
this.x = x;
this.y = y;
}
// Den Abstanz zwischen 2 Punkte rechnen
static distance( point1, point2) {
const dx = point1.x - point2.x;
const dy = point1.y - point2.y;
return Math.hypot(dx, dy);
}
}
// --- TEST ---
let point1 = new Point( 5, 10);
let point2 = new Point( 15, 20);
// Distance
let d = Point.distance(point1, point2);
console.log("Distance between 2 points: " + d);
Distance between 2 points: 14.142135623730951
5. Der Operator zum Vergleich des Objekt
Ein Operator für die Zuweisung eines Objekt AA durch ein Objekt BB erstellt keine Enitität in der Speicherung mehr. Er zeigt auf die Address von AA nach der Addresse von BB
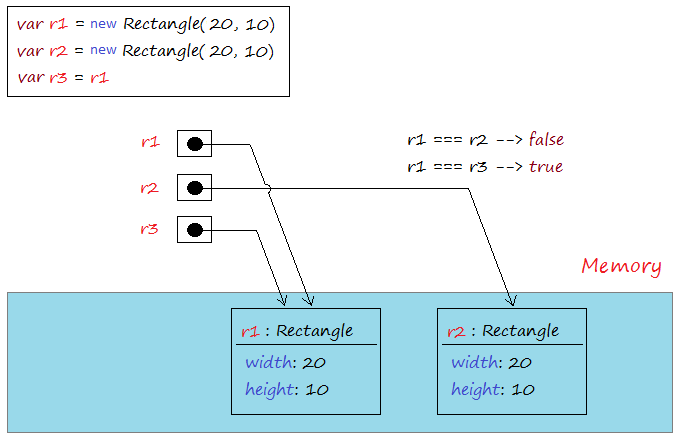
// Define a class.
class Rectangle {
constructor (width = 5 , height = 10) {
this.width = width;
this.height = height;
}
getArea() {
var area = this.width * this.height;
return area;
}
}
// Create object: r1
let r1 = new Rectangle( 20, 10);
// Create object: r2
let r2 = new Rectangle( 20, 10);
let r3 = r1;
let b12 = r1 === r2; // false
let b13 = r1 === r3; // true
console.log("r1 === r2 ? " + b12); // false
console.log("r1 === r3 ? " + b13); // true
var bb12 = r1 !== r2; // true
var bb13 = r1 !== r3; // false
console.log("r1 !== r2 ? " + bb12); // true
console.log("r1 !== r3 ? " + bb13); // false
r1 === r2 ? false
r1 === r3 ? true
r1 !== r2 ? true
r1 !== r3 ? false
Anleitungen ECMAScript, Javascript
- Einführung in Javascript und ECMAScript
- Schnellstart mit Javascript
- Dialogfeld Alert, Confirm, Prompt in Javascript
- Schnellstart mit JavaScript
- Die Anleitung zu JavaScript Variable
- Bitweise Operationen
- Die Anleitung zu JavaScript Array
- Schleifen in JavaScript
- Die Anleitung zu JavaScript Function
- Die Anleitung zu JavaScript Number
- Die Anleitung zu JavaScript Boolean
- Die Anleitung zu JavaScript String
- if else Anweisung in JavaScript
- Switch Anweisung in JavaScript
- Die Anleitung zu JavaScript Error
- Die Anleitung zu JavaScript Date
- Die Anleitung zu JavaScript Module
- Die Geschichte der Module in JavaScript
- Die Funktionen setTimeout und setInterval in JavaScript
- Die Anleitung zu Javascript Form Validation
- Die Anleitung zu JavaScript Web Cookie
- Schlüsselwort void in JavaScript
- Klassen und Objekte in JavaScript
- Klasse und Vererbung Simulationstechniken in JavaScript
- Vererbung und Polymorphismus in JavaScript
- Das Verständnis über Duck Typing in JavaScript
- Die Anleitung zu JavaScript Symbol
- Die Anleitung zu JavaScript Set Collection
- Die Anleitung zu JavaScript Map Collection
- Das Verständnis über JavaScript Iterable und Iterator
- Die Anleitung zu JavaScript Reguläre Ausdrücke
- Die Anleitung zu JavaScript Promise, Async Await
- Die Anleitung zu Javascript Window
- Die Anleitung zu Javascript Console
- Die Anleitung zu Javascript Screen
- Die Anleitung zu Javascript Navigator
- Die Anleitung zu Javascript Geolocation API
- Die Anleitung zu Javascript Location
- Die Anleitung zu Javascript History API
- Die Anleitung zu Javascript Statusbar
- Die Anleitung zu Javascript Locationbar
- Die Anleitung zu Javascript Scrollbars
- Die Anleitung zu Javascript Menubar
- Die Anleitung zu Javascript JSON
- Ereignisbehandlung in JavaScript
- Die Anleitung zu Javascript MouseEvent
- Die Anleitung zu Javascript WheelEvent
- Die Anleitung zu Javascript KeyboardEvent
- Die Anleitung zu Javascript FocusEvent
- Die Anleitung zu Javascript InputEvent
- Die Anleitung zu Javascript ChangeEvent
- Die Anleitung zu Javascript DragEvent
- Die Anleitung zu Javascript HashChangeEvent
- Die Anleitung zu Javascript URL Encoding
- Die Anleitung zu Javascript FileReader
- Die Anleitung zu Javascript XMLHttpRequest
- Die Anleitung zu Javascript Fetch API
- Analysieren Sie XML in Javascript mit DOMParser
- Einführung in Javascript HTML5 Canvas API
- Hervorhebung Code mit SyntaxHighlighter Javascript-Bibliothek
- Was sind Polyfills in der Programmierwissenschaft?