Die Anleitung zu Javascript Scrollbars
1. window.scrollbars
Das Property window.scrollbars gibt ein Objekt Scrollbars für die Vertretung der Scroll Bar zurück, das Viewport des Browser umgeben. Allerdings können Sie mit Scrollbars durch Javascript fast nicht inteaktivieren denn es gibt wenige API für Sie.
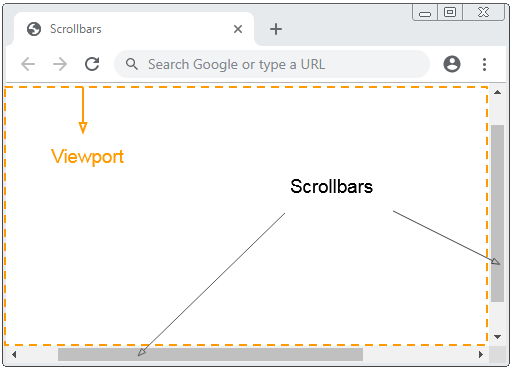
window.scrollbars
// Or Simple:
scrollbars
scrollbars.visible
Das Objekt Scrollbars hat ein einzige Property wie visible. Aber das Property ist unvertraulich, gibt scrollbars.visible die Wert true zurück. Das heißt nicht, Sie die Scroll Bar sehen.
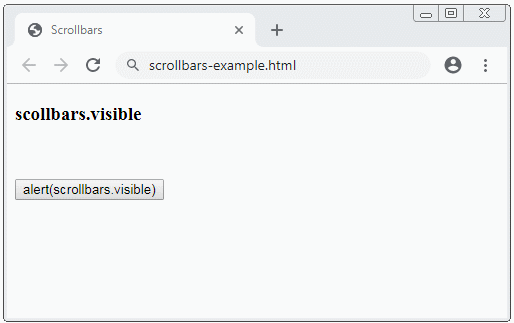
scrollbars-example.html
<!DOCTYPE html>
<html>
<head>
<title>Scrollbars</title>
<meta charset="UTF-8">
</head>
<body>
<h3>scollbars.visible</h3>
<br/><br/>
<button onclick="alert(scrollbars.visible)">
alert(scrollbars.visible)
</button>
</body>
</html>
Example:
Z.B: Verwenden Sie das Method window.open(..) um ein neues Fenster ohne das Scroll bar zu öffnen:
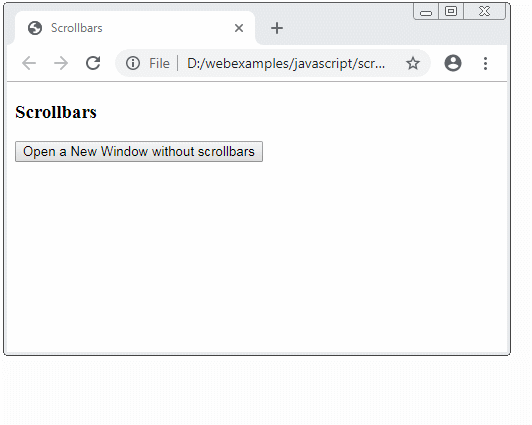
open-new-window-example.html
<!DOCTYPE html>
<html>
<head>
<title>Scrollbars</title>
<meta charset="UTF-8">
<script>
function openNewWindow() {
var winFeature = 'scrollbars=no,resizable=yes';
// Open a New Windows.
window.open('some-page.html','MyWinName',winFeature);
}
</script>
</head>
<body>
<h3>Scrollbars</h3>
<button onclick="openNewWindow()">
Open a New Window without scrollbars
</button>
</body>
</html>
some-page.html
<!DOCTYPE html>
<html>
<head>
<title>Some Page</title>
<meta charset="UTF-8">
<style>
/** CHROME: Make the scroll bar not appear */
body {
overflow: hidden;
}
</style>
</head>
<body>
<h3>Some Page</h3>
<p>1</p>
<p>1 2</p>
<p>1 2 3</p>
<p>1 2 3 4</p>
<p>1 2 3 4 5</p>
<p>1 2 3 4 5 6</p>
<p>1 2 3 4 5 6 7</p>
</body>
</html>
Example:
Zum Beispiel: Was machen Sie damit die Scroll Bar niemals anzeigt?
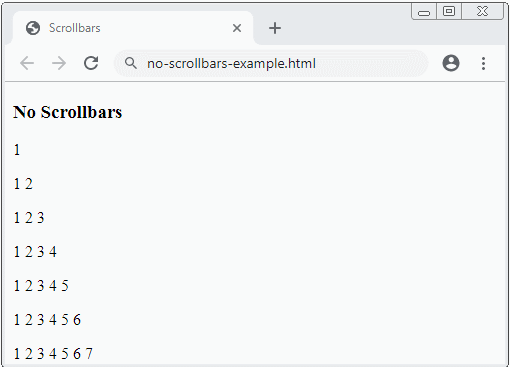
no-scrollbars-example.html
<!DOCTYPE html>
<html>
<head>
<title>Scrollbars</title>
<meta charset="UTF-8">
<style>
body {
overflow: hidden;
}
</style>
</head>
<body>
<h3>No Scrollbars</h3>
<p>1</p>
<p>1 2</p>
<p>1 2 3</p>
<p>1 2 3 4</p>
<p>1 2 3 4 5</p>
<p>1 2 3 4 5 6</p>
<p>1 2 3 4 5 6 7</p>
</body>
</html>
2. Die Scroll Bar entdecken
Manchmal möchten Sie entdecken, ob das Scroll Bar eines Element anzeigt oder nicht? Oder Sie möchten entdecken, ob das Scroll Bar von Viewport anzeigt oder nicht? Unten ist das eine kleine Bibliothek. Es ist Ihnen nützlich für diese Situation.
scrollbars-utils.js
// Private Function
// @axis: 'X', 'Y', 'x', 'y'
function __isScrollbarShowing__(domNode, axis, computedStyles) {
axis = axis.toUpperCase();
var type;
if(axis === 'Y') {
type = 'Height';
} else {
type = 'Width';
}
var scrollType = 'scroll' + type;
var clientType = 'client' + type;
var overflowAxis = 'overflow' + axis;
var hasScroll = domNode[scrollType] > domNode[clientType];
// Check the overflow and overflowY properties for "auto" and "visible" values
var cStyle = computedStyles || window.getComputedStyle(domNode)
return hasScroll && (cStyle[overflowAxis] == "visible"
|| cStyle[overflowAxis] == "auto"
)
|| cStyle[overflowAxis] == "scroll";
}
// @domNode: Optional.
function isScrollbarXShowing(domNode) {
if(!domNode) {
domNode = document.documentElement;
}
return __isScrollbarShowing__(domNode, 'x');
}
// @domNode: Optional.
function isScrollbarYShowing(domNode) {
if(!domNode) {
domNode = document.documentElement;
}
return __isScrollbarShowing__(domNode, 'y');
}
// Scrollbar X or Scrollbar Y is showing?
// @domNode: Optional.
function isScrollbarShowing(domNode) {
return isScrollbarXShowing(domNode) || isScrollbarYShowing(domNode);
}
Zum Beispiel: Verwenden Sie die Bibliothek oben um das Scroll Bar von Viewport zu entdecken.
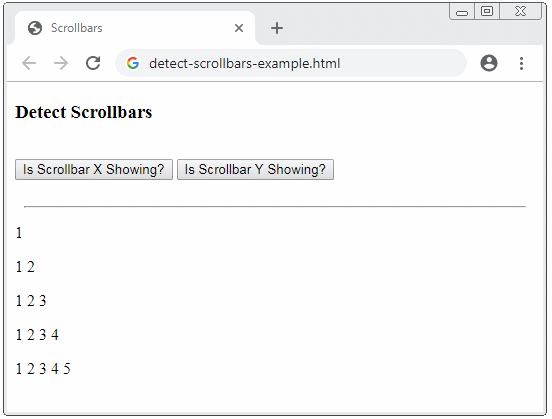
detect-scollbars-example.html
<!DOCTYPE html>
<html>
<head>
<title>Scrollbars</title>
<meta charset="UTF-8">
<script src="scrollbars-utils.js"></script>
</head>
<body>
<h3>Detect Scrollbars</h3>
<br/>
<button onclick="alert(isScrollbarXShowing())">
Is Scrollbar X Showing?
</button>
<button onclick="alert(isScrollbarYShowing())">
Is Scrollbar Y Showing?
</button>
<br/><br/>
<hr style="width: 500px;"/>
<p>1</p>
<p>1 2</p>
<p>1 2 3</p>
<p>1 2 3 4</p>
<p>1 2 3 4 5</p>
</body>
</html>
Zum Beispiel: Entdecken Sie das Scroll Bar des Element <div>:
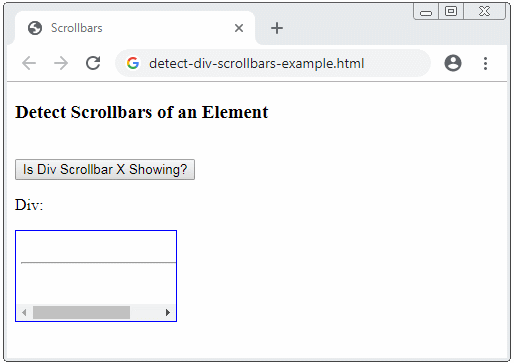
detect-div-scrollbars-example.html
<!DOCTYPE html>
<html>
<head>
<title>Scrollbars</title>
<meta charset="UTF-8">
<style>
#my-div {
border: 1px solid blue;
overflow-x: auto;
padding: 5px;
width: 150px;
height: 80px;
}
</style>
<script src="scrollbars-utils.js"></script>
<script>
function detectScrollbarXOfDiv() {
var domNode = document.getElementById("my-div");
var scX = isScrollbarXShowing(domNode);
var scY = isScrollbarYShowing(domNode);
alert("Is Div Scrollbar X Showing? " + scX);
}
</script>
</head>
<body>
<h3>Detect Scrollbars of an Element</h3>
<br/>
<button onclick="detectScrollbarXOfDiv()">
Is Div Scrollbar X Showing?
</button>
<p>Div:</p>
<div id="my-div">
<br>
<hr style="width:200px"/>
<br>
</div>
</body>
</html>
Anleitungen ECMAScript, Javascript
- Einführung in Javascript und ECMAScript
- Schnellstart mit Javascript
- Dialogfeld Alert, Confirm, Prompt in Javascript
- Schnellstart mit JavaScript
- Die Anleitung zu JavaScript Variable
- Bitweise Operationen
- Die Anleitung zu JavaScript Array
- Schleifen in JavaScript
- Die Anleitung zu JavaScript Function
- Die Anleitung zu JavaScript Number
- Die Anleitung zu JavaScript Boolean
- Die Anleitung zu JavaScript String
- if else Anweisung in JavaScript
- Switch Anweisung in JavaScript
- Die Anleitung zu JavaScript Error
- Die Anleitung zu JavaScript Date
- Die Anleitung zu JavaScript Module
- Die Geschichte der Module in JavaScript
- Die Funktionen setTimeout und setInterval in JavaScript
- Die Anleitung zu Javascript Form Validation
- Die Anleitung zu JavaScript Web Cookie
- Schlüsselwort void in JavaScript
- Klassen und Objekte in JavaScript
- Klasse und Vererbung Simulationstechniken in JavaScript
- Vererbung und Polymorphismus in JavaScript
- Das Verständnis über Duck Typing in JavaScript
- Die Anleitung zu JavaScript Symbol
- Die Anleitung zu JavaScript Set Collection
- Die Anleitung zu JavaScript Map Collection
- Das Verständnis über JavaScript Iterable und Iterator
- Die Anleitung zu JavaScript Reguläre Ausdrücke
- Die Anleitung zu JavaScript Promise, Async Await
- Die Anleitung zu Javascript Window
- Die Anleitung zu Javascript Console
- Die Anleitung zu Javascript Screen
- Die Anleitung zu Javascript Navigator
- Die Anleitung zu Javascript Geolocation API
- Die Anleitung zu Javascript Location
- Die Anleitung zu Javascript History API
- Die Anleitung zu Javascript Statusbar
- Die Anleitung zu Javascript Locationbar
- Die Anleitung zu Javascript Scrollbars
- Die Anleitung zu Javascript Menubar
- Die Anleitung zu Javascript JSON
- Ereignisbehandlung in JavaScript
- Die Anleitung zu Javascript MouseEvent
- Die Anleitung zu Javascript WheelEvent
- Die Anleitung zu Javascript KeyboardEvent
- Die Anleitung zu Javascript FocusEvent
- Die Anleitung zu Javascript InputEvent
- Die Anleitung zu Javascript ChangeEvent
- Die Anleitung zu Javascript DragEvent
- Die Anleitung zu Javascript HashChangeEvent
- Die Anleitung zu Javascript URL Encoding
- Die Anleitung zu Javascript FileReader
- Die Anleitung zu Javascript XMLHttpRequest
- Die Anleitung zu Javascript Fetch API
- Analysieren Sie XML in Javascript mit DOMParser
- Einführung in Javascript HTML5 Canvas API
- Hervorhebung Code mit SyntaxHighlighter Javascript-Bibliothek
- Was sind Polyfills in der Programmierwissenschaft?
Show More