Die Anleitung zu Javascript Fetch API
1. Die Überblich von Fetch API
Sehr regelmäßig brauchen Sie in der Programmierung von Javascript die Daten aus einer URL holen. Sie können an XMLHttpRequest API denken. Es ist wirklich ein API , das Ihnen bei der Schaffung des gewünschten Ding hilft. Aber es ist nicht freundlich. Sie müssen die Kode ziemlich lang schreiben um das Zweck zu erreichen. Das ist eine gute Idee, jQuery zu verwenden. Die Syntax jQuery.ajax(..) ist kürzlich und einfach zu verstehen. Aber Sie müssen die Bibliothek jQuery in Ihre Applikation einfügen.
ES6 wurde im Juni 2015 mit der vielen Funktionen einschließend Promise vorgestellt. Und Fetch API ist ein neues Standard um eine Anforderung nach dem Server zu machen und eine Daten zu holen. Sein Zweck ist ähnlich wie XMLHttpRequest. Der Unterschied ist das, dass Fetch API nach dem Begriff Promise geschrieben wird.
Es ist leichter wenn Sie den Begriff über Promise beherrschen bevor Sie die Unterricht starten. Und nach meiner Meinung sollen Sie meinen folgenden Artikel referenzieren. Er ist ganz verständlich für allen Personnen.
Fetch API baut eine Funktion fetch(url,options) um die Daten aus eine URL zu holen. Diese Funktion gibt eine Promise wie folgend zurück:
function fetch(url, options) {
// A Promise
var aPromise = new Promise (
function (resolve, reject) {
if (allthingOK) {
// ...
var respone = ...;
resolve(response);
} else {
var error = new Error('Error Cause');
reject(error);
}
}
);
return aPromise;
}
Und Fetch API ist einfach zu verwenden. Sie brauchen nur die Funktion fetch(url,options) aufrufen und eine Versprechung zur Lösung des Objekt response bekommen.
var url = "http://example.com/file";
var options = { method : 'GET', .... };
var aPromise = fetch(url, options);
aPromise
.then(function(response) {
})
.catch(function(error) {
});
var aPromise = fetch(url, {
method: "GET", // POST, PUT, DELETE, etc.
headers: {
"Content-Type": "text/plain;charset=UTF-8" // for a string body, depends on body
},
body: undefined // string, FormData, Blob, BufferSource, or URLSearchParams
referrer: "about:client", // "" for no-referrer, or an url from the current origin
referrerPolicy: "no-referrer-when-downgrade", // no-referrer, origin, same-origin...
mode: "cors", // same-origin, no-cors
credentials: "same-origin", // omit, include
cache: "default", // no-store, reload, no-cache, force-cache, or only-if-cached
redirect: "follow", // manual, error
integrity: "", // a hash, like "sha256-abcdef1234567890"
keepalive: false, // true
signal: undefined, // AbortController to abort request
window: window // null
});
2. Get Text - response.text()
Verwenden Sie Fetch API um eine Anforderung zu schicken und eine Text zu bekommen. Das ist eine einfachste und leicht zu verstehen Aufgabe.
This is a simple text data.
Die Funktion fetch(..) gibt eine Versprechung zur Lösung eines Objekt response zurück. Deshalb brauchen Sie den Schritt 1 wie folgend machen um eine Anforderung zur Holung einer Textdaten zu schicken
get-text-example.js (Step 1)
// A URL returns TEXT data.
var url = "https://ex1.o7planning.com/_testdatas_/simple-text-data.txt";
function doGetTEXT() {
// Call fetch(url) with default options.
// It returns a Promise object (Resolve response object)
var aPromise = fetch(url);
// Work with Promise object:
aPromise
.then(function(response) {
console.log("OK! Server returns a response object:");
console.log(response);
})
.catch(function(error) {
console.log("Noooooo! Something error:");
// Network Error!
console.log(error);
});
}
Das Method response.text() gibt eine Versprechung zur Lösung eines Objekt text zurück, so können Sie die Kode für das Beispiel wie folgend schreiben :
get-text-example-way1.js
// A URL returns TEXT data.
var url = "https://ex1.o7planning.com/_testdatas_/simple-text-data.txt";
function doGetTEXT() {
// Call fetch(url) with default options.
// It returns a Promise object (Resolve response object)
var aPromise = fetch(url);
// Work with Promise object:
aPromise
.then(function(response) {
console.log("OK! Server returns a response object:");
console.log(response);
if(!response.ok) {
throw new Error("HTTP error, status = " + response.status);
}
response.text()
.then(function(myText) {
console.log("Text:");
console.log(myText);
})
.catch(function(error) {
// Never happened.
});
})
.catch(function(error) {
console.log("Noooooo! Something error:");
// Network Error!
console.log(error);
});
}
Fetch API wird entworfen damit Sie die Aufgaben in eine Kette durchführen können. Das kann möglich sein denn die Methode promise.then(..), promise.catch(..) werden auch entworfen um eine Versprechung zurückzugeben.
Obwohl die Funktion function(response) , die Sie schreiben, eine normale Wert oder ein Objekt Promise zurückgibt, gibt das Method then() immer eine Promise zurück.
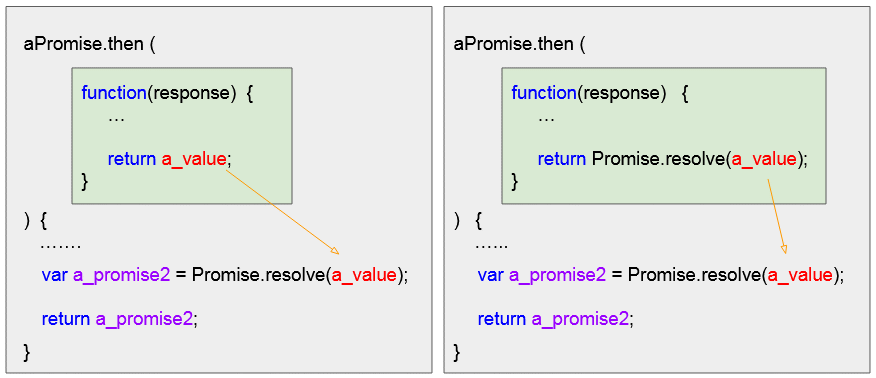
get-text-example-way2.js
// A URL returns TEXT data.
var url = "https://ex1.o7planning.com/_testdatas_/simple-text-data.txt";
function doGetTEXT() {
// Call fetch(url) with default options.
// It returns a Promise object (Resolve response object)
var aPromise = fetch(url);
// Work with Promise object:
aPromise
.then(function(response) {
console.log("OK! Server returns a response object:");
console.log(response);
if(!response.ok) {
throw new Error("HTTP error, status = " + response.status);
}
return response.text();
})
.then(function(myText) {
console.log("Text:");
console.log(myText);
})
.catch(function(error) {
console.log("Noooooo! Something error:");
// Network Error!
console.log(error);
});
}
get-text-example.html
<!DOCTYPE html>
<html>
<head>
<title>fetch get text</title>
<meta charset="UTF-8">
<script src="get-text-example-way2.js"></script>
</head>
<body>
<h3>fetch get text</h3>
<p style="color:red">
Click the button and see the results in the Console.
</p>
<button onclick="doGetJSON()">doGetJSON</button>
</body>
</html>
3. Get JSON - response.json()
Die Verwendung von Fetch API um eine Anforderung zu schicken und ein JSON zu bekommen ist einbißschen komplizierter als die Text zu bekommen. Denn Sie müssen die passierenden Fehler behandeln wenn JSON die ungültige Format oder die Daten NULL hat.
json-simple-data.json
{
"fullName" : "Tom Cat",
"gender" : "Male",
"address" : "Walt Disney"
}
Die Funktion fetch(..) gibt eine Versprechung zur Lösung des Objekt response zurück.
Der Schritt 1 (Step 1): Schreiben Sie Ihre Code einfach wie folgend:
get-json-example.js (Step 1)
// A URL returns JSON data.
var url = "https://ex1.o7planning.com/_testdatas_/json-simple-data.json";
function doGetJSON() {
// Call fetch(url) with default options.
// It returns a Promise object:
var aPromise = fetch(url);
// Work with Promise object:
aPromise
.then(function(response) {
console.log("OK! Server returns a response object:");
console.log(response);
})
.catch(function(error) {
console.log("Noooooo! Something error:");
console.log(error);
});
}
Way 1:
Das Method response.json() gibt eine Versprechung zur Lösung eines Objekt JSON zurück. Deshalb können Sie das Method response.json().then(..) benutzen.
get-json-example_way1.js
// A URL returns JSON data.
var url = "https://ex1.o7planning.com/_testdatas_/json-simple-data.json";
function doGetJSON() {
// Call fetch(url) with default options.
// It returns a Promise object:
var aPromise = fetch(url);
// Work with Promise object:
aPromise
.then(function(response) {
console.log("OK! Server returns a response object:");
console.log(response);
if(!response.ok) {
throw new Error("HTTP error, status = " + response.status);
}
// Get JSON Promise from response object:
var myJSON_promise = response.json();
// Work with Promise object:
myJSON_promise.then(function(myJSON)) {
console.log("OK! JSON:");
console.log(myJSON);
}
})
.catch(function(error) {
console.log("Noooooo! Something error:");
console.log(error);
});
}
Way 2:
get-json-example-way2.js
// A URL returns JSON data.
var url = "https://ex1.o7planning.com/_testdatas_/json-simple-data.json";
function doGetJSON() {
// Call fetch(url) with default options.
// It returns a Promise object:
var aPromise = fetch(url);
// Work with Promise object:
aPromise
.then(function(response) {
console.log("OK! Server returns a response object:");
console.log(response);
if(!response.ok) {
throw new Error("HTTP error, status = " + response.status);
}
// Get JSON Promise from response object:
var myJSON_promise = response.json();
// Returns a Promise object.
return myJSON_promise;
})
.then(function(myJSON) {
console.log("OK! JSON:");
console.log(myJSON);
})
.catch(function(error) {
console.log("Noooooo! Something error:");
console.log(error);
});
}
get-json-example.html
<!DOCTYPE html>
<html>
<head>
<title>fetch get json</title>
<meta charset="UTF-8">
<script src="get-json-example-way2.js"></script>
</head>
<body>
<h3>fetch get json</h3>
<p style="color:red">
Click the button and see the results in the Console.
</p>
<button onclick="doGetJSON()">doGetJSON</button>
</body>
</html>
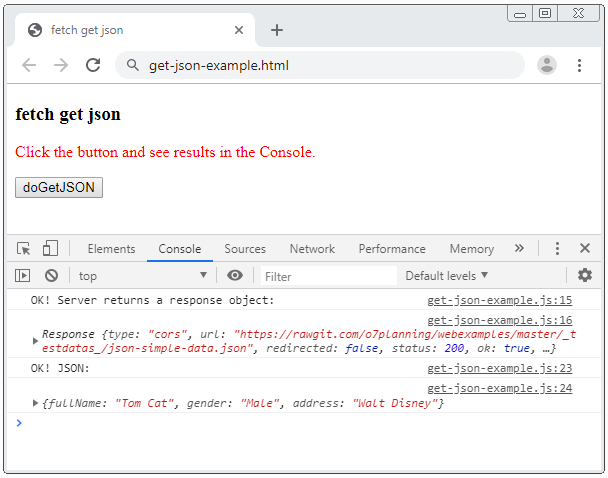
Null JSON Data?
Achtung: Das Method response.json() kann einen Fehler werfen. Wenn Ihre URL eine nicht-JSON Daten zurückgibt, oder ein ungültiges JSON oder eine leere Daten.
Angenommen, Sie schicken eine Anforderung (request) um die Information eines Mitarbeiter nach ID zu holen. Falls der Mitarbeiter existiert, bekommen Sie eine Daten JSON, umgekehrt wenn der Mitarbeiter existiert nicht, bekommen Sie eine Daten NULL. Allerdings verursacht eine Daten NULL den Fehler in einer Position, die response.json() genannt hat. Sehen Sie das Beispiel:
- http://example.com/employee?id=123
// Get information of Employee 123:
fetch("http://example.com/employee?id=123")
.then(function(response) {
console.log("OK! Server returns a response object:");
console.log(response);
if(!response.ok) {
throw new Error("HTTP error, status = " + response.status);
}
// Error if Employee 123 does not exist.
// (*** URL returns null).
var myJSON_promise = response.json(); // =====> Error
return myJSON_promise;
})
.then(function(myJSON) {
console.log("OK! JSON:");
console.log(myJSON);
})
.catch(function(error) {
console.log("Noooooo! Something error:");
console.log(error);
});
(Error)
SyntaxError: Unexpected end of JSON input
Verwenden Sie response.text() statt von response.json():
get-json-null-example.js
// A URL returns null JSON data.
var url = "https://ex1.o7planning.com/_testdatas_/json-null-data.json";
function doGetJSON() {
// Call fetch(url) with default options.
// It returns a Promise object:
var aPromise = fetch(url);
// Work with Promise object:
aPromise
.then(function(response) {
console.log("OK! Server returns a response object:");
console.log(response);
if(!response.ok) {
throw new Error("HTTP error, status = " + response.status);
}
// Get TEXT Promise object from response object:
var myText_promise = response.text();
return myText_promise;
})
.then(function(myText) {
console.log("OK! TEXT:");
console.log(myText);
var myJSON = null;
if(myText != null && myText.trim().length > 0) {
myJSON = JSON.parse(myText);
}
console.log("OK! JSON:");
console.log(myJSON);
})
.catch(function(error) {
console.log("Noooooo! Something error:");
console.log(error);
});
}
get-json-null-example.html
<!DOCTYPE html>
<html>
<head>
<title>fetch get json</title>
<meta charset="UTF-8">
<script src="get-json-null-example.js"></script>
</head>
<body>
<h3>fetch get null json</h3>
<p style="color:red">
Click the button and see the results in the Console.
</p>
<button onclick="doGetJSON()">doGetJSON</button>
</body>
</html>
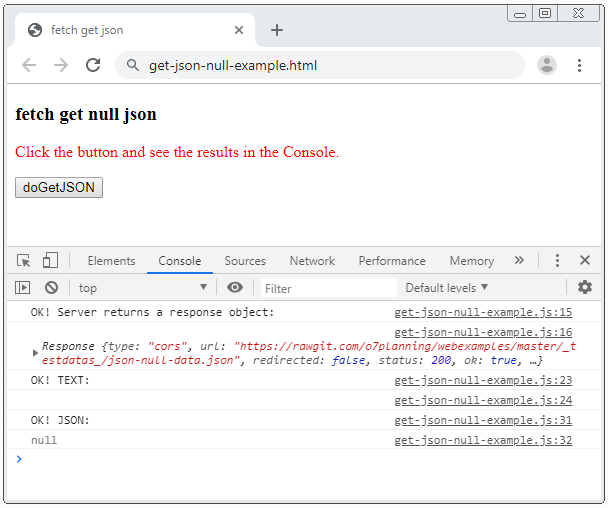
4. Get Blob - response.blob()
Das Method response.blob() gibt eine Versprechung zur Lösung eines Objekt Blob zurück.
In diesem folgenden Beispiel werde ich Fetch API benutzen um ein Foto, das durch eine URL gegeben wird herunterzuladen und es in eine Seite anzuzeigen.
get-blob-example.js
// A URL returns Image data (Blob).
var url = "https://ex1.o7planning.com/_testdatas_/triceratops.png";
function doGetBlob() {
// Call fetch(url) with default options.
// It returns a Promise object:
var aPromise = fetch(url);
// Work with Promise object:
aPromise
.then(function(response) {
console.log("OK! Server returns a response object:");
console.log(response);
if(!response.ok) {
throw new Error("HTTP error, status = " + response.status);
}
// Get Blob Promise from response object:
var myBlob_promise = response.blob();
return myBlob_promise;
})
.then(function(myBlob) {
console.log("OK! Blob:");
console.log(myBlob);
var objectURL = URL.createObjectURL(myBlob);
var myImage = document.getElementById("my-img");
myImage.src = objectURL;
})
.catch(function(error) {
console.log("Noooooo! Something error:");
console.log(error);
});
}
get-blob-example.html
<!DOCTYPE html>
<html>
<head>
<title>fetch get blob</title>
<meta charset="UTF-8">
<script src="get-blob-example.js"></script>
</head>
<body>
<h3>fetch get Blob</h3>
<p style="color:red">
Click the button to load Image.
</p>
<button onclick="doGetBlob()">doGetBlob</button>
<br/>
<img id="my-img" src="" height = 150 />
</body>
</html>
5. Get ArrayBuffer - response.arrayBuffer()
Das Method response.arrayBuffer() gibt eine Versprechung zur Lösung des Objekt ArrayBuffer zurück.
Die Daten werden als einen Puffer heruntergeladen, damit Sie sie sofort ohne die Warte auf das Herunterladen der ganzen Daten arbeiten können. Zum Beispiel, eine Video hat eine größe Kapazität. Sie können Video sehen während seine Daten in Ihrem Browser gerade heruntergeladet werden.
Unten ist das ein Beispiel für die Verwendung von Fetch API um eine Musik-File zu spielen.
get-arraybuffer-example.js
// A URL returns Audio data.
var url = "https://ex1.o7planning.com/_testdatas_/yodel.mp3";
// AudioContext
const audioContext = new AudioContext();
var bufferSource;
// Get ArrayBuffer (Audio data) and play it.
function doGetArrayBuffer() {
bufferSource = audioContext.createBufferSource();
bufferSource.onended = function () {
document.getElementById("play").disabled=false;
};
// Call fetch(url) with default options.
// It returns a Promise object:
var aPromise = fetch(url);
// Work with Promise object:
var retPromise =
aPromise
.then(function(response) {
if(!response.ok) {
throw new Error("HTTP error, status = " + response.status);
}
// Get ArrayBuffer Promise object from response object:
var myArrayBuffer_promise = response.arrayBuffer();
return myArrayBuffer_promise;
})
.then(function(myArrayBuffer) {
audioContext.decodeAudioData(myArrayBuffer, function(decodedData) {
bufferSource.buffer = decodedData;
bufferSource.connect(audioContext.destination);
});
});
return retPromise;
}
function playIt() {
console.clear();
doGetArrayBuffer()
.then(function() {
document.getElementById("play").disabled=true;
bufferSource.start(0);
})
.catch(function(error){
alert("Something Error: " + error.message);
});
}
function stopIt() {
bufferSource.stop(0);
document.getElementById("play").disabled=false;
}
get-arraybuffer-example.html
<!DOCTYPE html>
<html>
<head>
<title>fetch get ArrayBuffer</title>
<meta charset="UTF-8">
<script src="get-arraybuffer-example.js"></script>
</head>
<body>
<h3>fetch get ArrayBuffer</h3>
<p style="color:red">
Click the button to play Audio.
</p>
<button onclick="playIt()" id="play">Play Audio</button>
<button onclick="stopIt()" id="play">Stop Audio</button>
</body>
</html>
Web Audio API hilft Ihnen bei der Arbeiten mit Audio in die web Umwelt. Das ist ein größes API und bezieht sich auf Fetch API. Wenn Sie die Interesse haben, können Sie den folgenden Artikel referenzieren:
- Javascript Web Audio API
6. Get FormData - response.formData()
Das Method response.formData() gibt eine Versprechung zur Lösung eines Objekt FormData zurück. Sie benutzt oft das Method in Service Workers. Wenn der Benutzer die Daten Form zum Server schicken, wird diese Daten durch Service Workers vor dem Eintritt zum Server gehen. In Service Workers können Sie das Method response.formData() rufen und die Werte in FormData bearbeiten wenn möchten.
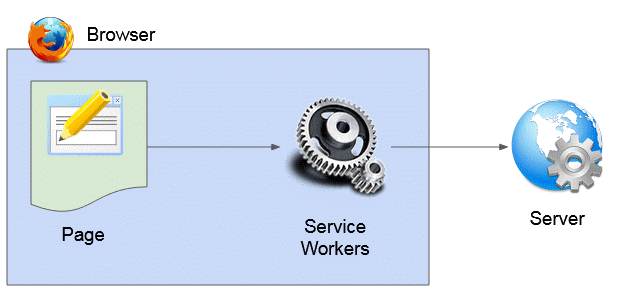
- Javascript Service Worker
Anleitungen ECMAScript, Javascript
- Einführung in Javascript und ECMAScript
- Schnellstart mit Javascript
- Dialogfeld Alert, Confirm, Prompt in Javascript
- Schnellstart mit JavaScript
- Die Anleitung zu JavaScript Variable
- Bitweise Operationen
- Die Anleitung zu JavaScript Array
- Schleifen in JavaScript
- Die Anleitung zu JavaScript Function
- Die Anleitung zu JavaScript Number
- Die Anleitung zu JavaScript Boolean
- Die Anleitung zu JavaScript String
- if else Anweisung in JavaScript
- Switch Anweisung in JavaScript
- Die Anleitung zu JavaScript Error
- Die Anleitung zu JavaScript Date
- Die Anleitung zu JavaScript Module
- Die Geschichte der Module in JavaScript
- Die Funktionen setTimeout und setInterval in JavaScript
- Die Anleitung zu Javascript Form Validation
- Die Anleitung zu JavaScript Web Cookie
- Schlüsselwort void in JavaScript
- Klassen und Objekte in JavaScript
- Klasse und Vererbung Simulationstechniken in JavaScript
- Vererbung und Polymorphismus in JavaScript
- Das Verständnis über Duck Typing in JavaScript
- Die Anleitung zu JavaScript Symbol
- Die Anleitung zu JavaScript Set Collection
- Die Anleitung zu JavaScript Map Collection
- Das Verständnis über JavaScript Iterable und Iterator
- Die Anleitung zu JavaScript Reguläre Ausdrücke
- Die Anleitung zu JavaScript Promise, Async Await
- Die Anleitung zu Javascript Window
- Die Anleitung zu Javascript Console
- Die Anleitung zu Javascript Screen
- Die Anleitung zu Javascript Navigator
- Die Anleitung zu Javascript Geolocation API
- Die Anleitung zu Javascript Location
- Die Anleitung zu Javascript History API
- Die Anleitung zu Javascript Statusbar
- Die Anleitung zu Javascript Locationbar
- Die Anleitung zu Javascript Scrollbars
- Die Anleitung zu Javascript Menubar
- Die Anleitung zu Javascript JSON
- Ereignisbehandlung in JavaScript
- Die Anleitung zu Javascript MouseEvent
- Die Anleitung zu Javascript WheelEvent
- Die Anleitung zu Javascript KeyboardEvent
- Die Anleitung zu Javascript FocusEvent
- Die Anleitung zu Javascript InputEvent
- Die Anleitung zu Javascript ChangeEvent
- Die Anleitung zu Javascript DragEvent
- Die Anleitung zu Javascript HashChangeEvent
- Die Anleitung zu Javascript URL Encoding
- Die Anleitung zu Javascript FileReader
- Die Anleitung zu Javascript XMLHttpRequest
- Die Anleitung zu Javascript Fetch API
- Analysieren Sie XML in Javascript mit DOMParser
- Einführung in Javascript HTML5 Canvas API
- Hervorhebung Code mit SyntaxHighlighter Javascript-Bibliothek
- Was sind Polyfills in der Programmierwissenschaft?
Show More