Die Anleitung zu Android Notifications
1. Android Notification
Eine Anmeldung (Notification) ist eine Nachricht, die Sie für den Benutzer außer der Applikation anzeigen können. Wenn Sie fordern, das System die Anmeldung gibt, erscheint sie zum ersten Mal wie ein Symbol in dem Anmeldungsbereich. Um die detailierten Nachricht zu sehen, öffnet der Benutzer die Anmeldungschublade (notification drawer). die beide Bereiche von dem Anmeldungsbereich und der Anmeldungsschublade werden von System kontrolliert und der Benutzer kann irgendwann sehen
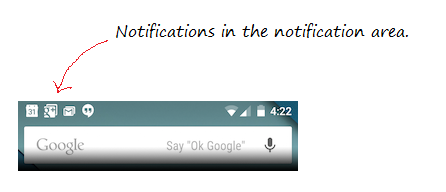
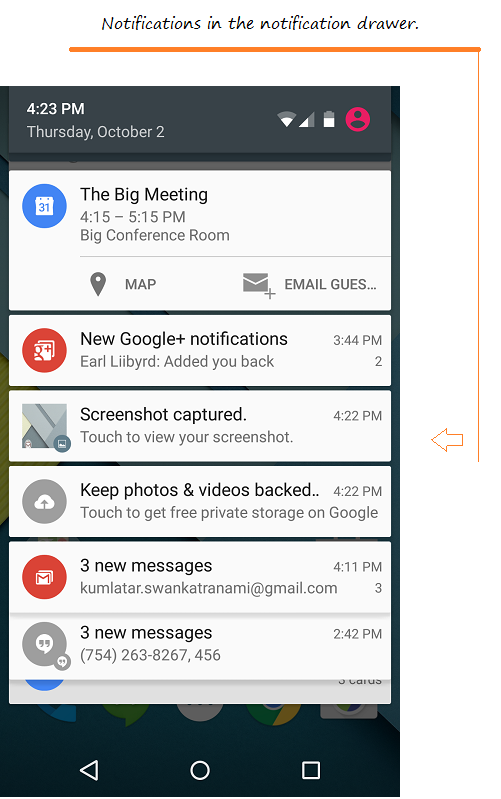
Notification Channel
Ab Version 8.0 (Oreo) beginnt Android, Benachrichtigungen in verschiedene Kanäle zu gruppieren. Jeder Kanal hat ein bestimmtes Verhalten, und dieses Verhalten wird auf alle Benachrichtigungen dieses Kanals angewendet. Jeder Kanal verfügt über eine ID, die ihn darstellt. .
- Android Notification Channel
2. Grundlegendes Beispiel
Erstellen Sie ein Android Projekt - AndroidNotification
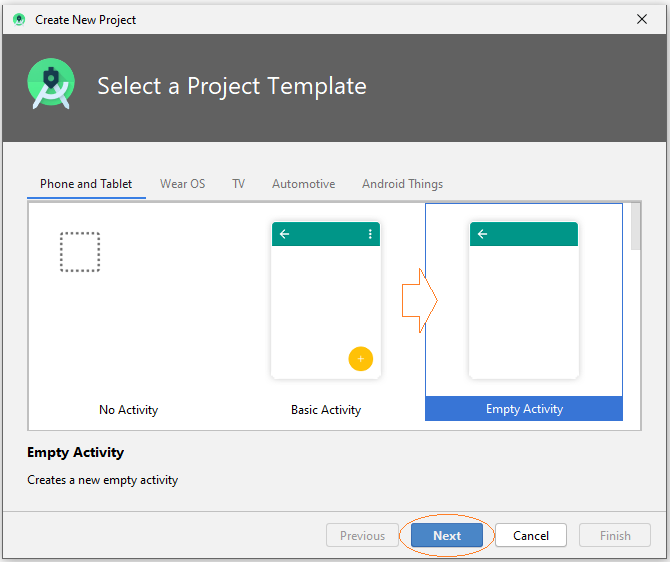
- Name: NotificationBasicExample
- Package name: org.o7planning.notificationbasicexample
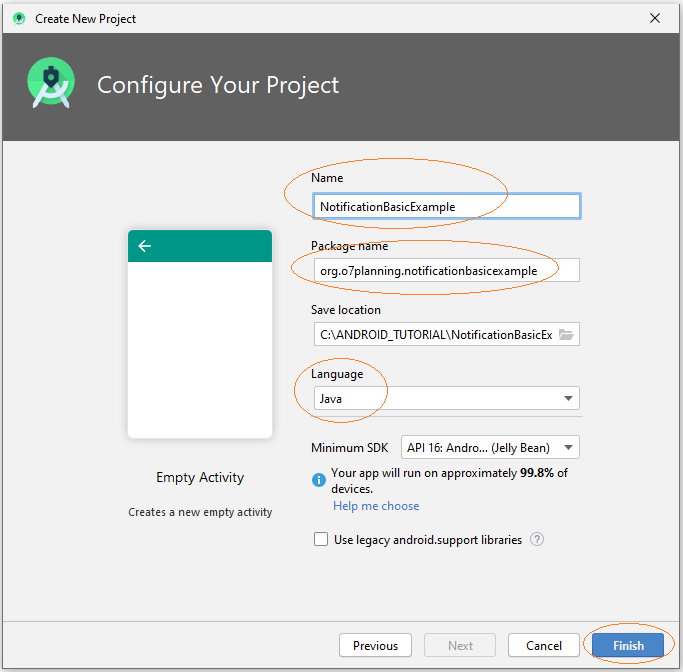
Icons
Android Studio verfügt über eine Bibliothek mit Symbolen, die für Ihre Anwendung nützlich sein können. Ich habe 2 Symbole aus dieser Bibliothek für die aktuelle Anwendung verwendet.
Hinweis: Wenn Sie die Icons in der Bibliothek von Android Studio nicht verwenden möchten, können Sie ein beliebiges Icon verwenden und es in den Ordner "drawable" des Projekts kopieren. Beispiel: icon_notify1.png, icon_notify2.png.
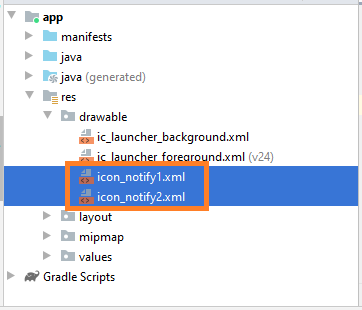
Die Interface der Anwendung:
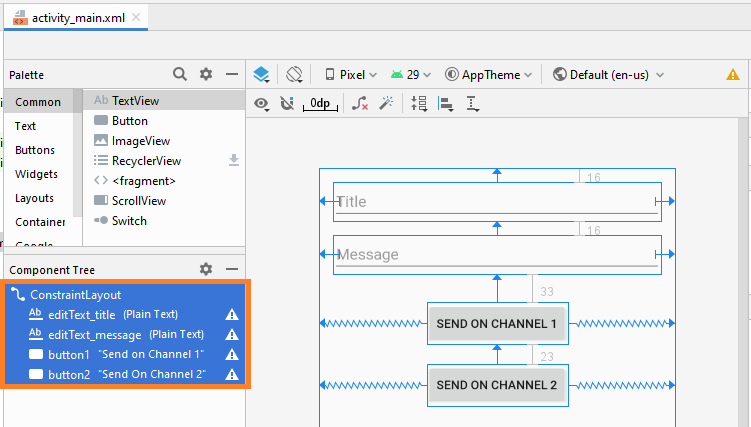
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity" >
<EditText
android:id="@+id/editText_title"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:ems="10"
android:hint="Title"
android:inputType="textPersonName"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<EditText
android:id="@+id/editText_message"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:ems="10"
android:hint="Message"
android:inputType="textPersonName"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editText_title" />
<Button
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="33dp"
android:text="Send on Channel 1"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editText_message" />
<Button
android:id="@+id/button2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="23dp"
android:text="Send On Channel 2"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button1" />
</androidx.constraintlayout.widget.ConstraintLayout>
Notification Application
NotificationApp.java
package org.o7planning.notificationbasicexample;
import android.app.Application;
import android.app.NotificationChannel;
import android.app.NotificationManager;
import android.os.Build;
public class NotificationApp extends Application {
public static final String CHANNEL_1_ID = "channel1";
public static final String CHANNEL_2_ID = "channel2";
@Override
public void onCreate() {
super.onCreate();
this.createNotificationChannels();
}
private void createNotificationChannels() {
if(Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
NotificationChannel channel1 = new NotificationChannel(
CHANNEL_1_ID,
"Channel 1",
NotificationManager.IMPORTANCE_HIGH
);
channel1.setDescription("This is channel 1");
NotificationChannel channel2 = new NotificationChannel(
CHANNEL_2_ID,
"Channel 2",
NotificationManager.IMPORTANCE_LOW
);
channel1.setDescription("This is channel 2");
NotificationManager manager = this.getSystemService(NotificationManager.class);
manager.createNotificationChannel(channel1);
manager.createNotificationChannel(channel2);
}
}
}
Deklarieren Sie NotificationApp in AndroidManifest.xml:
<application
android:name=".NotificationApp"
....
>
...
</application>
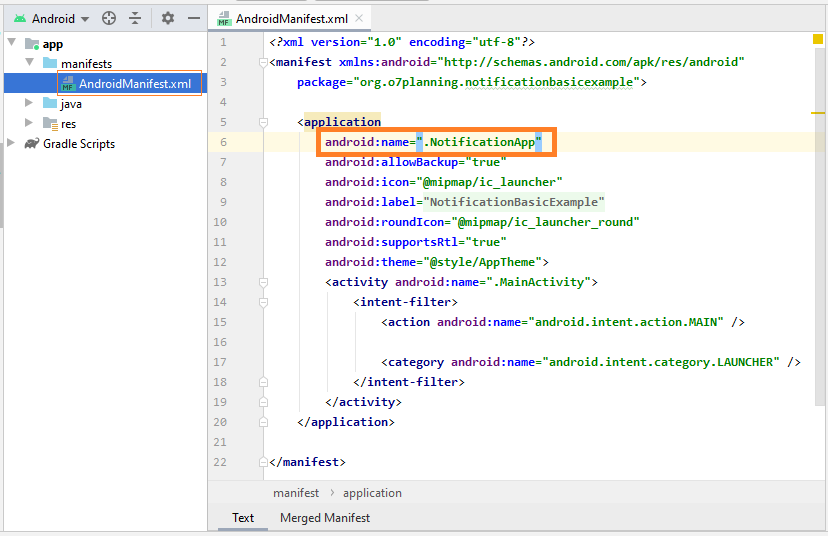
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="org.o7planning.notificationbasicexample">
<application
android:name=".NotificationApp"
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
MainActivity.java
package org.o7planning.notificationbasicexample;
import android.app.Notification;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.app.NotificationCompat;
import androidx.core.app.NotificationManagerCompat;
public class MainActivity extends AppCompatActivity {
private NotificationManagerCompat notificationManagerCompat;
private EditText editTextTitle;
private EditText editTextMessage;
private Button button1;
private Button button2;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.editTextTitle = (EditText) this.findViewById(R.id.editText_title);
this.editTextMessage = (EditText) this.findViewById(R.id.editText_message);
this.button1 = (Button) this.findViewById(R.id.button1);
this.button2 = (Button) this.findViewById(R.id.button2);
this.button1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
sendOnChannel1( );
}
});
this.button2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
sendOnChannel2( );
}
});
//
this.notificationManagerCompat = NotificationManagerCompat.from(this);
}
private void sendOnChannel1() {
String title = this.editTextTitle.getText().toString();
String message = this.editTextMessage.getText().toString();
Notification notification = new NotificationCompat.Builder(this, NotificationApp.CHANNEL_1_ID)
.setSmallIcon(R.drawable.icon_notify1)
.setContentTitle(title)
.setContentText(message)
.setPriority(NotificationCompat.PRIORITY_HIGH)
.setCategory(NotificationCompat.CATEGORY_MESSAGE)
.build();
int notificationId = 1;
this.notificationManagerCompat.notify(notificationId, notification);
}
private void sendOnChannel2() {
String title = this.editTextTitle.getText().toString();
String message = this.editTextMessage.getText().toString();
Notification notification = new NotificationCompat.Builder(this, NotificationApp.CHANNEL_2_ID)
.setSmallIcon(R.drawable.icon_notify2)
.setContentTitle(title)
.setContentText(message)
.setPriority(NotificationCompat.PRIORITY_LOW)
.setCategory(NotificationCompat.CATEGORY_PROMO) // Promotion.
.build();
int notificationId = 2;
this.notificationManagerCompat.notify(notificationId, notification);
}
}
Die Applikation laufen
Wenn eine wichtige Benachrichtigung an das System gesendet wird, können Benutzer einen Warnton hören.
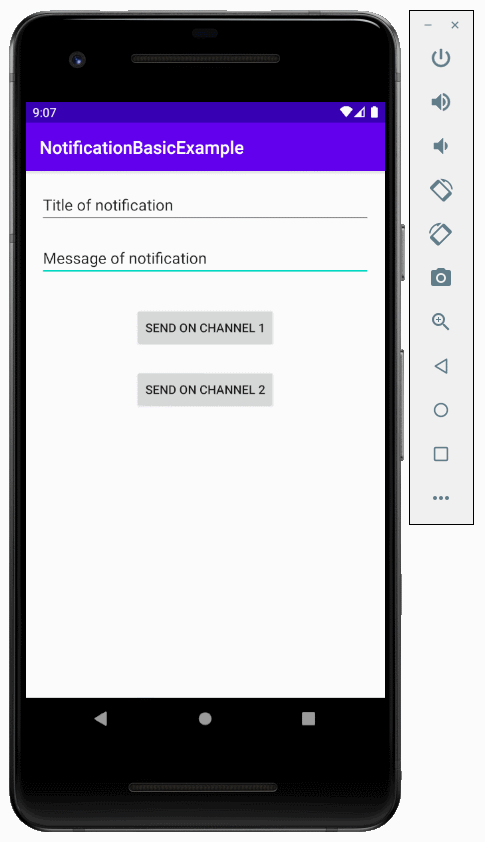
In der Zwischenzeit werden weniger wichtige Benachrichtigungen leise gesendet.
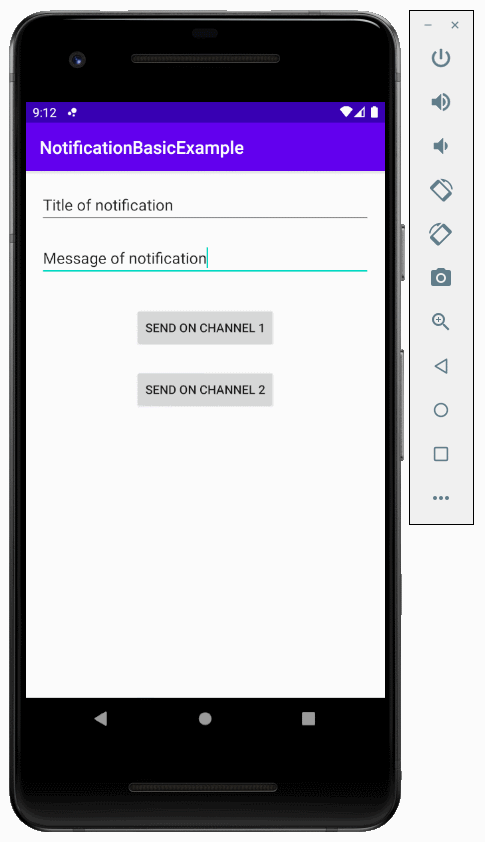
Anleitungen Android
- Was ist erforderlich, um mit Android zu beginnen?
- Installieren Sie Android Studio unter Windows
- Installieren Sie Intel® HAXM für Android Studio
- Konfigurieren Sie Android Emulator in Android Studio
- Die Anleitung zum Android für den Anfänger - Hello Android
- Die Anleitung zum Android für den Anfänger - Grundlegende Beispiele
- Verwenden Sie Image Asset und Icon Asset von Android Studio
- Verwenden des Android Device File Explorer
- Aktivieren Sie USB Debugging auf einem Android-Gerät
- Richten Sie die SDCard für den Emulator ein
- Woher weiß man die Telefonnummer von Android Emulator und ändere es
- Hinzufügen externer Bibliotheken zu Android Project in Android Studio
- Wie deaktiviere ich die Berechtigungen, die der Android-Anwendung bereits erteilt wurden?
- Wie entferne ich Anwendungen aus dem Android Emulator?
- Die Anleitung zu Android UI Layouts
- Die Anleitung zu Android LinearLayout
- Die Anleitung zu Android TableLayout
- Die Anleitung zu Android FrameLayout
- Die Anleitung zu Android Button
- Die Anleitung zu Android ToggleButton
- Die Anleitung zu Android Switch
- Die Anleitung zu Android ImageButton
- Die Anleitung zu Android FloatingActionButton
- Die Anleitung zu Android CheckBox
- Die Anleitung zu Android RadioGroup und RadioButton
- Die Anleitung zu Android Chip und ChipGroup
- ChipGroup und Chip Entry Beispiel
- Die Anleitung zu Android QuickContactBadge
- Die Anleitung zu Android Space
- Die Anleitung zu Android Toast
- Erstellen Sie einen benutzerdefinierten Android Toast
- Die Anleitung zu Android SnackBar
- Die Anleitung zu Android TextView
- Die Anleitung zu Android TextClock
- Die Anleitung zu Android EditText
- Die Anleitung zu Android TextInputLayout
- Die Anleitung zu Android TextWatcher
- Formatieren Sie die Kreditkartennummer mit Android TextWatcher
- Die Anleitung zu Android Clipboard
- Erstellen Sie einen einfachen File Chooser in Android
- Erstellen Sie einen einfachen File Finder Dialog in Android
- Die Anleitung zu Android AutoCompleteTextView und MultiAutoCompleteTextView
- Die Anleitung zu Android ImageView
- Die Anleitung zu Android ImageSwitcher
- Die Anleitung zu Android ScrollView und HorizontalScrollView
- Die Anleitung zu Android WebView
- Die Anleitung zu Android SeekBar
- Die Anleitung zu Android Dialog
- Die Anleitung zu Android AlertDialog
- Die Anleitung zu Android CharacterPickerDialog
- Die Anleitung zu Android DialogFragment
- Die Anleitung zu Android DatePicker
- Die Anleitung zu Android TimePicker
- Die Anleitung zu Android TimePickerDialog
- Die Anleitung zu Android DatePickerDialog
- Die Anleitung zu Android Chronometer
- Die Anleitung zu Android RatingBar
- Die Anleitung zu Android ProgressBar
- Die Anleitung zu Android Spinner
- Die Anleitung zu Android OptionMenu
- Die Anleitung zu Android ContextMenu
- Die Anleitung zu Android PopupMenu
- Die Anleitung zu Android Fragment
- Die Anleitung zu Android ListView
- Android ListView mit Checkbox verwenden ArrayAdapter
- Die Anleitung zu Android GridView
- Die Anleitung zu Android CardView
- Die Anleitung zu Android ViewPager2
- Die Anleitung zu Android StackView
- Die Anleitung zu Android Camera
- Die Anleitung zu Android MediaPlayer
- Die Anleitung zu Android VideoView
- Spielen Sie Sound-Effekte in Android mit SoundPool
- Die Anleitung zu Android Networking
- Die Anleitung zu Android JSON Parser
- Die Anleitung zu Android SharedPreferences
- Die Anleitung zu Android Internal Storage
- Die Anleitung zu Android External Storage
- Die Anleitung zu Android Intents
- Beispiel für eine explizite Android Intent, nennen Sie eine andere Intent
- Beispiel für implizite Android Intent, Öffnen Sie eine URL, senden Sie eine Email
- Die Anleitung zu Android Services
- Die Anleitung zu Android Notifications
- Die Anleitung zu Android SQLite Database
- Die Anleitung zu Google Maps Android API
- Text zu Sprache in Android
- Die Anleitung zu Android AsyncTask
- Die Anleitung zu Android AsyncTaskLoader
- Holen Sie sich die Telefonnummer in Android mit TelephonyManager
- Die Anleitung zu Android SMS
- Die Anleitung zu Android Phone Calls
- Die Anleitung zu Android Wifi Scanning
- Die Anleitung zum Android 2D Game für den Anfänger
Show More