Die Anleitung zu Android CheckBox
1. Android CheckBox
In Android ist CheckBox eine Button mit zwei Status, checked und unchecked. Es ist eine grundlegende Komponente und wird häufig in Android-Anwendungen verwendet.
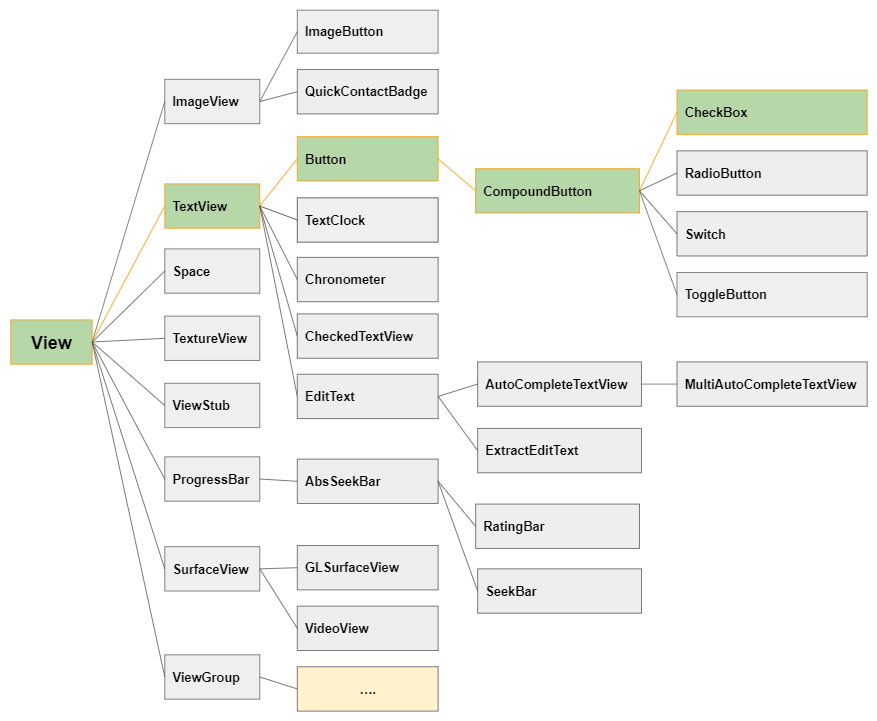
Grundsätzlich können Sie mehrere CheckBox in der Anwendung verwenden, damit Benutzer eine oder mehrere Optionen in einer Reihe von Werten auswählen können.
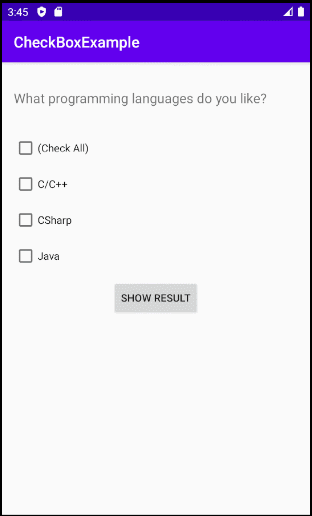
Standardmäßig haben die CheckBox den Status unchecked, sodass Sie den Status über das Attribut android:checked ändern können.
<CheckBox
android:id="@+id/someId"
android:checked="true"
... />
Einige wichtige Attribute von CheckBox:
android:checked | Geben Sie den aktuellen Status für das CheckBox. |
android:gravity | Richten Sie den Text der CheckBox aus. Die möglichen Werte sind left, right, center, top, ... |
android:text | Legen Sie text für CheckBox fest. |
android:textColor | Legen Sie die Schriftsfarbe für Text fest. |
android:textSize | Legen Sie die Schriftsgröße für Text fest. |
android:textStyle | Legen Sie das Stil für Text fest (bold, italic, bolditalic). |
android:background | Legen Sie die Hintergrundsfarbe für CheckBox fest. |
android:padding | Legen Sie Padding für CheckBox fest. |
android:onClick | Der Name vom Method wird aufgeruft wenn der Benutzer auf CheckBox klicken. |
toggle()
Die 4 Klassen ToggleButton, CheckBox, RadioButton, Switch sind die Sub-Klassen von CompoundButton, deshalb werden sie das Method toggle() geerbt. Das Method ist oft verwendet, das Modus vom Checked (ON) nach Unchecked (OFF) umzuwechseln und umgekehrt.
CompoundButton button = (CheckBox) findViewById(R.id.checkBox);
button.toggle();
2. CheckBox Events
Es gibt einige Ereignisse im Zusammenhang mit einer CheckBox, aber hier sind zwei Ereignisse, die am häufigsten verwendet werden:
- checkBox.setOnClickListener(View.OnClickListener)
- checkBox.setOnCheckedChangeListener(CompoundButton.OnCheckedChangeListener)
On Click Event:
Das Ereignis tritt auf, wenn der Benutzer auf das CheckBox klickt, ähnlich wie der Benutzer auf eine Button klickt.
CheckBox chk = (CheckBox) findViewById(R.id.chk1);
chk.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
boolean checked = ((CheckBox) v).isChecked();
// Check which checkbox was clicked
if (checked){
// Your code
}
else{
// Your code
}
}
});
On Checked Change Event:
Das Ereignis tritt auf, wenn die CheckBox ihren Status aufgrund der Aktion des Benutzers oder der Auswirkung des Aufrufs der Methode checkBox.setChecked(newState) ändert.
CheckBox chk = (CheckBox) findViewById(R.id.chk1);
chk.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
if(isChecked) {
// Your code
} else {
// Your code
}
}
});
3. Ein Beispiel vom CheckBox
Jetzt schauen Sie das Beispiel vor:
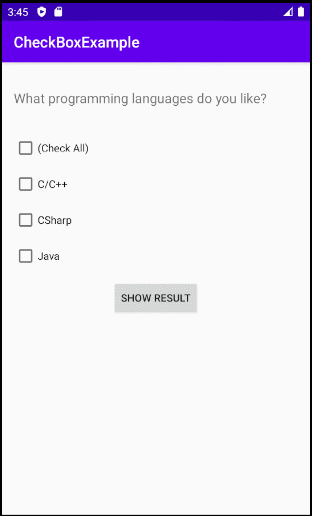
Die Interface der Anwendungsbeispiel wird wie das aussehen:
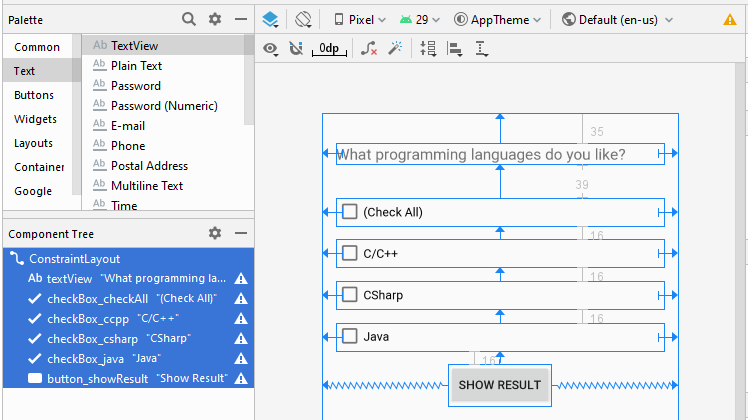
Hinweis: Wenn Sie an den Schritten zum Entwerfen der Interface dieser Anwendung interessiert sind, lesen Sie bitte den Anhang am Ende des Artikels.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="35dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="What programming languages do you like?"
android:textSize="18sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<CheckBox
android:id="@+id/checkBox_checkAll"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="39dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="(Check All)"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView" />
<CheckBox
android:id="@+id/checkBox_ccpp"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="C/C++"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/checkBox_checkAll" />
<CheckBox
android:id="@+id/checkBox_csharp"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="CSharp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/checkBox_ccpp" />
<CheckBox
android:id="@+id/checkBox_java"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Java"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/checkBox_csharp" />
<Button
android:id="@+id/button_showResult"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:text="Show Result"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/checkBox_java" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package com.example.checkboxexample;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.CheckBox;
import android.widget.CompoundButton;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
private CheckBox checkBoxCheckAll;
private CheckBox checkBoxCcpp;
private CheckBox checkBoxCsharp;
private CheckBox checkBoxJava;
private Button buttonShowResult;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.checkBoxCheckAll = (CheckBox) this.findViewById(R.id.checkBox_checkAll);
this.checkBoxCcpp = (CheckBox) this.findViewById(R.id.checkBox_ccpp);
this.checkBoxCsharp = (CheckBox) this.findViewById(R.id.checkBox_csharp);
this.checkBoxJava = (CheckBox) this.findViewById(R.id.checkBox_java);
this.buttonShowResult = (Button) this.findViewById(R.id.button_showResult);
this.buttonShowResult.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
showResult();
}
});
this.checkBoxCheckAll.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
checkAllCheckedChange(isChecked);
}
});
}
private void showResult() {
String message = null;
if(this.checkBoxCcpp.isChecked()) {
message = this.checkBoxCcpp.getText().toString();
}
if(this.checkBoxCsharp.isChecked()) {
if(message== null) {
message = this.checkBoxCsharp.getText().toString();
} else {
message += ", " + this.checkBoxCsharp.getText().toString();
}
}
if(this.checkBoxJava.isChecked()) {
if(message== null) {
message = this.checkBoxJava.getText().toString();
} else {
message += ", " + this.checkBoxJava.getText().toString();
}
}
message = message == null? "You select nothing": "You select: " + message;
Toast.makeText(this, message, Toast.LENGTH_LONG).show();
}
// When "Check All" change state.
private void checkAllCheckedChange(boolean isChecked) {
this.checkBoxCsharp.setChecked(isChecked);
this.checkBoxCcpp.setChecked(isChecked);
this.checkBoxJava.setChecked(isChecked);
}
}
Anleitungen Android
- Konfigurieren Sie Android Emulator in Android Studio
- Die Anleitung zu Android ToggleButton
- Erstellen Sie einen einfachen File Finder Dialog in Android
- Die Anleitung zu Android TimePickerDialog
- Die Anleitung zu Android DatePickerDialog
- Was ist erforderlich, um mit Android zu beginnen?
- Installieren Sie Android Studio unter Windows
- Installieren Sie Intel® HAXM für Android Studio
- Die Anleitung zu Android AsyncTask
- Die Anleitung zu Android AsyncTaskLoader
- Die Anleitung zum Android für den Anfänger - Grundlegende Beispiele
- Woher weiß man die Telefonnummer von Android Emulator und ändere es
- Die Anleitung zu Android TextInputLayout
- Die Anleitung zu Android CardView
- Die Anleitung zu Android ViewPager2
- Holen Sie sich die Telefonnummer in Android mit TelephonyManager
- Die Anleitung zu Android Phone Calls
- Die Anleitung zu Android Wifi Scanning
- Die Anleitung zum Android 2D Game für den Anfänger
- Die Anleitung zu Android DialogFragment
- Die Anleitung zu Android CharacterPickerDialog
- Die Anleitung zum Android für den Anfänger - Hello Android
- Verwenden des Android Device File Explorer
- Aktivieren Sie USB Debugging auf einem Android-Gerät
- Die Anleitung zu Android UI Layouts
- Die Anleitung zu Android SMS
- Die Anleitung zu Android SQLite Database
- Die Anleitung zu Google Maps Android API
- Text zu Sprache in Android
- Die Anleitung zu Android Space
- Die Anleitung zu Android Toast
- Erstellen Sie einen benutzerdefinierten Android Toast
- Die Anleitung zu Android SnackBar
- Die Anleitung zu Android TextView
- Die Anleitung zu Android TextClock
- Die Anleitung zu Android EditText
- Die Anleitung zu Android TextWatcher
- Formatieren Sie die Kreditkartennummer mit Android TextWatcher
- Die Anleitung zu Android Clipboard
- Erstellen Sie einen einfachen File Chooser in Android
- Die Anleitung zu Android AutoCompleteTextView und MultiAutoCompleteTextView
- Die Anleitung zu Android ImageView
- Die Anleitung zu Android ImageSwitcher
- Die Anleitung zu Android ScrollView und HorizontalScrollView
- Die Anleitung zu Android WebView
- Die Anleitung zu Android SeekBar
- Die Anleitung zu Android Dialog
- Die Anleitung zu Android AlertDialog
- Die Anleitung zu Android RatingBar
- Die Anleitung zu Android ProgressBar
- Die Anleitung zu Android Spinner
- Die Anleitung zu Android Button
- Die Anleitung zu Android Switch
- Die Anleitung zu Android ImageButton
- Die Anleitung zu Android FloatingActionButton
- Die Anleitung zu Android CheckBox
- Die Anleitung zu Android RadioGroup und RadioButton
- Die Anleitung zu Android Chip und ChipGroup
- Verwenden Sie Image Asset und Icon Asset von Android Studio
- Richten Sie die SDCard für den Emulator ein
- ChipGroup und Chip Entry Beispiel
- Hinzufügen externer Bibliotheken zu Android Project in Android Studio
- Wie deaktiviere ich die Berechtigungen, die der Android-Anwendung bereits erteilt wurden?
- Wie entferne ich Anwendungen aus dem Android Emulator?
- Die Anleitung zu Android LinearLayout
- Die Anleitung zu Android TableLayout
- Die Anleitung zu Android FrameLayout
- Die Anleitung zu Android QuickContactBadge
- Die Anleitung zu Android StackView
- Die Anleitung zu Android Camera
- Die Anleitung zu Android MediaPlayer
- Die Anleitung zu Android VideoView
- Spielen Sie Sound-Effekte in Android mit SoundPool
- Die Anleitung zu Android Networking
- Die Anleitung zu Android JSON Parser
- Die Anleitung zu Android SharedPreferences
- Die Anleitung zu Android Internal Storage
- Die Anleitung zu Android External Storage
- Die Anleitung zu Android Intents
- Beispiel für eine explizite Android Intent, nennen Sie eine andere Intent
- Beispiel für implizite Android Intent, Öffnen Sie eine URL, senden Sie eine Email
- Die Anleitung zu Android Services
- Die Anleitung zu Android Notifications
- Die Anleitung zu Android DatePicker
- Die Anleitung zu Android TimePicker
- Die Anleitung zu Android Chronometer
- Die Anleitung zu Android OptionMenu
- Die Anleitung zu Android ContextMenu
- Die Anleitung zu Android PopupMenu
- Die Anleitung zu Android Fragment
- Die Anleitung zu Android ListView
- Android ListView mit Checkbox verwenden ArrayAdapter
- Die Anleitung zu Android GridView
Show More