Die Anleitung zu Android Chip und ChipGroup
1. Chip
Chips sind neue Komponenten, die zuerst in Android SDK Version 28 eingeführt wurden. Sie sind im Grunde die Interfaceskomponenten, die aus Symbolen und Text bestehen, die in einem rechteckigen Hintergrund mit abgerundeten Ecken platziert werden.
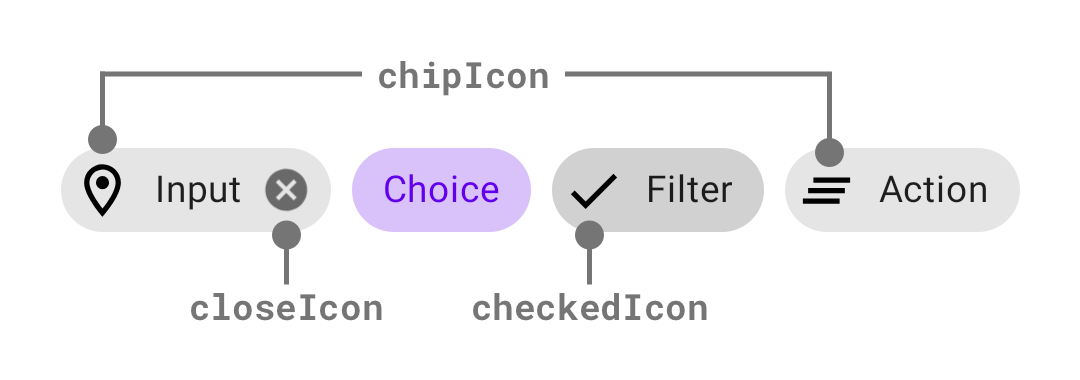
Um das Chip-Objekt im Projekt zu verwenden, müssen Sie es installieren.
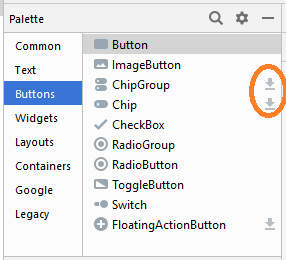
Nachdem sie erfolgreich installiert wurde, wird eine Bibliothek mit Chips in der Datei build.gradle (Modul app) deklariert.
implementation 'com.google.android.material:material:1.1.0'
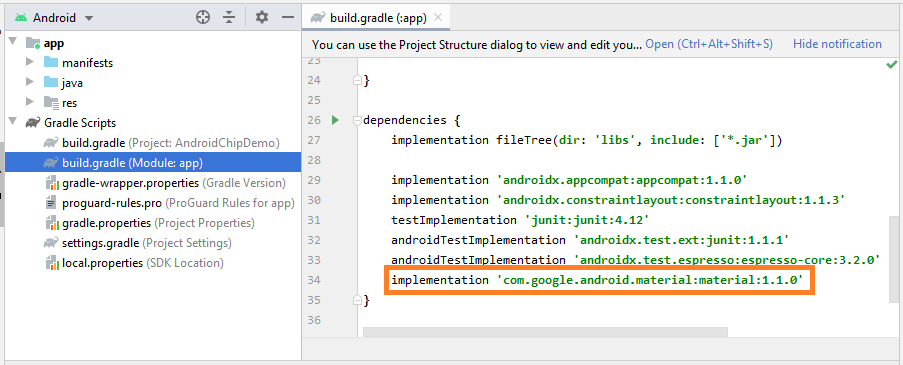
Die Code XML eines einfachen Chip :
Chip Sample
<!-- IMPORTANT: android:theme is required -->
<com.google.android.material.chip.Chip
android:id="@+id/chip1"
style="@style/Widget.MaterialComponents.Chip.Action"
android:theme="@style/Theme.MaterialComponents.Light"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Chip Action" />
theme attribute
Das android:theme-Attribut ist für einen Chip erforderlich. Ohne dieses Attribut funktioniert die gesamte Anwendung nicht, und sie benachrichtigt Sie auch nicht darüber. Ich war überrascht, als ich zum ersten Mal einen Chip benutzte.
- android:theme="@style/Theme.MaterialComponents"
- android:theme="@style/Theme.MaterialComponents.Light"
- android:theme="@style/Theme.MaterialComponents.***"
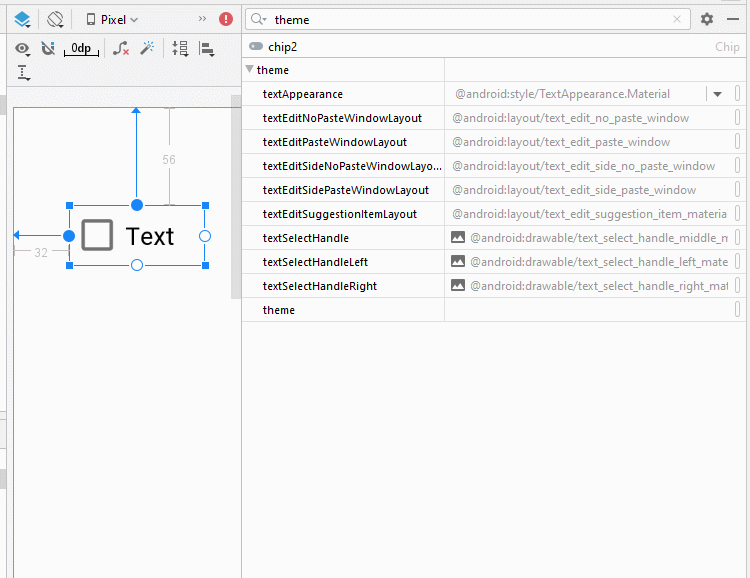
style attribute:
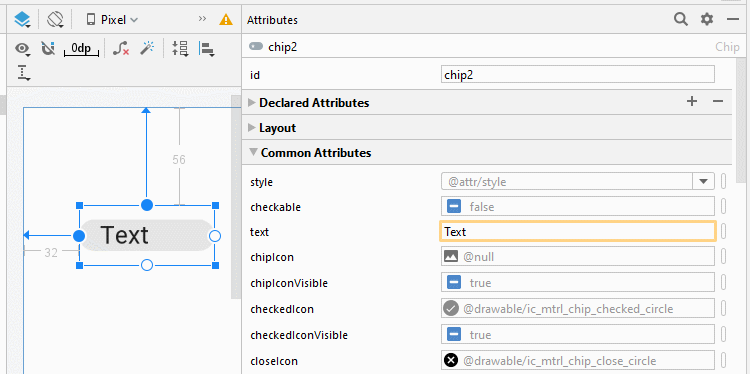
Das style-Attribut hat 4 Werte, mit denen Sie den Typ von Chips angeben können:
- @style/Widget.MaterialComponents.Chip.Action (Default)
- @style/Widget.MaterialComponents.Chip.Entry
- @style/Widget.MaterialComponents.Chip.Choice
- @style/Widget.MaterialComponents.Chip.Filter
Style Default
Das Style-Attribut ist optional, und der Standardwert wird als "@style/Widget.MaterialComponents.Chip.Action" bezeichnet.
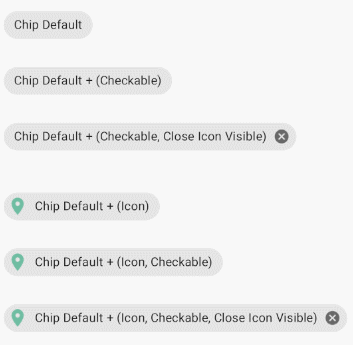
Chip Default Sample
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<com.google.android.material.chip.Chip
android:id="@+id/chip1"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="50dp"
android:text="Chip Default"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<com.google.android.material.chip.Chip
android:id="@+id/chip2"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Default + (Checkable)"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip1" />
<com.google.android.material.chip.Chip
android:id="@+id/chip3"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Default + (Checkable, Close Icon Visible)"
android:theme="@style/Theme.MaterialComponents.Light"
app:closeIconVisible="true"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip2" />
<com.google.android.material.chip.Chip
android:id="@+id/chip4"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="32dp"
android:checkable="true"
android:text="Chip Default + (Icon)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip3" />
<com.google.android.material.chip.Chip
android:id="@+id/chip5"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Default + (Icon, Checkable)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip4" />
<com.google.android.material.chip.Chip
android:id="@+id/chip6"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Default + (Icon, Checkable, Close Icon Visible)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:closeIconVisible="true"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip5" />
</androidx.constraintlayout.widget.ConstraintLayout>
style="@style/Widget.MaterialComponents.Chip.Action"
Das ist der Standardwert von style.
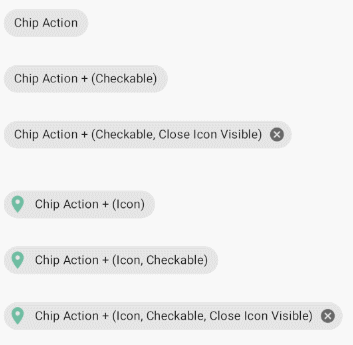
style="@style/Widget.MaterialComponents.Chip.Entry"
Chip Entry = Chip Default + Close Icon + Checkable
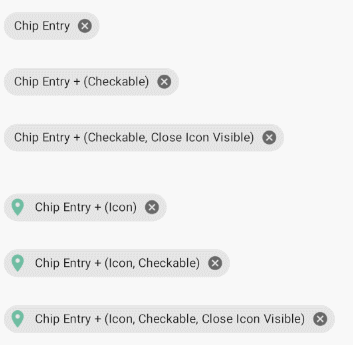
Chip Entry Sample
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<com.google.android.material.chip.Chip
android:id="@+id/chip1"
style="@style/Widget.MaterialComponents.Chip.Entry"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="50dp"
android:text="Chip Entry"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<com.google.android.material.chip.Chip
android:id="@+id/chip2"
style="@style/Widget.MaterialComponents.Chip.Entry"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Entry + (Checkable)"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip1" />
<com.google.android.material.chip.Chip
android:id="@+id/chip3"
style="@style/Widget.MaterialComponents.Chip.Entry"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Entry + (Checkable, Close Icon Visible)"
android:theme="@style/Theme.MaterialComponents.Light"
app:closeIconVisible="true"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip2" />
<com.google.android.material.chip.Chip
android:id="@+id/chip4"
style="@style/Widget.MaterialComponents.Chip.Entry"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="32dp"
android:checkable="true"
android:text="Chip Entry + (Icon)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip3" />
<com.google.android.material.chip.Chip
android:id="@+id/chip5"
style="@style/Widget.MaterialComponents.Chip.Entry"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Entry + (Icon, Checkable)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip4" />
<com.google.android.material.chip.Chip
android:id="@+id/chip6"
style="@style/Widget.MaterialComponents.Chip.Entry"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Entry + (Icon, Checkable, Close Icon Visible)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:closeIconVisible="true"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip5" />
</androidx.constraintlayout.widget.ConstraintLayout>
style="@style/Widget.MaterialComponents.Chip.Choice"
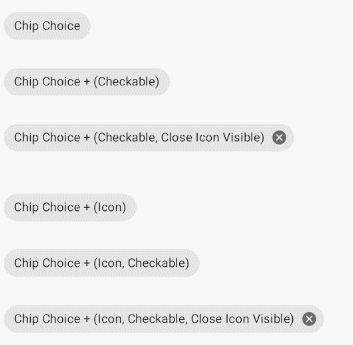
Here sind die Eigenschaften von Chip Choice:
- Wenn Chip Choice gewählt (selected) wird, wird sein Hintergrund dunkler.
- Kein Symbol check wird links angezeigt, auch wenn Sie checkable=true festlegen.
- Icon - wird nicht anzgezeigt auch wenn Sie den Wert für es festlegen.
Chip Choice Sample
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<com.google.android.material.chip.Chip
android:id="@+id/chip1"
style="@style/Widget.MaterialComponents.Chip.Choice"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="50dp"
android:text="Chip Choice"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<com.google.android.material.chip.Chip
android:id="@+id/chip2"
style="@style/Widget.MaterialComponents.Chip.Choice"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Choice + (Checkable)"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip1" />
<com.google.android.material.chip.Chip
android:id="@+id/chip3"
style="@style/Widget.MaterialComponents.Chip.Choice"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Choice + (Checkable, Close Icon Visible)"
android:theme="@style/Theme.MaterialComponents.Light"
app:closeIconVisible="true"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip2" />
<com.google.android.material.chip.Chip
android:id="@+id/chip4"
style="@style/Widget.MaterialComponents.Chip.Choice"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="32dp"
android:checkable="true"
android:text="Chip Choice + (Icon)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip3" />
<com.google.android.material.chip.Chip
android:id="@+id/chip5"
style="@style/Widget.MaterialComponents.Chip.Choice"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Choice + (Icon, Checkable)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip4" />
<com.google.android.material.chip.Chip
android:id="@+id/chip6"
style="@style/Widget.MaterialComponents.Chip.Choice"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Choice + (Icon, Checkable, Close Icon Visible)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:closeIconVisible="true"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip5" />
</androidx.constraintlayout.widget.ConstraintLayout>
style="@style/Widget.MaterialComponents.Chip.Filter"
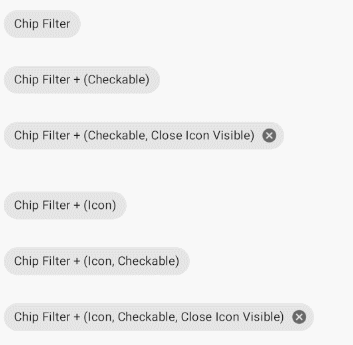
Die Eigenschaften von Chip Filter:
- Chip Filter = Chip Default + Checkable
- Icon - wird nicht angezeigt auch wenn Sie den Wert für es festlegen.
Chip Filter Sample
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<com.google.android.material.chip.Chip
android:id="@+id/chip1"
style="@style/Widget.MaterialComponents.Chip.Filter"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="50dp"
android:text="Chip Filter"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<com.google.android.material.chip.Chip
android:id="@+id/chip2"
style="@style/Widget.MaterialComponents.Chip.Filter"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Filter + (Checkable)"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip1" />
<com.google.android.material.chip.Chip
android:id="@+id/chip3"
style="@style/Widget.MaterialComponents.Chip.Filter"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Filter + (Checkable, Close Icon Visible)"
android:theme="@style/Theme.MaterialComponents.Light"
app:closeIconVisible="true"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip2" />
<com.google.android.material.chip.Chip
android:id="@+id/chip4"
style="@style/Widget.MaterialComponents.Chip.Filter"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="32dp"
android:checkable="true"
android:text="Chip Filter + (Icon)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip3" />
<com.google.android.material.chip.Chip
android:id="@+id/chip5"
style="@style/Widget.MaterialComponents.Chip.Filter"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Filter + (Icon, Checkable)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip4" />
<com.google.android.material.chip.Chip
android:id="@+id/chip6"
style="@style/Widget.MaterialComponents.Chip.Filter"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Filter + (Icon, Checkable, Close Icon Visible)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:closeIconVisible="true"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip5" />
</androidx.constraintlayout.widget.ConstraintLayout>
2. Chip Theme
Das Attribut android:theme ist für Chip erforderlich. Ihre Anwendung kann nicht funktionieren wenn Chip dieses Attribut nicht hat oder wenn Sie einen ungültigen Wert für es festlegen.
Die möglichen Werten sind:
- android:theme="@style/Theme.MaterialComponents.Light"
- android:theme="@style/Theme.MaterialComponents.Bridge"
- android:theme="@style/Theme.MaterialComponents.DayNight"
- .....
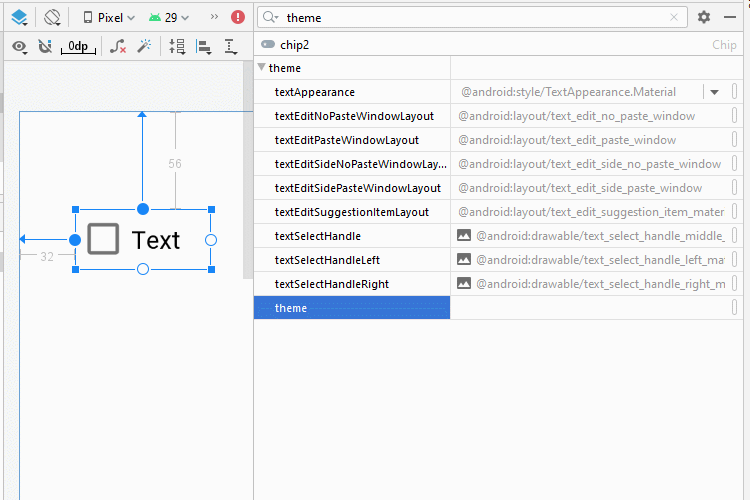
android:theme="@style/Theme.MaterialComponents.Light"
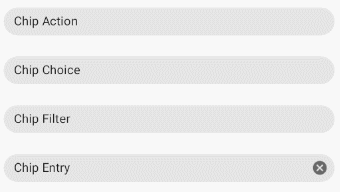
android:theme="@style/Theme.MaterialComponents.Bridge"
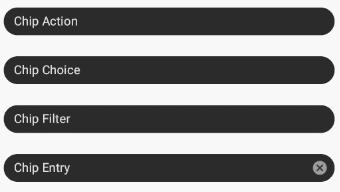
3. Chip Listener
Es gibt viele Ereignisse im Zusammenhang mit Chips, aber im Grunde gibt es 3 gemeinsame Ereignisse wie folgt:
- chip.setOnClickListener(View.OnClickListener)
- chip.setOnCloseIconClickListener(View.OnClickListener)
- chip.setOnCheckedChangeListener(CompoundButton.OnCheckedChangeListener)
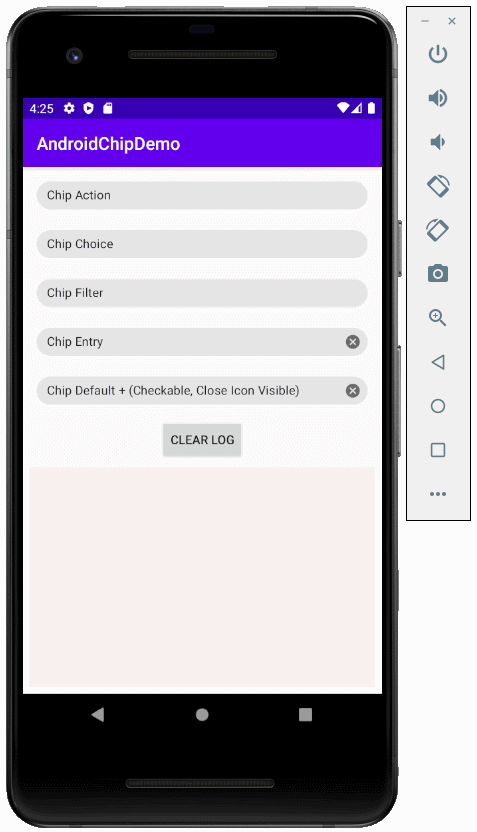
main_activity.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<com.google.android.material.chip.Chip
android:id="@+id/chip_action"
style="@style/Widget.MaterialComponents.Chip.Action"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:clickable="true"
android:text="Chip Action"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<com.google.android.material.chip.Chip
android:id="@+id/chip_choice"
style="@style/Widget.MaterialComponents.Chip.Choice"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Chip Choice"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip_action" />
<com.google.android.material.chip.Chip
android:id="@+id/chip_filter"
style="@style/Widget.MaterialComponents.Chip.Filter"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Chip Filter"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip_choice" />
<com.google.android.material.chip.Chip
android:id="@+id/chip_entry"
style="@style/Widget.MaterialComponents.Chip.Entry"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Chip Entry"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip_filter" />
<com.google.android.material.chip.Chip
android:id="@+id/chip_custom"
android:layout_width="0dp"
android:layout_height="48dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:checkable="true"
android:text="Chip Default + (Checkable, Close Icon Visible)"
android:theme="@style/Theme.MaterialComponents.Light"
app:closeIconVisible="true"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip_entry" />
<Button
android:id="@+id/button_clear"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="8dp"
android:text="Clear Log"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip_custom" />
<EditText
android:id="@+id/editText_log"
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="8dp"
android:layout_marginRight="8dp"
android:layout_marginBottom="8dp"
android:background="#F8EFEF"
android:ems="10"
android:gravity="top|left"
android:inputType="textMultiLine"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button_clear" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package org.o7planning.androidchipdemo;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.CompoundButton;
import android.widget.EditText;
import com.google.android.material.chip.Chip;
public class MainActivity extends AppCompatActivity {
private Chip chipAction;
private Chip chipChoice;
private Chip chipEntry;
private Chip chipFilter;
private Chip chipCustom;
private EditText editTextLog;
private Button buttonClear;
private View.OnClickListener clickListener;
private View.OnClickListener closeIconClickListener;
private CompoundButton.OnCheckedChangeListener checkedChangeListener;
private static final String LOG_TAG = "AndroidChipDemo";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.chipAction = (Chip) this.findViewById(R.id.chip_action);
this.chipChoice = (Chip) this.findViewById(R.id.chip_choice);
this.chipEntry = (Chip) this.findViewById(R.id.chip_entry);
this.chipFilter = (Chip) this.findViewById(R.id.chip_filter);
this.chipCustom = (Chip) this.findViewById(R.id.chip_custom);
this.editTextLog = (EditText) this.findViewById(R.id.editText_log);
this.buttonClear = (Button) this.findViewById(R.id.button_clear);
//
this.clickListener= new ClickListenerImpl();
this.closeIconClickListener = new CloseIconClickListenerImpl();
this.checkedChangeListener = new CheckedChangeListenerImpl();
//
this.chipAction.setOnClickListener( clickListener);
this.chipAction.setOnCloseIconClickListener(closeIconClickListener);
this.chipAction.setOnCheckedChangeListener(checkedChangeListener);
//
this.chipChoice.setOnClickListener( clickListener);
this.chipChoice.setOnCloseIconClickListener(closeIconClickListener);
this.chipChoice.setOnCheckedChangeListener(checkedChangeListener);
//
this.chipEntry.setOnClickListener( clickListener);
this.chipEntry.setOnCloseIconClickListener(closeIconClickListener);
this.chipEntry.setOnCheckedChangeListener(checkedChangeListener);
//
this.chipFilter.setOnClickListener( clickListener);
this.chipFilter.setOnCloseIconClickListener(closeIconClickListener);
this.chipFilter.setOnCheckedChangeListener(checkedChangeListener);
//
this.chipCustom.setOnClickListener( clickListener);
this.chipCustom.setOnCloseIconClickListener(closeIconClickListener);
this.chipCustom.setOnCheckedChangeListener(checkedChangeListener);
//
this.buttonClear.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
editTextLog.setText("");
}
});
}
private void appendLog(String text) {
this.editTextLog.append(text) ;
this.editTextLog.append("\n");
Log.i(LOG_TAG, text);
}
class ClickListenerImpl implements View.OnClickListener {
@Override
public void onClick(View v) {
appendLog("Clicked");
}
}
class CloseIconClickListenerImpl implements View.OnClickListener {
@Override
public void onClick(View v) {
appendLog("Close Icon Clicked");
}
}
class CheckedChangeListenerImpl implements CompoundButton.OnCheckedChangeListener {
@Override
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
appendLog("Checked Changed! isChecked? " + isChecked);
}
}
}
4. ChipGroup
ChipGroup wird benutzt um mehrere Chip zu enthalten. Standardmäßig werden Chips nach vielen Zeilen sortiert, wenn eine Zeile nicht alle Chips enthalten kann..
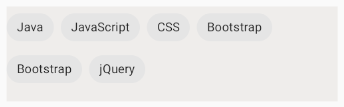
Sie können es jedoch so einstellen, dass die Chips in einer einzigen Zeile angezeigt werden.
<com.google.android.material.chip.ChipGroup
app:singleLine="true"
...>
</com.google.android.material.chip.ChipGroup>
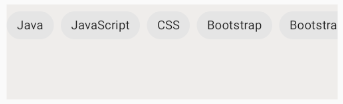
ChipGroup kann auch als RadioGroup arbeiten, was bedeutet, dass, wenn einer der Chips als checked festgelegt wird, wird der Rest der Chipsunchecked sein.
<com.google.android.material.chip.ChipGroup
app:singleSelection="true"
...>
</com.google.android.material.chip.ChipGroup>
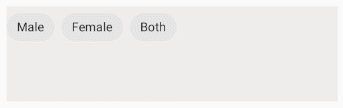
Anleitungen Android
- Konfigurieren Sie Android Emulator in Android Studio
- Die Anleitung zu Android ToggleButton
- Erstellen Sie einen einfachen File Finder Dialog in Android
- Die Anleitung zu Android TimePickerDialog
- Die Anleitung zu Android DatePickerDialog
- Was ist erforderlich, um mit Android zu beginnen?
- Installieren Sie Android Studio unter Windows
- Installieren Sie Intel® HAXM für Android Studio
- Die Anleitung zu Android AsyncTask
- Die Anleitung zu Android AsyncTaskLoader
- Die Anleitung zum Android für den Anfänger - Grundlegende Beispiele
- Woher weiß man die Telefonnummer von Android Emulator und ändere es
- Die Anleitung zu Android TextInputLayout
- Die Anleitung zu Android CardView
- Die Anleitung zu Android ViewPager2
- Holen Sie sich die Telefonnummer in Android mit TelephonyManager
- Die Anleitung zu Android Phone Calls
- Die Anleitung zu Android Wifi Scanning
- Die Anleitung zum Android 2D Game für den Anfänger
- Die Anleitung zu Android DialogFragment
- Die Anleitung zu Android CharacterPickerDialog
- Die Anleitung zum Android für den Anfänger - Hello Android
- Verwenden des Android Device File Explorer
- Aktivieren Sie USB Debugging auf einem Android-Gerät
- Die Anleitung zu Android UI Layouts
- Die Anleitung zu Android SMS
- Die Anleitung zu Android SQLite Database
- Die Anleitung zu Google Maps Android API
- Text zu Sprache in Android
- Die Anleitung zu Android Space
- Die Anleitung zu Android Toast
- Erstellen Sie einen benutzerdefinierten Android Toast
- Die Anleitung zu Android SnackBar
- Die Anleitung zu Android TextView
- Die Anleitung zu Android TextClock
- Die Anleitung zu Android EditText
- Die Anleitung zu Android TextWatcher
- Formatieren Sie die Kreditkartennummer mit Android TextWatcher
- Die Anleitung zu Android Clipboard
- Erstellen Sie einen einfachen File Chooser in Android
- Die Anleitung zu Android AutoCompleteTextView und MultiAutoCompleteTextView
- Die Anleitung zu Android ImageView
- Die Anleitung zu Android ImageSwitcher
- Die Anleitung zu Android ScrollView und HorizontalScrollView
- Die Anleitung zu Android WebView
- Die Anleitung zu Android SeekBar
- Die Anleitung zu Android Dialog
- Die Anleitung zu Android AlertDialog
- Die Anleitung zu Android RatingBar
- Die Anleitung zu Android ProgressBar
- Die Anleitung zu Android Spinner
- Die Anleitung zu Android Button
- Die Anleitung zu Android Switch
- Die Anleitung zu Android ImageButton
- Die Anleitung zu Android FloatingActionButton
- Die Anleitung zu Android CheckBox
- Die Anleitung zu Android RadioGroup und RadioButton
- Die Anleitung zu Android Chip und ChipGroup
- Verwenden Sie Image Asset und Icon Asset von Android Studio
- Richten Sie die SDCard für den Emulator ein
- ChipGroup und Chip Entry Beispiel
- Hinzufügen externer Bibliotheken zu Android Project in Android Studio
- Wie deaktiviere ich die Berechtigungen, die der Android-Anwendung bereits erteilt wurden?
- Wie entferne ich Anwendungen aus dem Android Emulator?
- Die Anleitung zu Android LinearLayout
- Die Anleitung zu Android TableLayout
- Die Anleitung zu Android FrameLayout
- Die Anleitung zu Android QuickContactBadge
- Die Anleitung zu Android StackView
- Die Anleitung zu Android Camera
- Die Anleitung zu Android MediaPlayer
- Die Anleitung zu Android VideoView
- Spielen Sie Sound-Effekte in Android mit SoundPool
- Die Anleitung zu Android Networking
- Die Anleitung zu Android JSON Parser
- Die Anleitung zu Android SharedPreferences
- Die Anleitung zu Android Internal Storage
- Die Anleitung zu Android External Storage
- Die Anleitung zu Android Intents
- Beispiel für eine explizite Android Intent, nennen Sie eine andere Intent
- Beispiel für implizite Android Intent, Öffnen Sie eine URL, senden Sie eine Email
- Die Anleitung zu Android Services
- Die Anleitung zu Android Notifications
- Die Anleitung zu Android DatePicker
- Die Anleitung zu Android TimePicker
- Die Anleitung zu Android Chronometer
- Die Anleitung zu Android OptionMenu
- Die Anleitung zu Android ContextMenu
- Die Anleitung zu Android PopupMenu
- Die Anleitung zu Android Fragment
- Die Anleitung zu Android ListView
- Android ListView mit Checkbox verwenden ArrayAdapter
- Die Anleitung zu Android GridView
Show More