Die Anleitung zu Android Internal Storage
1. Android Internal Storage
Android Internal Storage: ist ein Ort, wo speichert die individuellen Daten jeder Applikation. Diese Daten werden gespeichert und für die eigene Applikation benutzt. Die anderen Applikation können nicht zugreifen. Wenn die Applikation normaleweiser aus dem Gerät Android uninstalliert wird, werden die betreffenden Datenfile auch gelöscht
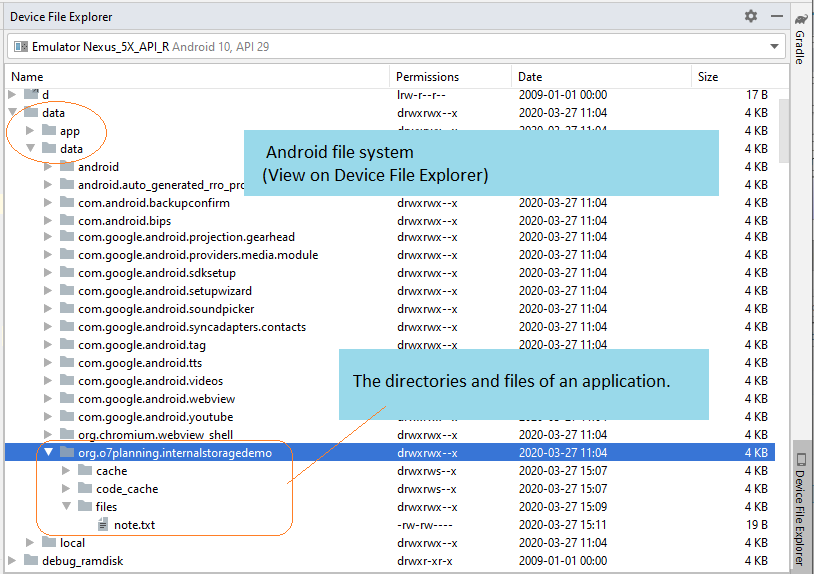
Ein noch mehr Merkmal beim Umgang mit der Datenfile in der internen Speicherung (Internal Storage) ist, dass Sie mit einem einfachen Filename bearbeiten können, und mit einer File mit einen Pfad nicht bearbeiten
Öffnen Sie die File des Daten-Aufschreiben
// Is a simple file name.
// Note!! Do not allow the path.
String simpleFileName ="note.txt";
// Open Stream to write file.
FileOutputStream out = openFileOutput(simpleFileName, MODE_PRIVATE);
Sie haben 4 Options zum Aufschreibensmodis:
Modus zur File-erstellung | Die Bezeichnung |
MODE_PRIVATE | Das ist Default-Modus, die geschriebene File wird nur von der Applikation, die sie erstellt, benutzt oder mit dem gleichen User ID getauscht |
MODE_APPEND | Das Modus zum Hinzufügen der Daten in die FIle wenn sie existiert |
MODE_ENABLE_WRITE_AHEAD_LOGGING | |
MODE_WORLD_READABLE | Der Modus ist sehr gefährlich. Er ist ein Sicherheitsloch im Android,es ist am besten, nicht zu benutzen,. Sie können die anderen alternativen Technik
|
MODE_WORLD_WRITEABLE | Der Modus ist sehr gefährlich. Er ist ein Sicherheitsloch im Android,es ist am besten, nicht zu benutzen,. Sie können die anderen alternativen Technik
|
MODE_MULTI_PROCESS | der Modus zum Multi-Prozess, der in die File schreiben darf. Aber es ist empfohlen, den Modus nicht zu benutzen denn er funktioniert in einigen Version von Android nicht. Sie können die anderen alternative Technik benutzen, zum Beispiel:
|
Öffnen Sie die File zum Lesen der Daten
// Is a simple file name.
// Note!! Do not allow the path.
String simpleFileName = "note.txt";
// Open stream to read file.
FileInputStream in = this.openFileInput(simpleFileName);
2. Zum Beispiel: die Daten in der internalen Speicherung lesen und schreiben
Jetzt können Sie ein Beispiel zum Aufschreiben der Daten in der File, die im inneren Speicher liegt, und zum Lesen des Daten der File, durch
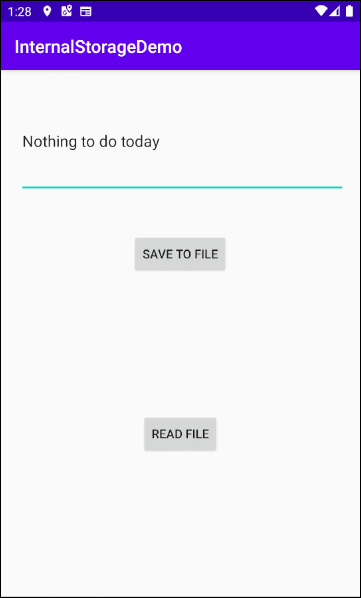
Erstellen Sie ein Projekt mit dem Name von InternalStorageDemo.
- File > New > New Project > Empty Activity
- Name: InternalStorageDemo
- Package name: org.o7planning.internalstoragedemo
- Language: Java
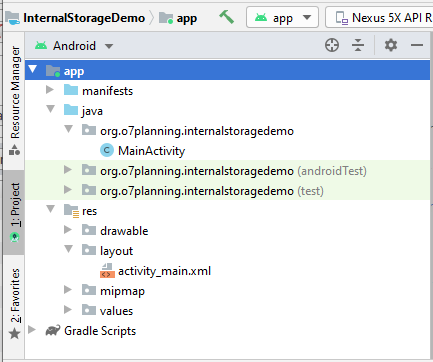
Die Interface der Applikation
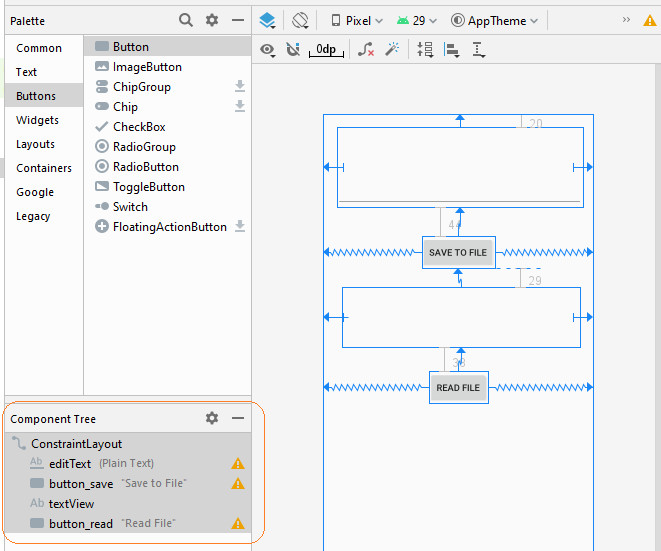
The interface of this application is very simple, if you are interested in the steps to create it, see the appendix at the end of the article.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<EditText
android:id="@+id/editText"
android:layout_width="0dp"
android:layout_height="122dp"
android:layout_marginStart="21dp"
android:layout_marginLeft="21dp"
android:layout_marginTop="20dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:ems="10"
android:inputType="textPersonName"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button_save"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="44dp"
android:text="Save to File"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editText" />
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="91dp"
android:layout_marginStart="29dp"
android:layout_marginLeft="29dp"
android:layout_marginTop="29dp"
android:layout_marginEnd="21dp"
android:layout_marginRight="21dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button_save" />
<Button
android:id="@+id/button_read"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="38dp"
android:text="Read File"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package org.o7planning.internalstoragedemo;
import android.os.Bundle;
import androidx.appcompat.app.AppCompatActivity;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import android.widget.Toast;
import java.io.BufferedReader;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.InputStreamReader;
public class MainActivity extends AppCompatActivity {
private Button saveButton;
private Button readButton;
private TextView textView;
private EditText editText;
// Is a simple file name.
// Note!! Do not allow the path.
private String simpleFileName = "note.txt";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.saveButton = (Button) this.findViewById(R.id.button_save);
this.readButton = (Button) this.findViewById(R.id.button_read);
this.textView = (TextView) this.findViewById(R.id.textView);
this.editText = (EditText) this.findViewById(R.id.editText);
this.saveButton.setOnClickListener(new Button.OnClickListener() {
@Override
public void onClick(View v) {
saveData();
}
});
this.readButton.setOnClickListener(new Button.OnClickListener() {
@Override
public void onClick(View v) {
readData();
}
});
}
private void saveData() {
String data = this.editText.getText().toString();
try {
// Open Stream to write file.
FileOutputStream out = this.openFileOutput(simpleFileName, MODE_PRIVATE);
// Ghi dữ liệu.
out.write(data.getBytes());
out.close();
Toast.makeText(this,"File saved!",Toast.LENGTH_SHORT).show();
} catch (Exception e) {
Toast.makeText(this,"Error:"+ e.getMessage(),Toast.LENGTH_SHORT).show();
}
}
private void readData() {
try {
// Open stream to read file.
FileInputStream in = this.openFileInput(simpleFileName);
BufferedReader br= new BufferedReader(new InputStreamReader(in));
StringBuilder sb= new StringBuilder();
String s= null;
while((s= br.readLine())!= null) {
sb.append(s).append("\n");
}
this.textView.setText(sb.toString());
} catch (Exception e) {
Toast.makeText(this,"Error:"+ e.getMessage(),Toast.LENGTH_SHORT).show();
}
}
}
Bei der Benutzung "Android Device Manager" können Sie die File, die im System erstellt werden, sehen
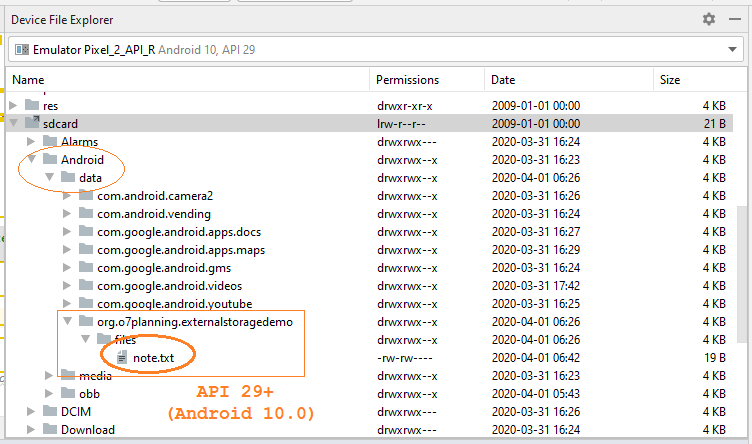
See more about "Device File Explorer":
Anleitungen Android
- Konfigurieren Sie Android Emulator in Android Studio
- Die Anleitung zu Android ToggleButton
- Erstellen Sie einen einfachen File Finder Dialog in Android
- Die Anleitung zu Android TimePickerDialog
- Die Anleitung zu Android DatePickerDialog
- Was ist erforderlich, um mit Android zu beginnen?
- Installieren Sie Android Studio unter Windows
- Installieren Sie Intel® HAXM für Android Studio
- Die Anleitung zu Android AsyncTask
- Die Anleitung zu Android AsyncTaskLoader
- Die Anleitung zum Android für den Anfänger - Grundlegende Beispiele
- Woher weiß man die Telefonnummer von Android Emulator und ändere es
- Die Anleitung zu Android TextInputLayout
- Die Anleitung zu Android CardView
- Die Anleitung zu Android ViewPager2
- Holen Sie sich die Telefonnummer in Android mit TelephonyManager
- Die Anleitung zu Android Phone Calls
- Die Anleitung zu Android Wifi Scanning
- Die Anleitung zum Android 2D Game für den Anfänger
- Die Anleitung zu Android DialogFragment
- Die Anleitung zu Android CharacterPickerDialog
- Die Anleitung zum Android für den Anfänger - Hello Android
- Verwenden des Android Device File Explorer
- Aktivieren Sie USB Debugging auf einem Android-Gerät
- Die Anleitung zu Android UI Layouts
- Die Anleitung zu Android SMS
- Die Anleitung zu Android SQLite Database
- Die Anleitung zu Google Maps Android API
- Text zu Sprache in Android
- Die Anleitung zu Android Space
- Die Anleitung zu Android Toast
- Erstellen Sie einen benutzerdefinierten Android Toast
- Die Anleitung zu Android SnackBar
- Die Anleitung zu Android TextView
- Die Anleitung zu Android TextClock
- Die Anleitung zu Android EditText
- Die Anleitung zu Android TextWatcher
- Formatieren Sie die Kreditkartennummer mit Android TextWatcher
- Die Anleitung zu Android Clipboard
- Erstellen Sie einen einfachen File Chooser in Android
- Die Anleitung zu Android AutoCompleteTextView und MultiAutoCompleteTextView
- Die Anleitung zu Android ImageView
- Die Anleitung zu Android ImageSwitcher
- Die Anleitung zu Android ScrollView und HorizontalScrollView
- Die Anleitung zu Android WebView
- Die Anleitung zu Android SeekBar
- Die Anleitung zu Android Dialog
- Die Anleitung zu Android AlertDialog
- Die Anleitung zu Android RatingBar
- Die Anleitung zu Android ProgressBar
- Die Anleitung zu Android Spinner
- Die Anleitung zu Android Button
- Die Anleitung zu Android Switch
- Die Anleitung zu Android ImageButton
- Die Anleitung zu Android FloatingActionButton
- Die Anleitung zu Android CheckBox
- Die Anleitung zu Android RadioGroup und RadioButton
- Die Anleitung zu Android Chip und ChipGroup
- Verwenden Sie Image Asset und Icon Asset von Android Studio
- Richten Sie die SDCard für den Emulator ein
- ChipGroup und Chip Entry Beispiel
- Hinzufügen externer Bibliotheken zu Android Project in Android Studio
- Wie deaktiviere ich die Berechtigungen, die der Android-Anwendung bereits erteilt wurden?
- Wie entferne ich Anwendungen aus dem Android Emulator?
- Die Anleitung zu Android LinearLayout
- Die Anleitung zu Android TableLayout
- Die Anleitung zu Android FrameLayout
- Die Anleitung zu Android QuickContactBadge
- Die Anleitung zu Android StackView
- Die Anleitung zu Android Camera
- Die Anleitung zu Android MediaPlayer
- Die Anleitung zu Android VideoView
- Spielen Sie Sound-Effekte in Android mit SoundPool
- Die Anleitung zu Android Networking
- Die Anleitung zu Android JSON Parser
- Die Anleitung zu Android SharedPreferences
- Die Anleitung zu Android Internal Storage
- Die Anleitung zu Android External Storage
- Die Anleitung zu Android Intents
- Beispiel für eine explizite Android Intent, nennen Sie eine andere Intent
- Beispiel für implizite Android Intent, Öffnen Sie eine URL, senden Sie eine Email
- Die Anleitung zu Android Services
- Die Anleitung zu Android Notifications
- Die Anleitung zu Android DatePicker
- Die Anleitung zu Android TimePicker
- Die Anleitung zu Android Chronometer
- Die Anleitung zu Android OptionMenu
- Die Anleitung zu Android ContextMenu
- Die Anleitung zu Android PopupMenu
- Die Anleitung zu Android Fragment
- Die Anleitung zu Android ListView
- Android ListView mit Checkbox verwenden ArrayAdapter
- Die Anleitung zu Android GridView
Show More