Spielen Sie Sound-Effekte in Android mit SoundPool
1. SoundPool & AudioManager
Zuerst lege ich eine Situation, Sie machen ein Game, darin es die Audio wie Ton des Gewehr, der Bombe gibt. Das sind die Audioseffekt im Spiel. Android bietet Sie eine Klasse SoundPool, es ist so gleich wie eine Enthalter der Musik-Quelle und es ist bereit, nach der Anforderung zu spielen.
SoundPool enthaltet eine Kollektion der Musik-Quelle, der Audio-Quelle, die in der Musik-File in der Applikation oder in der Systemsfile ... liegen. SoundPool unterstützt viele Musik einmal zu spielen.
SourcePool benutzt MediaPlayer service um das Ton zu spielen
SoundPool enthaltet eine Kollektion der Musik-Quelle, der Audio-Quelle, die in der Musik-File in der Applikation oder in der Systemsfile ... liegen. SoundPool unterstützt viele Musik einmal zu spielen.
SourcePool benutzt MediaPlayer service um das Ton zu spielen
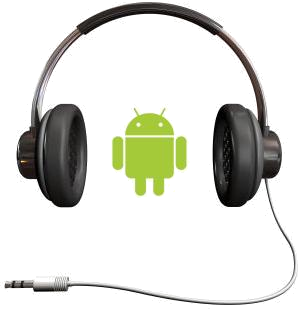
Sie können SoundPool bestimmen um das Audio bei einem bestimmten stream zu spielen. Es gibt einige folgenden Streamstypen:
Audio-Stream | Die Bezeichnung |
AudioManager.STREAM_ALARM | Die Audio-Stream für die Warnung |
AudioManager.STREAM DTMF | Die Audio-Stream für DTMF |
AudioManager.STREAM_MUSIC | Die Audio-Stream für das Playback der Musik |
AudioManager.STREAM_NOTIFICATION | Die Audio-Stream für die Benachrichtigung |
AudioManager.STREAM_RING | Die Audio-Stream für die Telefonklingel |
AudioManager.STREAM_SYSTEM | Die Audio-Stream für die System-Ton |
AudioManager.STREAM_VOICE_CALL | Die Audio-Stream für den Anruf |
AudioManager erlaubt Sie, das Volume des unterschiedlichen Audio-Stream einzustellen. Zum Beispiel, Sie hören ein Lied während des Gamespielen auf einen Gerät, können Sie das Volume des Game ohne die Auswirkung der Qualität der Liedsvolume einstellen
Ein Problem wird erwähnt, dass ein Audio-Gerät den Ton aussendet.
Mit STREAM_MUSIC wird das Ton durch ein Audio-Gerät verbindet mit dem Handy ausgesendet (Lautsprecher des Handy, Kopfhörer, Bluetooth-Lautsprecher ...).
Mit STREAM_RING wird das Ton durch alle Audio-Gerät verbindend mit dem Handy ausgesendet. Diese Aktion wird unterschied in jedem Gerät.
Mit STREAM_MUSIC wird das Ton durch ein Audio-Gerät verbindet mit dem Handy ausgesendet (Lautsprecher des Handy, Kopfhörer, Bluetooth-Lautsprecher ...).
Mit STREAM_RING wird das Ton durch alle Audio-Gerät verbindend mit dem Handy ausgesendet. Diese Aktion wird unterschied in jedem Gerät.
SoundPool API:
Die Methode | Die Bezeichnung |
int play(int soundID, float leftVolume, float rightVolume,
int priority, int loop, float rate) | Ein Musik spielen und ein ID des spielenden Stream rückgeben (streamID). |
void pause(int streamID) | Das spielende Audio-Stream mit ID : streamID pausen. |
void stop(int streamID) | Das Audio-Stream mit dem entsprechenden streamID.stoppen |
void setVolume(int streamID, float leftVolume, float rightVolume) | Die Volume für das Audio-Stream mit ID: streamID setzen |
void setLoop(int streamID, int loop) | Die Häufigkeit des Playback des Audio-Stream mit ID: streamID. setzen |
2. Das Beispiel mit SoundPool
Erstellen Sie ein Projekt SoundPoolDemo.
- Name: SoundPoolDemo
- Package name: org.o7planning.soundpooldemo
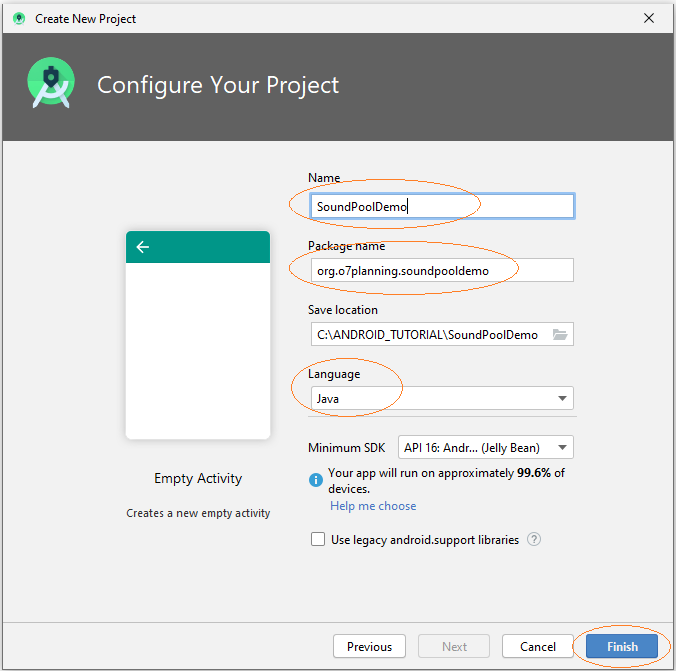
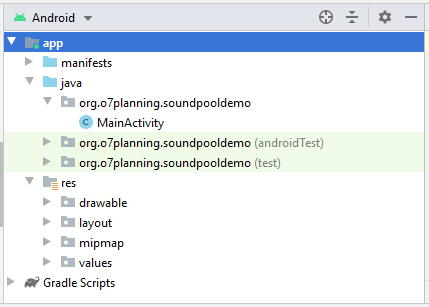
Erstellen Sie den Ordner raw , der der SubOrdner des Ordner res ist. Kopieren Sie 2 Musik-File in dem Ordner raw, Hier kopiere ich 2 File für die Audiosimulation der zerstörten Gegenstand (destroy.wav) und des Gewehr (gun.wav). Beachten Sie, dass Sie die Musik-File mit der Ende mp3 oder wav benutzen können.
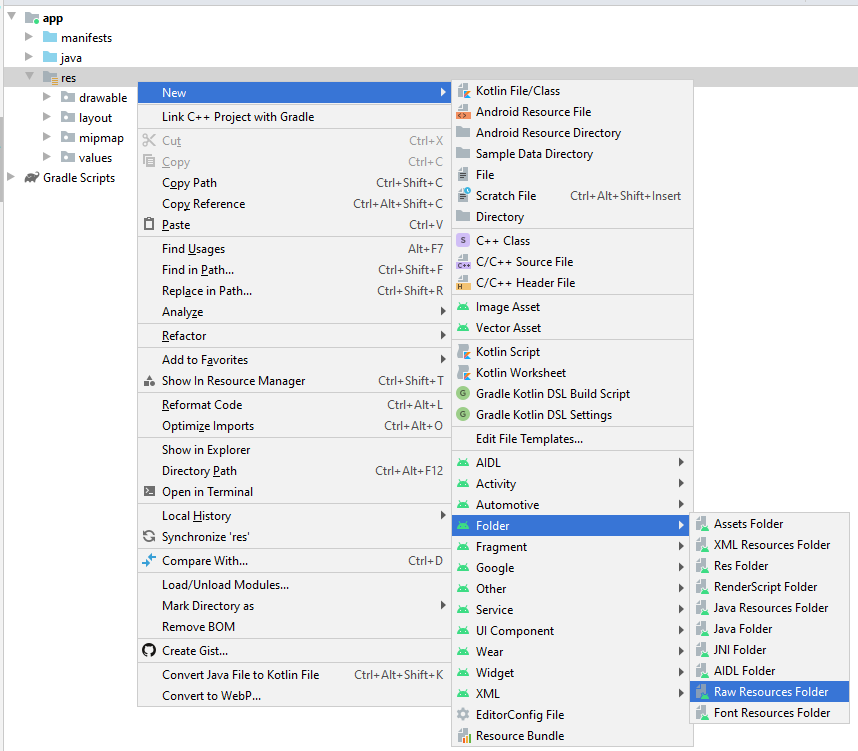
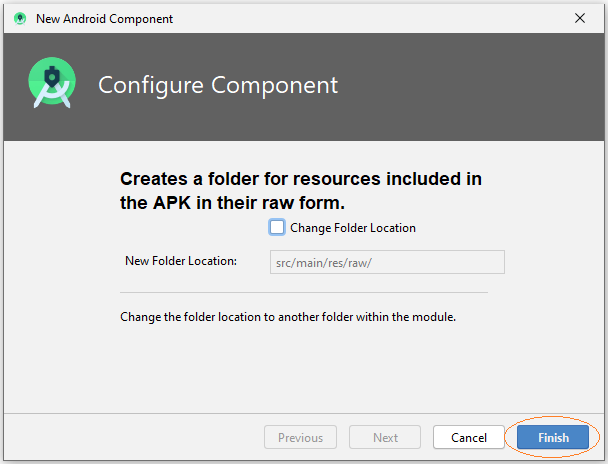
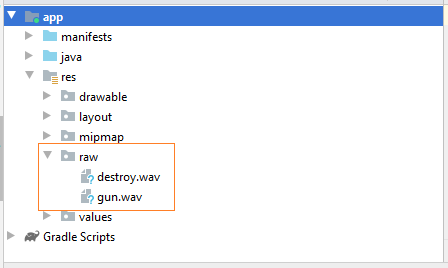
Das ist die Interface der Applikation Demo.
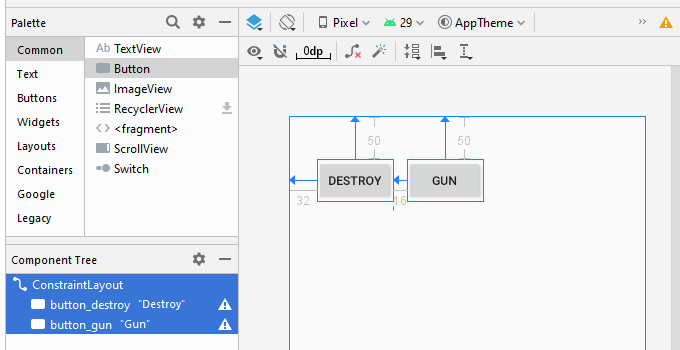
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/button_destroy"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="32dp"
android:layout_marginLeft="32dp"
android:layout_marginTop="50dp"
android:text="Destroy"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button_gun"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="50dp"
android:text="Gun"
app:layout_constraintStart_toEndOf="@+id/button_destroy"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package org.o7planning.soundpooldemo;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.media.AudioAttributes;
import android.media.AudioManager;
import android.media.SoundPool;
import android.os.Build;
import android.view.View;
import android.widget.Button;
public class MainActivity extends AppCompatActivity {
private SoundPool soundPool;
private AudioManager audioManager;
private Button buttonDestroy;
private Button buttonGun;
// Maximumn sound stream.
private static final int MAX_STREAMS = 5;
// Stream type.
private static final int streamType = AudioManager.STREAM_MUSIC;
private boolean loaded;
private int soundIdDestroy;
private int soundIdGun;
private float volume;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.buttonDestroy = (Button) this.findViewById(R.id.button_destroy);
this.buttonGun = (Button) this.findViewById(R.id.button_gun);
this.buttonDestroy.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
playSoundDestroy();
}
});
this.buttonGun.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
playSoundGun();
}
});
// AudioManager audio settings for adjusting the volume
audioManager = (AudioManager) getSystemService(AUDIO_SERVICE);
// Current volumn Index of particular stream type.
float currentVolumeIndex = (float) audioManager.getStreamVolume(streamType);
// Get the maximum volume index for a particular stream type.
float maxVolumeIndex = (float) audioManager.getStreamMaxVolume(streamType);
// Volumn (0 --> 1)
this.volume = currentVolumeIndex / maxVolumeIndex;
// Suggests an audio stream whose volume should be changed by
// the hardware volume controls.
this.setVolumeControlStream(streamType);
// For Android SDK >= 21
if (Build.VERSION.SDK_INT >= 21 ) {
AudioAttributes audioAttrib = new AudioAttributes.Builder()
.setUsage(AudioAttributes.USAGE_GAME)
.setContentType(AudioAttributes.CONTENT_TYPE_SONIFICATION)
.build();
SoundPool.Builder builder= new SoundPool.Builder();
builder.setAudioAttributes(audioAttrib).setMaxStreams(MAX_STREAMS);
this.soundPool = builder.build();
}
// for Android SDK < 21
else {
// SoundPool(int maxStreams, int streamType, int srcQuality)
this.soundPool = new SoundPool(MAX_STREAMS, AudioManager.STREAM_MUSIC, 0);
}
// When Sound Pool load complete.
this.soundPool.setOnLoadCompleteListener(new SoundPool.OnLoadCompleteListener() {
@Override
public void onLoadComplete(SoundPool soundPool, int sampleId, int status) {
loaded = true;
}
});
// Load sound file (destroy.wav) into SoundPool.
this.soundIdDestroy = this.soundPool.load(this, R.raw.destroy,1);
// Load sound file (gun.wav) into SoundPool.
this.soundIdGun = this.soundPool.load(this, R.raw.gun,1);
}
// When users click on the button "Gun"
public void playSoundGun( ) {
if(loaded) {
float leftVolumn = volume;
float rightVolumn = volume;
// Play sound of gunfire. Returns the ID of the new stream.
int streamId = this.soundPool.play(this.soundIdGun,leftVolumn, rightVolumn, 1, 0, 1f);
}
}
// When users click on the button "Destroy"
public void playSoundDestroy( ) {
if(loaded) {
float leftVolumn = volume;
float rightVolumn = volume;
// Play sound objects destroyed. Returns the ID of the new stream.
int streamId = this.soundPool.play(this.soundIdDestroy,leftVolumn, rightVolumn, 1, 0, 1f);
}
}
}
Die Applikation durchführen
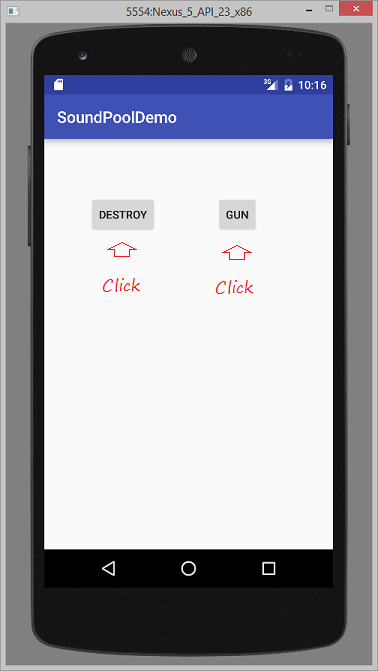
Anleitungen Android
- Konfigurieren Sie Android Emulator in Android Studio
- Die Anleitung zu Android ToggleButton
- Erstellen Sie einen einfachen File Finder Dialog in Android
- Die Anleitung zu Android TimePickerDialog
- Die Anleitung zu Android DatePickerDialog
- Was ist erforderlich, um mit Android zu beginnen?
- Installieren Sie Android Studio unter Windows
- Installieren Sie Intel® HAXM für Android Studio
- Die Anleitung zu Android AsyncTask
- Die Anleitung zu Android AsyncTaskLoader
- Die Anleitung zum Android für den Anfänger - Grundlegende Beispiele
- Woher weiß man die Telefonnummer von Android Emulator und ändere es
- Die Anleitung zu Android TextInputLayout
- Die Anleitung zu Android CardView
- Die Anleitung zu Android ViewPager2
- Holen Sie sich die Telefonnummer in Android mit TelephonyManager
- Die Anleitung zu Android Phone Calls
- Die Anleitung zu Android Wifi Scanning
- Die Anleitung zum Android 2D Game für den Anfänger
- Die Anleitung zu Android DialogFragment
- Die Anleitung zu Android CharacterPickerDialog
- Die Anleitung zum Android für den Anfänger - Hello Android
- Verwenden des Android Device File Explorer
- Aktivieren Sie USB Debugging auf einem Android-Gerät
- Die Anleitung zu Android UI Layouts
- Die Anleitung zu Android SMS
- Die Anleitung zu Android SQLite Database
- Die Anleitung zu Google Maps Android API
- Text zu Sprache in Android
- Die Anleitung zu Android Space
- Die Anleitung zu Android Toast
- Erstellen Sie einen benutzerdefinierten Android Toast
- Die Anleitung zu Android SnackBar
- Die Anleitung zu Android TextView
- Die Anleitung zu Android TextClock
- Die Anleitung zu Android EditText
- Die Anleitung zu Android TextWatcher
- Formatieren Sie die Kreditkartennummer mit Android TextWatcher
- Die Anleitung zu Android Clipboard
- Erstellen Sie einen einfachen File Chooser in Android
- Die Anleitung zu Android AutoCompleteTextView und MultiAutoCompleteTextView
- Die Anleitung zu Android ImageView
- Die Anleitung zu Android ImageSwitcher
- Die Anleitung zu Android ScrollView und HorizontalScrollView
- Die Anleitung zu Android WebView
- Die Anleitung zu Android SeekBar
- Die Anleitung zu Android Dialog
- Die Anleitung zu Android AlertDialog
- Die Anleitung zu Android RatingBar
- Die Anleitung zu Android ProgressBar
- Die Anleitung zu Android Spinner
- Die Anleitung zu Android Button
- Die Anleitung zu Android Switch
- Die Anleitung zu Android ImageButton
- Die Anleitung zu Android FloatingActionButton
- Die Anleitung zu Android CheckBox
- Die Anleitung zu Android RadioGroup und RadioButton
- Die Anleitung zu Android Chip und ChipGroup
- Verwenden Sie Image Asset und Icon Asset von Android Studio
- Richten Sie die SDCard für den Emulator ein
- ChipGroup und Chip Entry Beispiel
- Hinzufügen externer Bibliotheken zu Android Project in Android Studio
- Wie deaktiviere ich die Berechtigungen, die der Android-Anwendung bereits erteilt wurden?
- Wie entferne ich Anwendungen aus dem Android Emulator?
- Die Anleitung zu Android LinearLayout
- Die Anleitung zu Android TableLayout
- Die Anleitung zu Android FrameLayout
- Die Anleitung zu Android QuickContactBadge
- Die Anleitung zu Android StackView
- Die Anleitung zu Android Camera
- Die Anleitung zu Android MediaPlayer
- Die Anleitung zu Android VideoView
- Spielen Sie Sound-Effekte in Android mit SoundPool
- Die Anleitung zu Android Networking
- Die Anleitung zu Android JSON Parser
- Die Anleitung zu Android SharedPreferences
- Die Anleitung zu Android Internal Storage
- Die Anleitung zu Android External Storage
- Die Anleitung zu Android Intents
- Beispiel für eine explizite Android Intent, nennen Sie eine andere Intent
- Beispiel für implizite Android Intent, Öffnen Sie eine URL, senden Sie eine Email
- Die Anleitung zu Android Services
- Die Anleitung zu Android Notifications
- Die Anleitung zu Android DatePicker
- Die Anleitung zu Android TimePicker
- Die Anleitung zu Android Chronometer
- Die Anleitung zu Android OptionMenu
- Die Anleitung zu Android ContextMenu
- Die Anleitung zu Android PopupMenu
- Die Anleitung zu Android Fragment
- Die Anleitung zu Android ListView
- Android ListView mit Checkbox verwenden ArrayAdapter
- Die Anleitung zu Android GridView
Show More