Die Anleitung zu Android PopupMenu
1. Android Popup Menu
In Android ist das Popup Menu ein schwebendes Menü, das Sie erstellen und in jeder View (Ansicht) verankern können. In Bezug auf die Interface und Verwendung gibt es keinen Unterschied zu einem Context Menu.
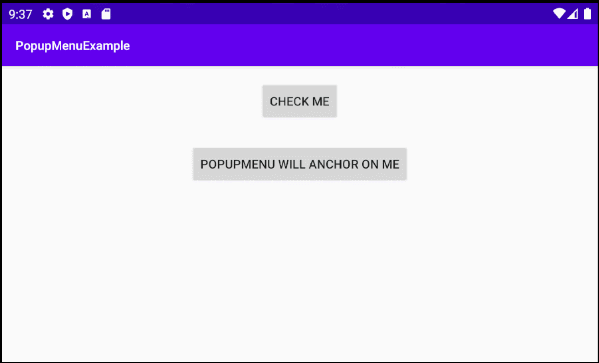
Sie können Android Resource File (XML) verwenden, um die Interface eines PopupMenu zu entwerfen. Das PopupMenu einer Anwendung ist jedoch recht einfach. Daher ist es auch eine gute Wahl, ein PopupMenu vollständig aus Java-Code zu erstellen (siehe Beispiel unten).
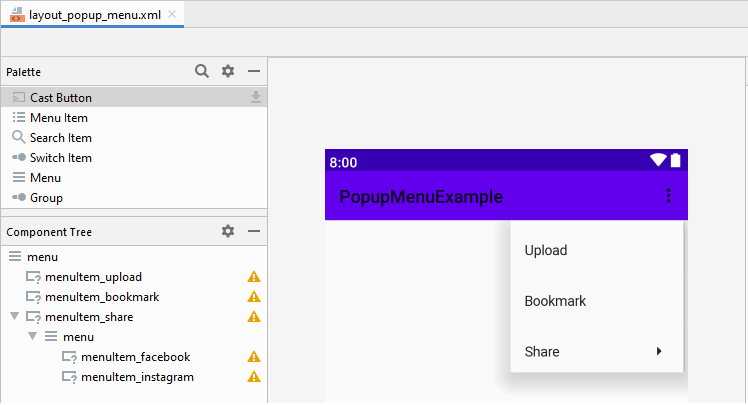
Hinweis: Einige Attribute des <item> (Menu Item) haben keine Auswirkung, wenn sie in ein Popup Menu eingefügt werden. Beispiel:
- app:showAsAction
- android:icon (Not work in Android 3.0+/API 11+)
2. Ein Beispiel für das Popup-Menu
In Android Studio erstellen Sie ein neues Projekt.
- File > New > New Project > Empty Activity
- Name: PopupMenu
- Package name: org.o7planning.popupmenuexample
- Language: Java
Achtung: Seit Android 3.0 (API 11) unterstützt PopupMenu keine icons (Symbole) mehr. Sie können jedoch einige der folgenden icons (Symbole) in den drawable folder (Zeichenordner) kopieren, um ältere Android-Geräte zu unterstützen, oder Sie können diesen Schritt einfach überspringen
icon_upload.png | icon_bookmark.png | icon_share.png |
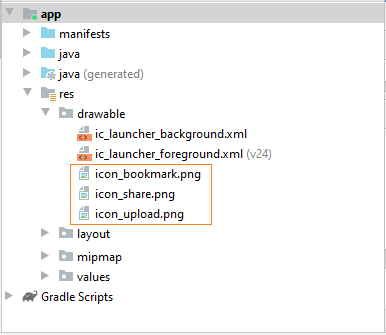
Die Haupt-Interface der Anwendung ist mit 2 Buttons (Tasten) sehr einfach. Wenn Benutzer auf Button1 klicken, wird ein PopupMenu angezeigt und in Button2 verankert.
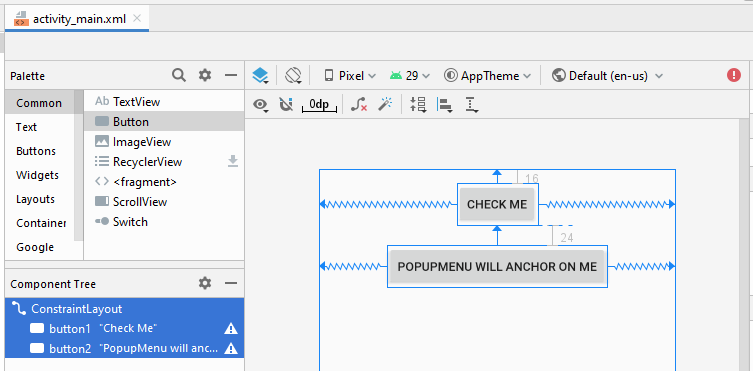
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:text="Check Me"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="24dp"
android:text="PopupMenu will anchor on me"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button1" />
</androidx.constraintlayout.widget.ConstraintLayout>
In Android Studio wählen Sie:
- File > New > Android Resource File
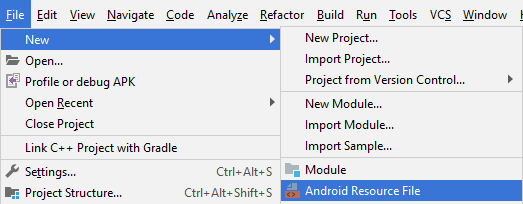
- File name: layout_popup_menu.xml
- Resource type: Menu
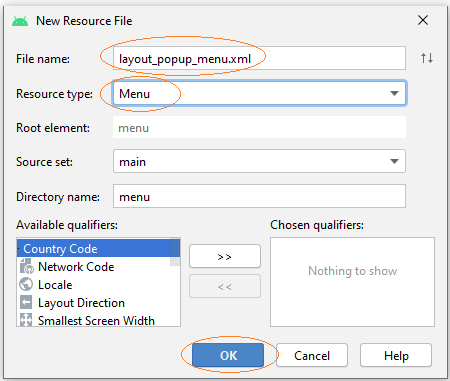
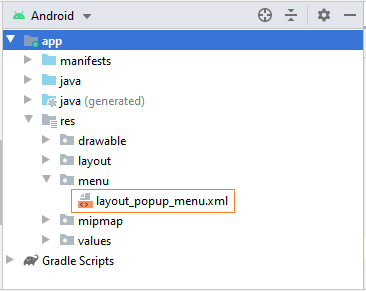
In Android Studio entwerfen Sie die Interface für Popup Menu:
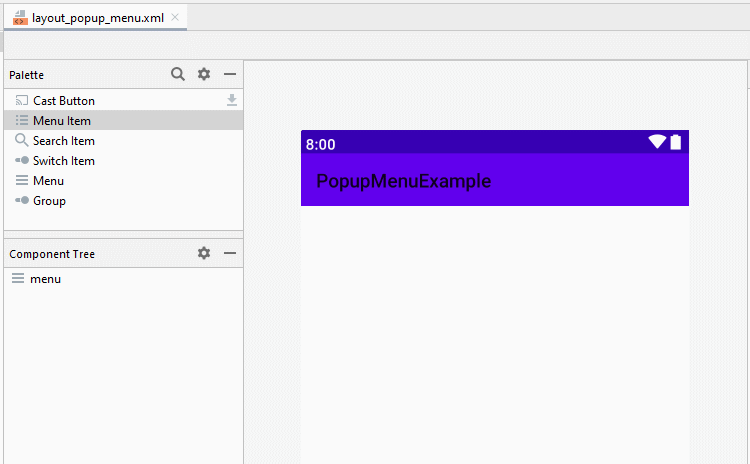
Legen Sie ID, Title, Icon für Menu Item fest:
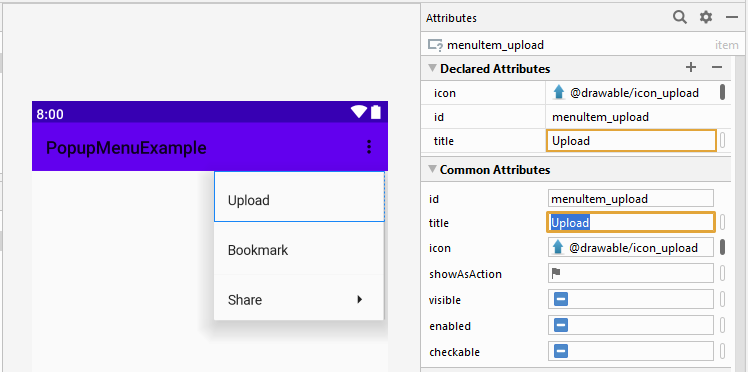
layout_popup_menu.xml
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:android="http://schemas.android.com/apk/res/android">
<item
android:id="@+id/menuItem_upload"
android:icon="@drawable/icon_upload"
android:title="Upload" />
<item
android:id="@+id/menuItem_bookmark"
android:icon="@drawable/icon_bookmark"
android:title="Bookmark" />
<item
android:id="@+id/menuItem_share"
android:icon="@drawable/icon_share"
android:title="Share">
<menu>
<item
android:id="@+id/menuItem_facebook"
android:title="Facebook" />
<item
android:id="@+id/menuItem_instagram"
android:title="Instagram" />
</menu>
</item>
</menu>
MainActivity.java
package org.o7planning.popupmenuexample;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.widget.Button;
import android.widget.PopupMenu;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
public static final String LOG_TAG = "PopupMenuExample";
private Button button1;
private Button button2;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.button1 = (Button) findViewById(R.id.button1);
this.button2 = (Button) findViewById(R.id.button2);
this.button1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
button1Clicked( );
}
});
}
// User click on the Button 1.
private void button1Clicked( ) {
// When user click on the Button 1, create a PopupMenu.
// And anchor Popup to the Button 2.
PopupMenu popup = new PopupMenu(this, this.button2);
popup.inflate(R.menu.layout_popup_menu);
Menu menu = popup.getMenu();
// com.android.internal.view.menu.MenuBuilder
Log.i(LOG_TAG, "Menu class: " + menu.getClass().getName());
// Register Menu Item Click event.
popup.setOnMenuItemClickListener(new PopupMenu.OnMenuItemClickListener() {
@Override
public boolean onMenuItemClick(MenuItem item) {
return menuItemClicked(item);
}
});
// Show the PopupMenu.
popup.show();
}
// When user click on Menu Item.
// @return true if event was handled.
private boolean menuItemClicked(MenuItem item) {
switch (item.getItemId()) {
case R.id.menuItem_bookmark:
Toast.makeText(this, "Bookmark", Toast.LENGTH_SHORT).show();
break;
case R.id.menuItem_upload:
Toast.makeText(this, "Upload", Toast.LENGTH_SHORT).show();
break;
case R.id.menuItem_facebook:
Toast.makeText(this, "Share Facebook", Toast.LENGTH_SHORT).show();
break;
case R.id.menuItem_instagram:
Toast.makeText(this, "Share Instagram", Toast.LENGTH_SHORT).show();
break;
default:
Toast.makeText(this, item.getTitle(), Toast.LENGTH_SHORT).show();
break;
}
return true;
}
}
Sie können PopupMenu durch die Java Code auch erstellen:
// Create a PopupMenu using Java.
public void createPopupMenu(Context activity, View anchorView ) {
// Create a PopupMenu and anchor it on a View.
PopupMenu popupMenu = new PopupMenu(activity, anchorView);
Menu menu = popupMenu.getMenu();
// groupId, itemId, order, title
MenuItem menuItemUpload = menu.add(1, 1, 1, "Upload");
MenuItem menuItemBookmark = menu.add(2, 2, 2, "Bookmark");
menuItemUpload.setIcon(R.drawable.icon_upload);
menuItemBookmark.setIcon(R.drawable.icon_bookmark);
// groupId, itemId, order, title
SubMenu subMenuShare= menu.addSubMenu(3, 3, 3, "Share");
subMenuShare.setIcon(R.drawable.icon_share);
subMenuShare.add(4, 31, 1, "Google" );
subMenuShare.add(5, 32, 2, "Instagram");
}
Anleitungen Android
- Konfigurieren Sie Android Emulator in Android Studio
- Die Anleitung zu Android ToggleButton
- Erstellen Sie einen einfachen File Finder Dialog in Android
- Die Anleitung zu Android TimePickerDialog
- Die Anleitung zu Android DatePickerDialog
- Was ist erforderlich, um mit Android zu beginnen?
- Installieren Sie Android Studio unter Windows
- Installieren Sie Intel® HAXM für Android Studio
- Die Anleitung zu Android AsyncTask
- Die Anleitung zu Android AsyncTaskLoader
- Die Anleitung zum Android für den Anfänger - Grundlegende Beispiele
- Woher weiß man die Telefonnummer von Android Emulator und ändere es
- Die Anleitung zu Android TextInputLayout
- Die Anleitung zu Android CardView
- Die Anleitung zu Android ViewPager2
- Holen Sie sich die Telefonnummer in Android mit TelephonyManager
- Die Anleitung zu Android Phone Calls
- Die Anleitung zu Android Wifi Scanning
- Die Anleitung zum Android 2D Game für den Anfänger
- Die Anleitung zu Android DialogFragment
- Die Anleitung zu Android CharacterPickerDialog
- Die Anleitung zum Android für den Anfänger - Hello Android
- Verwenden des Android Device File Explorer
- Aktivieren Sie USB Debugging auf einem Android-Gerät
- Die Anleitung zu Android UI Layouts
- Die Anleitung zu Android SMS
- Die Anleitung zu Android SQLite Database
- Die Anleitung zu Google Maps Android API
- Text zu Sprache in Android
- Die Anleitung zu Android Space
- Die Anleitung zu Android Toast
- Erstellen Sie einen benutzerdefinierten Android Toast
- Die Anleitung zu Android SnackBar
- Die Anleitung zu Android TextView
- Die Anleitung zu Android TextClock
- Die Anleitung zu Android EditText
- Die Anleitung zu Android TextWatcher
- Formatieren Sie die Kreditkartennummer mit Android TextWatcher
- Die Anleitung zu Android Clipboard
- Erstellen Sie einen einfachen File Chooser in Android
- Die Anleitung zu Android AutoCompleteTextView und MultiAutoCompleteTextView
- Die Anleitung zu Android ImageView
- Die Anleitung zu Android ImageSwitcher
- Die Anleitung zu Android ScrollView und HorizontalScrollView
- Die Anleitung zu Android WebView
- Die Anleitung zu Android SeekBar
- Die Anleitung zu Android Dialog
- Die Anleitung zu Android AlertDialog
- Die Anleitung zu Android RatingBar
- Die Anleitung zu Android ProgressBar
- Die Anleitung zu Android Spinner
- Die Anleitung zu Android Button
- Die Anleitung zu Android Switch
- Die Anleitung zu Android ImageButton
- Die Anleitung zu Android FloatingActionButton
- Die Anleitung zu Android CheckBox
- Die Anleitung zu Android RadioGroup und RadioButton
- Die Anleitung zu Android Chip und ChipGroup
- Verwenden Sie Image Asset und Icon Asset von Android Studio
- Richten Sie die SDCard für den Emulator ein
- ChipGroup und Chip Entry Beispiel
- Hinzufügen externer Bibliotheken zu Android Project in Android Studio
- Wie deaktiviere ich die Berechtigungen, die der Android-Anwendung bereits erteilt wurden?
- Wie entferne ich Anwendungen aus dem Android Emulator?
- Die Anleitung zu Android LinearLayout
- Die Anleitung zu Android TableLayout
- Die Anleitung zu Android FrameLayout
- Die Anleitung zu Android QuickContactBadge
- Die Anleitung zu Android StackView
- Die Anleitung zu Android Camera
- Die Anleitung zu Android MediaPlayer
- Die Anleitung zu Android VideoView
- Spielen Sie Sound-Effekte in Android mit SoundPool
- Die Anleitung zu Android Networking
- Die Anleitung zu Android JSON Parser
- Die Anleitung zu Android SharedPreferences
- Die Anleitung zu Android Internal Storage
- Die Anleitung zu Android External Storage
- Die Anleitung zu Android Intents
- Beispiel für eine explizite Android Intent, nennen Sie eine andere Intent
- Beispiel für implizite Android Intent, Öffnen Sie eine URL, senden Sie eine Email
- Die Anleitung zu Android Services
- Die Anleitung zu Android Notifications
- Die Anleitung zu Android DatePicker
- Die Anleitung zu Android TimePicker
- Die Anleitung zu Android Chronometer
- Die Anleitung zu Android OptionMenu
- Die Anleitung zu Android ContextMenu
- Die Anleitung zu Android PopupMenu
- Die Anleitung zu Android Fragment
- Die Anleitung zu Android ListView
- Android ListView mit Checkbox verwenden ArrayAdapter
- Die Anleitung zu Android GridView
Show More