Die Anleitung zu Android QuickContactBadge
1. Android QuickContactBadge
Android QuickContactBadge ist eine Unterklasse von ImageView. Es wird als kleines Abzeichen angezeigt, auf das der Benutzer klickt, um schnell einen Kontakt zu erstellen, z.B das Hinzufügen einer Telefonnumer zu den Kontakten, das Hinzufügen einer Email zu den Kontakten usw.
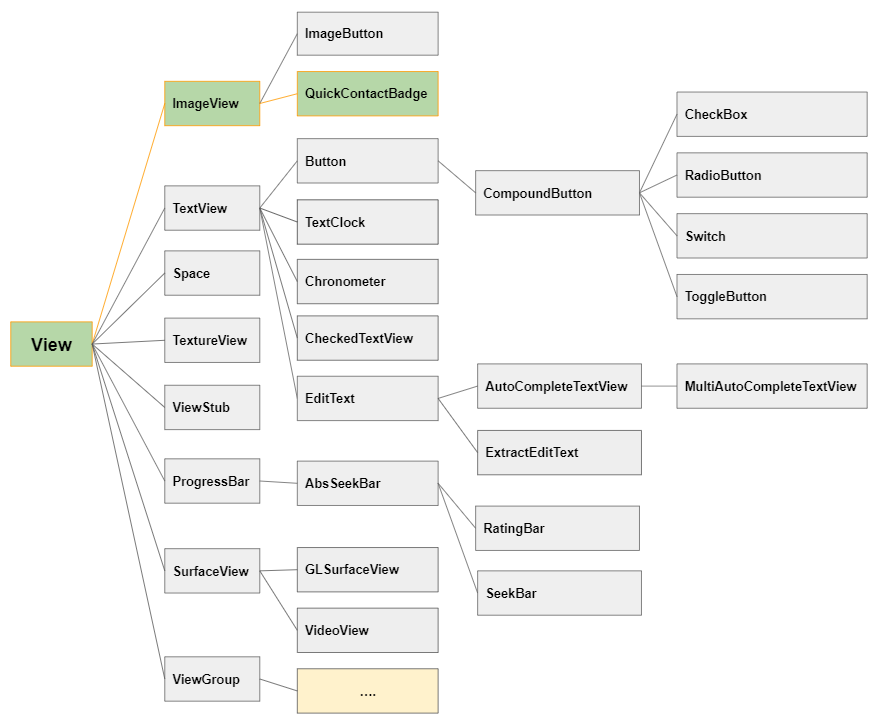
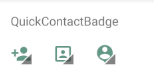
Hinweis: QuickContactBadge ist in Palette des Design-Fenster nicht verfügbar. Sie müssen daher den folgenden Code XML verwenden, um QuickContactBadge in die Interface hinzufügen.
QuickContactBadge
<QuickContactBadge
android:id="@+id/quickContactBadge11"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/icon_contact_add" ...>
</QuickContactBadge>
Getreu dem Namen "Quick Contact Badge" können Sie mit nur kurzen Code ganz einfach ein kleines "Badge" erstellen, auf das der Benutzer klicken kann, um einen Kontakt hinzufügen.
Beispiel: Legen Sie die Parameter für ein QuickContactBadge fest, um den Kontakten eine bestimmte Telefonnummer hinzufügen. Sie müssen nicht sogar die Behandlung des Ereignis OnClick für QuickContactBadge schreiben.
this.quickContactBadgePhone = (QuickContactBadge) findViewById(R.id.quickContactBadge_phone);
this.quickContactBadgePhone.assignContactFromPhone("+1-555-521-5554", true);
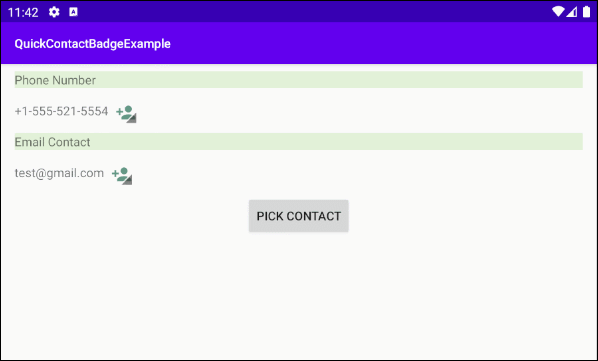
Sie können die neu hinzugefügten Telefonnumer zu den Kontakten sehen:
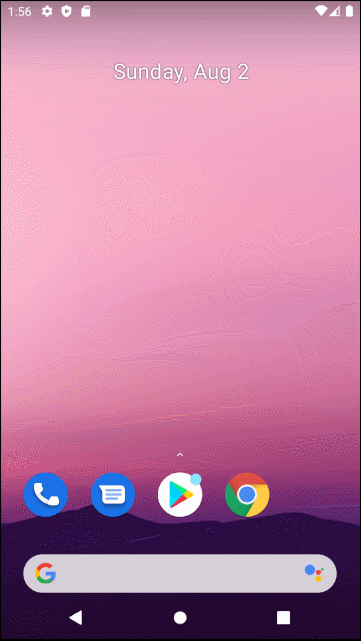
2. Die wichtigen Methode
Die wichtigen Methode von QuickContactBadge:
- assignContactFromPhone(String phoneNumber, boolean lazyLookup, Bundle extras)
- assignContactFromPhone(String phoneNumber, boolean lazyLookup)
- assignContactFromEmail(String emailAddress, boolean lazyLookup, Bundle extras)
- assignContactFromEmail(String emailAddress, boolean lazyLookup)
- assignContactUri(Uri contactUri)
3. Zum Beispiel: QuickContactBadge
In diesem Beispiel haben wir zwei QuickContactBadge. Mit dem ersten QuickContactBadge kann der Benutzer eine Telefonnummer schnell zu den Kontakten hinzufügen, und mit dem zweiten QuickContactBadge kann der Benutzer schnell eine Email zu den Kontakten hinzufügen.
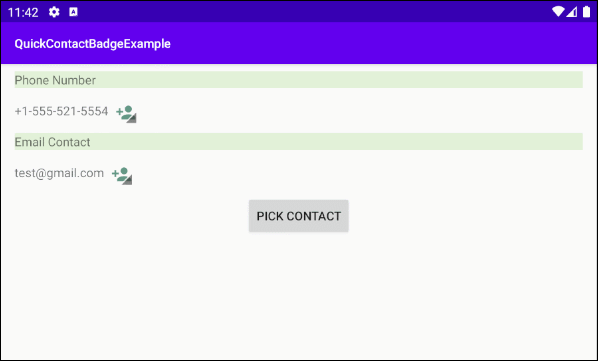
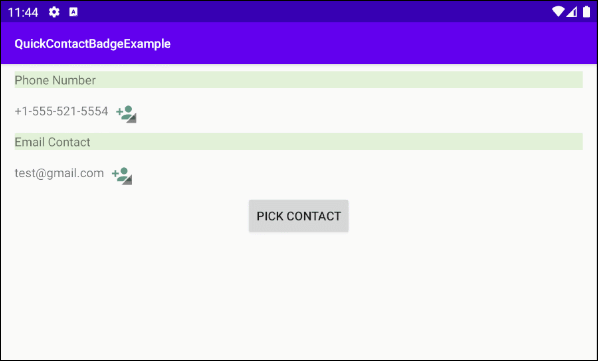
Mit Button "Pick Contact" kann der Benutzer einen vorhandenen Kontakt in den Kontakten auswählen.
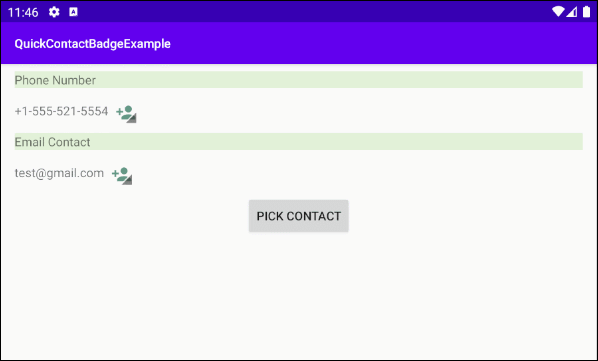
OK, Auf Android Studio erstellen Sie ein neues Projekt:
- File > New > New Project > Empty Activity
- Name: QuickContactBadgeExample
- Package name: com.example.quickcontactbadgeexample
- Language: Java
Die Interface des Beispiel sieht wie folgend aus:
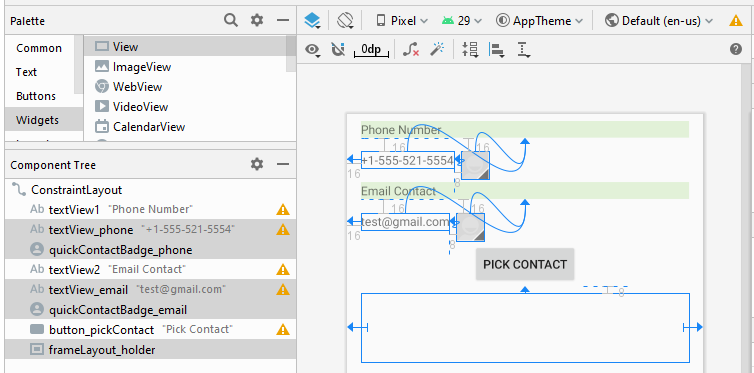
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView1"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:background="#E2F1D8"
android:text="Phone Number"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.0"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<TextView
android:id="@+id/textView_phone"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginTop="16dp"
android:inputType="textEmailAddress"
android:text="+1-555-521-5554"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView1" />
<QuickContactBadge
android:id="@+id/quickContactBadge_phone"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginTop="16dp"
android:scaleType="centerCrop"
android:src="@drawable/icon_contact_add"
app:layout_constraintStart_toEndOf="@+id/textView_phone"
app:layout_constraintTop_toBottomOf="@+id/textView1"></QuickContactBadge>
<TextView
android:id="@+id/textView2"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:background="#E2F1D8"
android:text="Email Contact"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.0"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView_phone" />
<TextView
android:id="@+id/textView_email"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginTop="16dp"
android:inputType="textEmailAddress"
android:text="test@gmail.com"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView2" />
<QuickContactBadge
android:id="@+id/quickContactBadge_email"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginTop="16dp"
android:scaleType="centerCrop"
android:src="@drawable/icon_contact_add"
app:layout_constraintStart_toEndOf="@+id/textView_email"
app:layout_constraintTop_toBottomOf="@+id/textView2"></QuickContactBadge>
<Button
android:id="@+id/button_pickContact"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:text="Pick Contact"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView_email"></Button>
<FrameLayout
android:id="@+id/frameLayout_holder"
android:layout_width="0dp"
android:layout_height="80dp"
android:layout_marginStart="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.0"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button_pickContact"></FrameLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package com.example.quickcontactbadgeexample;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.net.Uri;
import android.os.Bundle;
import android.provider.ContactsContract;
import android.view.View;
import android.widget.Button;
import android.widget.FrameLayout;
import android.widget.QuickContactBadge;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity {
public static final int CONTACT_PICKER_RESULT = 100;
private TextView textViewEmail;
private TextView textViewPhone;
private Button buttonPickContact;
private FrameLayout frameLayoutHolder;
private QuickContactBadge quickContactBadgeEmail;
private QuickContactBadge quickContactBadgePhone;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.textViewEmail = (TextView) this.findViewById(R.id.textView_email);
this.textViewPhone = (TextView) this.findViewById(R.id.textView_phone);
this.buttonPickContact = (Button) this.findViewById(R.id.button_pickContact);
this.frameLayoutHolder = (FrameLayout) this.findViewById(R.id.frameLayout_holder);
this.quickContactBadgeEmail = (QuickContactBadge) findViewById(R.id.quickContactBadge_email);
this.quickContactBadgePhone = (QuickContactBadge) findViewById(R.id.quickContactBadge_phone);
int textViewHeight = this.textViewEmail.getLayoutParams().height;
quickContactBadgeEmail.setMaxHeight(textViewHeight);
String email = this.textViewEmail.getText().toString(); // test@gmail.com
String phoneNumber = this.textViewPhone.getText().toString(); // +1-555-521-5554
// Set Parameters for quickContactBadgeEmail:
this.quickContactBadgeEmail.assignContactFromEmail(email, true);
this.quickContactBadgeEmail.setMode(ContactsContract.QuickContact.MODE_SMALL);
// Set Parameters for quickContactBadgePhone:
this.quickContactBadgePhone.assignContactFromPhone(phoneNumber, true);
this.buttonPickContact.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
pickContactHandler( );
}
});
}
public void pickContactHandler( ) {
Intent contactPickerIntent = new Intent(Intent.ACTION_PICK,
ContactsContract.Contacts.CONTENT_URI);
startActivityForResult(contactPickerIntent, CONTACT_PICKER_RESULT);
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (resultCode == RESULT_OK) {
switch (requestCode) {
case CONTACT_PICKER_RESULT:
Uri contactUri = data.getData();
QuickContactBadge badgeLarge = new QuickContactBadge(this);
badgeLarge.assignContactUri(contactUri);
badgeLarge.setMode(ContactsContract.QuickContact.MODE_LARGE);
badgeLarge.setImageResource(R.drawable.icon_contact1);
this.frameLayoutHolder.addView(badgeLarge);
break;
}
}
}
}
Anleitungen Android
- Konfigurieren Sie Android Emulator in Android Studio
- Die Anleitung zu Android ToggleButton
- Erstellen Sie einen einfachen File Finder Dialog in Android
- Die Anleitung zu Android TimePickerDialog
- Die Anleitung zu Android DatePickerDialog
- Was ist erforderlich, um mit Android zu beginnen?
- Installieren Sie Android Studio unter Windows
- Installieren Sie Intel® HAXM für Android Studio
- Die Anleitung zu Android AsyncTask
- Die Anleitung zu Android AsyncTaskLoader
- Die Anleitung zum Android für den Anfänger - Grundlegende Beispiele
- Woher weiß man die Telefonnummer von Android Emulator und ändere es
- Die Anleitung zu Android TextInputLayout
- Die Anleitung zu Android CardView
- Die Anleitung zu Android ViewPager2
- Holen Sie sich die Telefonnummer in Android mit TelephonyManager
- Die Anleitung zu Android Phone Calls
- Die Anleitung zu Android Wifi Scanning
- Die Anleitung zum Android 2D Game für den Anfänger
- Die Anleitung zu Android DialogFragment
- Die Anleitung zu Android CharacterPickerDialog
- Die Anleitung zum Android für den Anfänger - Hello Android
- Verwenden des Android Device File Explorer
- Aktivieren Sie USB Debugging auf einem Android-Gerät
- Die Anleitung zu Android UI Layouts
- Die Anleitung zu Android SMS
- Die Anleitung zu Android SQLite Database
- Die Anleitung zu Google Maps Android API
- Text zu Sprache in Android
- Die Anleitung zu Android Space
- Die Anleitung zu Android Toast
- Erstellen Sie einen benutzerdefinierten Android Toast
- Die Anleitung zu Android SnackBar
- Die Anleitung zu Android TextView
- Die Anleitung zu Android TextClock
- Die Anleitung zu Android EditText
- Die Anleitung zu Android TextWatcher
- Formatieren Sie die Kreditkartennummer mit Android TextWatcher
- Die Anleitung zu Android Clipboard
- Erstellen Sie einen einfachen File Chooser in Android
- Die Anleitung zu Android AutoCompleteTextView und MultiAutoCompleteTextView
- Die Anleitung zu Android ImageView
- Die Anleitung zu Android ImageSwitcher
- Die Anleitung zu Android ScrollView und HorizontalScrollView
- Die Anleitung zu Android WebView
- Die Anleitung zu Android SeekBar
- Die Anleitung zu Android Dialog
- Die Anleitung zu Android AlertDialog
- Die Anleitung zu Android RatingBar
- Die Anleitung zu Android ProgressBar
- Die Anleitung zu Android Spinner
- Die Anleitung zu Android Button
- Die Anleitung zu Android Switch
- Die Anleitung zu Android ImageButton
- Die Anleitung zu Android FloatingActionButton
- Die Anleitung zu Android CheckBox
- Die Anleitung zu Android RadioGroup und RadioButton
- Die Anleitung zu Android Chip und ChipGroup
- Verwenden Sie Image Asset und Icon Asset von Android Studio
- Richten Sie die SDCard für den Emulator ein
- ChipGroup und Chip Entry Beispiel
- Hinzufügen externer Bibliotheken zu Android Project in Android Studio
- Wie deaktiviere ich die Berechtigungen, die der Android-Anwendung bereits erteilt wurden?
- Wie entferne ich Anwendungen aus dem Android Emulator?
- Die Anleitung zu Android LinearLayout
- Die Anleitung zu Android TableLayout
- Die Anleitung zu Android FrameLayout
- Die Anleitung zu Android QuickContactBadge
- Die Anleitung zu Android StackView
- Die Anleitung zu Android Camera
- Die Anleitung zu Android MediaPlayer
- Die Anleitung zu Android VideoView
- Spielen Sie Sound-Effekte in Android mit SoundPool
- Die Anleitung zu Android Networking
- Die Anleitung zu Android JSON Parser
- Die Anleitung zu Android SharedPreferences
- Die Anleitung zu Android Internal Storage
- Die Anleitung zu Android External Storage
- Die Anleitung zu Android Intents
- Beispiel für eine explizite Android Intent, nennen Sie eine andere Intent
- Beispiel für implizite Android Intent, Öffnen Sie eine URL, senden Sie eine Email
- Die Anleitung zu Android Services
- Die Anleitung zu Android Notifications
- Die Anleitung zu Android DatePicker
- Die Anleitung zu Android TimePicker
- Die Anleitung zu Android Chronometer
- Die Anleitung zu Android OptionMenu
- Die Anleitung zu Android ContextMenu
- Die Anleitung zu Android PopupMenu
- Die Anleitung zu Android Fragment
- Die Anleitung zu Android ListView
- Android ListView mit Checkbox verwenden ArrayAdapter
- Die Anleitung zu Android GridView
Show More