Die Anleitung zu Android CardView
1. Android CardView
Android 5.0 (API Level 21) führte eine neue Komponente namens CardView ein, bei der es sich im Grunde um einen rechteckigen Container mit vier abgerundeten Ecken und Shawdow-Effekten an den Rändern handelt. CardView wird üblicherweise als Originalcontainer der Items in ListView, GridView oder RecyclerView.
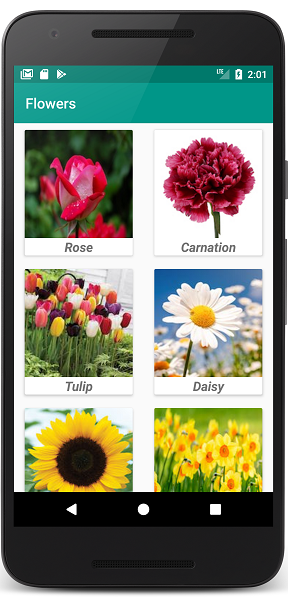
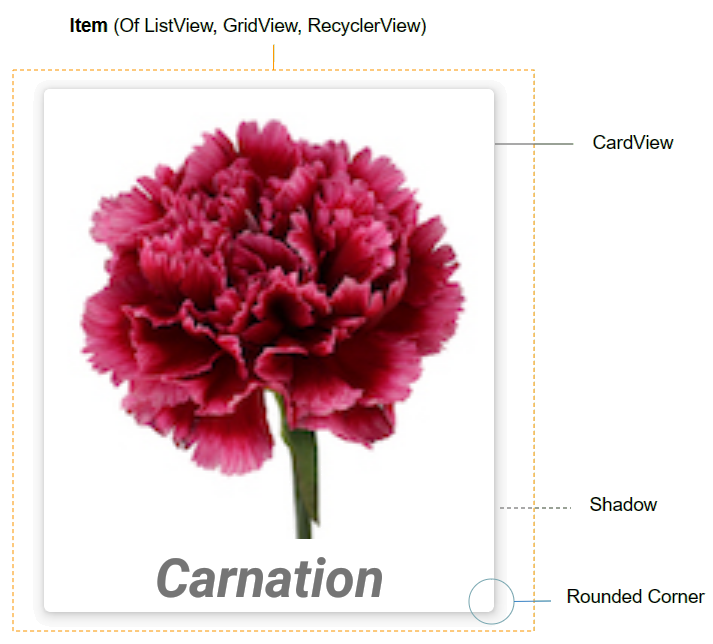
Standardmäßig ist CardView im Android SDK nicht verfügbar. Wenn Sie es verwenden möchten, müssen Sie Ihrem Projekt Unterstützungsbibliotheken hinzufügen.
Auf Android Studio können Sie die Bibilothek CardView aus Palette herunterladen.
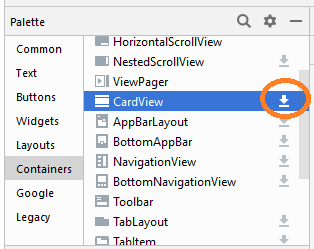
Nach der Installation wird die Bibliothek in build.gradle (Module: app) deklariert.
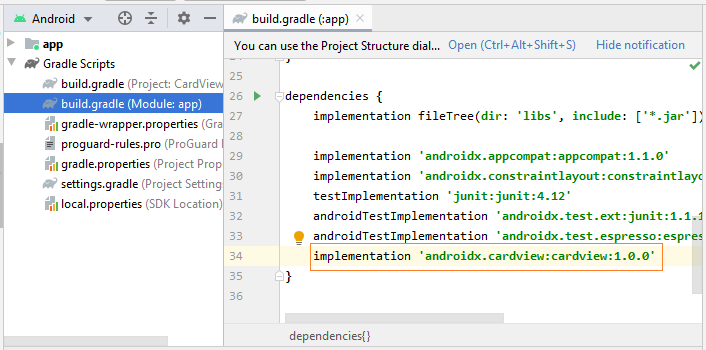
implementation 'androidx.cardview:cardview:1.0.0'
Hier sind einige wichtige Attribute von CardView, die Sie benötigen:
- android:layout_marginLeft
- android:layout_marginTop
- android:layout_marginRight
- android:layout_marginBottom
- app:cardBackgroundColor
2. Ein Beispiel von CardView
Lassen Sie uns ein Beispiel für RecyclerView und CardView setzen. Okay, hier ist das Vorschaubild dieses Beispiels:
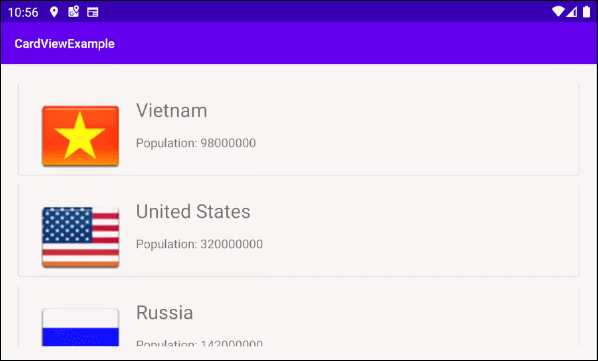
Auf Android Studio erstellen Sie ein Projekt:
- File > New > New Project > Empty Activity
- Name: CardViewExample
- Package name: org.o7planning.cardviewexample
Installieren Sie die Bibliothek RecyclerView und CardView in Ihr Projekt:
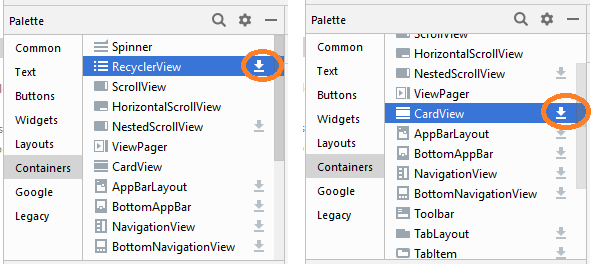
Nach der Installation werden Sie die deklarierten Bibliothek in build.gradle (Module: app):
implementation 'androidx.cardview:cardview:1.0.0'
implementation 'androidx.recyclerview:recyclerview:1.0.0'
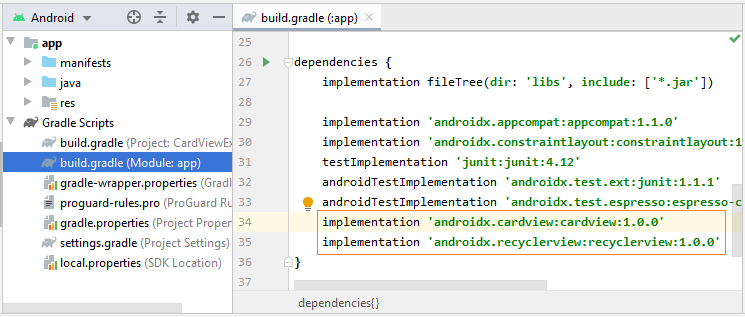
Sie brauchen einige Bilddatei, dann kopieren Sie in den Verzeichnis drawable vom Projekt:
vn.png | us.png | ru.png | jp.png | au.png |
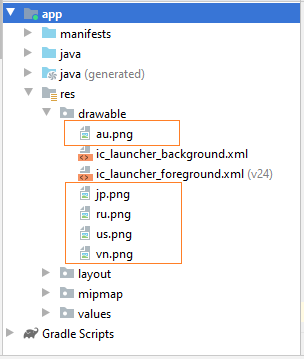
Zunäscht brauchen Sie Layout vom RecyclerView Item erstellen. Klicken Sie mit der recht-Maustasten auf den Verzeichnis "res/layout" und wählen Sie:
- New > Layout resource file
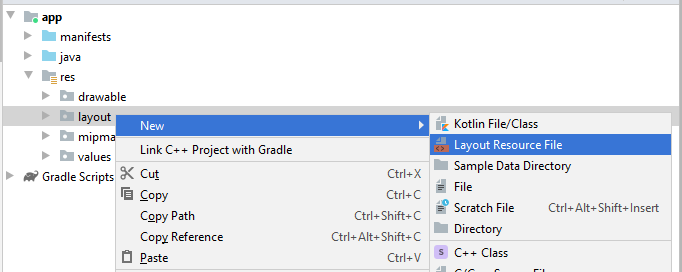
Geben Sie ein:
- File name: recyclerview_item_layout.xml
- Root element: androidx.cardview.widget.CardView
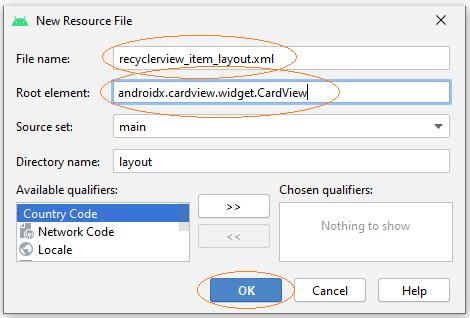
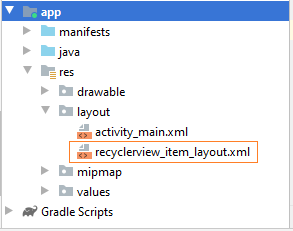
Dann entwerfen Sie die Interface von RecyclerView Item:
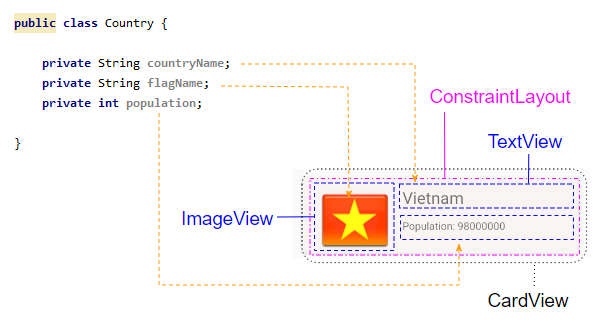
Öffnen Sie anschließend die Datei recyclerview_item_layout.xml und legen Sie einige wichtige CardView Attribute fest:
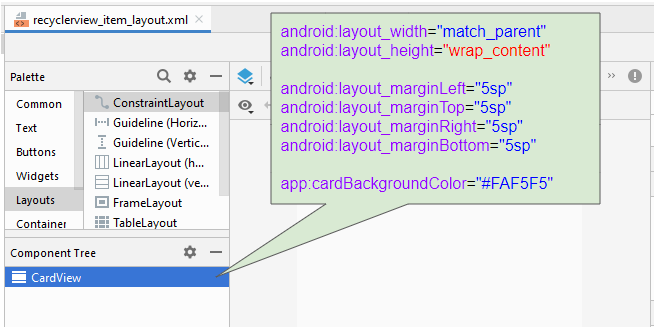
* recyclerview_item_layout.xml *
<?xml version="1.0" encoding="utf-8"?>
<androidx.cardview.widget.CardView
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="5sp"
android:layout_marginTop="5sp"
android:layout_marginRight="5sp"
android:layout_marginBottom="5sp"
app:cardBackgroundColor="#FAF5F5">
</androidx.cardview.widget.CardView>
Entwerfen Sie weiterhin die RecyclerView Item Interface:
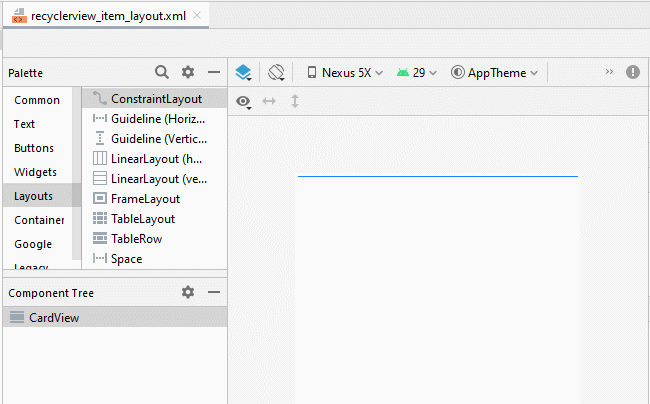
Passen Sie später die Positionen der Komponenten in der Interface an:
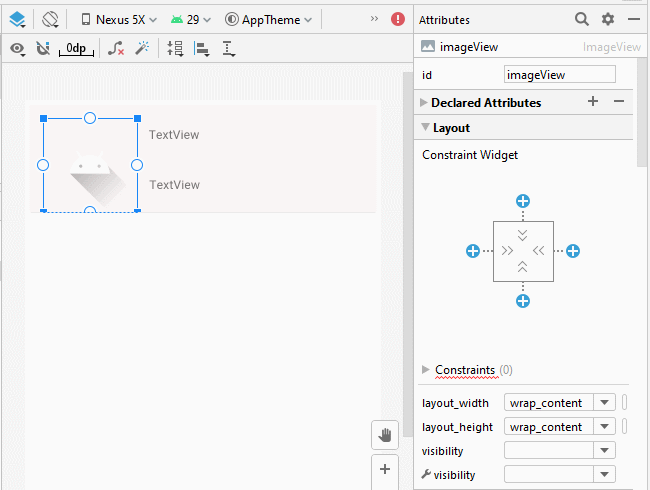
Legen Sie als Nächstes ID, Text, textSize für die Komponenten in der Interface fest:
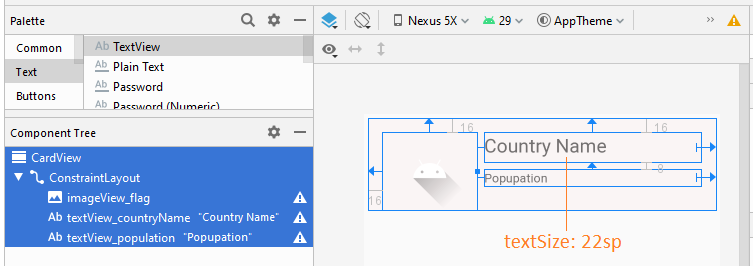
recyclerview_item_layout.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.cardview.widget.CardView
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="5sp"
android:layout_marginTop="5sp"
android:layout_marginRight="5sp"
android:layout_marginBottom="5sp"
app:cardBackgroundColor="#FAF5F5">
<androidx.constraintlayout.widget.ConstraintLayout
android:layout_width="match_parent"
android:layout_height="match_parent">
<ImageView
android:id="@+id/imageView_flag"
android:layout_width="110sp"
android:layout_height="90sp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:srcCompat="@drawable/ic_launcher_foreground"
tools:ignore="VectorDrawableCompat" />
<TextView
android:id="@+id/textView_countryName"
android:layout_width="0dp"
android:layout_height="35dp"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Country Name"
android:textSize="22sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toEndOf="@+id/imageView_flag"
app:layout_constraintTop_toTopOf="parent" />
<TextView
android:id="@+id/textView_population"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Popupation"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toEndOf="@+id/imageView_flag"
app:layout_constraintTop_toBottomOf="@+id/textView_countryName" />
</androidx.constraintlayout.widget.ConstraintLayout>
</androidx.cardview.widget.CardView>
Entwerfen Sie die Interface für activity_main.xml:
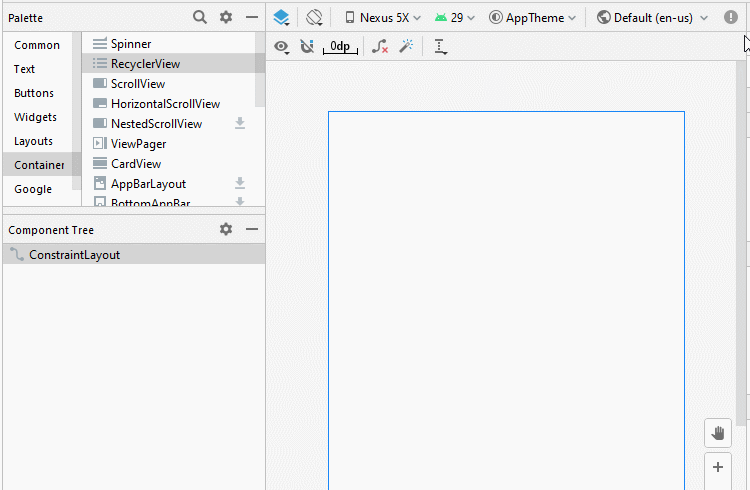
Country.java
package org.o7planning.cardviewexample;
public class Country {
private String countryName;
// Image name (Without extension)
private String flagName;
private int population;
public Country(String countryName, String flagName, int population) {
this.countryName= countryName;
this.flagName= flagName;
this.population= population;
}
public int getPopulation() {
return population;
}
public void setPopulation(int population) {
this.population = population;
}
public String getCountryName() {
return countryName;
}
public void setCountryName(String countryName) {
this.countryName = countryName;
}
public String getFlagName() {
return flagName;
}
public void setFlagName(String flagName) {
this.flagName = flagName;
}
@Override
public String toString() {
return this.countryName+" (Population: "+ this.population+")";
}
}
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/recyclerView"
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:layout_marginBottom="16dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
CustomRecyclerViewAdapter ist eine Klasse, die sich von RecyclerView.Adapter erstreckt. Sie ist für die Anzeige von Daten im RecyclerView Element verantwortlich.
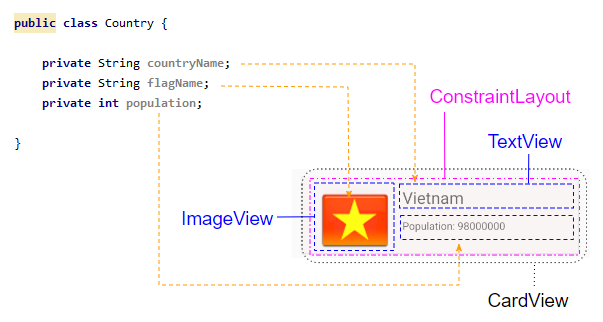
CustomRecyclerViewAdapter.java
package org.o7planning.cardviewexample;
import android.content.Context;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.Toast;
import androidx.recyclerview.widget.RecyclerView;
import java.util.List;
public class CustomRecyclerViewAdapter extends RecyclerView.Adapter<CountryViewHolder> {
private List<Country> countries;
private Context context;
private LayoutInflater mLayoutInflater;
public CustomRecyclerViewAdapter(Context context, List<Country> datas ) {
this.context = context;
this.countries = datas;
this.mLayoutInflater = LayoutInflater.from(context);
}
@Override
public CountryViewHolder onCreateViewHolder(final ViewGroup parent, int viewType) {
// Inflate view from recyclerview_item_layout.xml
View recyclerViewItem = mLayoutInflater.inflate(R.layout.recyclerview_item_layout, parent, false);
recyclerViewItem.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
handleRecyclerItemClick( (RecyclerView)parent, v);
}
});
return new CountryViewHolder(recyclerViewItem);
}
@Override
public void onBindViewHolder(CountryViewHolder holder, int position) {
// Cet country in countries via position
Country country = this.countries.get(position);
int imageResId = this.getDrawableResIdByName(country.getFlagName());
// Bind data to viewholder
holder.flagView.setImageResource(imageResId);
holder.countryNameView.setText(country.getCountryName() );
holder.populationView.setText("Population: "+ country.getPopulation());
}
@Override
public int getItemCount() {
return this.countries.size();
}
// Find Image ID corresponding to the name of the image (in the directory drawable).
public int getDrawableResIdByName(String resName) {
String pkgName = context.getPackageName();
// Return 0 if not found.
int resID = context.getResources().getIdentifier(resName , "drawable", pkgName);
Log.i(MainActivity.LOG_TAG, "Res Name: "+ resName+"==> Res ID = "+ resID);
return resID;
}
// Click on RecyclerView Item.
private void handleRecyclerItemClick(RecyclerView recyclerView, View itemView) {
int itemPosition = recyclerView.getChildLayoutPosition(itemView);
Country country = this.countries.get(itemPosition);
Toast.makeText(this.context, country.getCountryName(), Toast.LENGTH_LONG).show();
}
}
CountryViewHolder.java
package org.o7planning.cardviewexample;
import android.view.View;
import android.widget.ImageView;
import android.widget.TextView;
import androidx.annotation.NonNull;
import androidx.recyclerview.widget.RecyclerView;
public class CountryViewHolder extends RecyclerView.ViewHolder {
ImageView flagView;
TextView countryNameView;
TextView populationView;
// @itemView: recyclerview_item_layout.xml
public CountryViewHolder(@NonNull View itemView) {
super(itemView);
this.flagView = (ImageView) itemView.findViewById(R.id.imageView_flag);
this.countryNameView = (TextView) itemView.findViewById(R.id.textView_countryName);
this.populationView = (TextView) itemView.findViewById(R.id.textView_population);
}
}
MainActivity.java
package org.o7planning.cardviewexample;
import android.os.Bundle;
import androidx.appcompat.app.AppCompatActivity;
import androidx.recyclerview.widget.LinearLayoutManager;
import androidx.recyclerview.widget.RecyclerView;
import java.util.ArrayList;
import java.util.List;
public class MainActivity extends AppCompatActivity {
public static final String LOG_TAG = "AndroidExample";
private RecyclerView recyclerView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
List<Country> countries = getListData();
this.recyclerView = (RecyclerView) this.findViewById(R.id.recyclerView);
recyclerView.setAdapter(new CustomRecyclerViewAdapter(this, countries));
// RecyclerView scroll vertical
LinearLayoutManager linearLayoutManager = new LinearLayoutManager(this, LinearLayoutManager.VERTICAL, false);
recyclerView.setLayoutManager(linearLayoutManager);
}
private List<Country> getListData() {
List<Country> list = new ArrayList<Country>();
Country vietnam = new Country("Vietnam", "vn", 98000000);
Country usa = new Country("United States", "us", 320000000);
Country russia = new Country("Russia", "ru", 142000000);
Country autraylia = new Country("Autraylia", "au", 25000000);
Country japan = new Country("Japan", "jp", 126000000);
list.add(vietnam);
list.add(usa);
list.add(russia);
list.add(autraylia);
list.add(japan);
return list;
}
}
Anleitungen Android
- Konfigurieren Sie Android Emulator in Android Studio
- Die Anleitung zu Android ToggleButton
- Erstellen Sie einen einfachen File Finder Dialog in Android
- Die Anleitung zu Android TimePickerDialog
- Die Anleitung zu Android DatePickerDialog
- Was ist erforderlich, um mit Android zu beginnen?
- Installieren Sie Android Studio unter Windows
- Installieren Sie Intel® HAXM für Android Studio
- Die Anleitung zu Android AsyncTask
- Die Anleitung zu Android AsyncTaskLoader
- Die Anleitung zum Android für den Anfänger - Grundlegende Beispiele
- Woher weiß man die Telefonnummer von Android Emulator und ändere es
- Die Anleitung zu Android TextInputLayout
- Die Anleitung zu Android CardView
- Die Anleitung zu Android ViewPager2
- Holen Sie sich die Telefonnummer in Android mit TelephonyManager
- Die Anleitung zu Android Phone Calls
- Die Anleitung zu Android Wifi Scanning
- Die Anleitung zum Android 2D Game für den Anfänger
- Die Anleitung zu Android DialogFragment
- Die Anleitung zu Android CharacterPickerDialog
- Die Anleitung zum Android für den Anfänger - Hello Android
- Verwenden des Android Device File Explorer
- Aktivieren Sie USB Debugging auf einem Android-Gerät
- Die Anleitung zu Android UI Layouts
- Die Anleitung zu Android SMS
- Die Anleitung zu Android SQLite Database
- Die Anleitung zu Google Maps Android API
- Text zu Sprache in Android
- Die Anleitung zu Android Space
- Die Anleitung zu Android Toast
- Erstellen Sie einen benutzerdefinierten Android Toast
- Die Anleitung zu Android SnackBar
- Die Anleitung zu Android TextView
- Die Anleitung zu Android TextClock
- Die Anleitung zu Android EditText
- Die Anleitung zu Android TextWatcher
- Formatieren Sie die Kreditkartennummer mit Android TextWatcher
- Die Anleitung zu Android Clipboard
- Erstellen Sie einen einfachen File Chooser in Android
- Die Anleitung zu Android AutoCompleteTextView und MultiAutoCompleteTextView
- Die Anleitung zu Android ImageView
- Die Anleitung zu Android ImageSwitcher
- Die Anleitung zu Android ScrollView und HorizontalScrollView
- Die Anleitung zu Android WebView
- Die Anleitung zu Android SeekBar
- Die Anleitung zu Android Dialog
- Die Anleitung zu Android AlertDialog
- Die Anleitung zu Android RatingBar
- Die Anleitung zu Android ProgressBar
- Die Anleitung zu Android Spinner
- Die Anleitung zu Android Button
- Die Anleitung zu Android Switch
- Die Anleitung zu Android ImageButton
- Die Anleitung zu Android FloatingActionButton
- Die Anleitung zu Android CheckBox
- Die Anleitung zu Android RadioGroup und RadioButton
- Die Anleitung zu Android Chip und ChipGroup
- Verwenden Sie Image Asset und Icon Asset von Android Studio
- Richten Sie die SDCard für den Emulator ein
- ChipGroup und Chip Entry Beispiel
- Hinzufügen externer Bibliotheken zu Android Project in Android Studio
- Wie deaktiviere ich die Berechtigungen, die der Android-Anwendung bereits erteilt wurden?
- Wie entferne ich Anwendungen aus dem Android Emulator?
- Die Anleitung zu Android LinearLayout
- Die Anleitung zu Android TableLayout
- Die Anleitung zu Android FrameLayout
- Die Anleitung zu Android QuickContactBadge
- Die Anleitung zu Android StackView
- Die Anleitung zu Android Camera
- Die Anleitung zu Android MediaPlayer
- Die Anleitung zu Android VideoView
- Spielen Sie Sound-Effekte in Android mit SoundPool
- Die Anleitung zu Android Networking
- Die Anleitung zu Android JSON Parser
- Die Anleitung zu Android SharedPreferences
- Die Anleitung zu Android Internal Storage
- Die Anleitung zu Android External Storage
- Die Anleitung zu Android Intents
- Beispiel für eine explizite Android Intent, nennen Sie eine andere Intent
- Beispiel für implizite Android Intent, Öffnen Sie eine URL, senden Sie eine Email
- Die Anleitung zu Android Services
- Die Anleitung zu Android Notifications
- Die Anleitung zu Android DatePicker
- Die Anleitung zu Android TimePicker
- Die Anleitung zu Android Chronometer
- Die Anleitung zu Android OptionMenu
- Die Anleitung zu Android ContextMenu
- Die Anleitung zu Android PopupMenu
- Die Anleitung zu Android Fragment
- Die Anleitung zu Android ListView
- Android ListView mit Checkbox verwenden ArrayAdapter
- Die Anleitung zu Android GridView
Show More