ChipGroup und Chip Entry Beispiel
1. Ziele von Beispiel
In dieser Lektion zeige ich Ihnen, wie Sie eine Android-Anwendung mit ChipGroup und Chip Entry erstellen. Dies ist nur ein einfaches Beispiel, so dass es nicht viele detaillierte Erklärungen über ChipGroup und Chip geben wird. Aber wenn Sie eine vollständige Erklärung erhalten möchten, schauen Sie sich bitte den Artikel unten an:
Die Funktion eines Chip Entry ist, dass es checkbar ist und es enthält geschlossene Icons. Aus diesem Grund ist es für einige Anwendungen ähnlich den Beispielen in diesem Artikel geeignet.
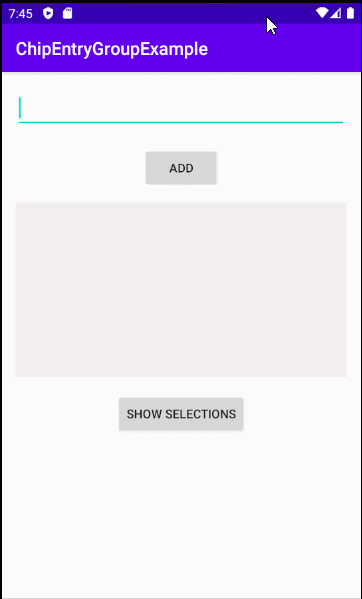
2. Beispiel von ChipGroup und Chip Entry
In Android Studio erstellen Sie ein neues Projekt:
- File > New > New Project > Empty Activity
- Name: ChipEntryGroupExample
- Package name: org.o7planning.chipentrygroupexample
- Language: Java
Zuerst sollen Sie die Bibliothek Chip in Ihrem Projekt installieren
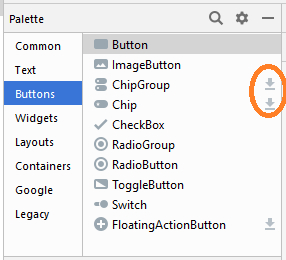
Oder Sie können die Bibliothek mit dem Komponent Chip in Ihrem Projekt manuell einfügen:
build.gradle (Module app)
dependencies {
...
implementation 'com.google.android.material:material:1.1.0'
}
Klicken Sie als Nächstes mit der rechten Maustaste auf den Ordner "Layout" und wählen Sie:
- New > Layout Resource File
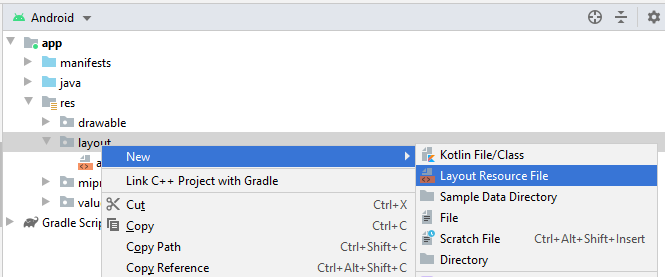
- File name: layout_chip_entry.xml
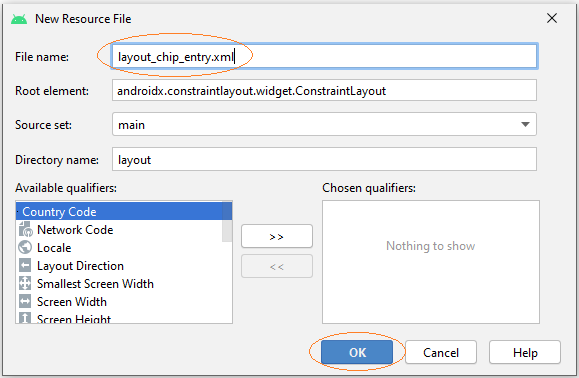
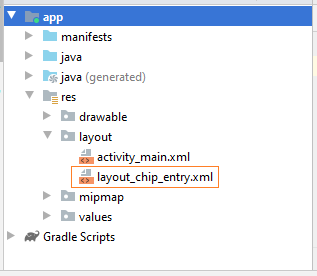
"Layout Resource File" allows you to define a part of the user interface, in this example it is very simple, including only a Chip Entry element.
layout_chip_entry.xml
<?xml version="1.0" encoding="utf-8"?>
<com.google.android.material.chip.Chip
xmlns:android="http://schemas.android.com/apk/res/android"
style="@style/Widget.MaterialComponents.Chip.Entry"
android:theme="@style/Theme.MaterialComponents.Light"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
/>
Zum letzten sieht die Interface der Anwendung aus:
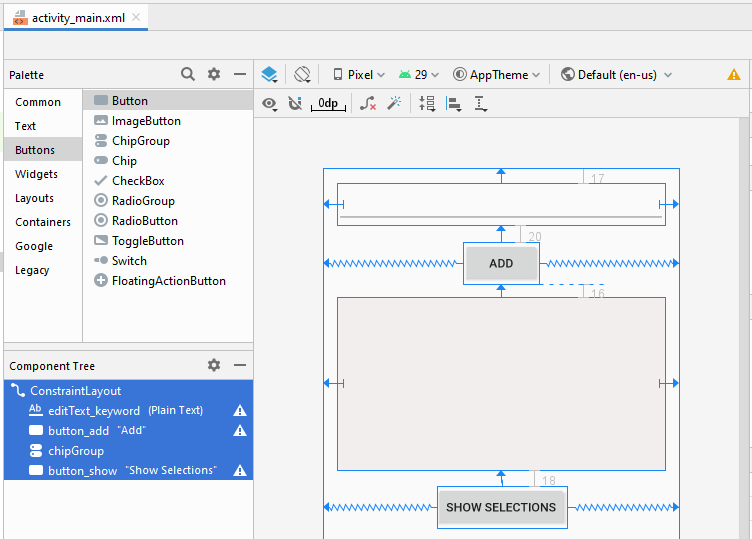
Wenn Sie an einigen Schritten zum Entwerfen der Interface dieser Anwendung interessiert sind, scrollen Sie bis zum Ende des Artikels nach unten, und sehen Sie mehr im Anhang.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<EditText
android:id="@+id/editText_keyword"
android:layout_width="0dp"
android:layout_height="48dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="17dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:ems="10"
android:inputType="textPersonName"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button_add"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
android:text="Add"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editText_keyword" />
<com.google.android.material.chip.ChipGroup
android:id="@+id/chipGroup"
android:layout_width="0dp"
android:layout_height="200dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:background="#F3EEEE"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button_add" />
<Button
android:id="@+id/button_show"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="18dp"
android:text="Show Selections"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chipGroup" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package org.o7planning.chipentrygroupexample;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.widget.Button;
import android.widget.CompoundButton;
import android.widget.EditText;
import android.widget.Toast;
import com.google.android.material.chip.Chip;
import com.google.android.material.chip.ChipGroup;
public class MainActivity extends AppCompatActivity {
private EditText editTextKeyword;
private ChipGroup chipGroup;
private Button buttonAdd;
private Button buttonShow;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.editTextKeyword = (EditText) this.findViewById(R.id.editText_keyword);
this.chipGroup = (ChipGroup) this.findViewById(R.id.chipGroup);
this.buttonAdd = (Button) this.findViewById(R.id.button_add);
this.buttonShow = (Button) this.findViewById(R.id.button_show);
this.buttonAdd.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
addNewChip();
}
});
this.buttonShow.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
showSelections();
}
});
}
private void addNewChip() {
String keyword = this.editTextKeyword.getText().toString();
if (keyword == null || keyword.isEmpty()) {
Toast.makeText(this, "Please enter the keyword!", Toast.LENGTH_LONG).show();
return;
}
try {
LayoutInflater inflater = LayoutInflater.from(this);
// Create a Chip from Layout.
Chip newChip = (Chip) inflater.inflate(R.layout.layout_chip_entry, this.chipGroup, false);
newChip.setText(keyword);
//
// Other methods:
//
// newChip.setCloseIconVisible(true);
// newChip.setCloseIconResource(R.drawable.your_icon);
// newChip.setChipIconResource(R.drawable.your_icon);
// newChip.setChipBackgroundColorResource(R.color.red);
// newChip.setTextAppearanceResource(R.style.ChipTextStyle);
// newChip.setElevation(15);
this.chipGroup.addView(newChip);
// Set Listener for the Chip:
newChip.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
handleChipCheckChanged((Chip) buttonView, isChecked);
}
});
newChip.setOnCloseIconClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
handleChipCloseIconClicked((Chip) v);
}
});
this.editTextKeyword.setText("");
} catch (Exception e) {
e.printStackTrace();
Toast.makeText(this, "Error: " + e.getMessage(), Toast.LENGTH_LONG).show();
}
}
// User click on "Show Selections" button.
private void showSelections() {
int count = this.chipGroup.getChildCount();
String s = null;
for(int i=0;i< count; i++) {
Chip child = (Chip) this.chipGroup.getChildAt(i);
if(!child.isChecked()) {
continue;
}
if(s == null) {
s = child.getText().toString();
} else {
s += ", " + child.getText().toString();
}
}
Toast.makeText(this, s, Toast.LENGTH_LONG).show();
}
// User close a Chip.
private void handleChipCloseIconClicked(Chip chip) {
ChipGroup parent = (ChipGroup) chip.getParent();
parent.removeView(chip);
}
// Chip Checked Changed
private void handleChipCheckChanged(Chip chip, boolean isChecked) {
}
}
Anleitungen Android
- Konfigurieren Sie Android Emulator in Android Studio
- Die Anleitung zu Android ToggleButton
- Erstellen Sie einen einfachen File Finder Dialog in Android
- Die Anleitung zu Android TimePickerDialog
- Die Anleitung zu Android DatePickerDialog
- Was ist erforderlich, um mit Android zu beginnen?
- Installieren Sie Android Studio unter Windows
- Installieren Sie Intel® HAXM für Android Studio
- Die Anleitung zu Android AsyncTask
- Die Anleitung zu Android AsyncTaskLoader
- Die Anleitung zum Android für den Anfänger - Grundlegende Beispiele
- Woher weiß man die Telefonnummer von Android Emulator und ändere es
- Die Anleitung zu Android TextInputLayout
- Die Anleitung zu Android CardView
- Die Anleitung zu Android ViewPager2
- Holen Sie sich die Telefonnummer in Android mit TelephonyManager
- Die Anleitung zu Android Phone Calls
- Die Anleitung zu Android Wifi Scanning
- Die Anleitung zum Android 2D Game für den Anfänger
- Die Anleitung zu Android DialogFragment
- Die Anleitung zu Android CharacterPickerDialog
- Die Anleitung zum Android für den Anfänger - Hello Android
- Verwenden des Android Device File Explorer
- Aktivieren Sie USB Debugging auf einem Android-Gerät
- Die Anleitung zu Android UI Layouts
- Die Anleitung zu Android SMS
- Die Anleitung zu Android SQLite Database
- Die Anleitung zu Google Maps Android API
- Text zu Sprache in Android
- Die Anleitung zu Android Space
- Die Anleitung zu Android Toast
- Erstellen Sie einen benutzerdefinierten Android Toast
- Die Anleitung zu Android SnackBar
- Die Anleitung zu Android TextView
- Die Anleitung zu Android TextClock
- Die Anleitung zu Android EditText
- Die Anleitung zu Android TextWatcher
- Formatieren Sie die Kreditkartennummer mit Android TextWatcher
- Die Anleitung zu Android Clipboard
- Erstellen Sie einen einfachen File Chooser in Android
- Die Anleitung zu Android AutoCompleteTextView und MultiAutoCompleteTextView
- Die Anleitung zu Android ImageView
- Die Anleitung zu Android ImageSwitcher
- Die Anleitung zu Android ScrollView und HorizontalScrollView
- Die Anleitung zu Android WebView
- Die Anleitung zu Android SeekBar
- Die Anleitung zu Android Dialog
- Die Anleitung zu Android AlertDialog
- Die Anleitung zu Android RatingBar
- Die Anleitung zu Android ProgressBar
- Die Anleitung zu Android Spinner
- Die Anleitung zu Android Button
- Die Anleitung zu Android Switch
- Die Anleitung zu Android ImageButton
- Die Anleitung zu Android FloatingActionButton
- Die Anleitung zu Android CheckBox
- Die Anleitung zu Android RadioGroup und RadioButton
- Die Anleitung zu Android Chip und ChipGroup
- Verwenden Sie Image Asset und Icon Asset von Android Studio
- Richten Sie die SDCard für den Emulator ein
- ChipGroup und Chip Entry Beispiel
- Hinzufügen externer Bibliotheken zu Android Project in Android Studio
- Wie deaktiviere ich die Berechtigungen, die der Android-Anwendung bereits erteilt wurden?
- Wie entferne ich Anwendungen aus dem Android Emulator?
- Die Anleitung zu Android LinearLayout
- Die Anleitung zu Android TableLayout
- Die Anleitung zu Android FrameLayout
- Die Anleitung zu Android QuickContactBadge
- Die Anleitung zu Android StackView
- Die Anleitung zu Android Camera
- Die Anleitung zu Android MediaPlayer
- Die Anleitung zu Android VideoView
- Spielen Sie Sound-Effekte in Android mit SoundPool
- Die Anleitung zu Android Networking
- Die Anleitung zu Android JSON Parser
- Die Anleitung zu Android SharedPreferences
- Die Anleitung zu Android Internal Storage
- Die Anleitung zu Android External Storage
- Die Anleitung zu Android Intents
- Beispiel für eine explizite Android Intent, nennen Sie eine andere Intent
- Beispiel für implizite Android Intent, Öffnen Sie eine URL, senden Sie eine Email
- Die Anleitung zu Android Services
- Die Anleitung zu Android Notifications
- Die Anleitung zu Android DatePicker
- Die Anleitung zu Android TimePicker
- Die Anleitung zu Android Chronometer
- Die Anleitung zu Android OptionMenu
- Die Anleitung zu Android ContextMenu
- Die Anleitung zu Android PopupMenu
- Die Anleitung zu Android Fragment
- Die Anleitung zu Android ListView
- Android ListView mit Checkbox verwenden ArrayAdapter
- Die Anleitung zu Android GridView
Show More