Die Anleitung zu Android JSON Parser
1. Was ist JSON?
JSON (JavaScript Object Notation) ist eine Datenaustauschformat (data exchange format). Es speichert die Daten nach dem Paar Schlüssel und Wert. Im Vergleich von XML ist JSON einfacher und leichter zu lesen.
Sehen Sie ein einfaches Beispiel von JSON:
{
"name" : "Tran",
"address" : "Hai Duong, Vietnam",
"phones" : [0121111111, 012222222]
}
Die Paaren Schlüssel- Wert (key-value) können eingenistet werden:
{
"id": 111 ,
"name":"Microsoft",
"websites": [
"http://microsoft.com",
"http://msn.com",
"http://hotmail.com"
],
"address":{
"street":"1 Microsoft Way",
"city":"Redmond"
}
}
Im Java gibt es viele open Source Code Bibliothek, damit Sie mit dem Dokument JSON manipulieren können, zum Beispiel:
- JSONP
- json.org
- Jackson
- Google GSON
- json-lib
- javax json
- json-simple
- json-smart
- flexjson
- fastjson
Android unterstützt verfügbar die Bibiothek um mit JSON zu arbeiten. Sie brauchen die anderen Bibliotheke nicht deklarieren. In diesem Dokument werde ich Sie bei der Arbeit mit JSON mit der Verwendung von vorhandenen JSON API in dem Betriebsystem von Android anleiten.
2. JSON & Java Object
Die folgende Illustration bezeichnet die Beziehung zwischen JSON und der Java Klassen.
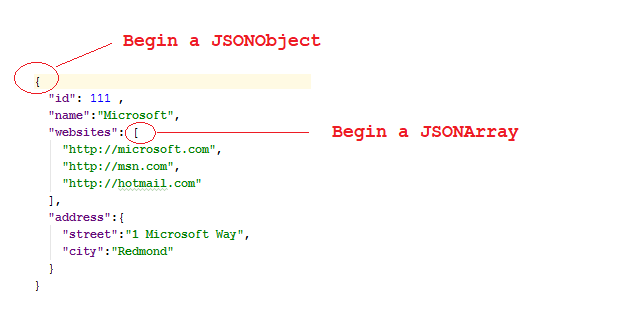
3. Schnell-erstellen Sie ein Projekt um mit JSON zu arbeiten
Ich erstelle ein Projekt mit dem Name vom JSONTutorial schnell um mit JSON durch die Beispiele zu arbeiten.
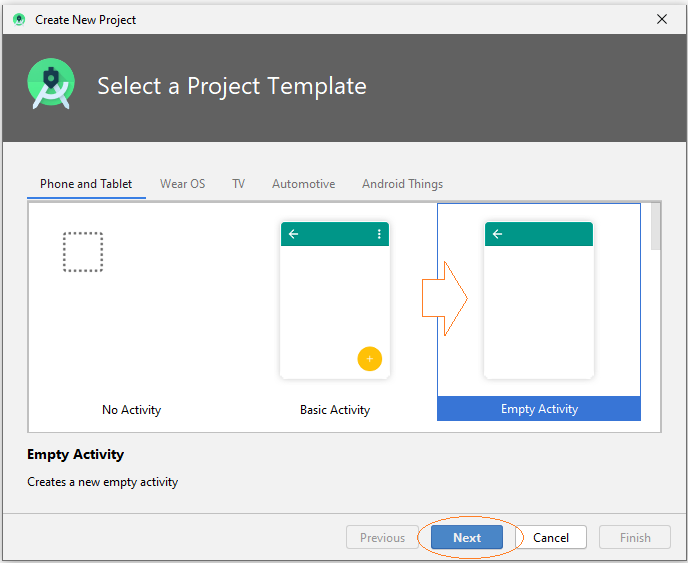
- Name: JSONTutorial
- Package name: org.o7planning.jsontutorial
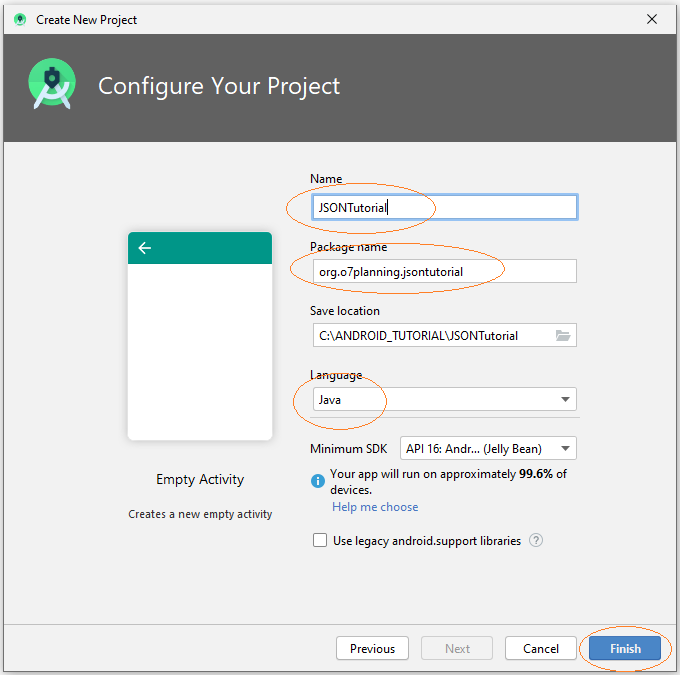
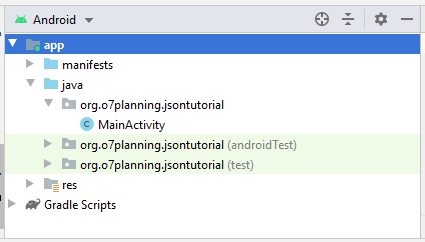
Erstellen Sie ein Verzeichnis raw:
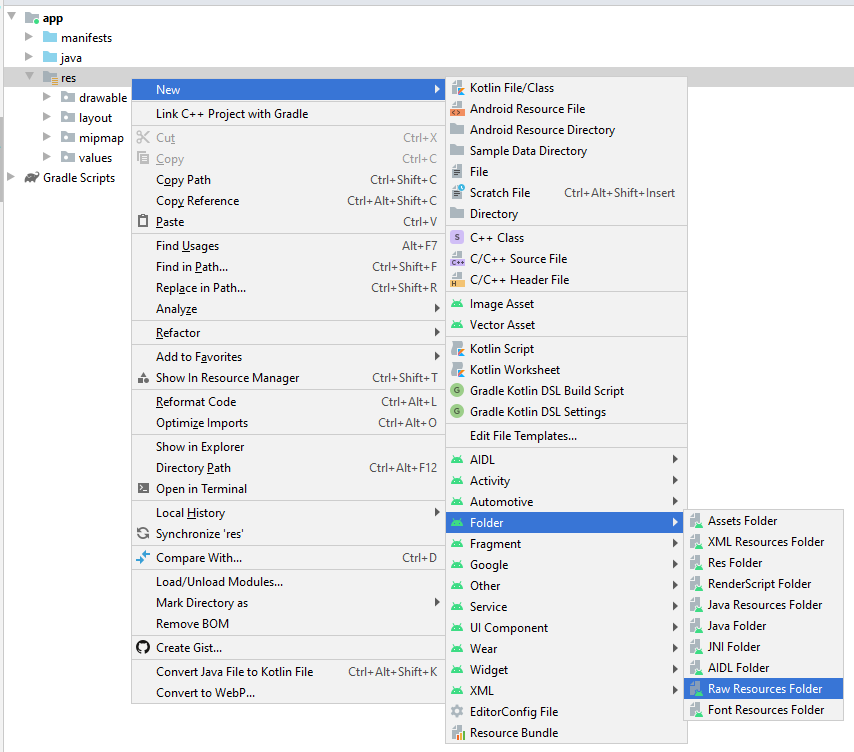
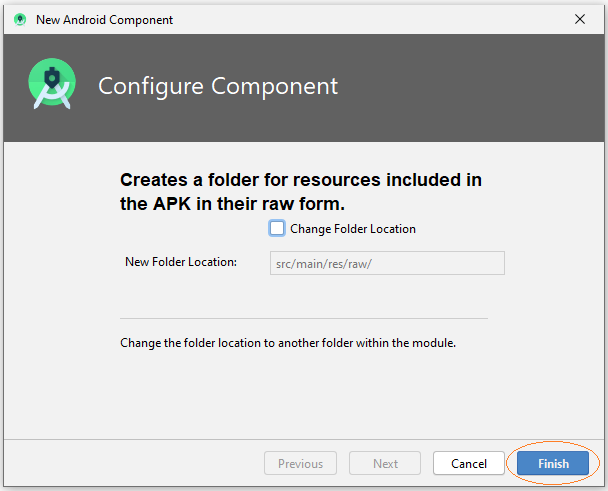
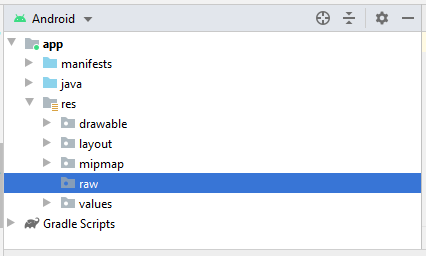
Ich erstelle ich einige File json im Verzeichnis 'raw'. Diese File werden an die Beispiele in dem Dokument teilnehmen.
Die Quelle der Versorgung von Daten JSON kann aus der File oder URL sein,... Am Ende des Dokument können Sie das Beispiel sehen,wie die Daten JSON aus URL abgerufen, in einer Applikation Android analysiert und angezeigt werden.
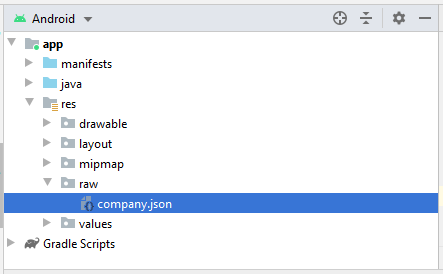
company.json
{
"id": 111 ,
"name":"Microsoft",
"websites": [
"http://microsoft.com",
"http://msn.com",
"http://hotmail.com"
],
"address":{
"street":"1 Microsoft Way",
"city":"Redmond"
}
}
Entwerfen Sie activity_main.xml:
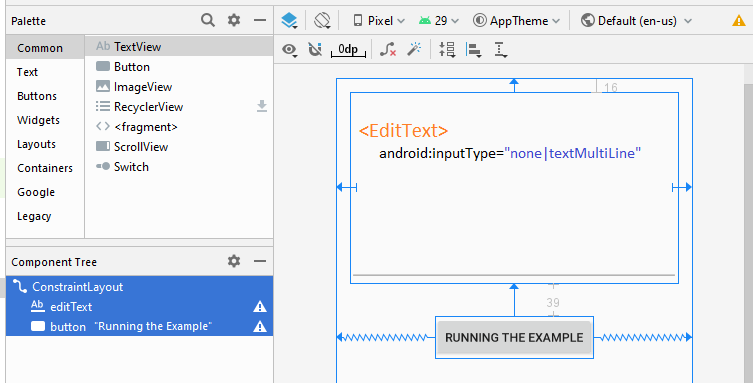
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<EditText
android:id="@+id/editText"
android:layout_width="0dp"
android:layout_height="220dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:ems="10"
android:inputType="none|textMultiLine"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="39dp"
android:text="Running the Example"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editText" />
</androidx.constraintlayout.widget.ConstraintLayout>
4. Java Beans classes
Einige Klassen nehmen an die Beispielen teil
Address.java
package org.o7planning.jsontutorial.beans;
public class Address {
private String street;
private String city;
public Address() {
}
public Address(String street, String city) {
this.street = street;
this.city = city;
}
public String getStreet() {
return street;
}
public void setStreet(String street) {
this.street = street;
}
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
@Override
public String toString() {
return street + ", " + city;
}
}
Company.java
package org.o7planning.jsontutorial.beans;
public class Company {
private int id;
private String name;
private String[] websites;
private Address address;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String[] getWebsites() {
return websites;
}
public void setWebsites(String[] websites) {
this.websites = websites;
}
public Address getAddress() {
return address;
}
public void setAddress(Address address) {
this.address = address;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("\n id:" + this.id);
sb.append("\n name:" + this.name);
if (this.websites != null) {
sb.append("\n website: ");
for (String website : this.websites) {
sb.append(website + ", ");
}
}
if (this.address != null) {
sb.append("\n address:" + this.address.toString());
}
return sb.toString();
}
}
5. Zum Beispiel: Die Daten JSON lesen, die in einem Java- Objekt übertragen wurden
In diesem Beispiel werden wir die Datenfile JSON lesen und es ins Java-Objekt übertragen.
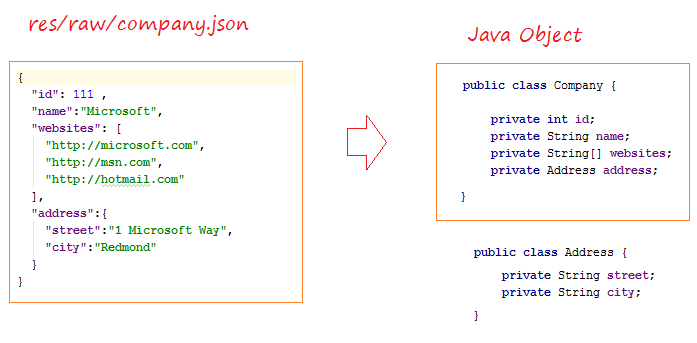
ReadJSONExample.java
package org.o7planning.jsontutorial.json;
import android.content.Context;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import org.o7planning.jsontutorial.R;
import org.o7planning.jsontutorial.beans.Address;
import org.o7planning.jsontutorial.beans.Company;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
public class ReadJSONExample {
// Read the company.json file and convert it to a java object.
public static Company readCompanyJSONFile(Context context) throws IOException,JSONException {
// Read content of company.json
String jsonText = readText(context, R.raw.company);
JSONObject jsonRoot = new JSONObject(jsonText);
int id= jsonRoot.getInt("id");
String name = jsonRoot.getString("name");
JSONArray jsonArray = jsonRoot.getJSONArray("websites");
String[] websites = new String[jsonArray.length()];
for(int i=0;i < jsonArray.length();i++) {
websites[i] = jsonArray.getString(i);
}
JSONObject jsonAddress = jsonRoot.getJSONObject("address");
String street = jsonAddress.getString("street");
String city = jsonAddress.getString("city");
Address address= new Address(street, city);
Company company = new Company();
company.setId(id);
company.setName(name);
company.setAddress(address);
company.setWebsites(websites);
return company;
}
private static String readText(Context context, int resId) throws IOException {
InputStream is = context.getResources().openRawResource(resId);
BufferedReader br= new BufferedReader(new InputStreamReader(is));
StringBuilder sb= new StringBuilder();
String s= null;
while(( s = br.readLine())!=null) {
sb.append(s);
sb.append("\n");
}
return sb.toString();
}
}
MainActivity.java
package org.o7planning.jsontutorial;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import org.o7planning.jsontutorial.beans.Company;
import org.o7planning.jsontutorial.json.ReadJSONExample;
public class MainActivity extends AppCompatActivity {
private EditText outputText;
private Button button;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.outputText = (EditText)this.findViewById(R.id.editText);
this.button = (Button) this.findViewById(R.id.button);
this.button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
runExample(view);
}
});
}
public void runExample(View view) {
try {
Company company = ReadJSONExample.readCompanyJSONFile(this);
outputText.setText(company.toString());
} catch(Exception e) {
outputText.setText(e.getMessage());
e.printStackTrace();
}
}
}
Das Beispiel laufen:
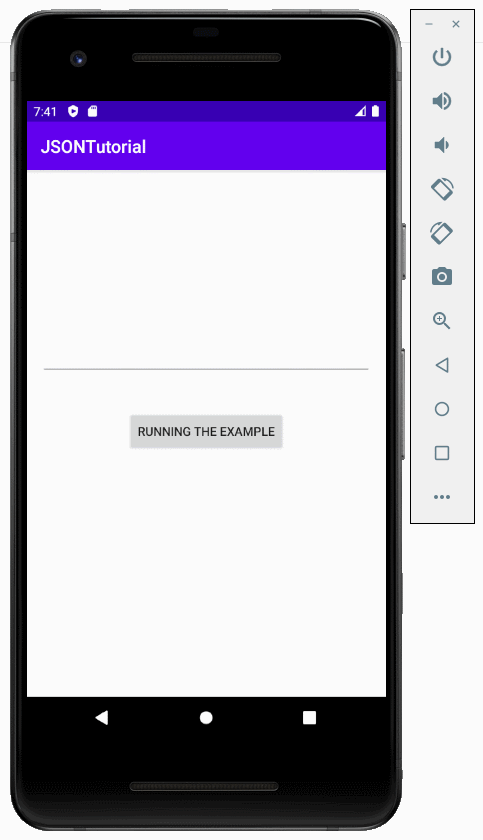
6. Zum Beispiel: Das Java-Objekt in JSON-Data konvertieren
Das folgende Beispiel konvertiert ein Java-Objekt in einem JSON-Daten.
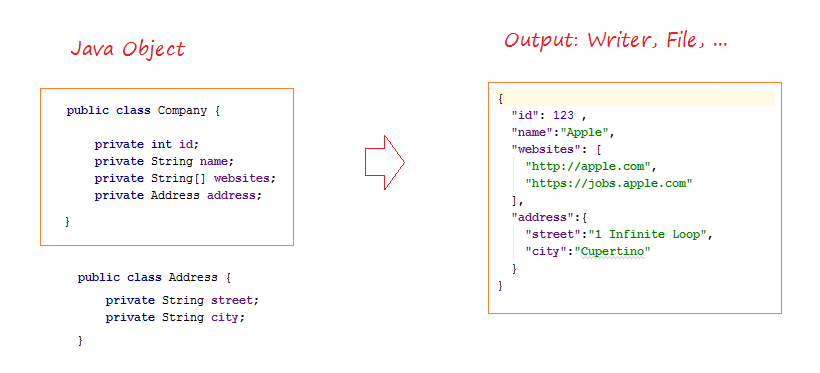
JsonWriterExample.java
package org.o7planning.jsontutorial.json;
import android.util.JsonWriter;
import org.o7planning.jsontutorial.beans.Address;
import org.o7planning.jsontutorial.beans.Company;
import java.io.IOException;
import java.io.Writer;
public class JsonWriterExample {
public static void writeJsonStream(Writer output, Company company ) throws IOException {
JsonWriter jsonWriter = new JsonWriter(output);
jsonWriter.beginObject();// begin root
jsonWriter.name("id").value(company.getId());
jsonWriter.name("name").value(company.getName());
String[] websites= company.getWebsites();
// "websites": [ ....]
jsonWriter.name("websites").beginArray(); // begin websites
for(String website: websites) {
jsonWriter.value(website);
}
jsonWriter.endArray();// end websites
// "address": { ... }
jsonWriter.name("address").beginObject(); // begin address
jsonWriter.name("street").value(company.getAddress().getStreet());
jsonWriter.name("city").value(company.getAddress().getCity());
jsonWriter.endObject();// end address
// end root
jsonWriter.endObject();
}
public static Company createCompany() {
Company company = new Company();
company.setId(123);
company.setName("Apple");
String[] websites = { "http://apple.com", "https://jobs.apple.com" };
company.setWebsites(websites);
Address address = new Address();
address.setCity("Cupertino");
address.setStreet("1 Infinite Loop");
company.setAddress(address);
return company;
}
}
MainActivity.java (2)
package org.o7planning.jsontutorial;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import org.o7planning.jsontutorial.beans.Company;
import org.o7planning.jsontutorial.json.JsonWriterExample;
import java.io.StringWriter;
public class MainActivity extends AppCompatActivity {
private EditText outputText;
private Button button;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.outputText = (EditText)this.findViewById(R.id.editText);
this.button = (Button) this.findViewById(R.id.button);
this.button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
runExample(view);
}
});
}
public void runExample(View view) {
try {
StringWriter output = new StringWriter();
Company company = JsonWriterExample.createCompany();
JsonWriterExample.writeJsonStream(output, company);
String jsonText = output.toString();
outputText.setText(jsonText);
} catch(Exception e) {
outputText.setText(e.getMessage());
e.printStackTrace();
}
}
}
Laufen Sie die Applikation:
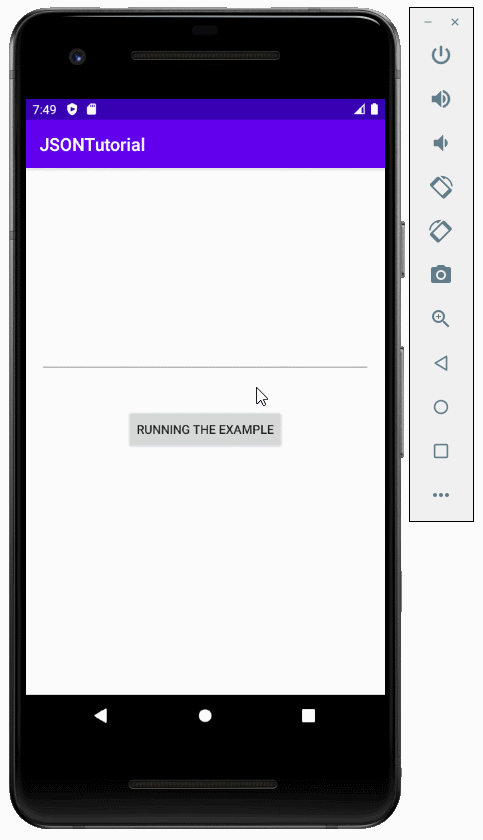
Anleitungen Android
- Konfigurieren Sie Android Emulator in Android Studio
- Die Anleitung zu Android ToggleButton
- Erstellen Sie einen einfachen File Finder Dialog in Android
- Die Anleitung zu Android TimePickerDialog
- Die Anleitung zu Android DatePickerDialog
- Was ist erforderlich, um mit Android zu beginnen?
- Installieren Sie Android Studio unter Windows
- Installieren Sie Intel® HAXM für Android Studio
- Die Anleitung zu Android AsyncTask
- Die Anleitung zu Android AsyncTaskLoader
- Die Anleitung zum Android für den Anfänger - Grundlegende Beispiele
- Woher weiß man die Telefonnummer von Android Emulator und ändere es
- Die Anleitung zu Android TextInputLayout
- Die Anleitung zu Android CardView
- Die Anleitung zu Android ViewPager2
- Holen Sie sich die Telefonnummer in Android mit TelephonyManager
- Die Anleitung zu Android Phone Calls
- Die Anleitung zu Android Wifi Scanning
- Die Anleitung zum Android 2D Game für den Anfänger
- Die Anleitung zu Android DialogFragment
- Die Anleitung zu Android CharacterPickerDialog
- Die Anleitung zum Android für den Anfänger - Hello Android
- Verwenden des Android Device File Explorer
- Aktivieren Sie USB Debugging auf einem Android-Gerät
- Die Anleitung zu Android UI Layouts
- Die Anleitung zu Android SMS
- Die Anleitung zu Android SQLite Database
- Die Anleitung zu Google Maps Android API
- Text zu Sprache in Android
- Die Anleitung zu Android Space
- Die Anleitung zu Android Toast
- Erstellen Sie einen benutzerdefinierten Android Toast
- Die Anleitung zu Android SnackBar
- Die Anleitung zu Android TextView
- Die Anleitung zu Android TextClock
- Die Anleitung zu Android EditText
- Die Anleitung zu Android TextWatcher
- Formatieren Sie die Kreditkartennummer mit Android TextWatcher
- Die Anleitung zu Android Clipboard
- Erstellen Sie einen einfachen File Chooser in Android
- Die Anleitung zu Android AutoCompleteTextView und MultiAutoCompleteTextView
- Die Anleitung zu Android ImageView
- Die Anleitung zu Android ImageSwitcher
- Die Anleitung zu Android ScrollView und HorizontalScrollView
- Die Anleitung zu Android WebView
- Die Anleitung zu Android SeekBar
- Die Anleitung zu Android Dialog
- Die Anleitung zu Android AlertDialog
- Die Anleitung zu Android RatingBar
- Die Anleitung zu Android ProgressBar
- Die Anleitung zu Android Spinner
- Die Anleitung zu Android Button
- Die Anleitung zu Android Switch
- Die Anleitung zu Android ImageButton
- Die Anleitung zu Android FloatingActionButton
- Die Anleitung zu Android CheckBox
- Die Anleitung zu Android RadioGroup und RadioButton
- Die Anleitung zu Android Chip und ChipGroup
- Verwenden Sie Image Asset und Icon Asset von Android Studio
- Richten Sie die SDCard für den Emulator ein
- ChipGroup und Chip Entry Beispiel
- Hinzufügen externer Bibliotheken zu Android Project in Android Studio
- Wie deaktiviere ich die Berechtigungen, die der Android-Anwendung bereits erteilt wurden?
- Wie entferne ich Anwendungen aus dem Android Emulator?
- Die Anleitung zu Android LinearLayout
- Die Anleitung zu Android TableLayout
- Die Anleitung zu Android FrameLayout
- Die Anleitung zu Android QuickContactBadge
- Die Anleitung zu Android StackView
- Die Anleitung zu Android Camera
- Die Anleitung zu Android MediaPlayer
- Die Anleitung zu Android VideoView
- Spielen Sie Sound-Effekte in Android mit SoundPool
- Die Anleitung zu Android Networking
- Die Anleitung zu Android JSON Parser
- Die Anleitung zu Android SharedPreferences
- Die Anleitung zu Android Internal Storage
- Die Anleitung zu Android External Storage
- Die Anleitung zu Android Intents
- Beispiel für eine explizite Android Intent, nennen Sie eine andere Intent
- Beispiel für implizite Android Intent, Öffnen Sie eine URL, senden Sie eine Email
- Die Anleitung zu Android Services
- Die Anleitung zu Android Notifications
- Die Anleitung zu Android DatePicker
- Die Anleitung zu Android TimePicker
- Die Anleitung zu Android Chronometer
- Die Anleitung zu Android OptionMenu
- Die Anleitung zu Android ContextMenu
- Die Anleitung zu Android PopupMenu
- Die Anleitung zu Android Fragment
- Die Anleitung zu Android ListView
- Android ListView mit Checkbox verwenden ArrayAdapter
- Die Anleitung zu Android GridView
Show More