Die Anleitung zu Android TextClock
1. Android TextClock
Im Android ist TextClock ein Sub-Klasse von TextView. Es wird benutzt um das aktuelle Datum und die Zeit vom System anzuzeigen.
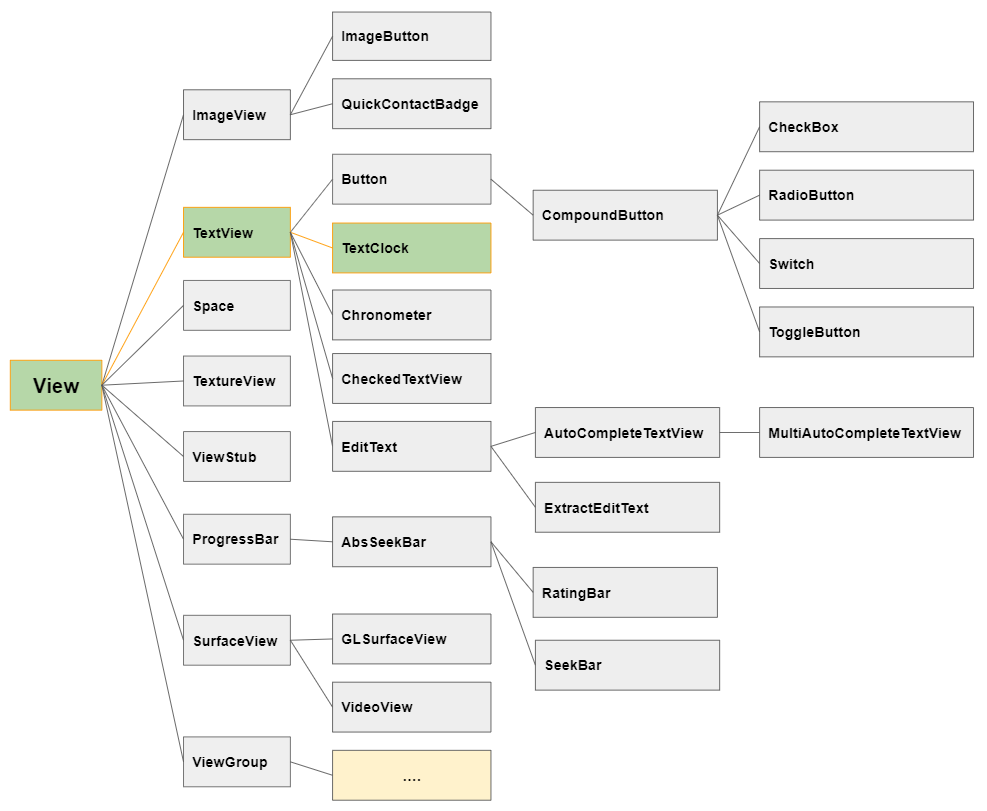
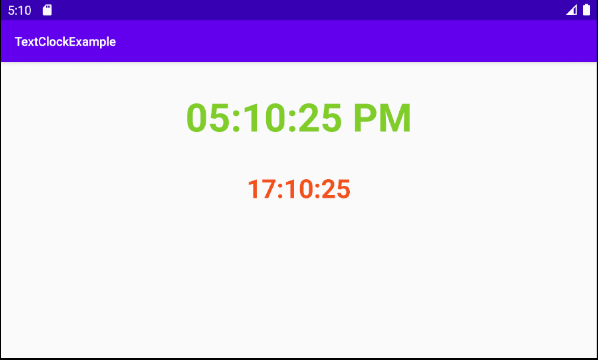
TextClock fordert API Level 17 (Android 4.2) oder neuer auf. Deshald wenn Sie TextClock in Ihrem Projekt benutzen möchten, sollen Sie den Wert von minSdkVersion in die Datei build.gradle (Module: app). Stellen Sie sicher, dass es gleich wie oder größer als 17 ist.
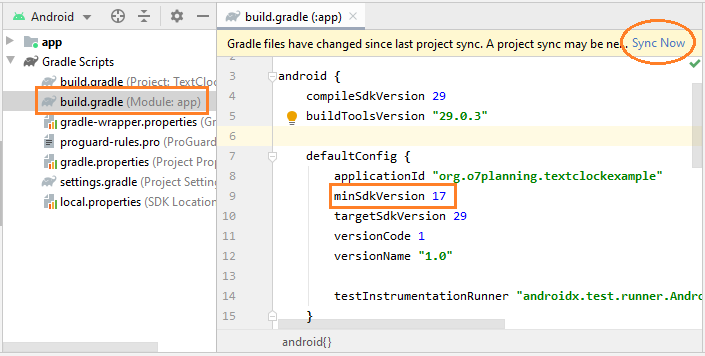
TextClock ist in Palette vom Design-Fenster nicht verfügbar, denn es ist vielleicht kein übliches Komponent. Deshalb brauchen Sie die Code XML zu benutzen danach in die Interface hinzufügen.
<!--
IMPORTANT NOTE:
You may get "Exception raised during rendering" error on design screen.
-->
<TextClock
android:id="@+id/textClock"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:format12Hour="hh:mm:ss a" />
Achtung: Sie können eine Fehlerbenachrichtung bekommen wenn Sie versuchen, die Interface mit der Anwesenheit von TextClock zu entwerfen. Das Problem wurde in Android Studio 3.x, 4.0 bestätigt. Einige Leuten haben die Reports nach Google zu Behandlung geschickt:
Exception raised during rendering.
java.lang.NullPointerException
at android.content.ContentResolver.registerContentObserver(ContentResolver.java:2263)
at android.widget.TextClock.registerObserver(TextClock.java:626)
at android.widget.TextClock.onAttachedToWindow(TextClock.java:545)
at android.view.View.dispatchAttachedToWindow(View.java:19575)
at android.view.ViewGroup.dispatchAttachedToWindow(ViewGroup.java:3437)
at android.view.ViewGroup.dispatchAttachedToWindow(ViewGroup.java:3437)
at android.view.ViewGroup.dispatchAttachedToWindow(ViewGroup.java:3437)
at android.view.ViewGroup.dispatchAttachedToWindow(ViewGroup.java:3437)
at android.view.AttachInfo_Accessor.setAttachInfo(AttachInfo_Accessor.java:42)
at com.android.layoutlib.bridge.impl.RenderSessionImpl.inflate(RenderSessionImpl.java:335)
at com.android.layoutlib.bridge.Bridge.createSession(Bridge.java:396)
at com.android.tools.idea.layoutlib.LayoutLibrary.createSession(LayoutLibrary.java:209)
at com.android.tools.idea.rendering.RenderTask.createRenderSession(RenderTask.java:608)
at com.android.tools.idea.rendering.RenderTask.lambda$inflate$6(RenderTask.java:734)
at java.util.concurrent.CompletableFuture$AsyncSupply.run(CompletableFuture.java:1590)
at java.util.concurrent.ThreadPoolExecutor.runWorker(ThreadPoolExecutor.java:1149)
at java.util.concurrent.ThreadPoolExecutor$Worker.run(ThreadPoolExecutor.java:624)
at java.lang.Thread.run(Thread.java:748)
Sowieso habe ich dieses Problem gelöst, indem ich eine Unterklasse von TextClock erstellt und verwendet habe (siehe mehr im Beispiel).
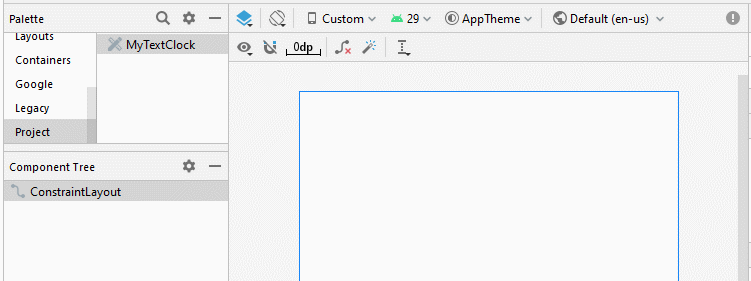
TextClock hat 2 Modus zur Zeitanzeige:
- 12Hour Mode (12Stunde-Modus)
- 24Hour Mode (24Stunde-Modus)
Zum ersten wird das Objekt TextClock das Method is24HourModeEnabled() aufrufen um zu prüfen, ob das Gerät des Benutzer das 24Stunden-Modus benutzt. Achtung: Der Benutzer kann 12Stunde Modus und 24Stunde Modus in Settings vom Gerät wechseln.
Es gibt 2 Möglichkeiten:
1 - Wenn das Gerät vom Benutzer im 24Stunde-Modus ist.
- TextClock zeigt die Zeit gemäß dem Format des von getFormat24Hour() zurückgegebenen Werts an, falls sie nicht null ist.
- Andernfalls wird die Zeit gemäß dem Format des von getFormat12Hour() zurückgegebenen Werts angezeigt, falls sie nicht null ist.
- Andernfalls wird die Zeit gemäß dem Standardformat "h:mm a" angezeigt.
2 - Wenn der Gerät vom Benutzer in dem 12Stunde Modus ist.
- TextClock zeigt die Zeit gemäß dem Format des von getFormat12Hour() zurückgegebenen Werts an, falls sie nicht null ist.
- Andernfalls wird die Zeit gemäß dem Format des von getFormat24Hour() zurückgegebenen Werts angezeigt, falls sie nicht null ist.
- Andernfalls wird die Zeit gemäß dem Standardformat, bsw. "h:mm a" angezeigt.
2. Das Beispiel vom TextClock
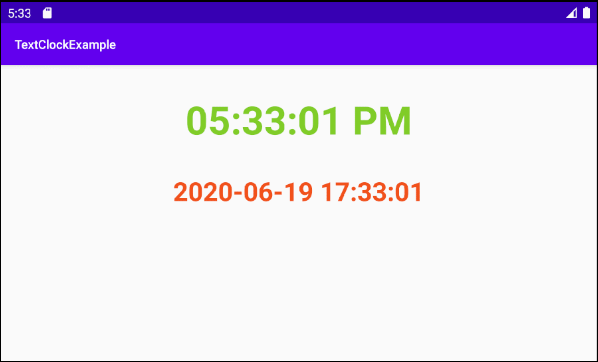
OK, Im Android Studio erstellen Sie ein neues Projekt:
- File > New > New Project > Empty Activity
- Name: TextClockExample
- Package name: org.o7planning.textclockexample
- Language: Java
TextClock fordert API Level 17 (Android 4.2) oder später auf. Deshalb wenn Sie TextClock in Ihrem Projekt benutzen möchten, sollen Sie den Wert von minSdkVersion in die Datei build.gradle (Module: app) ändern. Stellen Sie sicher, dass es gleich wie oder größer als 17 ist.
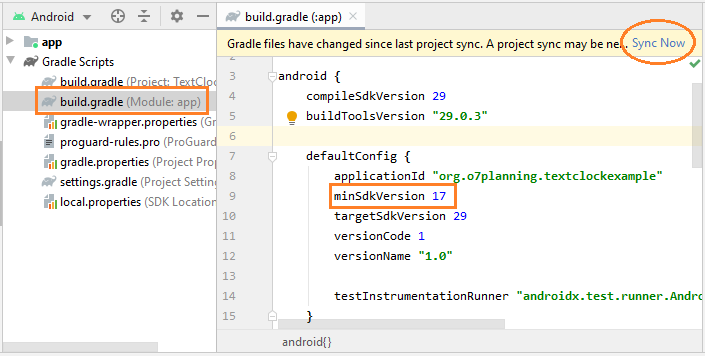
Wie oben erwähnt, erhalten Sie möglicherweise eine Fehlerbenachrichtigung, wenn Sie versuchen, die Schnittstelle in Anwesenheit von TextClock zu entwerfen. Möglicherweise wird das Problem in späteren Versionen von Android Studio von Google behoben. Um dieses Problem zu lösen, erstellen wir zunächst die Klasse MyTextClock, die sich von TextClock aus erstreckt, und verwenden sie dann.
MyTextClock.java
package org.o7planning.textclockexample;
import android.content.Context;
import android.os.Build;
import android.util.AttributeSet;
import android.widget.TextClock;
import androidx.annotation.RequiresApi;
public class MyTextClock extends TextClock {
public MyTextClock(Context context) {
super(context);
//
this.setDesigningText();
}
public MyTextClock(Context context, AttributeSet attrs) {
super(context, attrs);
//
this.setDesigningText();
}
public MyTextClock(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
//
this.setDesigningText();
}
@RequiresApi(api = Build.VERSION_CODES.LOLLIPOP)
public MyTextClock(Context context, AttributeSet attrs, int defStyleAttr, int defStyleRes) {
super(context, attrs, defStyleAttr, defStyleRes);
//
this.setDesigningText();
}
private void setDesigningText() {
// The default text is displayed when designing the interface.
this.setText("11:30:00");
}
//
// Fix error: Exception raised during rendering.
//
// java.lang.NullPointerException
// at android.content.ContentResolver.registerContentObserver(ContentResolver.java:2263)
// at android.widget.TextClock.registerObserver(TextClock.java:626)
// at android.widget.TextClock.onAttachedToWindow(TextClock.java:545)
// at android.view.View.dispatchAttachedToWindow(View.java:19575)
// at android.view.ViewGroup.dispatchAttachedToWindow(ViewGroup.java:3437)
// at android.view.ViewGroup.dispatchAttachedToWindow(ViewGroup.java:3437)
// at android.view.ViewGroup.dispatchAttachedToWindow(ViewGroup.java:3437)
// at android.view.ViewGroup.dispatchAttachedToWindow(ViewGroup.java:3437)
// at android.view.AttachInfo_Accessor.setAttachInfo(AttachInfo_Accessor.java:42)
// at com.android.layoutlib.bridge.impl.RenderSessionImpl.inflate(RenderSessionImpl.java:335)
// at com.android.layoutlib.bridge.Bridge.createSession(Bridge.java:396)
// at com.android.tools.idea.layoutlib.LayoutLibrary.createSession(LayoutLibrary.java:209)
// at com.android.tools.idea.rendering.RenderTask.createRenderSession(RenderTask.java:608)
// at com.android.tools.idea.rendering.RenderTask.lambda$inflate$6(RenderTask.java:734)
// at java.util.concurrent.CompletableFuture$AsyncSupply.run(CompletableFuture.java:1590)
// at java.util.concurrent.ThreadPoolExecutor.runWorker(ThreadPoolExecutor.java:1149)
// at java.util.concurrent.ThreadPoolExecutor$Worker.run(ThreadPoolExecutor.java:624)
// at java.lang.Thread.run(Thread.java:748)
@Override
protected void onAttachedToWindow() {
try {
super.onAttachedToWindow();
} catch(Exception e) {
}
}
}
Entwerfen Sie die Interface der Anwendung:
Achtung: Sie brauchen Re-Build vom Projekt machen und dann werden Sie sehen, dass MyTextClock in Palette vom Design-Fenster anzeigt.
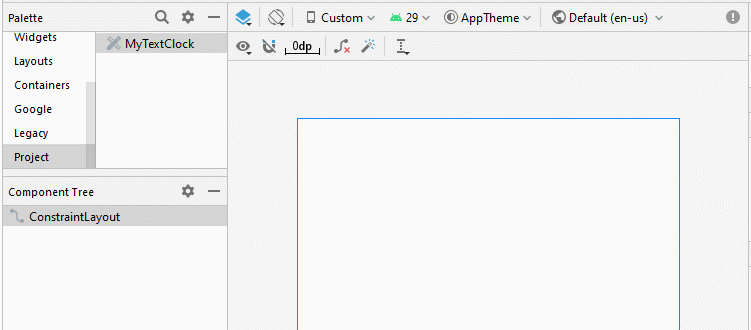
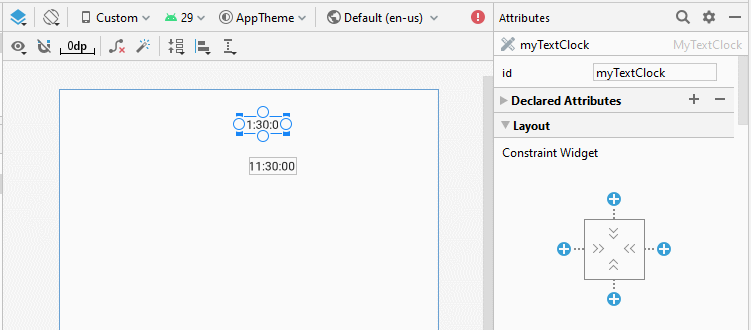
Legen Sie textSize, textStyle, textColor, format12Hour, format24Hour für TextClock fest.
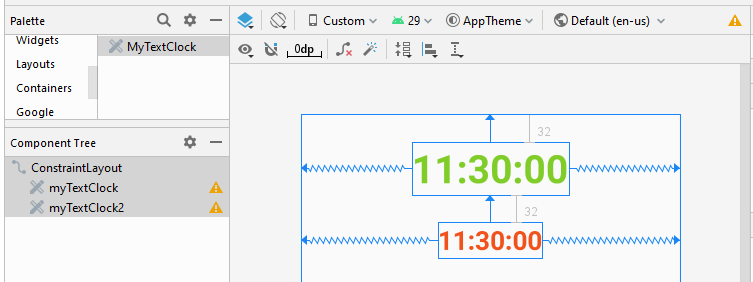
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<!-- 12 Hour Mode -->
<org.o7planning.textclockexample.MyTextClock
android:id="@+id/myTextClock"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="32dp"
android:format12Hour="hh:mm:ss a"
android:format24Hour="@null"
android:textColor="#80CC28"
android:textSize="45dp"
android:textStyle="bold"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<!-- 24 Hour Mode -->
<org.o7planning.textclockexample.MyTextClock
android:id="@+id/myTextClock2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="32dp"
android:format12Hour="@null"
android:format24Hour="yyyy-MM-dd HH:mm:ss"
android:textColor="#F1511B"
android:textSize="30dp"
android:textStyle="bold"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/myTextClock" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package org.o7planning.textclockexample;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.widget.TextClock;
public class MainActivity extends AppCompatActivity {
private TextClock textClock;
private TextClock textClock2;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.textClock = (TextClock) this.findViewById(R.id.myTextClock);
this.textClock2 = (TextClock) this.findViewById(R.id.myTextClock2);
// Disable 24 Hour mode (To use 12 Hour mode).
// (Make sure getFormat12Hour() is not null).
this.textClock.setFormat24Hour(null);
// Disable 12 Hour mode (To use 24 Hour mode).
// (Make sure getFormat24Hour() is not null).
this.textClock2.setFormat12Hour(null);
}
}
Anleitungen Android
- Konfigurieren Sie Android Emulator in Android Studio
- Die Anleitung zu Android ToggleButton
- Erstellen Sie einen einfachen File Finder Dialog in Android
- Die Anleitung zu Android TimePickerDialog
- Die Anleitung zu Android DatePickerDialog
- Was ist erforderlich, um mit Android zu beginnen?
- Installieren Sie Android Studio unter Windows
- Installieren Sie Intel® HAXM für Android Studio
- Die Anleitung zu Android AsyncTask
- Die Anleitung zu Android AsyncTaskLoader
- Die Anleitung zum Android für den Anfänger - Grundlegende Beispiele
- Woher weiß man die Telefonnummer von Android Emulator und ändere es
- Die Anleitung zu Android TextInputLayout
- Die Anleitung zu Android CardView
- Die Anleitung zu Android ViewPager2
- Holen Sie sich die Telefonnummer in Android mit TelephonyManager
- Die Anleitung zu Android Phone Calls
- Die Anleitung zu Android Wifi Scanning
- Die Anleitung zum Android 2D Game für den Anfänger
- Die Anleitung zu Android DialogFragment
- Die Anleitung zu Android CharacterPickerDialog
- Die Anleitung zum Android für den Anfänger - Hello Android
- Verwenden des Android Device File Explorer
- Aktivieren Sie USB Debugging auf einem Android-Gerät
- Die Anleitung zu Android UI Layouts
- Die Anleitung zu Android SMS
- Die Anleitung zu Android SQLite Database
- Die Anleitung zu Google Maps Android API
- Text zu Sprache in Android
- Die Anleitung zu Android Space
- Die Anleitung zu Android Toast
- Erstellen Sie einen benutzerdefinierten Android Toast
- Die Anleitung zu Android SnackBar
- Die Anleitung zu Android TextView
- Die Anleitung zu Android TextClock
- Die Anleitung zu Android EditText
- Die Anleitung zu Android TextWatcher
- Formatieren Sie die Kreditkartennummer mit Android TextWatcher
- Die Anleitung zu Android Clipboard
- Erstellen Sie einen einfachen File Chooser in Android
- Die Anleitung zu Android AutoCompleteTextView und MultiAutoCompleteTextView
- Die Anleitung zu Android ImageView
- Die Anleitung zu Android ImageSwitcher
- Die Anleitung zu Android ScrollView und HorizontalScrollView
- Die Anleitung zu Android WebView
- Die Anleitung zu Android SeekBar
- Die Anleitung zu Android Dialog
- Die Anleitung zu Android AlertDialog
- Die Anleitung zu Android RatingBar
- Die Anleitung zu Android ProgressBar
- Die Anleitung zu Android Spinner
- Die Anleitung zu Android Button
- Die Anleitung zu Android Switch
- Die Anleitung zu Android ImageButton
- Die Anleitung zu Android FloatingActionButton
- Die Anleitung zu Android CheckBox
- Die Anleitung zu Android RadioGroup und RadioButton
- Die Anleitung zu Android Chip und ChipGroup
- Verwenden Sie Image Asset und Icon Asset von Android Studio
- Richten Sie die SDCard für den Emulator ein
- ChipGroup und Chip Entry Beispiel
- Hinzufügen externer Bibliotheken zu Android Project in Android Studio
- Wie deaktiviere ich die Berechtigungen, die der Android-Anwendung bereits erteilt wurden?
- Wie entferne ich Anwendungen aus dem Android Emulator?
- Die Anleitung zu Android LinearLayout
- Die Anleitung zu Android TableLayout
- Die Anleitung zu Android FrameLayout
- Die Anleitung zu Android QuickContactBadge
- Die Anleitung zu Android StackView
- Die Anleitung zu Android Camera
- Die Anleitung zu Android MediaPlayer
- Die Anleitung zu Android VideoView
- Spielen Sie Sound-Effekte in Android mit SoundPool
- Die Anleitung zu Android Networking
- Die Anleitung zu Android JSON Parser
- Die Anleitung zu Android SharedPreferences
- Die Anleitung zu Android Internal Storage
- Die Anleitung zu Android External Storage
- Die Anleitung zu Android Intents
- Beispiel für eine explizite Android Intent, nennen Sie eine andere Intent
- Beispiel für implizite Android Intent, Öffnen Sie eine URL, senden Sie eine Email
- Die Anleitung zu Android Services
- Die Anleitung zu Android Notifications
- Die Anleitung zu Android DatePicker
- Die Anleitung zu Android TimePicker
- Die Anleitung zu Android Chronometer
- Die Anleitung zu Android OptionMenu
- Die Anleitung zu Android ContextMenu
- Die Anleitung zu Android PopupMenu
- Die Anleitung zu Android Fragment
- Die Anleitung zu Android ListView
- Android ListView mit Checkbox verwenden ArrayAdapter
- Die Anleitung zu Android GridView
Show More