Die Anleitung zu Android SharedPreferences
1. Was ist SharedPreferences?
Sehen Sie eine Situation um SharedPreferences zu kennen:
Sie spielen gerade ein Game auf Android. Vor dem Game-spielen wählen Sie die Parameter der Game, zum Beispiel die Helligkeit des Games, die Volume und die Schwierigkeit. Nach dem Spielen schließen Sie das Game und können das Game weiter spielen.SharedPreferences genehmigt Ihnen bei der Speicherung der von Ihnen gebildeteten Parameter, damit Sie bei dem weiteren Spielen von der vorherigen Parameter nicht wieder einstellen .
Sie spielen gerade ein Game auf Android. Vor dem Game-spielen wählen Sie die Parameter der Game, zum Beispiel die Helligkeit des Games, die Volume und die Schwierigkeit. Nach dem Spielen schließen Sie das Game und können das Game weiter spielen.SharedPreferences genehmigt Ihnen bei der Speicherung der von Ihnen gebildeteten Parameter, damit Sie bei dem weiteren Spielen von der vorherigen Parameter nicht wieder einstellen .
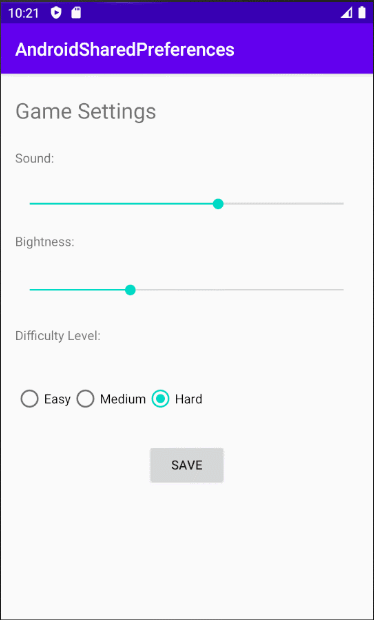
SharedPreferences speichert die Raw-Daten in der Form von dem Schlüssel und Wert (key-value) in der Datei der Applikation. Sie können ein der privaten Speicherungsmodus wählen, die die anderen Applikation in dieser Datei nicht zugreifen. Deshalb ist sie sicher .
2. Das Beispiel mit SharedPreferences
Schauen Sie das Bild der Applikationsinterface vor
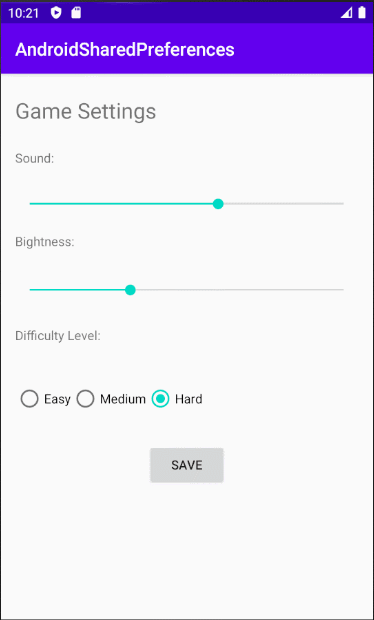
Erstellen Sie ein Projekt mit dem Name von AndroidSharedPreferences:
- File > New > New Project > Empty Activity
- Name: AndroidSharedPreferences
- Package name: org.o7planning.androidsharedpreferences
- Language: Java
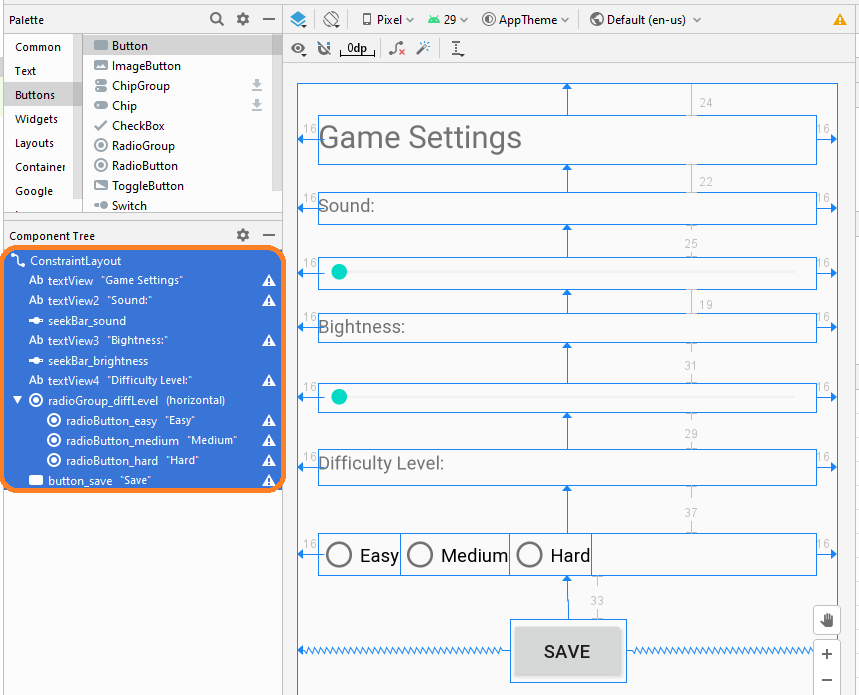
Wenn Sie sich für die Design-Schritten der Anwendungsinterface interessieren, sehen Sie bitte den Anhang am Ende vom Artikel
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="37dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="24dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Game Settings"
android:textSize="24sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<TextView
android:id="@+id/textView2"
android:layout_width="0dp"
android:layout_height="24dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="22dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Sound:"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView" />
<SeekBar
android:id="@+id/seekBar_sound"
android:layout_width="0dp"
android:layout_height="24dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="25dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView2" />
<TextView
android:id="@+id/textView3"
android:layout_width="0dp"
android:layout_height="22dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="19dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Bightness:"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/seekBar_sound" />
<SeekBar
android:id="@+id/seekBar_brightness"
android:layout_width="0dp"
android:layout_height="22dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="31dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView3" />
<TextView
android:id="@+id/textView4"
android:layout_width="0dp"
android:layout_height="27dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="29dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Difficulty Level:"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/seekBar_brightness" />
<RadioGroup
android:id="@+id/radioGroup_diffLevel"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="37dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:orientation="horizontal"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView4">
<RadioButton
android:id="@+id/radioButton_easy"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Easy" />
<RadioButton
android:id="@+id/radioButton_medium"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Medium" />
<RadioButton
android:id="@+id/radioButton_hard"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hard" />
</RadioGroup>
<Button
android:id="@+id/button_save"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="33dp"
android:text="Save"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/radioGroup_diffLevel" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package org.o7planning.androidsharedpreferences;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Context;
import android.content.SharedPreferences;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.RadioButton;
import android.widget.RadioGroup;
import android.widget.SeekBar;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
private SeekBar seekBarSound ;
private SeekBar seekBarBrightness;
private RadioGroup radioGroupDiffLevel;
private RadioButton radioButtonEasy;
private RadioButton radioButtonMedium;
private RadioButton radioButtonHard;
private Button buttonSave;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.seekBarBrightness= (SeekBar)this.findViewById(R.id.seekBar_brightness);
this.seekBarSound= (SeekBar)this.findViewById(R.id.seekBar_sound);
this.seekBarBrightness.setMax(100);
this.seekBarSound.setMax(100);
this.radioGroupDiffLevel= (RadioGroup) this.findViewById(R.id.radioGroup_diffLevel);
this.radioButtonEasy=(RadioButton) this.findViewById(R.id.radioButton_easy);
this.radioButtonMedium = (RadioButton)this.findViewById(R.id.radioButton_medium);
this.radioButtonHard=(RadioButton) this.findViewById(R.id.radioButton_hard);
this.buttonSave = (Button) this.findViewById(R.id.button_save);
this.buttonSave.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
MainActivity.this.doSave(view);
}
});
// Load saved game setting.
this.loadGameSetting();
}
private void loadGameSetting() {
SharedPreferences sharedPreferences= this.getSharedPreferences("gameSetting", Context.MODE_PRIVATE);
if(sharedPreferences!= null) {
int brightness = sharedPreferences.getInt("brightness", 90);
int sound = sharedPreferences.getInt("sound",95);
int checkedRadioButtonId = sharedPreferences.getInt("checkedRadioButtonId", R.id.radioButton_medium);
this.seekBarSound.setProgress(sound);
this.seekBarBrightness.setProgress(brightness);
this.radioGroupDiffLevel.check(checkedRadioButtonId);
} else {
this.radioGroupDiffLevel.check(R.id.radioButton_medium);
Toast.makeText(this,"Use the default game setting",Toast.LENGTH_LONG).show();
}
}
// Called when user click to Save button.
public void doSave(View view) {
// The created file can only be accessed by the calling application
// (or all applications sharing the same user ID).
SharedPreferences sharedPreferences= this.getSharedPreferences("gameSetting", Context.MODE_PRIVATE);
SharedPreferences.Editor editor = sharedPreferences.edit();
editor.putInt("brightness", this.seekBarBrightness.getProgress());
editor.putInt("sound", this.seekBarSound.getProgress());
// Checked RadioButton ID.
int checkedRadioButtonId = radioGroupDiffLevel.getCheckedRadioButtonId();
editor.putInt("checkedRadioButtonId", checkedRadioButtonId);
// Save.
editor.apply();
Toast.makeText(this,"Game Setting saved!",Toast.LENGTH_LONG).show();
}
}
Nach der Speicherung können Sie die Applikation schließen und wieder sie öffnen. Die Parameter, die von Ihnen gespeichert werden, werden automatisch für das Game gesetzt
Anleitungen Android
- Konfigurieren Sie Android Emulator in Android Studio
- Die Anleitung zu Android ToggleButton
- Erstellen Sie einen einfachen File Finder Dialog in Android
- Die Anleitung zu Android TimePickerDialog
- Die Anleitung zu Android DatePickerDialog
- Was ist erforderlich, um mit Android zu beginnen?
- Installieren Sie Android Studio unter Windows
- Installieren Sie Intel® HAXM für Android Studio
- Die Anleitung zu Android AsyncTask
- Die Anleitung zu Android AsyncTaskLoader
- Die Anleitung zum Android für den Anfänger - Grundlegende Beispiele
- Woher weiß man die Telefonnummer von Android Emulator und ändere es
- Die Anleitung zu Android TextInputLayout
- Die Anleitung zu Android CardView
- Die Anleitung zu Android ViewPager2
- Holen Sie sich die Telefonnummer in Android mit TelephonyManager
- Die Anleitung zu Android Phone Calls
- Die Anleitung zu Android Wifi Scanning
- Die Anleitung zum Android 2D Game für den Anfänger
- Die Anleitung zu Android DialogFragment
- Die Anleitung zu Android CharacterPickerDialog
- Die Anleitung zum Android für den Anfänger - Hello Android
- Verwenden des Android Device File Explorer
- Aktivieren Sie USB Debugging auf einem Android-Gerät
- Die Anleitung zu Android UI Layouts
- Die Anleitung zu Android SMS
- Die Anleitung zu Android SQLite Database
- Die Anleitung zu Google Maps Android API
- Text zu Sprache in Android
- Die Anleitung zu Android Space
- Die Anleitung zu Android Toast
- Erstellen Sie einen benutzerdefinierten Android Toast
- Die Anleitung zu Android SnackBar
- Die Anleitung zu Android TextView
- Die Anleitung zu Android TextClock
- Die Anleitung zu Android EditText
- Die Anleitung zu Android TextWatcher
- Formatieren Sie die Kreditkartennummer mit Android TextWatcher
- Die Anleitung zu Android Clipboard
- Erstellen Sie einen einfachen File Chooser in Android
- Die Anleitung zu Android AutoCompleteTextView und MultiAutoCompleteTextView
- Die Anleitung zu Android ImageView
- Die Anleitung zu Android ImageSwitcher
- Die Anleitung zu Android ScrollView und HorizontalScrollView
- Die Anleitung zu Android WebView
- Die Anleitung zu Android SeekBar
- Die Anleitung zu Android Dialog
- Die Anleitung zu Android AlertDialog
- Die Anleitung zu Android RatingBar
- Die Anleitung zu Android ProgressBar
- Die Anleitung zu Android Spinner
- Die Anleitung zu Android Button
- Die Anleitung zu Android Switch
- Die Anleitung zu Android ImageButton
- Die Anleitung zu Android FloatingActionButton
- Die Anleitung zu Android CheckBox
- Die Anleitung zu Android RadioGroup und RadioButton
- Die Anleitung zu Android Chip und ChipGroup
- Verwenden Sie Image Asset und Icon Asset von Android Studio
- Richten Sie die SDCard für den Emulator ein
- ChipGroup und Chip Entry Beispiel
- Hinzufügen externer Bibliotheken zu Android Project in Android Studio
- Wie deaktiviere ich die Berechtigungen, die der Android-Anwendung bereits erteilt wurden?
- Wie entferne ich Anwendungen aus dem Android Emulator?
- Die Anleitung zu Android LinearLayout
- Die Anleitung zu Android TableLayout
- Die Anleitung zu Android FrameLayout
- Die Anleitung zu Android QuickContactBadge
- Die Anleitung zu Android StackView
- Die Anleitung zu Android Camera
- Die Anleitung zu Android MediaPlayer
- Die Anleitung zu Android VideoView
- Spielen Sie Sound-Effekte in Android mit SoundPool
- Die Anleitung zu Android Networking
- Die Anleitung zu Android JSON Parser
- Die Anleitung zu Android SharedPreferences
- Die Anleitung zu Android Internal Storage
- Die Anleitung zu Android External Storage
- Die Anleitung zu Android Intents
- Beispiel für eine explizite Android Intent, nennen Sie eine andere Intent
- Beispiel für implizite Android Intent, Öffnen Sie eine URL, senden Sie eine Email
- Die Anleitung zu Android Services
- Die Anleitung zu Android Notifications
- Die Anleitung zu Android DatePicker
- Die Anleitung zu Android TimePicker
- Die Anleitung zu Android Chronometer
- Die Anleitung zu Android OptionMenu
- Die Anleitung zu Android ContextMenu
- Die Anleitung zu Android PopupMenu
- Die Anleitung zu Android Fragment
- Die Anleitung zu Android ListView
- Android ListView mit Checkbox verwenden ArrayAdapter
- Die Anleitung zu Android GridView
Show More