Die Anleitung zu Android ScrollView und HorizontalScrollView
1. ScrollView und HorizontalScrollView
In Android ist ScrollView ein Layout-Typ. Es ist ein rechteckiger Container mit vertikaler Bildlaufleiste und kann eine andere Komponente enthalten, die größer als diese ist. Ähnlich wie ScrollView ist HorizontalScrollView ein Container mit horizontaler Bildlaufleiste.
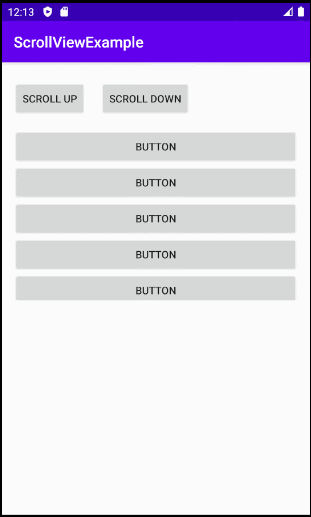
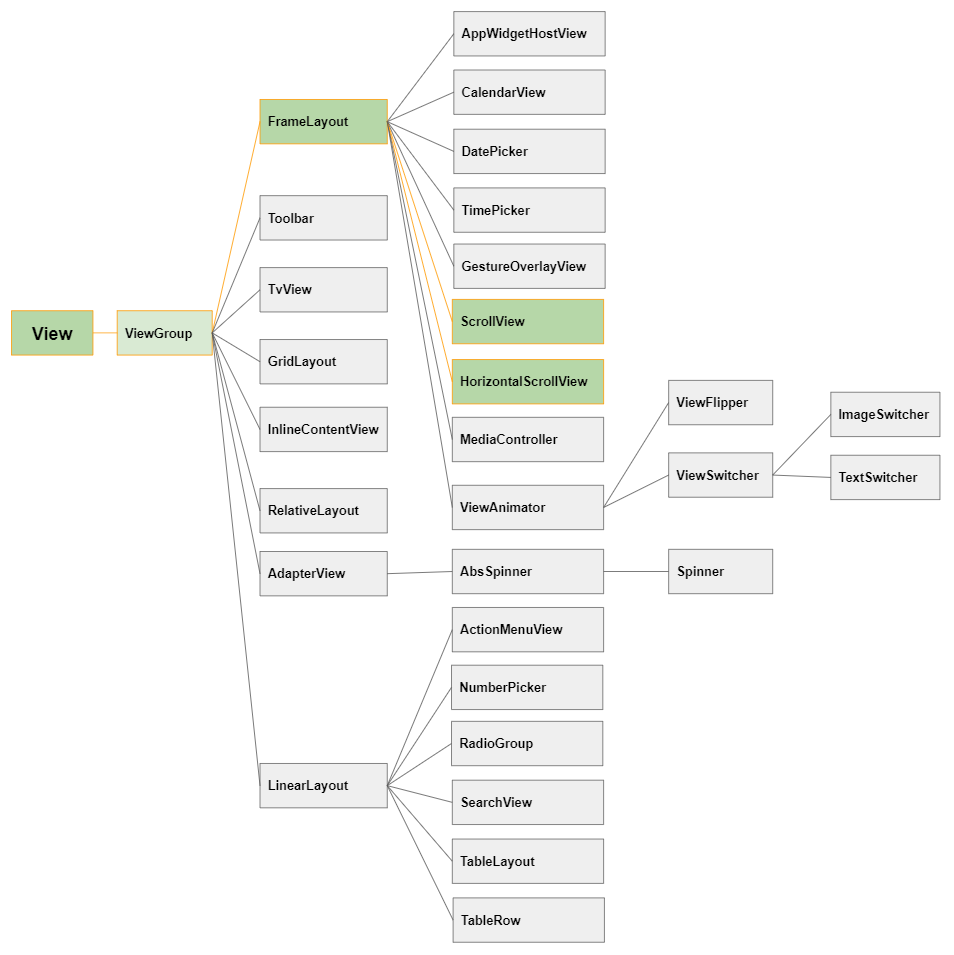
Im Allgemeinen sind ScrollView und HorizontalScrollView nützlich, sie werden verwendet, um großen Inhalt zu enthalten, und der Benutzer muss die Bildlaufleisten verwenden, um den Inhalt vollständig anzeigen zu können.
ScrollView und HorizontalScrollView kann nur eine direkte untergeordnete Komponente enthalten, daher ist das untergeordnete Element normalerweise ein anderer Container, der eine oder mehrere Unterkomponenten enthalten kann.
Wenn Sie in Android Studio eine ScrollView (oder HorizontalScrollView) in die Interface ziehen und ablegen, wird sie automatisch zu einer Unterkomponente, LinearLayout, hinzugefügt. Sie können diese Unterkomponente löschen, um eine andere Unterkomponente zu verwenden.
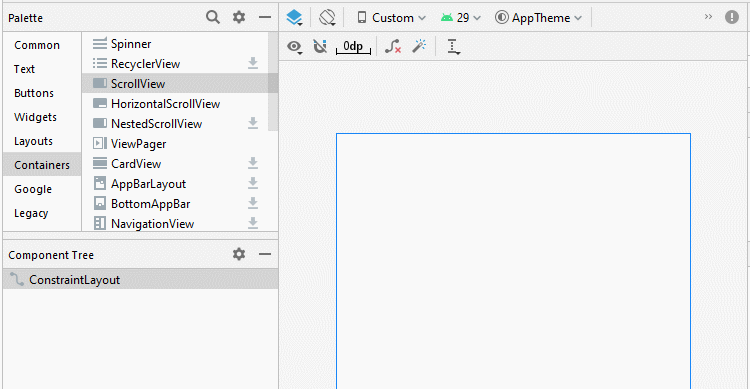
Sie sollten ScrollView besser nicht mit ListView- oder GridView-Komponenten kombinieren, da beide bereits über eigene vertikale Bildlaufleisten verfügen.
2. Ein Beispiel von ScrollView
In diesem Beispiel hat ein vertikales LinearLayout mehrere untergeordnete Komponenten. Wie deutlich zu sehen ist, braucht es einen Raum mit ausreichender Höhe. Die Bildschirmgröße ist jedoch begrenzt, daher ist es wichtig, in einer ScrollView platziert zu werden.
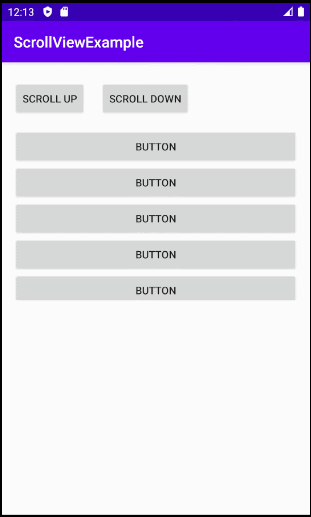
In diesem Beispiel werden einige Schritte zum Entwerfen der Interface von der Anwendung gezeigt:
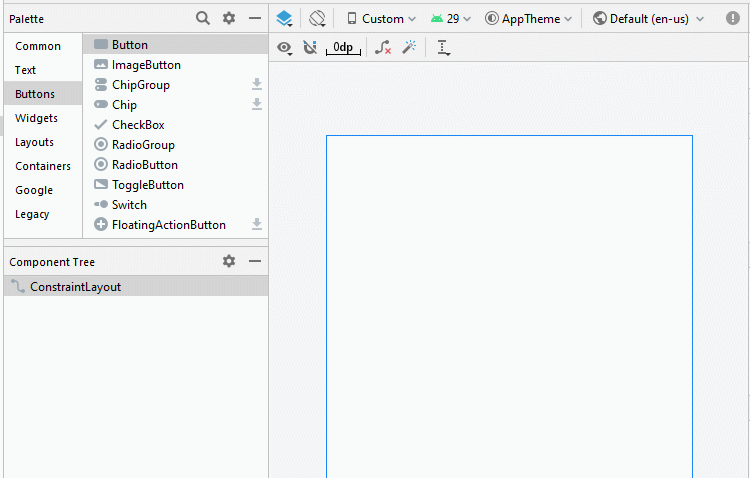
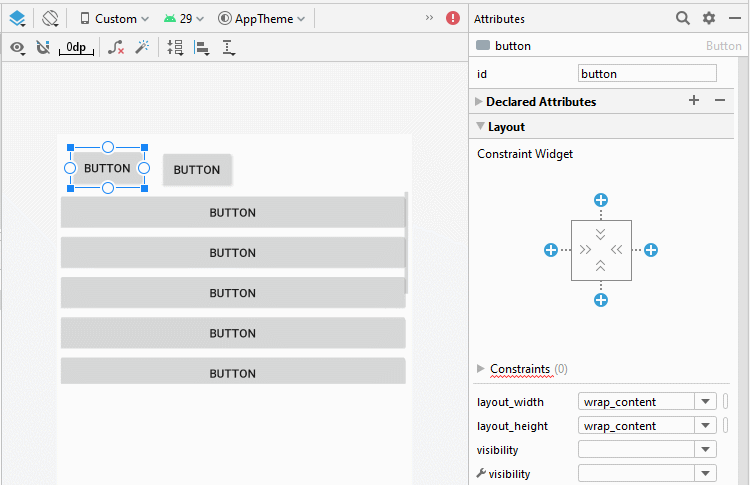
Legen Sie ID, Text für die Komponenten in die Interface fest:
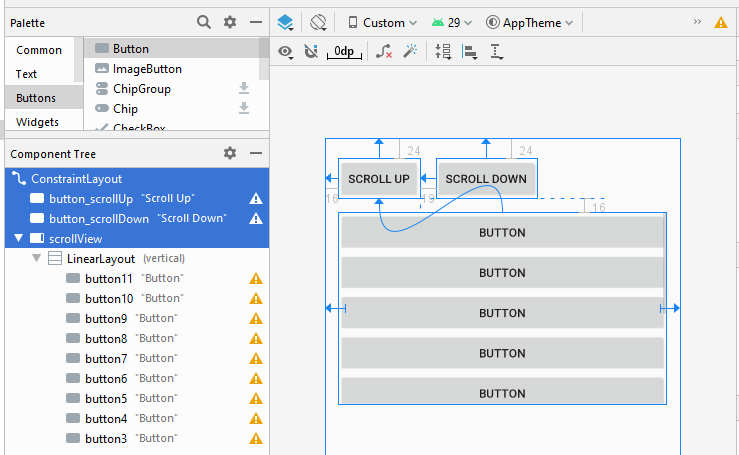
main_activity.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/button_scrollUp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="24dp"
android:text="Scroll Up"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button_scrollDown"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="19dp"
android:layout_marginLeft="19dp"
android:layout_marginTop="24dp"
android:text="Scroll Down"
app:layout_constraintStart_toEndOf="@+id/button_scrollUp"
app:layout_constraintTop_toTopOf="parent" />
<ScrollView
android:id="@+id/scrollView"
android:layout_width="0dp"
android:layout_height="229dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button_scrollUp">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<Button
android:id="@+id/button11"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Button" />
<Button
android:id="@+id/button10"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Button" />
<Button
android:id="@+id/button9"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Button" />
<Button
android:id="@+id/button8"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Button" />
<Button
android:id="@+id/button7"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Button" />
<Button
android:id="@+id/button6"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Button" />
<Button
android:id="@+id/button5"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Button" />
<Button
android:id="@+id/button4"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Button" />
<Button
android:id="@+id/button3"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Button" />
</LinearLayout>
</ScrollView>
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package com.example.scrollviewexample;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.ScrollView;
public class MainActivity extends AppCompatActivity {
private ScrollView scrollView;
private Button buttonScrollUp;
private Button buttonScrollDown;
public static final int SCROLL_DELTA = 15; // Pixel.
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.scrollView = (ScrollView) this.findViewById(R.id.scrollView);
this.buttonScrollUp = (Button) this.findViewById(R.id.button_scrollUp);
this.buttonScrollDown = (Button) this.findViewById(R.id.button_scrollDown);
this.buttonScrollUp.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
doScrollUp();
}
});
this.buttonScrollDown.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
doScrollDown();
}
});
}
private void doScrollUp() {
int x = this.scrollView.getScrollX();
int y = this.scrollView.getScrollY();
if(y - SCROLL_DELTA >= 0) {
this.scrollView.scrollTo(x, y-SCROLL_DELTA);
}
}
private void doScrollDown() {
int maxAmount = scrollView.getMaxScrollAmount();
int x = this.scrollView.getScrollX();
int y = this.scrollView.getScrollY();
if(y + SCROLL_DELTA <= maxAmount) {
this.scrollView.scrollTo(x, y + SCROLL_DELTA);
}
}
}
3. Z.B HorizontalScrollView
In diesem Beispiel enthält ein horizontales LinearLayout viele untergeordnete Komponenten. Es braucht definitiv einen Raum mit ausreichender Breite. Wie Sie sehen können, ist die Bildschirmgröße des Geräts des Benutzers begrenzt, daher muss es in einer HorizontalScrollView platziert werden.
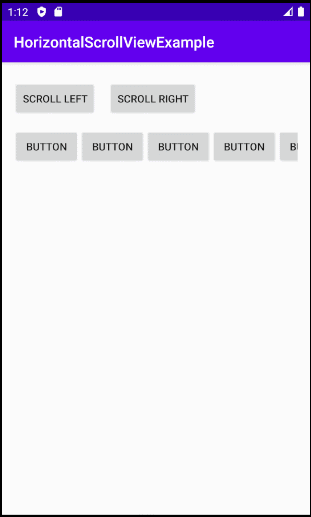
Entwerfen Sie die Interface:
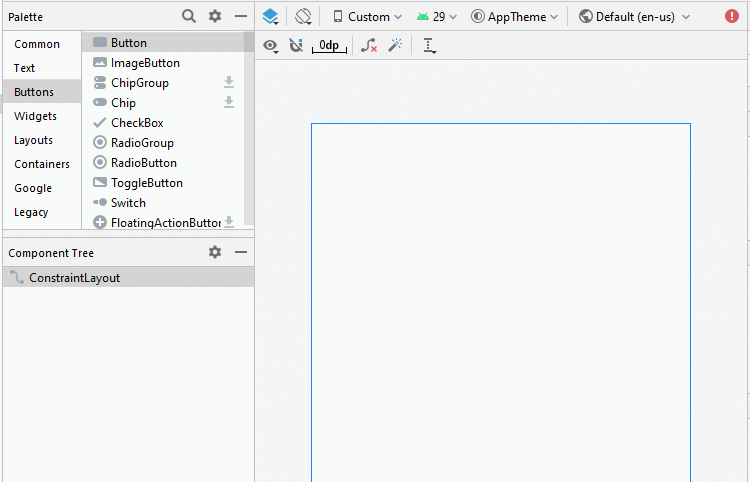
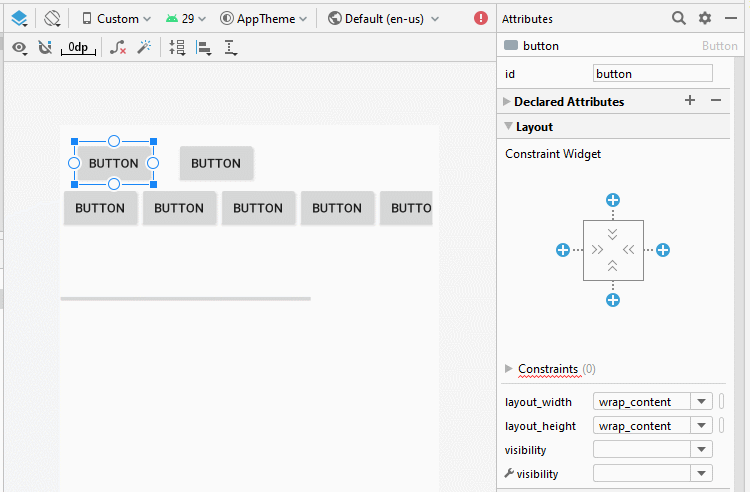
Legen Sie ID, Text für die Komponenten in die Interface fest:
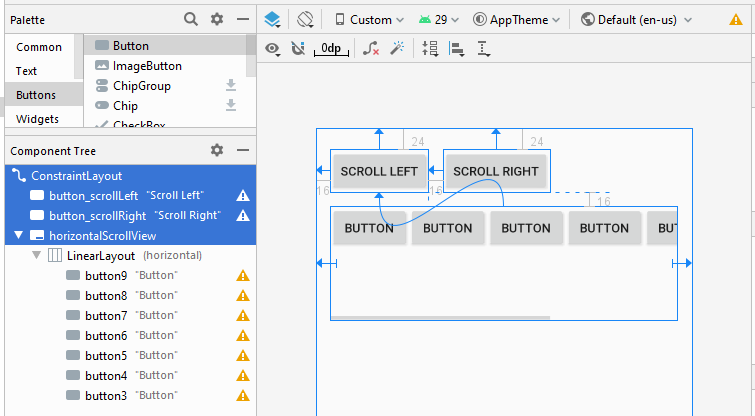
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/button_scrollLeft"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="24dp"
android:text="Scroll Left"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button_scrollRight"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="24dp"
android:text="Scroll Right"
app:layout_constraintStart_toEndOf="@+id/button_scrollLeft"
app:layout_constraintTop_toTopOf="parent" />
<HorizontalScrollView
android:id="@+id/horizontalScrollView"
android:layout_width="0dp"
android:layout_height="128dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button_scrollLeft">
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:orientation="horizontal">
<Button
android:id="@+id/button9"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Button" />
<Button
android:id="@+id/button8"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Button" />
<Button
android:id="@+id/button7"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Button" />
<Button
android:id="@+id/button6"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Button" />
<Button
android:id="@+id/button5"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Button" />
<Button
android:id="@+id/button4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Button" />
<Button
android:id="@+id/button3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Button" />
</LinearLayout>
</HorizontalScrollView>
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package com.example.horizontalscrollviewexample;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.HorizontalScrollView;
public class MainActivity extends AppCompatActivity {
private HorizontalScrollView horizontalScrollView;
private Button buttonScrollLeft;
private Button buttonScrollRight;
public static final int SCROLL_DELTA = 15; // Pixel.
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.horizontalScrollView = (HorizontalScrollView) this.findViewById(R.id.horizontalScrollView);
this.buttonScrollLeft = (Button) this.findViewById(R.id.button_scrollLeft);
this.buttonScrollRight = (Button) this.findViewById(R.id.button_scrollRight);
this.buttonScrollLeft.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
doScrollLeft();
}
});
this.buttonScrollRight.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
doScrollRight();
}
});
}
private void doScrollLeft() {
int x = this.horizontalScrollView.getScrollX();
int y = this.horizontalScrollView.getScrollY();
if(x - SCROLL_DELTA >= 0) {
this.horizontalScrollView.scrollTo(x - SCROLL_DELTA, y);
}
}
private void doScrollRight() {
int maxAmount = horizontalScrollView.getMaxScrollAmount();
int x = this.horizontalScrollView.getScrollX();
int y = this.horizontalScrollView.getScrollY();
if(x + SCROLL_DELTA <= maxAmount) {
this.horizontalScrollView.scrollTo(x + SCROLL_DELTA, y);
}
}
}
Anleitungen Android
- Konfigurieren Sie Android Emulator in Android Studio
- Die Anleitung zu Android ToggleButton
- Erstellen Sie einen einfachen File Finder Dialog in Android
- Die Anleitung zu Android TimePickerDialog
- Die Anleitung zu Android DatePickerDialog
- Was ist erforderlich, um mit Android zu beginnen?
- Installieren Sie Android Studio unter Windows
- Installieren Sie Intel® HAXM für Android Studio
- Die Anleitung zu Android AsyncTask
- Die Anleitung zu Android AsyncTaskLoader
- Die Anleitung zum Android für den Anfänger - Grundlegende Beispiele
- Woher weiß man die Telefonnummer von Android Emulator und ändere es
- Die Anleitung zu Android TextInputLayout
- Die Anleitung zu Android CardView
- Die Anleitung zu Android ViewPager2
- Holen Sie sich die Telefonnummer in Android mit TelephonyManager
- Die Anleitung zu Android Phone Calls
- Die Anleitung zu Android Wifi Scanning
- Die Anleitung zum Android 2D Game für den Anfänger
- Die Anleitung zu Android DialogFragment
- Die Anleitung zu Android CharacterPickerDialog
- Die Anleitung zum Android für den Anfänger - Hello Android
- Verwenden des Android Device File Explorer
- Aktivieren Sie USB Debugging auf einem Android-Gerät
- Die Anleitung zu Android UI Layouts
- Die Anleitung zu Android SMS
- Die Anleitung zu Android SQLite Database
- Die Anleitung zu Google Maps Android API
- Text zu Sprache in Android
- Die Anleitung zu Android Space
- Die Anleitung zu Android Toast
- Erstellen Sie einen benutzerdefinierten Android Toast
- Die Anleitung zu Android SnackBar
- Die Anleitung zu Android TextView
- Die Anleitung zu Android TextClock
- Die Anleitung zu Android EditText
- Die Anleitung zu Android TextWatcher
- Formatieren Sie die Kreditkartennummer mit Android TextWatcher
- Die Anleitung zu Android Clipboard
- Erstellen Sie einen einfachen File Chooser in Android
- Die Anleitung zu Android AutoCompleteTextView und MultiAutoCompleteTextView
- Die Anleitung zu Android ImageView
- Die Anleitung zu Android ImageSwitcher
- Die Anleitung zu Android ScrollView und HorizontalScrollView
- Die Anleitung zu Android WebView
- Die Anleitung zu Android SeekBar
- Die Anleitung zu Android Dialog
- Die Anleitung zu Android AlertDialog
- Die Anleitung zu Android RatingBar
- Die Anleitung zu Android ProgressBar
- Die Anleitung zu Android Spinner
- Die Anleitung zu Android Button
- Die Anleitung zu Android Switch
- Die Anleitung zu Android ImageButton
- Die Anleitung zu Android FloatingActionButton
- Die Anleitung zu Android CheckBox
- Die Anleitung zu Android RadioGroup und RadioButton
- Die Anleitung zu Android Chip und ChipGroup
- Verwenden Sie Image Asset und Icon Asset von Android Studio
- Richten Sie die SDCard für den Emulator ein
- ChipGroup und Chip Entry Beispiel
- Hinzufügen externer Bibliotheken zu Android Project in Android Studio
- Wie deaktiviere ich die Berechtigungen, die der Android-Anwendung bereits erteilt wurden?
- Wie entferne ich Anwendungen aus dem Android Emulator?
- Die Anleitung zu Android LinearLayout
- Die Anleitung zu Android TableLayout
- Die Anleitung zu Android FrameLayout
- Die Anleitung zu Android QuickContactBadge
- Die Anleitung zu Android StackView
- Die Anleitung zu Android Camera
- Die Anleitung zu Android MediaPlayer
- Die Anleitung zu Android VideoView
- Spielen Sie Sound-Effekte in Android mit SoundPool
- Die Anleitung zu Android Networking
- Die Anleitung zu Android JSON Parser
- Die Anleitung zu Android SharedPreferences
- Die Anleitung zu Android Internal Storage
- Die Anleitung zu Android External Storage
- Die Anleitung zu Android Intents
- Beispiel für eine explizite Android Intent, nennen Sie eine andere Intent
- Beispiel für implizite Android Intent, Öffnen Sie eine URL, senden Sie eine Email
- Die Anleitung zu Android Services
- Die Anleitung zu Android Notifications
- Die Anleitung zu Android DatePicker
- Die Anleitung zu Android TimePicker
- Die Anleitung zu Android Chronometer
- Die Anleitung zu Android OptionMenu
- Die Anleitung zu Android ContextMenu
- Die Anleitung zu Android PopupMenu
- Die Anleitung zu Android Fragment
- Die Anleitung zu Android ListView
- Android ListView mit Checkbox verwenden ArrayAdapter
- Die Anleitung zu Android GridView
Show More